How to Collaborate Effectively with Teams Using Git
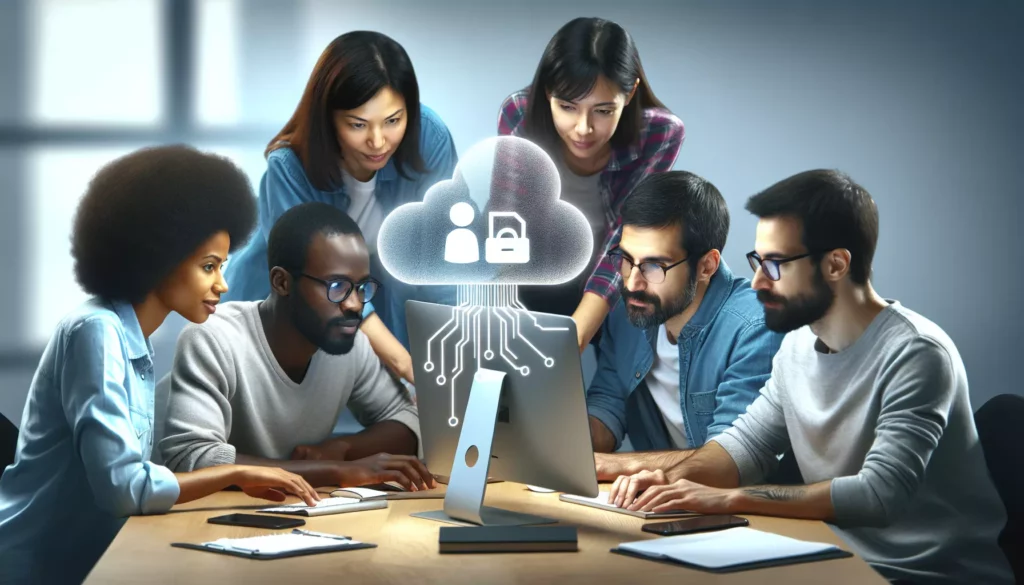
In the world of software development, collaboration is key to creating successful projects. As teams grow and projects become more complex, having a robust version control system becomes crucial. Git, the distributed version control system created by Linus Torvalds, has become the industry standard for managing code repositories and facilitating teamwork. In this comprehensive guide, we’ll explore how to collaborate effectively with teams using Git, covering everything from basic concepts to advanced workflows.
Understanding Git: The Basics
Before diving into collaboration techniques, it’s essential to have a solid understanding of Git fundamentals. Git is a distributed version control system that allows multiple developers to work on the same project simultaneously. It tracks changes in source code during software development and provides a history of all modifications.
Key Git Concepts
- Repository: A container for your project, including all files and their version history.
- Commit: A snapshot of your repository at a specific point in time.
- Branch: A parallel version of the repository that allows you to work on different features independently.
- Merge: The process of combining changes from different branches.
- Pull Request: A way to propose changes and request that someone review and merge them into a branch.
Setting Up Your Git Environment
To start collaborating with Git, you need to set up your local environment:
- Install Git on your machine (if not already installed).
- Configure your Git username and email:
git config --global user.name "Your Name"
git config --global user.email "your.email@example.com"
Creating and Cloning Repositories
To begin collaborating, you need a repository. You can either create a new one or clone an existing repository:
Creating a New Repository
mkdir my-project
cd my-project
git init
Cloning an Existing Repository
git clone https://github.com/username/repository.git
Branching Strategies for Effective Collaboration
Branching is a powerful feature in Git that allows teams to work on different aspects of a project simultaneously. Here are some popular branching strategies:
1. GitFlow
GitFlow is a branching model that defines a strict branching structure designed around project releases. It uses the following branch types:
- master: The main branch containing production-ready code.
- develop: The integration branch for features.
- feature/: Individual feature branches.
- release/: Branches for preparing new production releases.
- hotfix/: Branches for quickly patching production releases.
2. GitHub Flow
GitHub Flow is a simpler alternative to GitFlow, suitable for teams that deploy frequently:
- The main branch always contains deployable code.
- Create feature branches from main for new work.
- Open a pull request to propose changes.
- After review and approval, merge the feature branch into main.
- Deploy immediately after merging to main.
3. Trunk-Based Development
Trunk-Based Development is a branching strategy where developers collaborate on a single branch called “trunk” (usually main or master):
- Developers commit directly to the trunk branch.
- Feature toggles are used to hide incomplete features in production.
- Short-lived feature branches may be used for larger changes.
Best Practices for Collaborative Git Workflows
To ensure smooth collaboration using Git, follow these best practices:
1. Commit Often and Keep Commits Atomic
Make small, focused commits that address a single issue or feature. This makes it easier to understand changes, revert if necessary, and merge without conflicts.
2. Write Clear Commit Messages
Use descriptive commit messages that explain the why behind the changes, not just the what. Follow a consistent format, such as:
Short (50 chars or less) summary of changes
More detailed explanatory text, if necessary. Wrap it to about 72
characters or so. In some contexts, the first line is treated as the
subject of an email and the rest of the text as the body.
- Bullet points are okay, too
- Use a hyphen or asterisk followed by a single space
3. Use Feature Branches
Create a new branch for each feature or bug fix. This isolates changes and makes it easier to review and merge code.
git checkout -b feature/new-awesome-feature
4. Keep Your Branch Up to Date
Regularly update your feature branch with changes from the main branch to avoid conflicts:
git checkout main
git pull origin main
git checkout feature/your-feature
git merge main
5. Use Pull Requests for Code Review
Create pull requests to propose changes and facilitate code review. This allows team members to discuss the changes, suggest improvements, and ensure code quality before merging.
6. Leverage Git Hooks
Use Git hooks to automate tasks such as running tests, linting code, or formatting before commits or pushes. This helps maintain code quality and consistency across the team.
Resolving Conflicts
Conflicts occur when Git can’t automatically merge changes. To resolve conflicts:
- Open the conflicting file(s) in your text editor.
- Look for conflict markers (<<<<<<<, =======, >>>>>>>).
- Manually edit the file to resolve the conflict.
- Remove the conflict markers.
- Stage the resolved files:
git add <resolved-file>
- Complete the merge by committing:
git commit -m "Resolve merge conflict"
Advanced Git Techniques for Collaboration
1. Interactive Rebasing
Use interactive rebasing to clean up your commit history before merging:
git rebase -i HEAD~3 # Rebase the last 3 commits
2. Cherry-Picking
Apply specific commits from one branch to another:
git cherry-pick <commit-hash>
3. Git Submodules
Manage dependencies or split large projects into smaller repositories:
git submodule add <repository-url> <path>
4. Git Worktrees
Work on multiple branches simultaneously without switching:
git worktree add -b feature/new-feature ../new-feature main
Collaboration Tools and Platforms
Several platforms and tools can enhance Git collaboration:
1. GitHub
GitHub is a web-based hosting service for Git repositories that offers features like pull requests, issues, and project management tools.
2. GitLab
GitLab is a complete DevOps platform that provides source code management, CI/CD, and more.
3. Bitbucket
Bitbucket is a Git repository management solution that offers both cloud and server deployments.
4. GitKraken
GitKraken is a powerful Git GUI client that simplifies complex Git operations and enhances visualization of branches and commits.
Best Practices for Git Security
When collaborating with Git, it’s crucial to maintain security:
1. Use SSH Keys for Authentication
Instead of using passwords, set up SSH keys for secure authentication:
ssh-keygen -t ed25519 -C "your_email@example.com"
2. Enable Two-Factor Authentication (2FA)
Enable 2FA on your Git hosting platform to add an extra layer of security.
3. Be Careful with Sensitive Information
Never commit sensitive information like passwords or API keys. Use environment variables or secure secret management tools instead.
4. Sign Your Commits
Sign your commits to verify their authenticity:
git config --global user.signingkey <your-gpg-key-id>
git commit -S -m "Signed commit message"
Continuous Integration and Deployment with Git
Integrating Git with CI/CD pipelines can streamline your development process:
1. Set Up CI/CD Pipelines
Use tools like Jenkins, Travis CI, or GitHub Actions to automatically build, test, and deploy your code when changes are pushed to specific branches.
2. Implement Git Hooks for Pre-Commit Checks
Use pre-commit hooks to run tests, linters, or formatters before allowing commits:
#!/bin/sh
# Pre-commit hook to run tests
npm test
# If tests fail, prevent the commit
if [ $? -ne 0 ]; then
echo "Tests failed. Commit aborted."
exit 1
fi
3. Automate Release Management
Use Git tags and release branches to manage software versions and automate the release process.
Measuring and Improving Collaboration with Git Analytics
Analyze your team’s Git usage to identify areas for improvement:
1. Use Git Analytics Tools
Tools like GitPrime or GitHub Insights provide metrics on commit frequency, code review time, and overall team productivity.
2. Monitor Branch Lifetimes
Keep track of how long branches live before being merged. Long-lived branches can lead to integration challenges.
3. Analyze Commit Patterns
Look for patterns in commit frequency and size to identify potential bottlenecks or areas where the team might need support.
Conclusion
Effective collaboration using Git is essential for modern software development teams. By understanding Git fundamentals, implementing smart branching strategies, following best practices, and leveraging advanced techniques, teams can significantly improve their productivity and code quality.
Remember that Git is a powerful tool, but it’s just one part of the collaboration puzzle. Combine Git best practices with clear communication, well-defined processes, and a culture of continuous improvement to create a truly effective collaborative environment.
As you continue to work with Git and refine your team’s processes, you’ll discover new ways to optimize your workflow and enhance collaboration. Stay curious, keep learning, and don’t be afraid to experiment with different Git techniques to find what works best for your team.
Happy collaborating!