The Role of Continuous Integration in Version Control: Enhancing Software Development Workflows
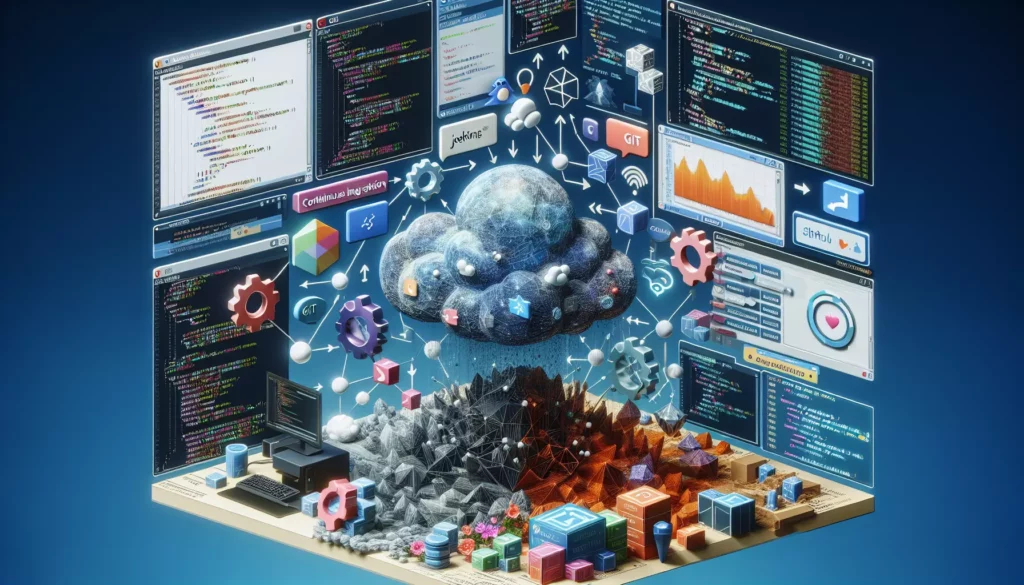
In the fast-paced world of software development, efficient and reliable processes are crucial for delivering high-quality products. Two key practices that have revolutionized the way development teams work are version control and continuous integration (CI). While version control systems help manage code changes and collaboration, continuous integration takes it a step further by automating the integration and testing of code changes. In this comprehensive guide, we’ll explore the role of continuous integration in version control and how it enhances software development workflows.
Understanding Version Control
Before diving into continuous integration, let’s briefly review what version control is and why it’s essential in modern software development.
What is Version Control?
Version control, also known as source control, is a system that helps track and manage changes to code over time. It allows developers to:
- Keep a history of code changes
- Collaborate with team members
- Revert to previous versions if needed
- Create branches for experimental features
- Merge different versions of code
Popular version control systems include Git, Subversion (SVN), and Mercurial.
Benefits of Version Control
Version control offers numerous advantages to development teams:
- Improved collaboration: Multiple developers can work on the same project simultaneously
- Code history: Easily track changes and identify when and why specific modifications were made
- Backup and recovery: Maintain a complete history of your codebase, allowing for easy recovery in case of data loss
- Experimentation: Create branches to test new features without affecting the main codebase
- Code review: Facilitate peer review processes through pull requests and code diffs
Introduction to Continuous Integration
Now that we understand version control, let’s explore continuous integration and its role in enhancing software development workflows.
What is Continuous Integration?
Continuous Integration (CI) is a software development practice where team members integrate their work frequently, usually multiple times a day. Each integration is verified by an automated build and automated tests to detect integration errors as quickly as possible.
Key Components of Continuous Integration
A typical CI system consists of the following components:
- Version Control System: Stores the source code and tracks changes
- CI Server: Monitors the version control system for changes and triggers builds
- Build Script: Automates the process of compiling code, running tests, and generating reports
- Notification System: Alerts team members about build status and test results
- Artifact Repository: Stores build artifacts and dependencies
The Role of Continuous Integration in Version Control
Continuous Integration plays a crucial role in enhancing version control practices and improving overall software development workflows. Let’s explore the key ways CI complements and extends version control:
1. Automated Build and Test Processes
One of the primary roles of CI in version control is to automate the build and test processes. When developers commit changes to the version control system, the CI server automatically:
- Fetches the latest code from the repository
- Compiles the code
- Runs unit tests, integration tests, and other automated checks
- Generates reports on build status and test results
This automation ensures that every code change is thoroughly tested, reducing the likelihood of introducing bugs or breaking existing functionality.
2. Early Detection of Integration Issues
By integrating code changes frequently and running automated tests, CI helps detect integration issues early in the development process. This early detection allows developers to:
- Identify and fix problems quickly
- Reduce the cost and time associated with bug fixes
- Maintain a stable and reliable codebase
3. Enforcing Coding Standards and Best Practices
CI systems can be configured to run static code analysis tools and linters as part of the build process. This helps enforce coding standards and best practices across the team by:
- Checking for code style violations
- Identifying potential security vulnerabilities
- Detecting code smells and suggesting improvements
By integrating these checks into the CI pipeline, teams can ensure consistent code quality and adherence to project standards.
4. Facilitating Code Reviews
CI systems can be integrated with version control platforms to enhance the code review process. When a developer creates a pull request, the CI system can:
- Automatically run builds and tests on the proposed changes
- Provide feedback on code quality and test coverage
- Block merges if certain criteria are not met (e.g., failing tests or low code coverage)
This integration streamlines the code review process and helps maintain high code quality standards.
5. Continuous Feedback Loop
CI provides a continuous feedback loop to developers by quickly reporting on the status of their code changes. This feedback helps developers:
- Identify and fix issues promptly
- Gain confidence in their changes
- Learn from mistakes and improve their coding practices
6. Simplifying Release Management
CI plays a crucial role in simplifying release management by:
- Automating the build and packaging of release artifacts
- Ensuring that only tested and approved code is deployed
- Facilitating the creation of release branches and tags in version control
This automation reduces the risk of human error in the release process and makes it easier to maintain multiple release versions.
Implementing Continuous Integration with Version Control
Now that we understand the role of CI in version control, let’s explore how to implement CI in your development workflow:
1. Choose a Version Control System
Select a version control system that best suits your team’s needs. Git is a popular choice due to its distributed nature and robust branching capabilities.
2. Set Up a CI Server
Choose and set up a CI server to monitor your version control system and run automated builds. Popular CI servers include:
- Jenkins
- GitLab CI/CD
- Travis CI
- CircleCI
- GitHub Actions
3. Define Build and Test Scripts
Create scripts that automate your build and test processes. These scripts should include:
- Compiling the code
- Running unit tests
- Running integration tests
- Performing static code analysis
- Generating code coverage reports
4. Configure CI Pipeline
Configure your CI server to monitor your version control system and trigger builds when changes are detected. A typical CI pipeline might include the following stages:
- Checkout: Fetch the latest code from the version control system
- Build: Compile the code and resolve dependencies
- Test: Run automated tests (unit tests, integration tests, etc.)
- Analysis: Perform static code analysis and generate reports
- Package: Create deployable artifacts
- Deploy: (Optional) Deploy to staging or production environments
5. Set Up Notifications
Configure your CI system to send notifications about build status and test results. This can include:
- Email notifications
- Slack or other chat platform integrations
- Dashboard displays for real-time monitoring
6. Integrate with Version Control Platform
Many version control platforms offer integrations with CI systems. Set up these integrations to:
- Display build status in pull requests
- Block merges for failing builds
- Automatically update status checks
7. Establish CI Best Practices
To get the most out of CI, establish and follow these best practices:
- Commit code frequently (at least daily)
- Keep the main branch stable and deployable at all times
- Write comprehensive automated tests
- Fix broken builds immediately
- Use feature flags for long-running features
Continuous Integration Tools and Integrations
There are numerous tools and integrations available to enhance your CI workflow with version control. Here are some popular options:
1. Jenkins
Jenkins is a widely-used, open-source CI server that offers extensive customization and a large plugin ecosystem. It integrates well with various version control systems and provides flexible pipeline configuration options.
2. GitLab CI/CD
GitLab CI/CD is a built-in CI/CD solution for GitLab repositories. It offers tight integration with GitLab’s version control features and provides an intuitive YAML-based pipeline configuration.
3. GitHub Actions
GitHub Actions is a CI/CD platform integrated directly into GitHub repositories. It allows you to automate your software development workflows, including CI, directly from your GitHub repository.
4. Travis CI
Travis CI is a popular cloud-based CI service that integrates well with GitHub repositories. It offers easy setup and configuration for various programming languages and frameworks.
5. CircleCI
CircleCI is another cloud-based CI/CD platform that integrates with various version control systems. It offers parallel test execution and customizable workflows.
6. SonarQube
SonarQube is a code quality and security analysis tool that can be integrated into your CI pipeline to provide detailed reports on code quality, test coverage, and potential vulnerabilities.
Best Practices for Combining CI and Version Control
To maximize the benefits of continuous integration in your version control workflow, consider the following best practices:
1. Trunk-Based Development
Adopt a trunk-based development approach, where developers frequently integrate their changes into a single branch (usually “main” or “trunk”). This approach reduces merge conflicts and promotes continuous integration.
2. Feature Flags
Use feature flags to decouple feature releases from code deployments. This allows you to merge code into the main branch more frequently while controlling when features are made available to users.
3. Comprehensive Test Coverage
Invest in writing comprehensive automated tests, including unit tests, integration tests, and end-to-end tests. This ensures that your CI pipeline can effectively catch issues before they reach production.
4. Fast Feedback
Optimize your CI pipeline for speed to provide fast feedback to developers. Consider techniques such as:
- Parallelizing test execution
- Using incremental builds
- Caching dependencies
5. Consistent Environments
Ensure that your CI environment closely mirrors your production environment to catch environment-specific issues early. Consider using containerization technologies like Docker to maintain consistency across environments.
6. Code Review Integration
Integrate your CI process with code reviews by:
- Automatically running CI checks on pull requests
- Displaying build and test results in the code review interface
- Enforcing passing builds before allowing merges
7. Monitored Metrics
Track and monitor key metrics related to your CI process, such as:
- Build success rate
- Build duration
- Test coverage
- Code quality trends
Use these metrics to continuously improve your CI process and identify areas for optimization.
Challenges and Considerations
While continuous integration offers numerous benefits when combined with version control, there are some challenges and considerations to keep in mind:
1. Initial Setup and Learning Curve
Setting up a CI system and integrating it with your version control workflow can be complex and time-consuming. Teams may need to invest time in learning new tools and processes.
2. Maintenance Overhead
CI systems require ongoing maintenance, including updating build scripts, managing dependencies, and troubleshooting integration issues.
3. Resource Requirements
Running frequent builds and tests can be resource-intensive, potentially requiring significant computing power and storage capacity.
4. False Positives and Flaky Tests
Automated tests may occasionally produce false positives or exhibit flaky behavior, leading to unnecessary failed builds and reduced confidence in the CI process.
5. Security Considerations
CI systems often require access to sensitive information, such as deployment credentials. Proper security measures must be implemented to protect these assets.
Conclusion
Continuous Integration plays a crucial role in enhancing version control practices and improving overall software development workflows. By automating build and test processes, detecting integration issues early, and providing rapid feedback to developers, CI helps teams maintain high code quality and deliver software more efficiently.
When implemented effectively, CI complements version control by:
- Ensuring code changes are thoroughly tested before integration
- Facilitating collaboration and code reviews
- Enforcing coding standards and best practices
- Simplifying release management
- Providing a continuous feedback loop for developers
By adopting CI best practices and leveraging the right tools and integrations, development teams can significantly improve their productivity, code quality, and ability to deliver value to users consistently and reliably.
As you embark on your journey to implement or improve CI in your version control workflow, remember that it’s an iterative process. Start small, continuously refine your approach, and adapt to the specific needs of your team and project. With time and dedication, you’ll reap the full benefits of continuous integration in your software development process.