How to Manage Branches in Git for Collaborative Projects
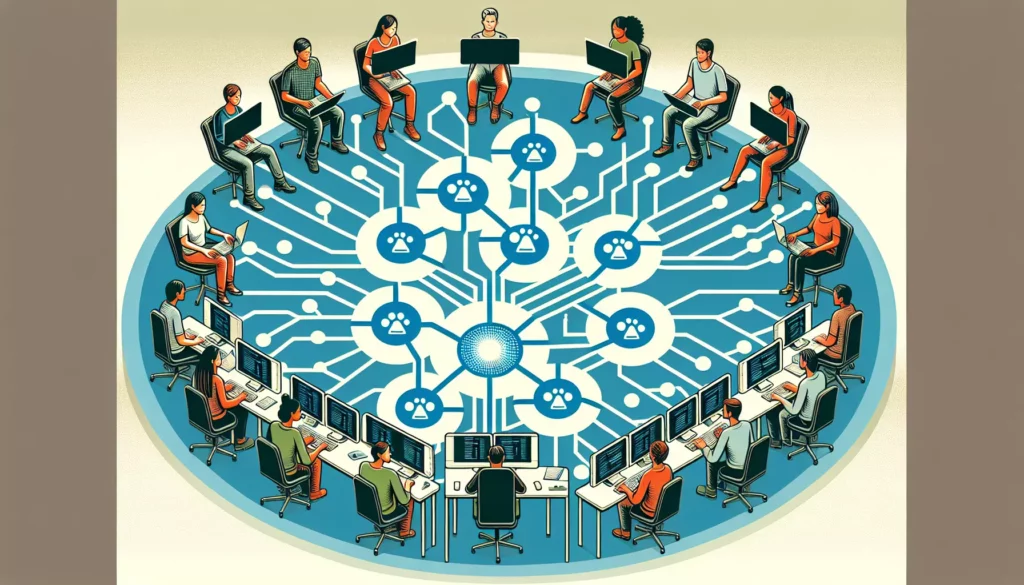
In the world of software development, collaboration is key. As projects grow in complexity and team sizes increase, managing code changes becomes increasingly challenging. This is where Git, a distributed version control system, comes to the rescue. One of Git’s most powerful features is its branching system, which allows developers to work on different features or bug fixes simultaneously without interfering with each other’s work. In this comprehensive guide, we’ll explore how to effectively manage branches in Git for collaborative projects, ensuring smooth teamwork and efficient code integration.
Understanding Git Branches
Before diving into branch management techniques, it’s crucial to understand what branches are and why they’re important in Git.
What is a Branch?
In Git, a branch is essentially a lightweight movable pointer to a commit. It represents an independent line of development, allowing you to diverge from the main line of development and work on different features or experiments without affecting the main codebase.
Why Use Branches?
Branches serve several important purposes in collaborative development:
- Isolation: Developers can work on new features or bug fixes without affecting the main codebase.
- Parallel Development: Multiple features can be developed simultaneously by different team members.
- Easy Experimentation: Branches allow for risk-free experimentation with new ideas.
- Code Review: Feature branches facilitate easier code reviews before merging into the main branch.
- Release Management: Branches can be used to manage different versions of the software.
Git Branch Management Strategies
Now that we understand the importance of branches, let’s explore some effective strategies for managing them in collaborative projects.
1. The Git Flow Model
Git Flow is a popular branching model that defines a strict branching structure designed around project releases. It involves the following main branches:
- master: The main branch that contains production-ready code.
- develop: The main branch for ongoing development.
- feature: Branches for developing new features.
- release: Branches for preparing new production releases.
- hotfix: Branches for quickly patching production releases.
Here’s how you might create and use these branches:
# Create and switch to a new feature branch
git checkout -b feature/new-login-page develop
# Work on the feature and commit changes
git add .
git commit -m "Implement new login page"
# Merge the feature back into develop
git checkout develop
git merge --no-ff feature/new-login-page
# Delete the feature branch
git branch -d feature/new-login-page
2. GitHub Flow
GitHub Flow is a simpler alternative to Git Flow, suitable for projects with continuous delivery. It involves:
- A main branch that is always deployable.
- Feature branches created from main for new work.
- Pull requests for merging feature branches back into main.
Here’s a typical workflow using GitHub Flow:
# Create a new feature branch
git checkout -b feature-xyz main
# Make changes and commit
git add .
git commit -m "Implement feature XYZ"
# Push the branch to the remote repository
git push -u origin feature-xyz
# Create a pull request on GitHub
# After review and approval, merge the pull request
3. Trunk-Based Development
Trunk-Based Development is a branching strategy where developers collaborate on code in a single branch called ‘trunk’ (usually the main or master branch). This approach emphasizes:
- Frequent, small commits to the main branch.
- Feature flags for managing incomplete features in production.
- Short-lived feature branches, if used at all.
Here’s how you might work with Trunk-Based Development:
# Pull the latest changes from the main branch
git pull origin main
# Make your changes and commit frequently
git add .
git commit -m "Implement part of feature XYZ"
# Push changes to the main branch
git push origin main
Best Practices for Branch Management
Regardless of the branching strategy you choose, following these best practices will help ensure smooth collaboration:
1. Keep Branches Short-Lived
Long-lived branches can lead to integration headaches. Aim to merge feature branches back into the main development branch as quickly as possible.
2. Use Descriptive Branch Names
Choose clear, descriptive names for your branches. This makes it easier for team members to understand the purpose of each branch at a glance.
# Good branch names
feature/user-authentication
bugfix/login-error
hotfix/security-patch-123
3. Regularly Update Your Branches
Keep your feature branches up to date with the latest changes from the main development branch. This reduces the likelihood of merge conflicts later on.
# Update your feature branch with changes from develop
git checkout feature/my-feature
git rebase develop
4. Use Pull Requests
Pull requests (or merge requests in GitLab) provide a way to review code before merging it into the main branch. This helps maintain code quality and catch potential issues early.
5. Clean Up Merged Branches
After a branch has been merged, delete it to keep your repository clean and manageable.
# Delete a local branch
git branch -d feature/completed-feature
# Delete a remote branch
git push origin --delete feature/completed-feature
6. Use Branch Protection Rules
Many Git hosting platforms allow you to set up branch protection rules. These can enforce code review, require status checks to pass before merging, and prevent force pushes to important branches.
Advanced Branch Management Techniques
As your project grows and your team becomes more comfortable with Git, you might want to explore some advanced branch management techniques:
1. Cherry-Picking
Cherry-picking allows you to apply specific commits from one branch to another. This can be useful when you want to bring a bug fix from a feature branch into the main branch without merging the entire feature.
# Cherry-pick a specific commit
git cherry-pick <commit-hash>
2. Rebasing
Rebasing is the process of moving or combining a sequence of commits to a new base commit. It can help maintain a cleaner, more linear project history.
# Rebase your feature branch onto the latest main
git checkout feature/my-feature
git rebase main
3. Interactive Rebasing
Interactive rebasing allows you to modify commits in various ways as you move them to a new base. This is great for cleaning up your commit history before merging a feature branch.
# Start an interactive rebase
git rebase -i HEAD~3 # Rebase the last 3 commits
4. Git Hooks
Git hooks are scripts that Git executes before or after events such as commit, push, and receive. They can be used to enforce coding standards, run tests, or perform other automated checks.
Handling Merge Conflicts
Even with the best branch management practices, merge conflicts can still occur. Here’s how to handle them:
1. Identify the Conflict
When Git encounters a merge conflict, it will mark the conflicting areas in the affected files:
<<<<<<< HEAD
Your changes
=======
Changes from the branch you're merging
>>>>>>> feature/other-branch
2. Resolve the Conflict
Open the conflicting files and manually resolve the conflicts. Decide which changes to keep and remove the conflict markers.
3. Stage and Commit
After resolving conflicts, stage the changed files and create a new commit to complete the merge:
git add <resolved-file>
git commit -m "Merge branch 'feature/other-branch' and resolve conflicts"
Tools for Branch Management
While Git’s command-line interface is powerful, there are several tools that can make branch management easier:
1. GitKraken
GitKraken is a popular Git GUI client that provides a visual representation of your branches and commit history. It makes operations like branching, merging, and rebasing more intuitive.
2. Sourcetree
Sourcetree is another Git GUI that offers a user-friendly interface for managing branches, commits, and repositories.
3. GitHub Desktop
For those working with GitHub repositories, GitHub Desktop provides a simple way to manage branches and perform common Git operations.
4. Git Extensions
Git Extensions is an open-source tool that extends the functionality of Git, providing a graphical user interface and shell extension.
Conclusion
Effective branch management is crucial for successful collaboration in software development projects. By understanding Git’s branching system and implementing appropriate strategies, you can streamline your team’s workflow, reduce conflicts, and maintain a clean, organized codebase.
Remember, the key to successful branch management lies in clear communication within your team, consistent adherence to your chosen branching strategy, and a willingness to adapt your practices as your project evolves. With these principles in mind, you’ll be well-equipped to handle the complexities of collaborative development and deliver high-quality software efficiently.
As you continue to work with Git and explore its capabilities, you’ll discover that mastering branch management is not just about following rules, but about understanding the underlying principles and applying them creatively to solve your team’s unique challenges. Happy coding, and may your branches always merge smoothly!