Introduction to Git: Why Every Developer Should Learn It
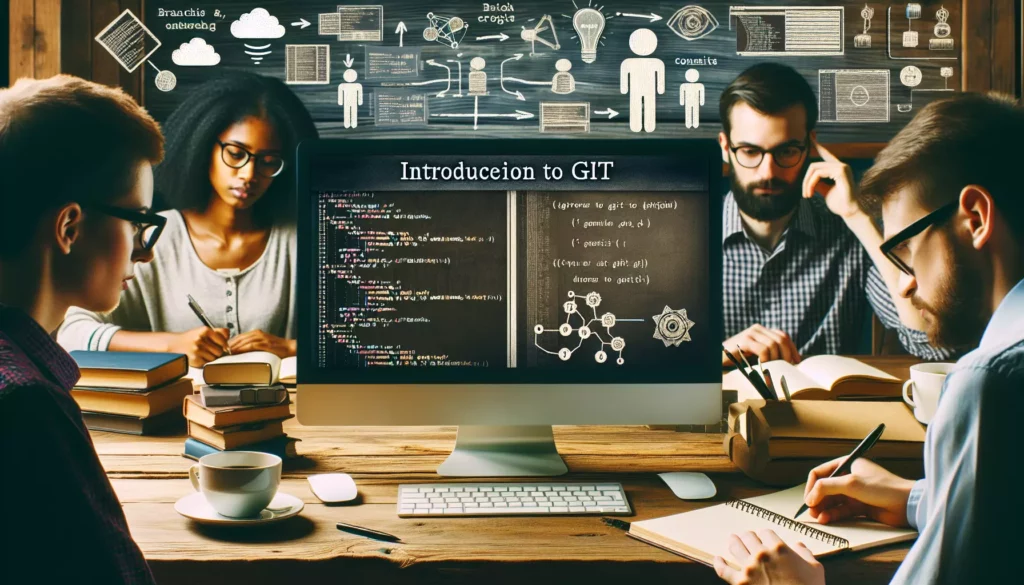
In the fast-paced world of software development, version control systems have become an indispensable tool for developers of all levels. Among these systems, Git stands out as the most widely adopted and powerful option. Whether you’re a beginner just starting your coding journey or an experienced developer looking to enhance your skills, understanding Git is crucial for success in the modern development landscape.
In this comprehensive guide, we’ll explore what Git is, why it’s essential, and how it can benefit your development process. We’ll also cover some basic Git commands and best practices to help you get started on your Git journey.
Table of Contents
- What is Git?
- Why Should Every Developer Learn Git?
- Key Concepts in Git
- Basic Git Commands
- Git Best Practices
- Git vs. Other Version Control Systems
- Getting Started with Git
- Advanced Git Features
- Git in Team Collaboration
- Conclusion
1. What is Git?
Git is a distributed version control system created by Linus Torvalds in 2005. It was designed to manage the development of the Linux kernel, but its usefulness quickly spread beyond that initial purpose. Git allows developers to track changes in their code over time, collaborate with others, and manage different versions of their projects.
Unlike centralized version control systems, Git is distributed, meaning that every developer’s working copy of the code is also a repository that can contain the full history of all changes. This distributed nature offers several advantages, including:
- Improved speed and performance
- The ability to work offline
- Enhanced security and data integrity
- Greater flexibility in branching and merging
2. Why Should Every Developer Learn Git?
Learning Git is essential for several reasons:
2.1 Industry Standard
Git has become the de facto standard for version control in the software industry. Most companies and open-source projects use Git, making it a crucial skill for any developer looking to work professionally or contribute to open-source projects.
2.2 Collaboration
Git facilitates seamless collaboration among developers. It allows multiple people to work on the same project simultaneously without interfering with each other’s work. This is particularly important in team environments and large-scale projects.
2.3 Version Control
With Git, you can track changes to your code over time. This means you can revert to previous versions if something goes wrong, compare changes, and understand how your project has evolved.
2.4 Backup and Recovery
Git serves as a distributed backup system for your code. Even if your local machine fails, you can recover your entire project history from a remote repository.
2.5 Experimentation
Git’s branching model allows you to experiment with new features or ideas without affecting the main codebase. You can create separate branches for different features and merge them back when they’re ready.
2.6 Career Advancement
Proficiency in Git is often listed as a required skill in job postings for developers. Learning Git can enhance your resume and make you a more attractive candidate to potential employers.
3. Key Concepts in Git
Before diving into Git commands, it’s important to understand some key concepts:
3.1 Repository
A repository (or “repo”) is a directory where Git has been initialized to start version controlling your files. This is where Git stores the metadata and object database for your project.
3.2 Commit
A commit is a snapshot of your repository at a specific point in time. It’s like a save point in a video game, allowing you to return to this state later if needed.
3.3 Branch
A branch is a parallel version of your repository. It allows you to work on different parts of your project without impacting the main part. You can merge branches back together when you’re ready.
3.4 Remote
A remote is a common repository that all team members use to exchange their changes. In most cases, it’s stored on a code hosting service like GitHub or GitLab.
3.5 Clone
Cloning is the process of creating a local copy of a remote repository on your machine.
3.6 Push
Pushing is how you transfer commits from your local repository to a remote repo.
3.7 Pull
Pulling is the opposite of pushing. It’s how you fetch changes from a remote repository to your local repo.
4. Basic Git Commands
Here are some essential Git commands to get you started:
4.1 git init
This command initializes a new Git repository in your current directory.
git init
4.2 git clone
Use this command to create a copy of an existing repository.
git clone <repository-url>
4.3 git add
This command adds files to the staging area, preparing them for a commit.
git add <file-name>
git add . # Adds all changed files
4.4 git commit
This command creates a new commit with the changes in your staging area.
git commit -m "Your commit message here"
4.5 git status
Use this command to see the current state of your repository, including which files have been modified and which are staged for commit.
git status
4.6 git push
This command uploads your local repository content to a remote repository.
git push origin <branch-name>
4.7 git pull
Use this command to fetch and download content from a remote repository and immediately update your local repository to match that content.
git pull origin <branch-name>
4.8 git branch
This command allows you to create, list, rename, and delete branches.
git branch # Lists all local branches
git branch <branch-name> # Creates a new branch
git branch -d <branch-name> # Deletes a branch
4.9 git checkout
Use this command to switch between branches in a repository.
git checkout <branch-name>
4.10 git merge
This command merges changes from different branches.
git merge <branch-name>
5. Git Best Practices
To make the most of Git, follow these best practices:
5.1 Commit Often
Make small, frequent commits rather than large, infrequent ones. This makes it easier to track changes and revert if necessary.
5.2 Write Clear Commit Messages
Your commit messages should be clear and descriptive. A good format is a short summary (50 characters or less) followed by a blank line and then a more detailed explanation if necessary.
5.3 Use Branches
Create separate branches for different features or experiments. This keeps your main branch clean and makes it easier to manage different aspects of your project.
5.4 Pull Before You Push
Always pull the latest changes from the remote repository before pushing your own changes. This helps avoid merge conflicts.
5.5 Use .gitignore
Create a .gitignore file to specify which files or directories Git should ignore. This is useful for excluding build artifacts, temporary files, and sensitive information.
5.6 Review Your Changes
Before committing, use git diff
to review your changes. This helps ensure you’re only committing what you intend to.
6. Git vs. Other Version Control Systems
While Git is the most popular version control system, it’s not the only one. Let’s compare Git with some other systems:
6.1 Git vs. SVN (Subversion)
- Git is distributed, while SVN is centralized.
- Git allows for easier branching and merging.
- Git is faster for most operations.
- SVN is simpler for beginners but less powerful overall.
6.2 Git vs. Mercurial
- Both are distributed version control systems.
- Git has a larger community and more third-party tools.
- Mercurial is considered easier to learn but less flexible.
6.3 Git vs. Perforce
- Perforce is centralized, while Git is distributed.
- Perforce handles large binary files better.
- Git is free and open-source, while Perforce is proprietary.
7. Getting Started with Git
Now that you understand the basics, here’s how to get started with Git:
7.1 Install Git
First, download and install Git from the official Git website. Follow the installation instructions for your operating system.
7.2 Configure Git
After installation, set up your identity. This is important because Git embeds this information into each commit.
git config --global user.name "Your Name"
git config --global user.email "your.email@example.com"
7.3 Create Your First Repository
Navigate to your project directory and initialize a new Git repository:
cd /path/to/your/project
git init
7.4 Make Your First Commit
Add your files to the staging area and make your first commit:
git add .
git commit -m "Initial commit"
7.5 Create a Remote Repository
Create a new repository on a hosting service like GitHub or GitLab. Then, add it as a remote to your local repository:
git remote add origin <remote-repository-url>
7.6 Push Your Code
Finally, push your code to the remote repository:
git push -u origin master
8. Advanced Git Features
As you become more comfortable with Git, you can explore its advanced features:
8.1 Git Rebase
Rebase is an alternative to merging. It allows you to move or combine a sequence of commits to a new base commit.
git rebase <base-branch>
8.2 Git Stash
Stash allows you to temporarily store modified, tracked files in order to switch branches.
git stash
git stash pop
8.3 Git Cherry-pick
Cherry-pick allows you to apply the changes introduced by some existing commits.
git cherry-pick <commit-hash>
8.4 Git Hooks
Hooks are scripts that Git executes before or after events such as commit, push, and receive.
8.5 Git Submodules
Submodules allow you to keep a Git repository as a subdirectory of another Git repository.
git submodule add <repository-url> <path>
9. Git in Team Collaboration
Git truly shines in team environments. Here are some practices for using Git effectively in a team:
9.1 Use Feature Branches
Create separate branches for each feature or bug fix. This keeps the main branch clean and makes code reviews easier.
9.2 Pull Requests
Use pull requests (or merge requests in GitLab) to propose changes to the main branch. This allows for code review before merging.
9.3 Code Reviews
Implement a code review process using Git. This improves code quality and helps team members learn from each other.
9.4 Continuous Integration
Set up continuous integration to automatically test your code when changes are pushed to the repository.
9.5 Git Flow
Consider adopting a Git workflow like Git Flow for larger projects. This provides a structured approach to branching and releasing.
10. Conclusion
Git is an essential tool for modern software development. Its distributed nature, powerful branching and merging capabilities, and widespread adoption make it invaluable for both individual developers and teams. By learning Git, you’re not only gaining a crucial skill for your career but also empowering yourself to manage your projects more effectively and collaborate more efficiently with others.
Remember, mastering Git takes time and practice. Start with the basics, and gradually explore more advanced features as you become comfortable. Don’t be afraid to make mistakes – Git’s design allows you to recover from most errors easily.
As you continue your journey in software development, Git will be a constant companion, helping you track your progress, collaborate with others, and manage your code with confidence. Happy coding, and may your commits always be clear and your merges conflict-free!