The Alchemist Coder: Transforming Base Code into Algorithmic Gold
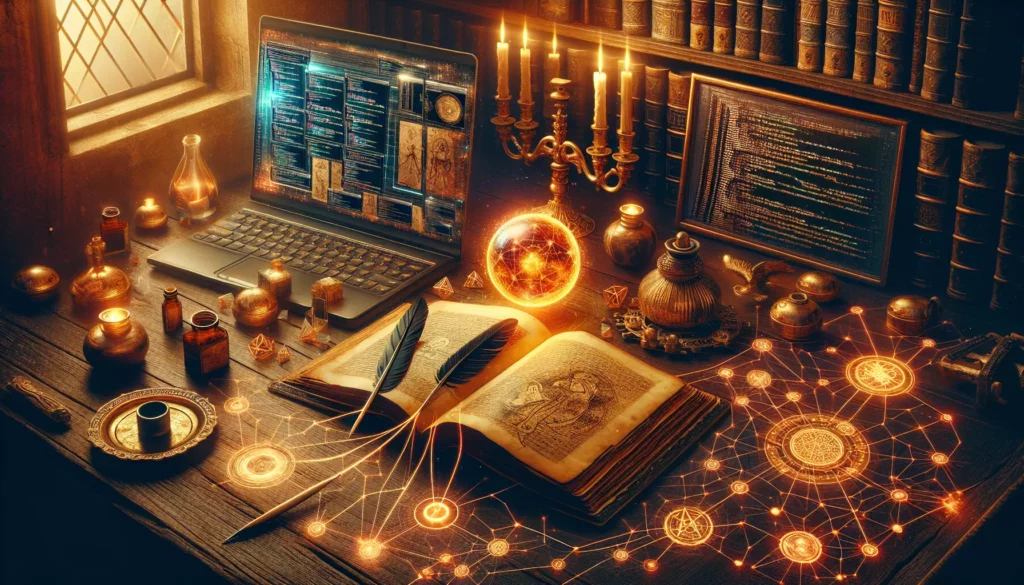
In the realm of computer science and software development, there exists a special breed of programmer – the Alchemist Coder. These skilled artisans possess the ability to transmute ordinary code into extraordinary algorithms, much like the legendary alchemists of old who sought to turn base metals into gold. In this comprehensive guide, we’ll explore the art and science of algorithmic transformation, delving into the techniques, mindset, and skills required to become a true Alchemist Coder.
The Essence of Algorithmic Alchemy
Before we dive into the specifics of code transformation, it’s crucial to understand what sets the Alchemist Coder apart from the average programmer. The key lies in their ability to see beyond the surface-level functionality of code and recognize its underlying potential for optimization and elegance.
Algorithmic alchemy is not just about making code faster or more efficient – though that’s certainly part of it. It’s about reimagining the very structure and approach to problem-solving within a program. The Alchemist Coder doesn’t just refactor; they revolutionize.
The Pillars of Algorithmic Transformation
- Efficiency: Optimizing time and space complexity
- Elegance: Crafting clean, readable, and maintainable code
- Scalability: Ensuring solutions can grow with increasing demands
- Adaptability: Creating flexible code that can evolve with changing requirements
The Alchemist’s Toolkit: Essential Skills and Knowledge
To transform base code into algorithmic gold, one must first master a set of fundamental skills and acquire a deep well of knowledge. Let’s explore the essential components of the Alchemist Coder’s toolkit.
1. Data Structures Mastery
At the heart of every efficient algorithm lies a well-chosen data structure. The Alchemist Coder must have an intimate understanding of various data structures and their properties:
- Arrays and Linked Lists
- Stacks and Queues
- Trees and Graphs
- Hash Tables
- Heaps
- Tries
Knowing when and how to use each data structure is crucial for optimizing both time and space complexity.
2. Algorithm Design Paradigms
The ability to recognize and apply various algorithm design paradigms is what separates the Alchemist Coder from the novice. Some key paradigms include:
- Divide and Conquer
- Dynamic Programming
- Greedy Algorithms
- Backtracking
- Branch and Bound
Each paradigm offers a unique approach to problem-solving, and the true Alchemist knows how to select and adapt these strategies to fit the task at hand.
3. Time and Space Complexity Analysis
Understanding the performance characteristics of code is crucial for optimization. The Alchemist Coder must be adept at:
- Analyzing time complexity using Big O notation
- Identifying space complexity issues
- Recognizing trade-offs between time and space
This knowledge allows for informed decisions when choosing between different algorithmic approaches.
4. Pattern Recognition
Many programming problems share common patterns. The ability to recognize these patterns allows the Alchemist Coder to apply proven solutions or adapt existing algorithms to new contexts. Some common patterns include:
- Two-pointer technique
- Sliding window
- Fast and slow pointers
- Merge intervals
- Topological sort
5. Language Proficiency
While algorithmic thinking transcends specific programming languages, the Alchemist Coder must be proficient in at least one language to effectively implement their ideas. This includes:
- Deep understanding of language-specific features and optimizations
- Familiarity with standard libraries and built-in functions
- Ability to write idiomatic code in the chosen language
The Transformation Process: From Base Code to Algorithmic Gold
Now that we’ve explored the foundational skills, let’s delve into the actual process of transforming code. The Alchemist Coder follows a systematic approach to turn ordinary solutions into extraordinary algorithms.
Step 1: Understand the Problem Deeply
Before any transformation can begin, it’s crucial to have a thorough understanding of the problem at hand. This involves:
- Analyzing the problem statement
- Identifying edge cases and constraints
- Recognizing the underlying patterns or similarities to known problems
Step 2: Analyze the Existing Solution
With a clear understanding of the problem, the next step is to critically examine the current implementation:
- Identify the time and space complexity
- Recognize any inefficiencies or redundancies
- Understand the logic and flow of the existing code
Step 3: Brainstorm Alternative Approaches
This is where the Alchemist Coder’s creativity and knowledge come into play. Consider different ways to solve the problem:
- Apply various algorithm design paradigms
- Explore different data structures that might be more suitable
- Consider trade-offs between time and space complexity
Step 4: Implement the Chosen Solution
Once a promising approach is identified, it’s time to implement the new solution:
- Write clean, well-documented code
- Use appropriate naming conventions and structure
- Implement error handling and edge case management
Step 5: Optimize and Refine
The initial implementation is rarely the final version. The Alchemist Coder continually refines their solution:
- Look for opportunities to reduce time or space complexity
- Simplify logic and remove redundancies
- Ensure the code is readable and maintainable
Step 6: Test and Validate
Thorough testing is crucial to ensure the transformed code is correct and efficient:
- Write comprehensive unit tests
- Test with various input sizes to verify scalability
- Benchmark the new solution against the original implementation
Case Study: Transforming a Simple Search Algorithm
Let’s walk through a practical example of how an Alchemist Coder might transform a basic search algorithm into a more efficient solution.
The Original Problem: Finding a Number in a Sorted Array
Consider the following simple implementation of a linear search in Python:
def linear_search(arr, target):
for i in range(len(arr)):
if arr[i] == target:
return i
return -1
# Example usage
sorted_array = [1, 3, 5, 7, 9, 11, 13, 15, 17]
result = linear_search(sorted_array, 11)
print(f"Element found at index: {result}")
This implementation has a time complexity of O(n), where n is the length of the array. While it works, it doesn’t take advantage of the fact that the array is sorted.
The Alchemist’s Transformation: Binary Search
Recognizing that the array is sorted, an Alchemist Coder would immediately think of using a binary search algorithm, which has a time complexity of O(log n). Here’s how they might implement it:
def binary_search(arr, target):
left, right = 0, len(arr) - 1
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1
# Example usage
sorted_array = [1, 3, 5, 7, 9, 11, 13, 15, 17]
result = binary_search(sorted_array, 11)
print(f"Element found at index: {result}")
Analysis of the Transformation
Let’s break down the alchemical process that led to this transformation:
- Problem Understanding: The Alchemist recognized that the array was sorted, a crucial detail for optimization.
- Existing Solution Analysis: The linear search was identified as inefficient for a sorted array.
- Alternative Approach: Binary search was chosen as an optimal algorithm for searching sorted arrays.
- Implementation: The binary search algorithm was implemented with clear, concise code.
- Optimization: The implementation uses integer division to avoid floating-point operations, a small but meaningful optimization.
- Testing: The new implementation would be tested with various array sizes and edge cases to ensure correctness and efficiency.
The result is a significant improvement in time complexity from O(n) to O(log n), which can make a substantial difference for large datasets.
Advanced Techniques for the Aspiring Alchemist
As you progress in your journey to become an Alchemist Coder, there are several advanced techniques and concepts you should explore:
1. Bit Manipulation
Mastering bit manipulation can lead to extremely efficient algorithms for certain problems. Key concepts include:
- Bitwise operators (AND, OR, XOR, NOT)
- Bit shifting
- Using bitmasks for set operations
2. Advanced Data Structures
Familiarize yourself with more complex data structures that can solve specific problems efficiently:
- Segment Trees
- Fenwick Trees (Binary Indexed Trees)
- Disjoint Set Union (Union-Find)
- Suffix Arrays and Suffix Trees
3. Randomized Algorithms
Some problems benefit from probabilistic approaches:
- Monte Carlo algorithms
- Las Vegas algorithms
- Randomized data structures (e.g., Skip Lists)
4. Approximation Algorithms
For NP-hard problems, approximation algorithms can provide near-optimal solutions:
- Greedy approximation algorithms
- Polynomial-time approximation schemes (PTAS)
5. Parallel and Distributed Algorithms
In the age of multi-core processors and distributed systems, parallel algorithms are increasingly important:
- Parallel sorting and searching algorithms
- MapReduce paradigm
- Distributed consensus algorithms
The Ethical Alchemist: Considerations Beyond Efficiency
As we strive to transform code into algorithmic gold, it’s crucial to remember that true alchemy in programming goes beyond mere efficiency. The ethical Alchemist Coder also considers:
1. Code Readability and Maintainability
While optimizing for performance, never sacrifice the readability of your code. Remember:
- Clear variable and function names
- Well-structured code with appropriate comments
- Adherence to coding standards and best practices
2. Scalability and Future-Proofing
Consider how your algorithmic transformations will hold up as the problem size or requirements change:
- Design for extensibility
- Consider potential future use cases
- Document assumptions and limitations
3. Resource Consumption
In an age of cloud computing and mobile devices, consider the broader impact of your algorithms:
- Energy efficiency
- Memory usage in resource-constrained environments
- Impact on battery life for mobile applications
4. Ethical Implications
Some algorithmic transformations, especially those involving AI and machine learning, can have significant ethical implications:
- Bias in algorithms and data
- Privacy concerns
- Transparency and explainability of complex algorithms
Continuous Learning: The Alchemist’s Journey Never Ends
The field of computer science and software development is constantly evolving. To maintain your status as an Alchemist Coder, you must commit to lifelong learning:
1. Stay Updated with Research
- Read academic papers and attend conferences
- Follow prominent computer scientists and researchers
- Experiment with cutting-edge algorithms and techniques
2. Practice Regularly
- Solve coding challenges on platforms like LeetCode, HackerRank, or CodeForces
- Participate in coding competitions
- Contribute to open-source projects
3. Teach and Share Knowledge
- Write blog posts or articles about your algorithmic insights
- Mentor junior developers
- Give talks or workshops at local meetups or conferences
4. Explore Interdisciplinary Connections
- Study how algorithms are applied in fields like biology, physics, or finance
- Learn about the philosophical and mathematical foundations of computer science
- Investigate the intersection of algorithms with emerging technologies like quantum computing
Conclusion: Embracing the Alchemist’s Mindset
Becoming an Alchemist Coder is not just about acquiring a set of skills or memorizing algorithms. It’s about developing a mindset – a way of approaching problems that combines creativity, analytical thinking, and a deep understanding of computational principles.
As you embark on your journey to transform base code into algorithmic gold, remember that true mastery comes from practice, perseverance, and a willingness to continuously learn and adapt. Embrace the challenges, celebrate the breakthroughs, and always strive to push the boundaries of what’s possible with code.
The path of the Alchemist Coder is one of constant discovery and transformation. With each problem you solve and each algorithm you optimize, you’re not just improving code – you’re evolving as a programmer and contributing to the advancement of the field.
So, take up your keyboard, sharpen your mind, and begin your alchemical journey. The world of algorithmic wonders awaits, ready to be transformed by your ingenuity and skill. Remember, in the realm of code, you are the Alchemist, and the potential for creating digital gold is limitless.