The Coder’s Séance: Channeling the Spirits of Programming Pioneers for Guidance
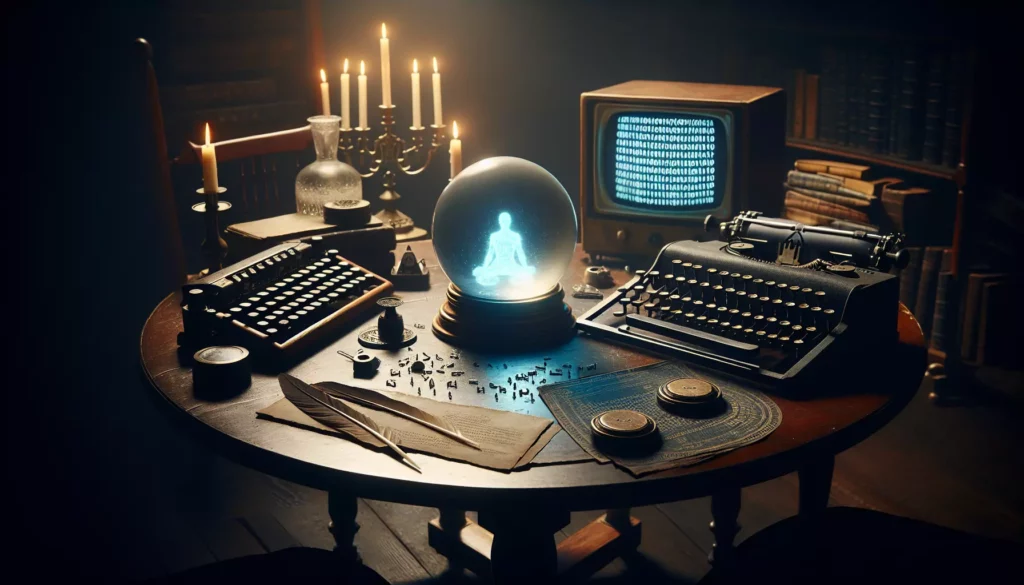
In the dimly lit room of a coder’s sanctuary, surrounded by humming servers and glowing monitors, an unusual gathering is about to take place. Tonight, we’re not just debugging code or optimizing algorithms; we’re attempting to bridge the gap between the physical and digital realms. Welcome to the Coder’s Séance, where we’ll channel the spirits of programming pioneers to seek their timeless wisdom and guidance.
As we embark on this mystical journey through the annals of computer science, we’ll explore how the insights of past luminaries can illuminate our path in modern software development. From the early days of computing to the cutting-edge technologies of today, the spirits of these pioneers have much to teach us about problem-solving, innovation, and the very essence of programming itself.
Setting the Stage for Our Digital Divination
Before we begin our séance, let’s set the mood. Dim the lights in your coding cave, power up your favorite IDE, and prepare to open your mind to the whispers of the binary beyond. As we call upon these legendary figures, remember that their legacy lives on in every line of code we write and every problem we solve.
Our spiritual guides for this evening’s journey include:
- Ada Lovelace – The world’s first programmer
- Alan Turing – Father of theoretical computer science and artificial intelligence
- Grace Hopper – Pioneer of computer programming who invented the first compiler
- John von Neumann – Architect of the modern computer
- Dennis Ritchie – Creator of the C programming language and co-developer of Unix
As we channel these spirits, we’ll explore how their groundbreaking ideas and philosophies can guide us in our modern coding practices, from tackling complex algorithms to preparing for technical interviews at top tech companies.
Invoking the Spirit of Ada Lovelace: The Poetry of Programming
We begin our séance by calling upon the spirit of Ada Lovelace, often regarded as the world’s first computer programmer. In the flickering candlelight, we can almost see her ghostly figure materializing before us, ready to impart her wisdom.
“My dear modern programmers,” Ada’s spirit whispers, “remember that programming is not merely a science, but an art form. Approach your code with the imagination of a poet and the precision of a mathematician.”
Ada Lovelace’s unique perspective on programming as a blend of creativity and logic is as relevant today as it was in the 19th century. As we prepare for technical interviews or work on complex projects, we should embrace this duality:
- Cultivate creativity in problem-solving
- Look for elegant solutions that balance efficiency and readability
- Don’t be afraid to think outside the box when tackling algorithmic challenges
Let’s channel Ada’s spirit into a modern coding challenge. Imagine we’re tasked with implementing a function to generate the Fibonacci sequence, a problem often encountered in coding interviews. Here’s how we might approach it with both creativity and mathematical precision:
def fibonacci_generator(n):
a, b = 0, 1
for _ in range(n):
yield a
a, b = b, a + b
# Using the generator
fib_sequence = list(fibonacci_generator(10))
print(fib_sequence) # Output: [0, 1, 1, 2, 3, 5, 8, 13, 21, 34]
This implementation uses a generator function, combining the mathematical logic of the Fibonacci sequence with the creative use of Python’s yield statement. It’s both efficient and expressive, embodying Ada’s philosophy of merging art and science in programming.
Channeling Alan Turing: The Essence of Computational Thinking
As the séance continues, the air grows thick with anticipation. Suddenly, a spectral machine begins to whir and click, heralding the arrival of Alan Turing’s spirit. The father of theoretical computer science and artificial intelligence has much to teach us about the fundamentals of computational thinking.
“To truly master the art of programming,” Turing’s spirit intones, “one must think not in terms of specific languages or frameworks, but in the universal concepts of computation itself.”
Turing’s advice reminds us of the importance of understanding core computer science concepts, especially when preparing for technical interviews at top tech companies. Here are some key takeaways:
- Focus on algorithmic thinking and problem decomposition
- Understand the theoretical limits and possibilities of computation
- Practice implementing basic algorithms and data structures from scratch
Let’s apply Turing’s wisdom to a classic problem: implementing a simple Turing machine that checks if a binary string has an equal number of 0s and 1s. This exercise combines theoretical computer science with practical coding skills:
class TuringMachine:
def __init__(self):
self.tape = []
self.head = 0
self.state = 'q0'
def run(self, input_string):
self.tape = list(input_string) + ['B'] # 'B' represents a blank
self.head = 0
self.state = 'q0'
while self.state != 'qAccept' and self.state != 'qReject':
self.step()
return self.state == 'qAccept'
def step(self):
current_symbol = self.tape[self.head]
if self.state == 'q0':
if current_symbol == '0':
self.tape[self.head] = 'X'
self.head += 1
self.state = 'q1'
elif current_symbol == '1':
self.tape[self.head] = 'X'
self.head += 1
self.state = 'q2'
elif current_symbol == 'B':
self.state = 'qAccept'
elif self.state == 'q1':
if current_symbol == '0':
self.head += 1
elif current_symbol == '1':
self.head += 1
self.state = 'q2'
elif current_symbol == 'B':
self.state = 'qReject'
elif self.state == 'q2':
if current_symbol == '0':
self.head += 1
self.state = 'q1'
elif current_symbol == '1':
self.head += 1
elif current_symbol == 'B':
self.state = 'qReject'
# Test the Turing Machine
tm = TuringMachine()
print(tm.run("01010101")) # Output: True
print(tm.run("0011")) # Output: True
print(tm.run("0001")) # Output: False
This implementation of a Turing machine demonstrates the power of computational thinking. By breaking down the problem into states and transitions, we create a solution that’s both theoretically sound and practically applicable.
Summoning Grace Hopper: The Art of Compiler Design
The room grows colder as we call upon the spirit of Grace Hopper, the pioneering computer scientist who developed the first compiler. Her presence brings with it the scent of old punch cards and the soft whirring of early computers.
“Young coders,” Grace’s spirit advises, “remember that abstraction is the key to managing complexity. Just as a compiler bridges the gap between human-readable code and machine instructions, you must learn to create layers of abstraction in your own programs.”
Grace Hopper’s insights are particularly valuable for those preparing for technical interviews or working on large-scale projects. Here’s how we can apply her wisdom:
- Practice creating clean, modular code with well-defined interfaces
- Understand the importance of different levels of abstraction in software design
- Learn to balance low-level optimization with high-level readability
Let’s channel Grace’s spirit by implementing a simple lexical analyzer, the first step in building a compiler. This example will tokenize a basic arithmetic expression:
import re
class Token:
def __init__(self, type, value):
self.type = type
self.value = value
def __str__(self):
return f'Token({self.type}, {self.value})'
def lexical_analyzer(input_string):
token_specification = [
('NUMBER', r'\d+(\.\d*)?'), # Integer or decimal number
('PLUS', r'\+'), # Plus operator
('MINUS', r'-'), # Minus operator
('TIMES', r'\*'), # Multiplication operator
('DIVIDE', r'/'), # Division operator
('LPAREN', r'\('), # Left parenthesis
('RPAREN', r'\)'), # Right parenthesis
('WHITESPACE', r'\s+'), # Whitespace
]
tok_regex = '|'.join('(?P<%s>%s)' % pair for pair in token_specification)
for mo in re.finditer(tok_regex, input_string):
kind = mo.lastgroup
value = mo.group()
if kind == 'WHITESPACE':
continue
yield Token(kind, value)
# Test the lexical analyzer
expression = "3.14 + (2 * 5) - 10 / 2"
for token in lexical_analyzer(expression):
print(token)
This lexical analyzer demonstrates the power of abstraction in compiler design. By breaking down the input into tokens, we create a higher-level representation that’s easier to work with in subsequent stages of compilation or interpretation.
Invoking John von Neumann: The Architecture of Efficient Computing
As we delve deeper into our séance, the spirit of John von Neumann materializes, bringing with him the essence of modern computer architecture. The air crackles with the energy of his brilliant mind.
“To truly excel in the art of programming,” von Neumann’s spirit declares, “one must understand the underlying hardware. The most elegant algorithms are those that align with the architecture of the machine.”
Von Neumann’s advice is crucial for optimizing code performance, a skill often tested in technical interviews at top tech companies. Here’s how we can apply his wisdom:
- Study computer architecture and understand how code interacts with hardware
- Learn to write cache-friendly code to improve performance
- Consider memory layout and access patterns when designing data structures
Let’s channel von Neumann’s spirit by implementing a cache-friendly matrix multiplication algorithm. This example demonstrates how understanding computer architecture can lead to more efficient code:
import numpy as np
def matrix_multiply(A, B):
n = A.shape[0]
C = np.zeros((n, n))
block_size = 32 # Choose a block size that fits well in the cache
for i in range(0, n, block_size):
for j in range(0, n, block_size):
for k in range(0, n, block_size):
# Multiply block submatrices
block_A = A[i:i+block_size, k:k+block_size]
block_B = B[k:k+block_size, j:j+block_size]
C[i:i+block_size, j:j+block_size] += np.dot(block_A, block_B)
return C
# Test the cache-friendly matrix multiplication
A = np.random.rand(1024, 1024)
B = np.random.rand(1024, 1024)
result = matrix_multiply(A, B)
print("Matrix multiplication completed.")
This implementation uses block matrix multiplication, which improves cache utilization by operating on smaller blocks of the matrices that can fit into the CPU cache. By aligning our algorithm with the underlying hardware architecture, we achieve better performance, especially for large matrices.
Channeling Dennis Ritchie: The Power of Simplicity and Portability
As our séance nears its conclusion, we call upon the spirit of Dennis Ritchie, creator of the C programming language and co-developer of Unix. The room fills with the nostalgic aroma of coffee and the soft glow of old CRT monitors.
“In the world of programming,” Ritchie’s spirit intones, “simplicity and portability are virtues of the highest order. Create tools and languages that are powerful yet adaptable, and your code will stand the test of time.”
Ritchie’s philosophy is especially relevant in today’s diverse computing landscape. Here’s how we can apply his wisdom:
- Strive for clarity and simplicity in code design
- Write portable code that can run on multiple platforms
- Create modular, reusable components that can be easily integrated into larger systems
Let’s channel Ritchie’s spirit by implementing a simple, portable linked list in C. This example demonstrates the power of creating flexible, reusable data structures:
#include <stdio.h>
#include <stdlib.h>
struct Node {
int data;
struct Node* next;
};
struct Node* createNode(int data) {
struct Node* newNode = (struct Node*)malloc(sizeof(struct Node));
if (newNode == NULL) {
printf("Memory allocation failed\n");
exit(1);
}
newNode->data = data;
newNode->next = NULL;
return newNode;
}
void insertAtBeginning(struct Node** head, int data) {
struct Node* newNode = createNode(data);
newNode->next = *head;
*head = newNode;
}
void printList(struct Node* head) {
struct Node* current = head;
while (current != NULL) {
printf("%d -> ", current->data);
current = current->next;
}
printf("NULL\n");
}
void freeList(struct Node* head) {
struct Node* current = head;
struct Node* next;
while (current != NULL) {
next = current->next;
free(current);
current = next;
}
}
int main() {
struct Node* head = NULL;
insertAtBeginning(&head, 3);
insertAtBeginning(&head, 2);
insertAtBeginning(&head, 1);
printf("Linked List: ");
printList(head);
freeList(head);
return 0;
}
This implementation of a linked list in C embodies Ritchie’s principles of simplicity and portability. It’s a fundamental data structure that can be easily adapted and used across various platforms and applications.
Closing the Séance: Integrating the Wisdom of the Ages
As our Coder’s Séance comes to an end, the spirits of these programming pioneers begin to fade, leaving behind a wealth of knowledge and inspiration. The candles flicker one last time, and we find ourselves back in the present, surrounded by our modern development environments and cutting-edge technologies.
But the wisdom imparted by these legendary figures remains with us, offering valuable guidance for our coding journeys:
- From Ada Lovelace, we learned to approach programming as both an art and a science, balancing creativity with logical precision.
- Alan Turing taught us the importance of computational thinking and understanding the fundamental concepts that underlie all programming languages.
- Grace Hopper emphasized the power of abstraction and the importance of bridging the gap between human thinking and machine execution.
- John von Neumann reminded us of the crucial relationship between software and hardware, and how understanding computer architecture can lead to more efficient code.
- Dennis Ritchie instilled in us the values of simplicity, portability, and creating tools that stand the test of time.
As we return to our daily coding tasks and prepare for technical interviews, let’s carry these lessons with us. Whether we’re tackling complex algorithms, designing system architectures, or crafting elegant user interfaces, the combined wisdom of these programming pioneers can guide us towards becoming better, more thoughtful developers.
Applying the Spirits’ Wisdom to Modern Coding Challenges
Now that we’ve channeled the spirits of these programming legends, let’s consider how their collective wisdom can be applied to some common coding challenges and interview questions you might encounter, especially when preparing for interviews at top tech companies:
1. Optimizing Algorithms
When faced with a problem that requires optimizing an algorithm, channel Ada Lovelace’s creativity and Alan Turing’s computational thinking. Start by understanding the problem deeply, then consider multiple approaches before settling on a solution. Here’s an example of optimizing a function to find the nth Fibonacci number:
def fibonacci_optimized(n):
if n <= 1:
return n
a, b = 0, 1
for _ in range(2, n + 1):
a, b = b, a + b
return b
# Test the optimized function
print(fibonacci_optimized(100)) # Output: 354224848179261915075
This solution uses dynamic programming principles to achieve O(n) time complexity, a significant improvement over the naive recursive approach.
2. Designing Scalable Systems
When designing systems that need to scale, remember Grace Hopper’s lessons on abstraction and John von Neumann’s insights on efficient computing. Consider this high-level design for a distributed cache system:
import hashlib
class DistributedCache:
def __init__(self, nodes):
self.nodes = nodes
def _get_node(self, key):
hash_value = hashlib.md5(key.encode()).hexdigest()
node_index = int(hash_value, 16) % len(self.nodes)
return self.nodes[node_index]
def set(self, key, value):
node = self._get_node(key)
node.set(key, value)
def get(self, key):
node = self._get_node(key)
return node.get(key)
# Usage
cache_nodes = [Node("192.168.0.1"), Node("192.168.0.2"), Node("192.168.0.3")]
cache = DistributedCache(cache_nodes)
cache.set("user_1", {"name": "Alice", "age": 30})
user_data = cache.get("user_1")
This design uses consistent hashing to distribute data across multiple nodes, allowing for scalability and fault tolerance.
3. Writing Clean, Maintainable Code
When writing code that others will read and maintain, channel Dennis Ritchie’s emphasis on simplicity and portability. Here’s an example of a clean, well-documented Python class:
class BankAccount:
"""A simple bank account class with basic operations."""
def __init__(self, account_number, balance=0):
"""
Initialize a new bank account.
Args:
account_number (str): The account number.
balance (float, optional): Initial balance. Defaults to 0.
"""
self.account_number = account_number
self.balance = balance
def deposit(self, amount):
"""
Deposit money into the account.
Args:
amount (float): The amount to deposit.
Raises:
ValueError: If the amount is negative.
"""
if amount < 0:
raise ValueError("Deposit amount must be positive")
self.balance += amount
def withdraw(self, amount):
"""
Withdraw money from the account.
Args:
amount (float): The amount to withdraw.
Raises:
ValueError: If the amount is negative or exceeds the balance.
"""
if amount < 0:
raise ValueError("Withdrawal amount must be positive")
if amount > self.balance:
raise ValueError("Insufficient funds")
self.balance -= amount
def get_balance(self):
"""Return the current balance of the account."""
return self.balance
# Usage
account = BankAccount("1234567890")
account.deposit(1000)
account.withdraw(500)
print(f"Balance: ${account.get_balance()}") # Output: Balance: $500
This class demonstrates clean code principles with clear documentation, error handling, and a simple, intuitive interface.
Conclusion: The Eternal Legacy of Programming Pioneers
As we conclude our Coder’s Séance, we’re left with a profound appreciation for the giants upon whose shoulders we stand. The spirits of Ada Lovelace, Alan Turing, Grace Hopper, John von Neumann, and Dennis Ritchie have not only shaped the history of computing but continue to influence and inspire modern software development.
Their collective wisdom reminds us that programming is more than just writing code. It’s a blend of art and science, a practice that requires both creativity and logical rigor. It’s about understanding the fundamental principles of computation, creating layers of abstraction, optimizing for hardware, and striving for simplicity and portability.
As you continue your journey in software development, whether you’re preparing for technical interviews at top tech companies or working on personal projects, remember the lessons imparted by these programming pioneers. Embrace the duality of creativity and logic in your problem-solving. Think in terms of computational processes rather than specific languages. Create abstractions that simplify complex systems. Optimize your code with an understanding of the underlying hardware. And always strive for clarity and simplicity in your designs.
The spirits of these programming legends live on in every line of code we write, every algorithm we optimize, and every system we design. By channeling their wisdom, we can push the boundaries of what’s possible in software development and continue to innovate in ways that would make our digital ancestors proud.
So the next time you’re faced with a challenging coding problem or a complex system design, take a moment to invoke the spirits of these programming pioneers. Their timeless wisdom may just provide the inspiration you need to create elegant, efficient, and groundbreaking solutions.
Remember, in the ever-evolving world of technology, we are all part of a grand continuum – learning from the past, innovating in the present, and shaping the future of computing. May the spirits of programming pioneers guide your keystrokes and illuminate your path in the vast digital realm.