Coding in Nursery Rhymes: Simplifying Complex Algorithms for Child-Like Understanding
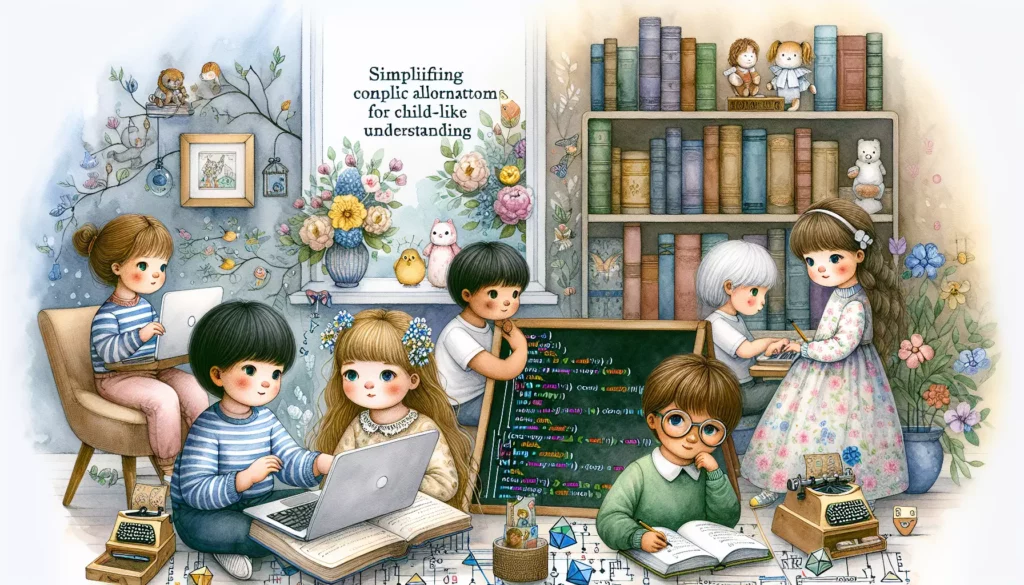
In the world of computer science and programming, algorithms can often seem like complex, intimidating concepts. But what if we could break them down into something as simple and familiar as nursery rhymes? This innovative approach not only makes coding more accessible but also adds a touch of whimsy to the learning process. In this article, we’ll explore how we can use the structure and simplicity of nursery rhymes to explain and understand various algorithms, making them more approachable for beginners and even children.
1. The Importance of Simplifying Algorithms
Before we dive into our rhyming adventures, let’s consider why simplifying algorithms is so crucial:
- Accessibility: Breaking down complex concepts into simpler terms makes coding more accessible to a wider audience, including younger learners.
- Memorability: Rhymes and songs are easier to remember than dry, technical explanations.
- Engagement: A playful approach can make learning more enjoyable and increase motivation to explore further.
- Foundational Understanding: Simplifying algorithms helps build a strong foundation for more advanced concepts later on.
2. “Bubble Sort, Bubble Sort, Floating to the Top”
Let’s start with a classic sorting algorithm: Bubble Sort. Here’s how we can explain it through a nursery rhyme:
Bubble Sort, Bubble Sort, floating to the top,
Compare two numbers, the bigger one will hop.
Swap them if they're wrong, keep them if they're right,
Do this again and again, until all is tight.
One more pass, just to be sure,
If no swaps happen, the list is pure.
Bubble Sort, Bubble Sort, now your list is neat,
From smallest to largest, the order is complete!
This rhyme captures the essence of the Bubble Sort algorithm:
- Compare adjacent elements
- Swap them if they’re in the wrong order
- Repeat the process for the entire list
- Continue until no more swaps are needed
3. “Binary Search, Binary Search, Cut the List in Half”
Next, let’s tackle the efficient searching algorithm, Binary Search:
Binary Search, Binary Search, cut the list in half,
Look at the middle, is it more or less?
If it's what you seek, then you're done with glee,
If it's too small, look right; if too big, look left you'll see.
Keep on splitting, again and again,
Until you find the number, or reach the end.
Binary Search, Binary Search, so quick and so smart,
In sorted lists, it plays a winning part!
This rhyme explains the key steps of Binary Search:
- Start with a sorted list
- Check the middle element
- If it’s the target, you’re done
- If not, eliminate half the list and repeat
4. “Recursive Rhyme, Recursive Rhyme, Calling Itself in Time”
Recursion can be a tricky concept, but let’s break it down with a recursive nursery rhyme:
Recursive Rhyme, Recursive Rhyme, calling itself in time,
I'll tell you a story, but just a line.
If there's more to tell, I'll call once more,
Recursive Rhyme, pick up where I was before.
When the story's done, no more calls we make,
Back up the stack, results we'll take.
Recursive Rhyme, Recursive Rhyme, now you understand,
How functions call themselves, isn't it grand?
This rhyme illustrates the key concepts of recursion:
- A function that calls itself
- A base case to stop the recursion
- Building up the call stack
- Unwinding the stack with results
5. “Merge Sort, Merge Sort, Divide and Conquer All”
Let’s explore the efficient Merge Sort algorithm through a nursery rhyme:
Merge Sort, Merge Sort, divide and conquer all,
Split the list in two, no matter how small.
Keep on splitting, till one item you see,
Then start combining, in order they'll be.
Compare the first of each, take the smaller one,
Place it in order, till both lists are done.
Merge Sort, Merge Sort, efficient and true,
Sorting made easy, just for you!
This rhyme captures the essence of the Merge Sort algorithm:
- Divide the list into smaller sublists
- Continue dividing until you have individual elements
- Merge the sublists back together in sorted order
- Compare elements from each sublist when merging
6. “Graph Traversal, Graph Traversal, Visiting Every Node”
Graph traversal algorithms are fundamental in computer science. Let’s explain them through a nursery rhyme:
Graph Traversal, Graph Traversal, visiting every node,
BFS or DFS, which path will you code?
Breadth-First Search, level by level we go,
Using a queue, neighbors all in a row.
Depth-First Search, dive deep with no fear,
Using a stack, or recursion so clear.
Graph Traversal, Graph Traversal, exploring with glee,
Every vertex and edge, we're sure to see!
This rhyme introduces two main graph traversal algorithms:
- Breadth-First Search (BFS)
- Uses a queue
- Explores neighbors before moving deeper
- Depth-First Search (DFS)
- Uses a stack or recursion
- Explores as far as possible along each branch
7. “Dynamic Programming, Dynamic Programming, Remember What You’ve Done”
Dynamic Programming is a powerful technique for solving complex problems. Let’s break it down with a nursery rhyme:
Dynamic Programming, Dynamic Programming, remember what you've done,
Break the problem down, solve sub-problems one by one.
Store the answers neatly, in a table or array,
Reuse them when needed, no need to compute twice in a day.
Bottom-up or top-down, choose your favorite way,
Optimize your solution, make algorithms less gray.
Dynamic Programming, Dynamic Programming, efficiency at its best,
Solving tricky problems, putting recursion to rest!
This rhyme highlights the key aspects of Dynamic Programming:
- Break down problems into smaller subproblems
- Store solutions to subproblems (memoization)
- Reuse stored solutions to avoid redundant computations
- Can be implemented bottom-up or top-down
8. “Greedy Algorithm, Greedy Algorithm, Always Choose the Best”
Greedy algorithms make locally optimal choices. Let’s explain this concept through a nursery rhyme:
Greedy Algorithm, Greedy Algorithm, always choose the best,
At each step take the most, forget about the rest.
Sometimes it works wonders, solving problems fast,
But be careful, my friend, it may not always last.
Optimal substructure, and greedy choice property,
These are the keys, to greedy algorithm notoriety.
Greedy Algorithm, Greedy Algorithm, quick decisions to make,
But global optimum, it might not always take!
This rhyme outlines the characteristics of greedy algorithms:
- Make the locally optimal choice at each step
- Hope that these choices lead to a global optimum
- Requires optimal substructure and greedy choice property
- May not always give the best overall solution
9. “Hash Table, Hash Table, Find Things in a Flash”
Hash tables are essential data structures for quick lookups. Let’s explain them with a nursery rhyme:
Hash Table, Hash Table, find things in a flash,
Take your key, run it through a special hash.
The result tells you where to store or to seek,
In constant time, you'll find what you need.
Collisions may happen, but don't you fret,
Chaining or probing will help you get.
Hash Table, Hash Table, speedy and true,
O(1) lookups, just for you!
This rhyme covers the main concepts of hash tables:
- Use a hash function to convert keys to array indices
- Allow for quick insertion and lookup operations
- Handle collisions through chaining or open addressing
- Provide average-case O(1) time complexity for operations
10. “Big O Notation, Big O Notation, How Fast Will It Go?”
Understanding time complexity is crucial in algorithm analysis. Let’s explain Big O Notation through a nursery rhyme:
Big O Notation, Big O Notation, how fast will it go?
As input grows larger, complexity will show.
O(1) is constant, it's always the same,
O(log n) is searching, binary search is its name.
O(n) is linear, growing step by step,
O(n log n) for sorting, that's as good as we get.
O(n²) is quadratic, nested loops you'll see,
O(2^n) exponential, complexity breaking free!
Big O Notation, Big O Notation, upper bound so clear,
Helping us compare, which algorithms to hold dear.
This rhyme introduces common time complexities:
- O(1): Constant time
- O(log n): Logarithmic time
- O(n): Linear time
- O(n log n): Linearithmic time
- O(n²): Quadratic time
- O(2^n): Exponential time
Conclusion: The Power of Simplification
By transforming complex algorithms into simple, memorable nursery rhymes, we’ve demonstrated how even the most intricate concepts can be broken down into digestible pieces. This approach not only makes learning more enjoyable but also helps in retaining information and building a strong foundation for more advanced topics.
Remember, the goal of these rhymes is not to provide a comprehensive understanding of each algorithm, but rather to offer a friendly introduction and a memory aid for key concepts. As learners progress, they can build upon this foundation to explore the algorithms in greater depth.
Whether you’re a beginner just starting your coding journey, a teacher looking for innovative ways to explain algorithms, or an experienced programmer wanting a fresh perspective, these algorithm nursery rhymes can serve as a valuable tool in your learning arsenal.
So the next time you encounter a challenging algorithm, why not try breaking it down into a simple rhyme? You might just find that the complex becomes comprehensible, and the daunting becomes delightful. Happy coding, and may your algorithms always flow as smoothly as a well-crafted nursery rhyme!