Pair Programming with Historical Figures: WWAD (What Would Ada Lovelace Do?)
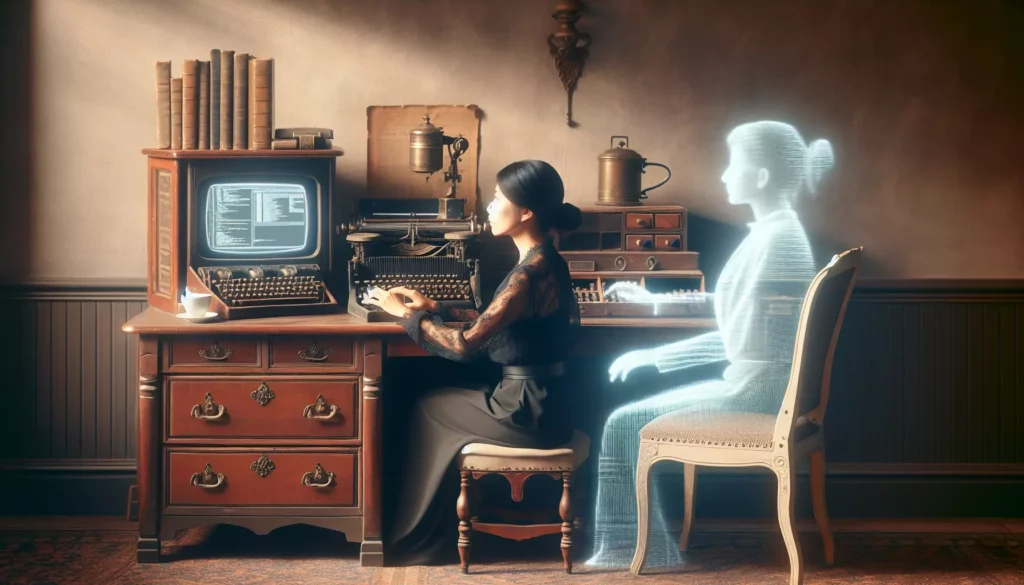
In the ever-evolving world of software development, pair programming has emerged as a powerful technique to enhance code quality, knowledge sharing, and problem-solving skills. But have you ever wondered what it would be like to pair program with some of the greatest minds in computing history? Today, we’re going to embark on an imaginative journey to pair program with none other than Ada Lovelace, the world’s first computer programmer. Welcome to our thought experiment: “What Would Ada Lovelace Do?” (WWAD)
Who Was Ada Lovelace?
Before we dive into our pair programming session, let’s take a moment to appreciate the brilliance of Ada Lovelace. Born in 1815, Augusta Ada King, Countess of Lovelace, was a British mathematician and writer. She is widely recognized as the world’s first computer programmer, thanks to her work on Charles Babbage’s proposed mechanical general-purpose computer, the Analytical Engine.
Lovelace’s notes on the Analytical Engine include what is considered to be the first algorithm intended to be processed by a machine. Her foresight and understanding of computing concepts were far ahead of her time, making her an ideal partner for our imaginary pair programming session.
Setting the Stage for Pair Programming with Ada
Imagine we’ve invented a time machine (because why not?) and brought Ada Lovelace to the present day. After giving her a crash course in modern computing (which she’d probably grasp faster than most of us), we sit down for a pair programming session. Our goal? To solve a complex algorithmic problem while leveraging Ada’s unique perspective and analytical prowess.
The Problem: Implementing a Basic Neural Network
Let’s choose a problem that bridges the gap between Ada’s mathematical background and modern computing: implementing a simple neural network from scratch. This task combines mathematical concepts that Ada would be familiar with (like matrix operations) with modern machine learning principles.
Ada’s Approach: Mathematical Foundations First
As we begin our pair programming session, Ada would likely insist on starting with a solid mathematical foundation. She’d probably say something like, “Before we write a single line of code, we must understand the underlying mathematical principles of neural networks.”
Ada would guide us through the mathematical concepts:
- Matrix operations for weight calculations
- Activation functions and their derivatives
- The chain rule for backpropagation
- Gradient descent for optimization
She might even sketch out the equations on a whiteboard, ensuring we have a clear understanding before proceeding to implementation.
Translating Math to Code
With the mathematical foundation laid out, we’d start translating these concepts into code. Ada, being the analytical thinker she was, would likely advocate for a structured, modular approach. Let’s imagine how she might guide us in implementing the core components of our neural network:
1. Defining the Neural Network Structure
Ada might suggest starting with a clear definition of our neural network structure:
class NeuralNetwork:
def __init__(self, input_size, hidden_size, output_size):
self.input_size = input_size
self.hidden_size = hidden_size
self.output_size = output_size
# Initialize weights and biases
self.weights_input_hidden = np.random.randn(self.input_size, self.hidden_size)
self.bias_hidden = np.zeros((1, self.hidden_size))
self.weights_hidden_output = np.random.randn(self.hidden_size, self.output_size)
self.bias_output = np.zeros((1, self.output_size))
Ada would likely emphasize the importance of properly initializing the weights and biases, drawing parallels to the initial state of the Analytical Engine.
2. Implementing the Activation Function
For the activation function, Ada might suggest using the sigmoid function, as it’s mathematically elegant and bounded between 0 and 1:
def sigmoid(x):
return 1 / (1 + np.exp(-x))
def sigmoid_derivative(x):
return x * (1 - x)
She’d likely explain how this function mimics the firing of neurons in the brain, a concept she’d find fascinating given her interest in the human nervous system.
3. Forward Propagation
Ada would guide us through the forward propagation process, emphasizing the step-by-step nature of the computation:
def forward(self, X):
# Input to hidden layer
self.hidden = np.dot(X, self.weights_input_hidden) + self.bias_hidden
self.hidden_output = sigmoid(self.hidden)
# Hidden to output layer
self.output = np.dot(self.hidden_output, self.weights_hidden_output) + self.bias_output
self.final_output = sigmoid(self.output)
return self.final_output
She might draw parallels between this process and the way the Analytical Engine would process information step by step.
4. Backpropagation
The concept of backpropagation would likely fascinate Ada. She’d probably see it as an elegant way to “debug” the network’s thinking process:
def backward(self, X, y, output):
# Calculate error
self.output_error = y - output
self.output_delta = self.output_error * sigmoid_derivative(output)
# Hidden layer error
self.hidden_error = self.output_delta.dot(self.weights_hidden_output.T)
self.hidden_delta = self.hidden_error * sigmoid_derivative(self.hidden_output)
# Update weights
self.weights_hidden_output += self.hidden_output.T.dot(self.output_delta)
self.weights_input_hidden += X.T.dot(self.hidden_delta)
# Update biases
self.bias_output += np.sum(self.output_delta, axis=0, keepdims=True)
self.bias_hidden += np.sum(self.hidden_delta, axis=0, keepdims=True)
Ada would likely draw connections between this process and her work on the Analytical Engine, noting how the machine could theoretically modify its own instructions based on previous computations.
5. Training the Network
Finally, Ada would guide us in putting it all together in a training function:
def train(self, X, y, epochs):
for _ in range(epochs):
output = self.forward(X)
self.backward(X, y, output)
She might compare this iterative process to the looping capabilities of the Analytical Engine, emphasizing how repeated calculations can lead to increasingly accurate results.
Ada’s Insights and Contributions
Throughout our pair programming session, Ada would likely offer numerous insights that bridge her 19th-century knowledge with modern computing concepts:
1. Emphasis on Algorithmic Thinking
Ada was renowned for her ability to think algorithmically. She would likely emphasize the importance of breaking down complex problems into smaller, manageable steps. This approach aligns perfectly with modern software development practices and would be invaluable in tackling complex coding challenges.
2. Visualization of Data Flow
Given her work on the Analytical Engine, Ada might suggest creating visual representations of data flow within our neural network. This could lead to the development of helpful diagrams or even simple visualization tools to aid in understanding and debugging the network’s behavior.
3. Interdisciplinary Connections
Ada was known for her ability to connect ideas from different fields. She might encourage us to look beyond pure computer science and draw inspiration from other disciplines like biology (neural systems) or physics (optimization principles) to enhance our neural network implementation.
4. Attention to Edge Cases
Ada’s meticulous nature would likely lead her to ask about edge cases and potential pitfalls in our implementation. She might encourage us to add robust error handling and input validation to our code:
def forward(self, X):
if X.shape[1] != self.input_size:
raise ValueError(f"Input size mismatch. Expected {self.input_size}, got {X.shape[1]}")
# ... rest of the forward propagation code ...
5. Documentation and Explanation
Given her background in writing extensive notes on the Analytical Engine, Ada would likely insist on comprehensive documentation for our code. She might guide us in writing clear docstrings and comments to explain the purpose and functionality of each component:
class NeuralNetwork:
"""
A simple feedforward neural network with one hidden layer.
This network uses sigmoid activation functions and implements
backpropagation for training.
Attributes:
input_size (int): Number of input features
hidden_size (int): Number of neurons in the hidden layer
output_size (int): Number of output neurons
... (other attributes) ...
"""
def __init__(self, input_size, hidden_size, output_size):
# ... initialization code ...
Learning from Ada’s Approach
Our imaginary pair programming session with Ada Lovelace offers valuable lessons for modern developers:
1. Start with a Strong Foundation
Ada’s insistence on understanding the mathematical principles before coding reminds us of the importance of a solid theoretical foundation. In the context of AlgoCademy, this translates to thoroughly understanding algorithmic concepts before diving into implementation.
2. Think Algorithmically
Ada’s approach to breaking down complex problems into step-by-step processes is a crucial skill in algorithm design and problem-solving. This aligns perfectly with AlgoCademy’s focus on developing strong algorithmic thinking skills.
3. Embrace Interdisciplinary Thinking
Ada’s ability to connect ideas from different fields encourages us to think beyond traditional computer science boundaries. This can lead to innovative solutions and approaches in tackling coding challenges.
4. Pay Attention to Detail
Ada’s meticulous nature and concern for edge cases remind us of the importance of writing robust, error-resistant code. This is particularly crucial when preparing for technical interviews, where edge case handling is often a key evaluation criterion.
5. Document and Explain Your Work
Ada’s emphasis on documentation aligns with best practices in software development. Clear explanations of your code and thought process are invaluable, both for collaboration and for demonstrating your understanding in interview situations.
Applying WWAD to Your Coding Journey
As you progress through your coding education and prepare for technical interviews, consider adopting the “What Would Ada Do?” (WWAD) mindset:
- Approach problems methodically: Break down complex tasks into smaller, manageable steps.
- Seek deep understanding: Don’t just memorize solutions; strive to understand the underlying principles and mathematics.
- Think creatively: Look for connections between different concepts and don’t be afraid to draw inspiration from various fields.
- Pay attention to details: Consider edge cases and potential errors in your implementations.
- Communicate clearly: Practice explaining your code and thought process, as if you were writing notes for future generations of programmers.
Conclusion: The Timeless Wisdom of Ada Lovelace
Our imaginary pair programming session with Ada Lovelace serves as a reminder that many of the fundamental principles of good programming and problem-solving are timeless. Ada’s approach to understanding complex systems, breaking down problems, and thinking algorithmically remains as relevant today as it was in the 19th century.
As you continue your journey with AlgoCademy, learning to code, solving algorithmic problems, and preparing for technical interviews, channel the spirit of Ada Lovelace. Embrace her analytical thinking, her attention to detail, and her ability to see the bigger picture. By adopting a WWAD mindset, you’ll not only become a better programmer but also develop the kind of analytical and creative thinking skills that are highly valued in the tech industry.
Remember, every time you sit down to code, you’re not just writing instructions for a machine – you’re continuing a legacy of computational thinking that stretches back to the very beginnings of computer science. So, the next time you’re faced with a challenging coding problem, take a moment to ask yourself: “What Would Ada Lovelace Do?”