Extreme Refactoring: Rewriting Famous Algorithms in 140 Characters or Less
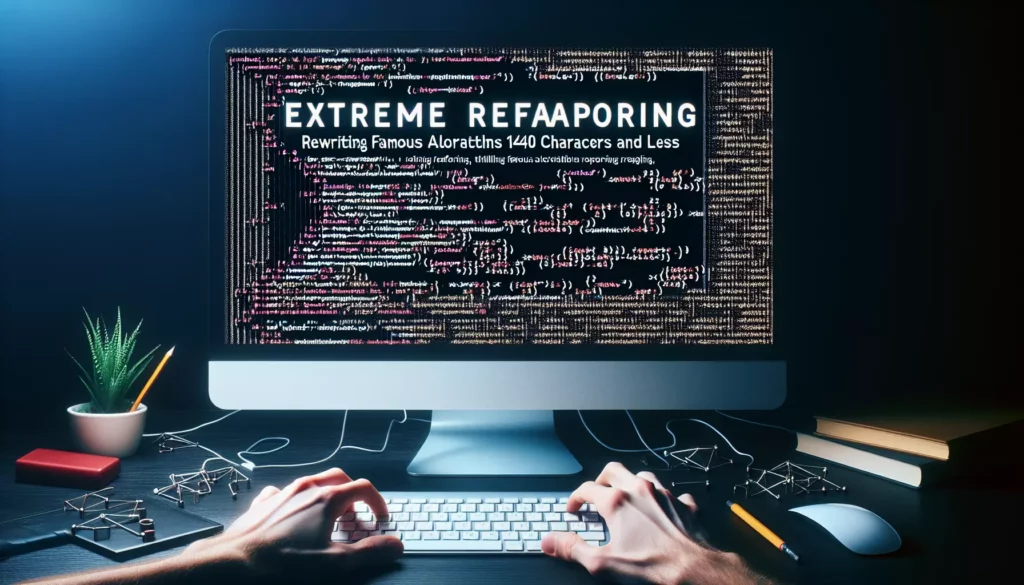
In the world of programming, efficiency and conciseness are often celebrated. But what happens when we push these concepts to the extreme? Welcome to the challenge of rewriting famous algorithms in 140 characters or less – a task that combines the art of algorithm design with the constraints of a tweet-sized code snippet. This exercise not only tests our understanding of algorithms but also pushes us to think creatively about code optimization and readability.
Why 140 Characters?
The choice of 140 characters harkens back to Twitter’s original character limit. While Twitter has since expanded to 280 characters, the 140-character constraint remains an interesting challenge for programmers. It forces us to strip algorithms down to their bare essentials, often leading to innovative approaches and a deeper understanding of the underlying concepts.
The Benefits of Extreme Refactoring
Before we dive into specific algorithms, let’s consider why this exercise is valuable:
- Deep Understanding: To condense an algorithm, you must truly understand its core principles.
- Creative Problem-Solving: The character limit forces you to think outside the box and find novel solutions.
- Code Optimization: You’ll learn to write more efficient code by eliminating unnecessary elements.
- Language Mastery: You’ll become more familiar with language-specific shortcuts and features.
- Fun Challenge: It’s an enjoyable way to test your skills and compete with fellow programmers.
Famous Algorithms in 140 Characters or Less
Let’s explore some well-known algorithms and see how we can rewrite them within our 140-character constraint. We’ll use Python for our examples, as its concise syntax lends itself well to this challenge.
1. Bubble Sort
Bubble Sort is a simple sorting algorithm that repeatedly steps through the list, compares adjacent elements, and swaps them if they’re in the wrong order. Here’s a 140-character implementation:
def b(l):
for i in range(len(l)-1,0,-1):
for j in range(i):
if l[j]>l[j+1]:l[j],l[j+1]=l[j+1],l[j]
return l
This implementation maintains the core logic of Bubble Sort while using shorthand variable names and removing unnecessary whitespace.
2. Binary Search
Binary Search is an efficient algorithm for finding an item from a sorted list of items. Here’s a recursive implementation in 140 characters:
def b(l,x,s=0,e=None):
if e is None:e=len(l)-1
m=(s+e)//2
return m if x==l[m] else b(l,x,s,m-1)if x<l[m]else b(l,x,m+1,e)if s<=e else-1
This version uses recursion and ternary operators to condense the logic into a single line of code.
3. Quicksort
Quicksort is a divide-and-conquer algorithm that picks an element as a pivot and partitions the array around the pivot. Here’s a concise implementation:
def q(l):
return l if len(l)<=1 else q([x for x in l[1:]if x<=l[0]])+[l[0]]+q([x for x in l[1:]if x>l[0]])
This one-liner uses list comprehensions and recursion to implement the Quicksort algorithm elegantly.
4. Depth-First Search (DFS)
DFS is an algorithm for traversing or searching tree or graph data structures. Here’s a 140-character implementation for a graph represented as a dictionary:
def d(g,s,v=None):
if v is None:v=set()
v.add(s)
for n in g[s]-v:d(g,n,v)
return v
This recursive implementation uses a set to keep track of visited nodes and explores as deeply as possible along each branch.
5. Fibonacci Sequence
The Fibonacci sequence is a series of numbers where each number is the sum of the two preceding ones. Here’s a one-line implementation using a lambda function:
f=lambda n:n if n<2 else f(n-1)+f(n-2)
This recursive lambda function calculates the nth Fibonacci number in just 140 characters.
Challenges and Considerations
While these extreme refactoring exercises are fun and educational, they come with some important considerations:
Readability vs. Conciseness
One of the main challenges in this exercise is balancing readability with conciseness. In many cases, the 140-character versions sacrifice readability for brevity. In real-world applications, it’s generally more important to write clear, maintainable code than to minimize character count.
Performance Implications
Some of these condensed versions may not be as performant as their full-length counterparts. For example, the recursive Fibonacci implementation has exponential time complexity, which becomes inefficient for large inputs.
Language-Specific Features
These implementations often rely on language-specific features and syntax. What works in Python might not translate directly to other languages. It’s important to understand the underlying principles rather than focusing solely on the syntax.
Advanced Techniques for Extreme Refactoring
To achieve these compact implementations, several advanced techniques are employed:
1. List Comprehensions
List comprehensions allow us to create lists concisely. They’re particularly useful in algorithms like Quicksort:
[x for x in l[1:] if x <= l[0]]
This creates a list of all elements in l[1:]
that are less than or equal to l[0]
.
2. Lambda Functions
Lambda functions allow us to create small anonymous functions. They’re useful for defining simple functions in a single line, as seen in the Fibonacci example:
f = lambda n: n if n < 2 else f(n-1) + f(n-2)
3. Recursion
Recursion is heavily used in these implementations. It allows us to define algorithms in terms of themselves, often leading to more concise code.
4. Ternary Operators
Ternary operators allow us to write if-else statements in a single line. They’re used extensively in these implementations to save space:
x if condition else y
5. Default Arguments
Default arguments can help reduce the number of characters needed to call a function. For example, in the Binary Search implementation:
def b(l, x, s=0, e=None):
This allows us to call the function with just the list and the search value, while still having the option to specify start and end indices.
Real-World Applications
While these extreme refactoring exercises are primarily academic, the skills and techniques learned can have practical applications:
1. Code Golf
Code Golf is a type of recreational computer programming competition in which participants strive to achieve the shortest possible code that implements a certain algorithm. The skills learned in these 140-character exercises directly apply to Code Golf challenges.
2. Resource-Constrained Environments
In some resource-constrained environments, such as embedded systems or when working with limited storage, the ability to write compact code can be valuable.
3. Understanding Language Features
These exercises force you to explore and understand advanced language features, which can be beneficial when writing any kind of code.
4. Interview Preparation
While you wouldn’t write code like this in an actual interview, the deep understanding of algorithms required for these exercises can be very helpful in technical interviews.
Beyond 140 Characters: The Importance of Clean Code
While the 140-character challenge is an interesting exercise, it’s crucial to remember that in professional software development, clean, readable, and maintainable code is far more important than concise code. Let’s look at some principles of clean code that should be prioritized in real-world scenarios:
1. Meaningful Names
Use descriptive, intention-revealing names for variables, functions, and classes. For example, instead of:
def b(l, x, s=0, e=None):
A cleaner version would be:
def binary_search(sorted_list, target, start=0, end=None):
2. Functions Should Do One Thing
Functions should have a single responsibility. This makes them easier to understand, test, and maintain.
3. Comments and Documentation
While good code should be self-explanatory, comments and documentation are crucial for explaining why certain decisions were made and how to use the code.
4. Proper Formatting
Consistent indentation, line breaks, and spacing make code much easier to read and understand.
5. Error Handling
Proper error handling and input validation are crucial in production code but often omitted in these condensed versions.
Learning from Extreme Refactoring
While we wouldn’t use these 140-character implementations in production code, the exercise of creating them can teach us valuable lessons:
1. Core Algorithm Understanding
To condense an algorithm this much, you need to understand its core principles intimately. This deep understanding is valuable in all programming contexts.
2. Language Mastery
These exercises push you to explore and master advanced language features and idioms.
3. Creative Problem-Solving
The strict character limit forces you to think creatively and approach problems from new angles.
4. Code Optimization
While taken to an extreme here, the skill of optimizing and refactoring code is crucial in software development.
5. Appreciation for Readability
After working with these condensed versions, you’ll likely have a greater appreciation for clean, readable code in your day-to-day work.
Conclusion
Rewriting famous algorithms in 140 characters or less is a challenging and enlightening exercise. It pushes us to understand algorithms at a fundamental level, master programming language features, and think creatively about code optimization. However, it’s crucial to remember that this is primarily an academic exercise. In real-world software development, prioritize writing clean, readable, and maintainable code over extreme conciseness.
These exercises serve as a valuable tool for learning and skill development. They can help prepare you for technical interviews, improve your problem-solving abilities, and deepen your understanding of both algorithms and programming languages. But always remember to apply these skills judiciously in your professional work, balancing the need for efficiency with the equally important needs for readability, maintainability, and robustness.
As you continue your journey in software development, challenge yourself with these exercises, but also challenge yourself to write the cleanest, most understandable code possible. Both skills will serve you well in your programming career.