The Collector’s Approach to Coding: Building a Personal Museum of Elegant Solutions
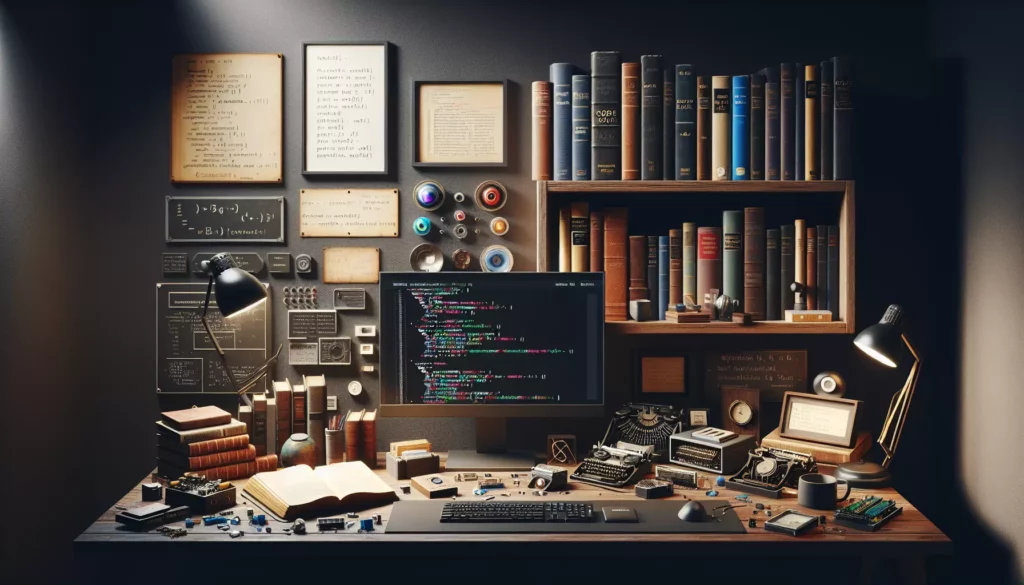
In the vast world of programming, where lines of code stretch as far as the eye can see, there’s a unique approach that can transform the way we learn and grow as developers. Welcome to the collector’s approach to coding – a method that treats elegant solutions and clever algorithms as prized artifacts in a personal museum of programming excellence.
Just as a passionate art collector curates a gallery of masterpieces, a programmer can assemble a collection of coding gems that inspire, educate, and elevate their craft. This blog post will explore how adopting a collector’s mindset can revolutionize your journey in software development, from novice to expert, and help you build a rich repository of knowledge that will serve you throughout your career.
The Art of Code Collection
Before we dive into the specifics of building your code collection, let’s understand what it means to be a code collector:
- Curiosity-Driven: Like collectors in any field, code collectors are driven by an insatiable curiosity to discover new and interesting solutions.
- Appreciation for Craftsmanship: They have a keen eye for well-crafted code, recognizing the beauty in efficiency, readability, and innovation.
- Preservation Mindset: Code collectors understand the value of preserving knowledge and techniques for future reference and learning.
- Continuous Learning: The act of collecting becomes a powerful learning tool, constantly exposing the collector to new ideas and approaches.
Starting Your Collection: The Fundamentals
Every great collection starts with the basics. In the world of coding, this means building a solid foundation of fundamental algorithms and data structures. These are the building blocks upon which more complex solutions are constructed.
1. Sorting Algorithms
Begin your collection with classic sorting algorithms. Each has its unique characteristics and use cases:
- Bubble Sort: Simple but inefficient, great for understanding basic sorting principles.
- Quick Sort: A divide-and-conquer algorithm known for its efficiency.
- Merge Sort: Another efficient sorting algorithm that uses the divide-and-conquer technique.
- Heap Sort: An efficient, comparison-based sorting algorithm.
Here’s an example of a simple but elegant implementation of Quick Sort in Python:
def quick_sort(arr):
if len(arr) <= 1:
return arr
pivot = arr[len(arr) // 2]
left = [x for x in arr if x < pivot]
middle = [x for x in arr if x == pivot]
right = [x for x in arr if x > pivot]
return quick_sort(left) + middle + quick_sort(right)
# Example usage
unsorted_list = [3, 6, 8, 10, 1, 2, 1]
sorted_list = quick_sort(unsorted_list)
print(sorted_list) # Output: [1, 1, 2, 3, 6, 8, 10]
2. Data Structures
No code collection is complete without a robust set of data structures. These form the backbone of efficient algorithm design:
- Arrays and Linked Lists: The most basic linear data structures.
- Stacks and Queues: Essential for many algorithms and real-world applications.
- Trees (Binary Trees, BSTs, AVL Trees): Hierarchical structures with numerous applications.
- Graphs: Powerful for representing complex relationships and networks.
- Hash Tables: The key to efficient lookups and data organization.
Here’s a simple implementation of a binary search tree node in Java:
public class TreeNode {
int val;
TreeNode left;
TreeNode right;
TreeNode(int val) {
this.val = val;
this.left = null;
this.right = null;
}
public void insert(int value) {
if (value <= this.val) {
if (this.left == null) {
this.left = new TreeNode(value);
} else {
this.left.insert(value);
}
} else {
if (this.right == null) {
this.right = new TreeNode(value);
} else {
this.right.insert(value);
}
}
}
}
Expanding Your Collection: Problem-Solving Patterns
As you grow your collection, you’ll start to recognize patterns in problem-solving. These patterns are like the different styles or periods in art – they represent approaches that can be applied across various coding challenges.
1. Two Pointer Technique
This technique is particularly useful for array manipulations and string problems. It involves using two pointers that either move towards each other or in the same direction to solve a problem efficiently.
Here’s an example of using two pointers to reverse a string in C++:
#include <string>
#include <iostream>
using namespace std;
string reverseString(string s) {
int left = 0, right = s.length() - 1;
while (left < right) {
swap(s[left], s[right]);
left++;
right--;
}
return s;
}
int main() {
string input = "Hello, World!";
cout << reverseString(input) << endl; // Output: !dlroW ,olleH
return 0;
}
2. Sliding Window
The sliding window technique is perfect for problems involving subarrays or substrings. It maintains a window that either grows or shrinks as it moves through the data.
Here’s a JavaScript example that finds the maximum sum subarray of size k:
function maxSubarraySum(arr, k) {
if (k > arr.length) return null;
let maxSum = 0;
let windowSum = 0;
// Sum of first k elements
for (let i = 0; i < k; i++) {
windowSum += arr[i];
}
maxSum = windowSum;
// Slide the window
for (let i = k; i < arr.length; i++) {
windowSum = windowSum - arr[i - k] + arr[i];
maxSum = Math.max(maxSum, windowSum);
}
return maxSum;
}
// Example usage
const arr = [1, 4, 2, 10, 23, 3, 1, 0, 20];
const k = 4;
console.log(maxSubarraySum(arr, k)); // Output: 39
3. Divide and Conquer
This classic algorithmic paradigm breaks down a problem into smaller subproblems, solves them, and then combines the results. It’s the foundation for many efficient algorithms like merge sort and quick sort.
4. Dynamic Programming
Dynamic programming is a powerful technique for solving optimization problems by breaking them down into simpler subproblems. It’s particularly useful when the problem has overlapping subproblems and optimal substructure.
Here’s a classic example of dynamic programming: the Fibonacci sequence implemented in Python with memoization:
def fibonacci(n, memo={}):
if n in memo:
return memo[n]
if n <= 1:
return n
memo[n] = fibonacci(n-1, memo) + fibonacci(n-2, memo)
return memo[n]
# Example usage
print(fibonacci(100)) # Output: 354224848179261915075
Advanced Acquisitions: Algorithm Design Techniques
As your collection grows, you’ll want to add more sophisticated pieces. These advanced algorithm design techniques are like the rare, valuable pieces in a collector’s showcase:
1. Greedy Algorithms
Greedy algorithms make locally optimal choices at each step with the hope of finding a global optimum. While they don’t always yield the best solution, when they do, they’re often very efficient.
Here’s an example of a greedy algorithm for the coin change problem in Java:
import java.util.Arrays;
public class CoinChange {
public static int minCoins(int[] coins, int amount) {
Arrays.sort(coins);
int count = 0;
for (int i = coins.length - 1; i >= 0; i--) {
while (amount >= coins[i]) {
amount -= coins[i];
count++;
}
}
return amount == 0 ? count : -1;
}
public static void main(String[] args) {
int[] coins = {1, 5, 10, 25};
int amount = 67;
System.out.println(minCoins(coins, amount)); // Output: 5 (25 + 25 + 10 + 5 + 2)
}
}
2. Backtracking
Backtracking is an algorithmic technique that considers searching every possible combination in order to solve a computational problem. It’s particularly useful for solving constraint satisfaction problems.
Here’s a classic example of backtracking: solving the N-Queens problem in Python:
def solve_n_queens(n):
def is_safe(board, row, col):
# Check this row on left side
for i in range(col):
if board[row][i] == 1:
return False
# Check upper diagonal on left side
for i, j in zip(range(row, -1, -1), range(col, -1, -1)):
if board[i][j] == 1:
return False
# Check lower diagonal on left side
for i, j in zip(range(row, n, 1), range(col, -1, -1)):
if board[i][j] == 1:
return False
return True
def solve(board, col):
if col >= n:
return True
for i in range(n):
if is_safe(board, i, col):
board[i][col] = 1
if solve(board, col + 1):
return True
board[i][col] = 0
return False
board = [[0 for _ in range(n)] for _ in range(n)]
if solve(board, 0) == False:
print("Solution does not exist")
return False
return board
# Example usage
n = 4
solution = solve_n_queens(n)
if solution:
for row in solution:
print(row)
3. Graph Algorithms
Graph algorithms are essential for solving problems involving networks, paths, and connections. Some key algorithms to add to your collection include:
- Depth-First Search (DFS) and Breadth-First Search (BFS)
- Dijkstra’s Algorithm for finding shortest paths
- Kruskal’s and Prim’s algorithms for minimum spanning trees
- Topological Sorting for directed acyclic graphs
Here’s an implementation of Depth-First Search in C++:
#include <iostream>
#include <vector>
#include <stack>
using namespace std;
class Graph {
int V;
vector<vector<int>> adj;
public:
Graph(int v) : V(v), adj(v) {}
void addEdge(int v, int w) {
adj[v].push_back(w);
}
void DFS(int s) {
vector<bool> visited(V, false);
stack<int> stack;
stack.push(s);
while (!stack.empty()) {
s = stack.top();
stack.pop();
if (!visited[s]) {
cout << s << " ";
visited[s] = true;
}
for (auto i = adj[s].rbegin(); i != adj[s].rend(); ++i) {
if (!visited[*i])
stack.push(*i);
}
}
}
};
int main() {
Graph g(4);
g.addEdge(0, 1);
g.addEdge(0, 2);
g.addEdge(1, 2);
g.addEdge(2, 0);
g.addEdge(2, 3);
g.addEdge(3, 3);
cout << "Depth First Traversal (starting from vertex 2): ";
g.DFS(2);
return 0;
}
Curating Your Collection: Best Practices
As your code collection grows, it’s important to curate it effectively. Here are some best practices to keep your personal museum of elegant solutions organized and valuable:
1. Documentation is Key
Just as a museum curator would carefully label and describe each piece in an exhibit, you should document your code snippets thoroughly. Include:
- A brief description of what the code does
- The problem it solves
- Time and space complexity analysis
- Any specific use cases or limitations
2. Categorize and Tag
Organize your collection into categories and use tags to make it easy to find specific solutions later. Some possible categories include:
- Data Structures
- Sorting Algorithms
- Search Algorithms
- Dynamic Programming Solutions
- Graph Algorithms
- String Manipulation
3. Version Control
Use a version control system like Git to track changes to your collection over time. This allows you to see how your understanding and implementation of algorithms evolve.
4. Regular Review and Refactoring
Periodically review your collection. As you grow as a programmer, you may find ways to improve or optimize your earlier solutions. This process of refactoring keeps your collection fresh and up-to-date.
5. Share and Collaborate
Consider sharing your collection with others. This could be through a blog, a GitHub repository, or discussions with fellow programmers. Collaboration can lead to new insights and improvements in your code.
The Benefits of Building Your Code Collection
Adopting a collector’s approach to coding offers numerous benefits:
- Deep Understanding: By curating a collection of elegant solutions, you gain a deeper understanding of algorithmic principles and coding techniques.
- Problem-Solving Skills: Your collection becomes a toolbox of techniques that you can apply to new problems, enhancing your problem-solving abilities.
- Interview Preparation: A well-curated collection is an excellent resource for preparing for technical interviews, especially for positions at major tech companies.
- Continuous Learning: The act of collecting and curating code keeps you engaged in continuous learning and improvement.
- Code Reusability: Your collection becomes a personal library of reusable code snippets that you can adapt for future projects.
Expanding Your Horizons: Beyond Classic Algorithms
While classic algorithms form the core of any good code collection, don’t hesitate to expand into more specialized areas:
1. Machine Learning Algorithms
As AI and machine learning continue to grow in importance, consider adding implementations of key ML algorithms to your collection:
- Linear Regression
- Logistic Regression
- K-Means Clustering
- Decision Trees
- Neural Network basics
Here’s a simple implementation of linear regression using gradient descent in Python:
import numpy as np
def linear_regression(X, y, learning_rate=0.01, iterations=1000):
m = len(y)
theta = np.zeros(X.shape[1])
for _ in range(iterations):
h = np.dot(X, theta)
gradient = np.dot(X.T, (h - y)) / m
theta -= learning_rate * gradient
return theta
# Example usage
X = np.array([[1, 1], [1, 2], [1, 3]])
y = np.array([1, 2, 3])
theta = linear_regression(X, y)
print("Theta:", theta)
2. Cryptography Algorithms
Understanding basic cryptography algorithms can be valuable, especially for security-minded developers:
- Caesar Cipher
- RSA Algorithm
- Diffie-Hellman Key Exchange
3. Parsing and Compilation Techniques
For those interested in language design or working with compilers:
- Recursive Descent Parsing
- Lexical Analysis
- Abstract Syntax Tree (AST) construction
The Journey of a Code Collector
As you embark on your journey as a code collector, remember that it’s not just about accumulating a large number of algorithms and solutions. It’s about understanding each piece in your collection, appreciating its elegance, and knowing when and how to apply it.
Your collection will evolve as you grow as a programmer. What starts as a simple array of basic sorting algorithms might grow into a comprehensive library of solutions spanning multiple domains of computer science.
Here are some milestones you might encounter in your journey:
- The Novice Collector: You begin by gathering fundamental algorithms and data structures, focusing on understanding and implementing them correctly.
- The Curious Explorer: As you gain confidence, you start exploring different problem-solving techniques and more advanced algorithms.
- The Efficient Optimizer: You begin to focus on optimizing your solutions, considering time and space complexity, and refining your existing collection.
- The Creative Innovator: You start combining different techniques to create novel solutions to complex problems.
- The Wise Curator: Your collection becomes a well-organized, comprehensive resource that you can effortlessly navigate and apply to real-world problems.
Conclusion: Your Personal Museum of Code
Building a personal museum of elegant coding solutions is more than just a hobby or a study aid – it’s a powerful approach to mastering the art of programming. By carefully collecting, studying, and curating a diverse array of algorithms and techniques, you create a valuable resource that grows with you throughout your career.
This collector’s approach to coding aligns perfectly with the goals of platforms like AlgoCademy, which aims to help learners progress from beginner-level coding to mastering complex algorithms and acing technical interviews. Your personal code collection becomes a tangible representation of your journey, showcasing your growth, understanding, and the elegant solutions you’ve mastered along the way.
Remember, every great programmer has, in essence, built their own collection of coding knowledge over time. By consciously adopting the collector’s mindset, you accelerate this process, creating a rich, personal resource that will serve you well in your studies, your interview preparations, and throughout your professional life.
So, start your collection today. Begin with the basics, expand into more complex territories, and don’t forget to appreciate the beauty and elegance of each solution you add to your personal museum of code. Happy collecting!