Guerrilla Coding: Unconventional Tactics for Surviving Surprise Technical Interviews
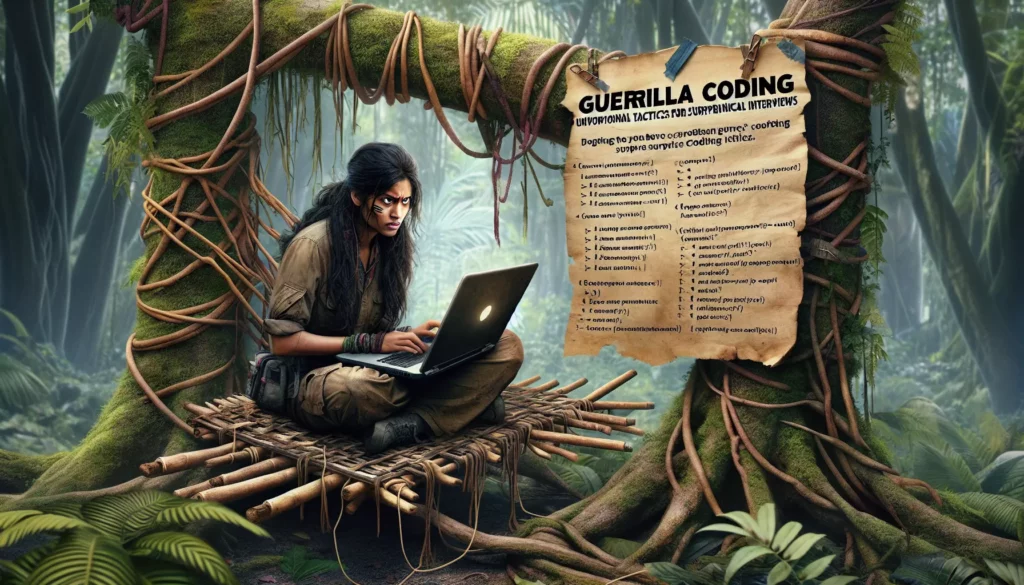
In the high-stakes world of tech recruitment, surprise technical interviews have become an increasingly common hurdle for aspiring developers. These unexpected challenges can catch even the most prepared candidates off guard, testing not just their coding skills but also their ability to think on their feet. This article will explore unconventional tactics—what we’re calling “guerrilla coding”—to help you survive and thrive in these pressure-cooker situations.
Understanding the Surprise Technical Interview
Before diving into tactics, it’s crucial to understand what a surprise technical interview entails. Unlike scheduled interviews where you have time to prepare, these impromptu sessions can occur at any point during the hiring process. They might happen during an initial phone screening, a casual meet-and-greet, or even as an unexpected addition to a planned interview.
The goals of surprise interviews are multifaceted:
- To assess a candidate’s raw problem-solving abilities
- To evaluate how well they perform under pressure
- To gauge their real-time coding skills without preparation
- To observe their communication and thought processes in action
Given these objectives, traditional preparation methods might fall short. This is where guerrilla coding tactics come into play.
Guerrilla Coding Tactic #1: The Mental Code Library
One of the most effective guerrilla coding tactics is developing a mental library of code snippets and algorithms. This involves internalizing common coding patterns and solutions to the point where you can recall and adapt them quickly.
Building Your Mental Library
To build your mental code library:
- Focus on Fundamentals: Memorize the basic structure of key algorithms like binary search, quicksort, and depth-first search.
- Practice Implementation: Regularly implement these algorithms from scratch to reinforce your understanding.
- Create Mnemonics: Develop memory tricks to quickly recall algorithm steps or code structures.
- Visualize Code: Practice visualizing code execution in your mind, strengthening your ability to “see” solutions without writing them.
Example: Quick Binary Search Recall
Here’s a mnemonic to quickly recall the structure of a binary search:
LAMP: Low, Assert, Mid, Probe
L - Set Low to 0, High to array length - 1
A - Assert condition (while Low <= High)
M - Calculate Mid
P - Probe: compare, then adjust Low or High
With practice, this mnemonic can help you quickly implement a binary search even under pressure.
Guerrilla Coding Tactic #2: The Skeleton Key Approach
The Skeleton Key Approach involves mastering a set of versatile problem-solving patterns that can be adapted to a wide range of coding challenges. By deeply understanding these patterns, you can quickly recognize which one to apply in a given situation.
Key Problem-Solving Patterns
- Two Pointers: Useful for array manipulation, string problems, and linked list operations.
- Sliding Window: Effective for substring problems and contiguous sequence challenges.
- Fast and Slow Pointers: Ideal for cycle detection in linked lists or arrays.
- Merge Intervals: Helpful for scheduling and time-based problems.
- Dynamic Programming: Crucial for optimization problems and certain types of counting problems.
Applying the Skeleton Key Approach
When faced with a problem in a surprise interview:
- Quickly analyze the problem statement.
- Identify which pattern(s) might be applicable.
- Adapt the pattern to fit the specific problem requirements.
For example, if you encounter a problem involving finding a subarray with a specific sum, you can quickly recognize it as a potential sliding window problem and adapt that pattern to solve it.
Guerrilla Coding Tactic #3: The Rapid Prototyping Technique
In a surprise interview, time is of the essence. The Rapid Prototyping Technique involves quickly sketching out a basic solution and iteratively improving it. This approach allows you to demonstrate your problem-solving process and ability to optimize code on the fly.
Steps for Rapid Prototyping
- Sketch the Brute Force: Quickly outline the simplest possible solution, even if it’s inefficient.
- Communicate Your Approach: Explain your initial solution and its limitations to the interviewer.
- Iterative Improvement: Identify bottlenecks and suggest improvements step by step.
- Code Refactoring: Implement improvements, explaining your thought process as you go.
Example: Improving a Solution
Let’s say you’re asked to find the kth largest element in an unsorted array. Your rapid prototyping process might look like this:
- Brute Force: Sort the array and return the kth element from the end.
- Improvement 1: Use a min-heap of size k to reduce space complexity.
- Improvement 2: Implement QuickSelect algorithm for average O(n) time complexity.
Here’s a sketch of the QuickSelect improvement:
def quickSelect(arr, k):
k = len(arr) - k # Convert to kth smallest
def partition(left, right):
pivot = arr[right]
i = left
for j in range(left, right):
if arr[j] <= pivot:
arr[i], arr[j] = arr[j], arr[i]
i += 1
arr[i], arr[right] = arr[right], arr[i]
return i
def select(left, right):
if left == right:
return arr[left]
pivotIndex = partition(left, right)
if k == pivotIndex:
return arr[k]
elif k < pivotIndex:
return select(left, pivotIndex - 1)
else:
return select(pivotIndex + 1, right)
return select(0, len(arr) - 1)
By rapidly prototyping and improving your solution, you demonstrate not just coding ability but also problem-solving skills and the capacity to optimize code—key qualities that interviewers look for.
Guerrilla Coding Tactic #4: The Pseudocode Parachute
When faced with a complex problem or if you’re drawing a blank on the exact syntax, the Pseudocode Parachute can be a lifesaver. This tactic involves quickly drafting a solution in pseudocode, which can help you organize your thoughts and demonstrate your problem-solving approach even if you can’t remember specific language details.
Benefits of the Pseudocode Parachute
- Allows you to focus on the algorithm rather than syntax
- Demonstrates your ability to break down complex problems
- Provides a roadmap for implementation if time allows
- Shows interviewers your thought process, which is often more valuable than perfect syntax
How to Deploy the Pseudocode Parachute
- Start by clearly stating the problem and your understanding of it.
- Outline the main steps of your algorithm in plain English or a mix of English and code-like statements.
- Use indentation to show structure and flow.
- Include comments about time and space complexity considerations.
- If possible, start translating pseudocode into actual code, prioritizing the most critical parts.
Example: Graph Traversal Pseudocode
Suppose you’re asked to implement a function to find the shortest path in a graph, but you’re unsure about the exact implementation of a priority queue in your language. Here’s how you might use the Pseudocode Parachute:
Function FindShortestPath(graph, start, end):
// Initialize distances and priority queue
Set all distances to infinity except start (0)
Create priority queue PQ
Add (start, 0) to PQ
While PQ is not empty:
current_node, current_distance = PQ.pop_minimum()
If current_node is end:
Return current_distance
For each neighbor of current_node:
new_distance = current_distance + edge_weight
If new_distance < distance[neighbor]:
Update distance[neighbor]
Add (neighbor, new_distance) to PQ
Return "No path found"
// Note: This is Dijkstra's algorithm
// Time complexity: O((V + E) log V) with binary heap
// Space complexity: O(V) for the priority queue and distance array
// TODO: Implement priority queue if time allows
By using the Pseudocode Parachute, you’ve demonstrated your understanding of Dijkstra’s algorithm and graph traversal concepts, even if you couldn’t immediately recall the exact implementation details of a priority queue.
Guerrilla Coding Tactic #5: The Test-Driven Survival Strategy
In the heat of a surprise interview, it’s easy to rush into coding without properly validating your solution. The Test-Driven Survival Strategy borrows from test-driven development practices to help you write more robust code and catch errors early, even under pressure.
Implementing the Test-Driven Survival Strategy
- Start with Edge Cases: Before writing any implementation code, quickly jot down test cases for edge scenarios.
- Write Simple Tests: Create basic test cases that cover the main functionality.
- Implement Incrementally: Write just enough code to pass one test at a time.
- Refactor as You Go: Clean up your code after each passing test.
- Verbalize Your Process: Explain your testing strategy to the interviewer as you work.
Example: Testing a String Reversal Function
Let’s say you’re asked to implement a function to reverse a string. Here’s how you might apply the Test-Driven Survival Strategy:
def reverse_string(s):
# Implementation to be added
# Test cases
assert reverse_string("") == "", "Empty string should return empty string"
assert reverse_string("a") == "a", "Single character should return itself"
assert reverse_string("hello") == "olleh", "Basic string reversal"
assert reverse_string("Hello, World!") == "!dlroW ,olleH", "String with punctuation"
# Implement the function
def reverse_string(s):
return s[::-1]
# Run tests again to verify
print("All tests passed!")
By starting with tests, you ensure that you’ve considered various scenarios and have a clear goal for your implementation. This approach can help you catch errors early and demonstrate a methodical, quality-focused coding style to your interviewer.
Guerrilla Coding Tactic #6: The Analogy Arsenal
Sometimes, the best way to approach a complex coding problem is to draw analogies to real-world situations or simpler problems you’re more familiar with. The Analogy Arsenal tactic involves building a repertoire of analogies that can help you quickly understand and explain complex algorithms or data structures.
Building Your Analogy Arsenal
- Identify core computer science concepts and algorithms.
- For each concept, create a relatable real-world analogy.
- Practice explaining these concepts using your analogies.
- Use these analogies to help you reason about problems during interviews.
Example Analogies
- Hash Tables: Like a library card catalog, allowing quick lookup of book locations.
- Binary Search Trees: Similar to a family tree, with each person having at most two children.
- Depth-First Search: Exploring a maze by always choosing the leftmost unexplored path until you hit a dead end, then backtracking.
- Quicksort: Organizing a bookshelf by choosing a book as a divider and moving all shorter books to the left and taller ones to the right, then repeating for each section.
Applying Analogies in Interviews
When faced with a problem, try to relate it to one of your analogies. This can help you:
- Quickly grasp the core of the problem
- Explain your thought process clearly to the interviewer
- Come up with creative solutions by thinking outside the box
For example, if asked to implement a cache with O(1) lookup and insertion time, you might think of a coat check room. This analogy can lead you to consider using a hash table for quick lookups and a doubly linked list for maintaining order, effectively describing the structure of an LRU (Least Recently Used) cache.
Guerrilla Coding Tactic #7: The Time Complexity Compass
In technical interviews, understanding and optimizing time complexity is crucial. The Time Complexity Compass tactic involves quickly estimating the efficiency of your algorithms and using that information to guide your problem-solving approach.
Using the Time Complexity Compass
- Assess the input size constraints given in the problem.
- Estimate the maximum allowable time complexity based on those constraints.
- Use this estimate to eliminate certain approaches and guide you towards efficient solutions.
Time Complexity Quick Reference
- O(1) – Constant time: Suitable for any input size
- O(log n) – Logarithmic time: Suitable for very large inputs
- O(n) – Linear time: Good for inputs up to millions of elements
- O(n log n) – Linearithmic time: Acceptable for moderately large inputs
- O(n^2) – Quadratic time: May be too slow for inputs larger than a few thousand elements
- O(2^n) – Exponential time: Typically only suitable for very small inputs (n < 20)
Example: Applying the Time Complexity Compass
Suppose you’re given a problem to find all pairs of numbers in an array that sum to a target value. The array size is up to 10^5 elements.
Applying the Time Complexity Compass:
- Input size: 10^5
- Estimated maximum allowable complexity: O(n log n) or O(n)
- Eliminate O(n^2) brute force approach
- Consider solutions using sorting (O(n log n)) or hash tables (O(n))
Based on this analysis, you might implement a solution using a hash table to achieve O(n) time complexity:
def find_pairs(arr, target):
seen = set()
pairs = []
for num in arr:
complement = target - num
if complement in seen:
pairs.append((num, complement))
seen.add(num)
return pairs
# Example usage
arr = [1, 5, 7, 1, 5, 3, 4, 2]
target = 6
result = find_pairs(arr, target)
print(result) # Output: [(5, 1), (3, 3), (4, 2)]
By using the Time Complexity Compass, you’ve quickly arrived at an efficient solution that meets the problem’s constraints.
Guerrilla Coding Tactic #8: The Modular Mindset
When tackling complex problems under pressure, it’s easy to get overwhelmed. The Modular Mindset tactic involves breaking down the problem into smaller, manageable modules. This approach not only makes the problem easier to solve but also demonstrates your ability to write clean, organized code.
Implementing the Modular Mindset
- Analyze the problem and identify distinct components or steps.
- Create a high-level outline of functions or modules you’ll need.
- Implement each module separately, starting with the most critical or complex parts.
- Use clear function names and add brief comments to explain each module’s purpose.
- Combine the modules to create the final solution.
Benefits of the Modular Mindset
- Easier debugging and testing of individual components
- Improved code readability and maintainability
- Flexibility to optimize or change specific parts of the solution
- Demonstrates your ability to write production-quality code
Example: Modular Approach to Solving a Maze
Let’s say you’re asked to write a function that finds a path through a maze. Here’s how you might apply the Modular Mindset:
def solve_maze(maze):
start = find_start(maze)
end = find_end(maze)
path = find_path(maze, start, end)
return path
def find_start(maze):
# Implementation to find the starting point
pass
def find_end(maze):
# Implementation to find the ending point
pass
def find_path(maze, start, end):
# Implementation of pathfinding algorithm (e.g., BFS or DFS)
pass
def is_valid_move(maze, position):
# Check if a move is valid within the maze
pass
# Main solver function
def solve_maze(maze):
start = find_start(maze)
end = find_end(maze)
return find_path(maze, start, end)
# Example usage
maze = [
["#", "#", "#", "#", "#"],
["S", ".", "#", ".", "#"],
["#", ".", ".", ".", "#"],
["#", "#", "#", ".", "E"],
["#", "#", "#", "#", "#"]
]
path = solve_maze(maze)
print(path) # Output: [(1,0), (1,1), (2,1), (2,2), (2,3), (3,3), (3,4)]
By breaking down the maze-solving problem into smaller functions, you’ve created a more manageable and readable solution. This approach allows you to focus on one part of the problem at a time, making it easier to handle the complexity of the overall task.
Conclusion: Mastering the Art of Guerrilla Coding
Surviving surprise technical interviews requires more than just coding knowledge—it demands quick thinking, adaptability, and a strategic approach to problem-solving. The guerrilla coding tactics we’ve explored in this article are designed to give you an edge in these high-pressure situations:
- The Mental Code Library
- The Skeleton Key Approach
- The Rapid Prototyping Technique
- The Pseudocode Parachute
- The Test-Driven Survival Strategy
- The Analogy Arsenal
- The Time Complexity Compass
- The Modular Mindset
By incorporating these tactics into your interview preparation and problem-solving toolkit, you’ll be better equipped to handle unexpected challenges and demonstrate your coding prowess under pressure. Remember, the goal of these tactics is not just to solve the problem at hand, but to showcase your thought process, adaptability, and approach to complex coding challenges.
As you continue to hone your skills, consider incorporating these guerrilla coding tactics into your regular practice routine. Platforms like AlgoCademy offer interactive coding tutorials and resources that can help you sharpen these skills and prepare for technical interviews with major tech companies.
With persistence and practice, you’ll find that these unconventional tactics become second nature, allowing you to approach even the most surprising technical interviews with confidence and skill. Happy coding, and may your future interviews be successful conquests in the world of guerrilla coding!