Method Acting for Coders: Becoming One with Your Code for Deep Understanding
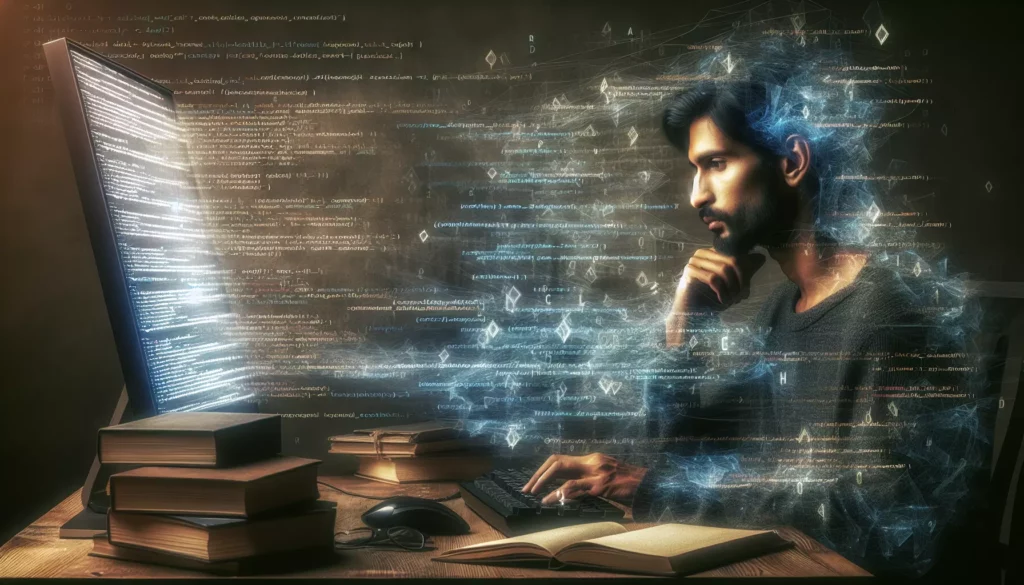
In the world of programming, understanding your code on a deep level is crucial for creating efficient, elegant, and bug-free solutions. But how can we achieve this level of comprehension? Enter “Method Acting for Coders,” a unique approach that borrows from the dramatic arts to help programmers truly embody their code. In this comprehensive guide, we’ll explore how adopting the mindset of a method actor can revolutionize your coding practice and take your skills to the next level.
What is Method Acting?
Before we dive into how method acting applies to coding, let’s briefly explore what method acting is in its original context. Method acting is a technique developed by Constantin Stanislavski and later refined by Lee Strasberg. It involves actors fully immersing themselves in their characters, drawing on their own emotions and experiences to create authentic performances.
Key principles of method acting include:
- Emotional memory: Drawing on personal experiences to inform character emotions
- Sense memory: Recreating physical sensations to enhance performance
- Character analysis: Deeply understanding a character’s motivations and background
- Improvisation: Spontaneously reacting in character to unexpected situations
Now, you might be wondering, “What does this have to do with coding?” As it turns out, quite a lot!
The Parallels Between Acting and Coding
At first glance, acting and coding might seem like completely different disciplines. However, there are several parallels that make the method acting approach relevant to programming:
- Character/Code Understanding: Just as actors must understand their characters inside and out, programmers need to comprehend every aspect of their code.
- Emotional Connection: Actors connect emotionally with their characters; programmers can benefit from developing an emotional connection to their code.
- Improvisation: Actors must react spontaneously on stage; programmers often need to adapt quickly to new requirements or bugs.
- Performance: Both acting and coding culminate in a performance – whether on stage or when the program runs.
With these parallels in mind, let’s explore how we can apply method acting techniques to coding.
Becoming One with Your Code: Method Acting Techniques for Programmers
1. Character Analysis: Dissecting Your Code
In method acting, actors perform a detailed character analysis to understand their role’s background, motivations, and behaviors. As a programmer, you can apply this technique to your code:
- Code Biography: Write a detailed “biography” for your code. What problem is it solving? What’s its history? How has it evolved?
- Function Motivations: For each function or method, define its “motivation.” What is its primary purpose? What drives it to perform its task?
- Variable Personalities: Think of variables as characters. What role do they play in the overall story of your code?
Example: Let’s say you’re working on a sorting algorithm. Your “character analysis” might look like this:
Algorithm Biography:
Name: QuickSort
Born: 1959, created by Tony Hoare
Motivation: To efficiently sort large datasets
Personality: Fast, divide-and-conquer mentality, works well under pressure
Weaknesses: Can struggle with already sorted lists
Function Motivation:
partition(): To divide and organize, creating order from chaos
quickSort(): To oversee the entire sorting process, delegating tasks to partition()
Variable Personalities:
pivot: The decision-maker, sets the standard for comparison
left: The eager helper, always looking to move forward
right: The careful checker, ensuring nothing is left behind
2. Emotional Memory: Connecting with Your Code
Method actors tap into their personal emotional experiences to inform their performances. As a coder, you can use this technique to develop a deeper connection with your code:
- Code Emotions: Associate emotions with different parts of your code. How does a particularly elegant solution make you feel? What about a stubborn bug?
- Problem-Solving Memories: Recall the emotions you experienced when solving similar problems in the past. How can these inform your current coding process?
- User Empathy: Imagine the emotions of the end-user interacting with your program. How can you translate these into code improvements?
Exercise: Next time you’re debugging, don’t just think about the logical steps. Try to recall the frustration of past debugging sessions, the excitement of finding a solution, and channel these emotions into your problem-solving process.
3. Sense Memory: Visualizing Code Execution
Actors use sense memory to recreate physical sensations, making their performances more authentic. Programmers can adapt this technique to better understand code execution:
- Code Visualization: Mentally (or physically) act out the flow of your code. Move around your space as if you were a variable being passed between functions.
- Data Structure Physicalization: Create physical representations of data structures. Use objects to represent nodes in a linked list or stack chairs to visualize a stack data structure.
- Algorithm Choreography: Design a dance or series of movements that represent your algorithm’s steps. Perform it to internalize the process.
Example: To understand a bubble sort algorithm, line up some books of different heights. Physically swap them as you would in the algorithm, feeling the weight of each “swap” operation.
4. Improvisation: Adapting to Code Changes
Improvisation is a key skill for both actors and programmers. Here’s how to apply improv techniques to your coding:
- Code Scenarios: Practice reacting to unexpected code scenarios. Have a colleague introduce a random bug or new requirement, and adapt your code on the spot.
- “Yes, and” Coding: When faced with new features or changes, adopt the improv mindset of “Yes, and.” Accept the change and build upon it creatively.
- Pair Programming Improv: Engage in spontaneous pair programming sessions where you build on each other’s code without extensive planning.
Exercise: Set up a “code improv” session with your team. Take turns introducing unexpected elements (new requirements, performance issues, etc.) and challenge each other to adapt the code in real-time.
Practical Applications of Method Acting in Coding
Now that we’ve explored the techniques, let’s look at how method acting for coders can be applied in real-world scenarios:
1. Debugging Like a Detective
Approach debugging as if you’re a detective in a crime drama. Immerse yourself in the role:
- Create a “crime scene” for your bug. What evidence do you have? What are the witness statements (error messages, logs)?
- Develop hypotheses and test them methodically.
- Interview your “suspects” (different parts of the code) by stepping through with a debugger.
Example scenario:
// The crime scene
function calculateTotal(items) {
let total = 0;
for (let i = 0; i <= items.length; i++) {
total += items[i].price;
}
return total;
}
// The witness statement (console output)
// Uncaught TypeError: Cannot read property 'price' of undefined
As a detective, you might reason: “The error suggests we’re trying to access a property of an undefined item. This likely means we’re going beyond the array bounds. Let’s check our loop condition…”
2. Refactoring as Character Development
When refactoring code, think of it as developing a character’s arc in a story:
- Identify the “character flaws” in your current code (inefficiencies, lack of clarity, etc.).
- Envision the “character growth” – how can the code evolve to become better?
- Implement changes that align with this growth, maintaining the core “personality” (functionality) of the code.
Before refactoring:
function doTheThing(x, y, z) {
let a = x + y;
let b = a * z;
let c = Math.pow(b, 2);
return c;
}
After refactoring (character development):
function calculateSquaredResult(base, addend, multiplier) {
const sum = base + addend;
const product = sum * multiplier;
return Math.pow(product, 2);
}
3. Writing Documentation as Scriptwriting
Approach documentation as if you’re writing a script for your code to perform:
- Write an engaging “synopsis” for your README file.
- Create “character descriptions” for key functions and classes.
- Develop “dialogue” (API descriptions) that clearly communicates how different parts of your code interact.
Example of a function description in JSDoc format:
/**
* The hero of our sorting saga. Brings order to chaos with lightning speed.
*
* @param {Array} arr - The unruly mob of elements yearning to be sorted.
* @param {number} low - The starting point of our hero's journey.
* @param {number} high - The final destination, where order shall be restored.
* @returns {Array} - The same array, but transformed by the power of QuickSort.
*/
function quickSort(arr, low, high) {
// ... implementation details ...
}
Overcoming Challenges in Method Acting for Coders
While the method acting approach can be powerful, it’s not without its challenges. Here are some common obstacles and how to overcome them:
1. Maintaining Objectivity
Challenge: Getting too emotionally invested in your code can cloud your judgment.
Solution: Practice “stepping out of character.” Regularly take breaks to assess your code objectively. Use code reviews and pair programming to get external perspectives.
2. Balancing Creativity and Structure
Challenge: The creative aspects of method acting might clash with the structured nature of coding.
Solution: Use creativity in the problem-solving and design phases, but maintain discipline in implementation. Follow coding standards and best practices while allowing your “character” to inform your approach.
3. Time Management
Challenge: Deep immersion in your code can lead to losing track of time.
Solution: Set clear boundaries and use techniques like the Pomodoro method to maintain focus while taking regular breaks. Think of these breaks as “intermissions” in your coding performance.
Measuring the Impact of Method Acting on Coding Performance
To ensure that the method acting approach is truly beneficial, it’s important to measure its impact on your coding performance. Here are some metrics you can use:
- Code Quality: Use static analysis tools to measure improvements in code complexity, maintainability, and adherence to best practices.
- Bug Reduction: Track the number of bugs found in code written using the method acting approach compared to your previous work.
- Time to Solution: Measure how quickly you can solve problems or implement features using this technique.
- Code Comprehension: Test your ability to explain your code to others or return to it after a period of time.
- Team Feedback: Gather input from colleagues on the clarity and effectiveness of your code and documentation.
Consider keeping a “performance journal” where you log your experiences, insights, and metrics as you apply method acting techniques to your coding practice.
Integrating Method Acting into Your Coding Workflow
To make method acting for coders a sustainable part of your development process, consider the following steps:
- Warm-up Routines: Start your coding sessions with a brief “character warm-up.” Spend a few minutes visualizing your code and getting into the right mindset.
- Code Rehearsals: Before implementing a solution, “rehearse” it by writing pseudocode or discussing it with a rubber duck (or willing colleague).
- Performance Reviews: After completing a feature or project, conduct a “performance review.” Reflect on how well you embodied your code and what you could improve next time.
- Ensemble Practice: Organize regular “ensemble” sessions with your team where you collectively act out complex systems or algorithms.
- Continuous Learning: Just as actors constantly hone their craft, dedicate time to learning new “method coding” techniques and expanding your emotional and technical range.
Conclusion: The Standing Ovation
Method acting for coders is more than just a quirky analogy – it’s a powerful tool for deepening your understanding of code, improving problem-solving skills, and fostering creativity in programming. By fully immersing yourself in your code, treating functions as characters, and approaching coding challenges as scenes to be performed, you can elevate your programming from a mere technical exercise to an art form.
Remember, the goal is not to become a different person when you code, but to bring your full self – emotions, experiences, and creativity – to the programming process. As you practice these techniques, you’ll likely find that your code becomes more intuitive, your solutions more elegant, and your debugging more insightful.
So, the next time you sit down to code, take a deep breath, get into character, and prepare to give the performance of a lifetime. Your code is your stage, your functions are your cast, and with practice, you’ll be well on your way to a standing ovation from users, colleagues, and perhaps most importantly, yourself.
Break a leg, code stars!