Why Problem-Solving in Teams is Different from Solo Coding
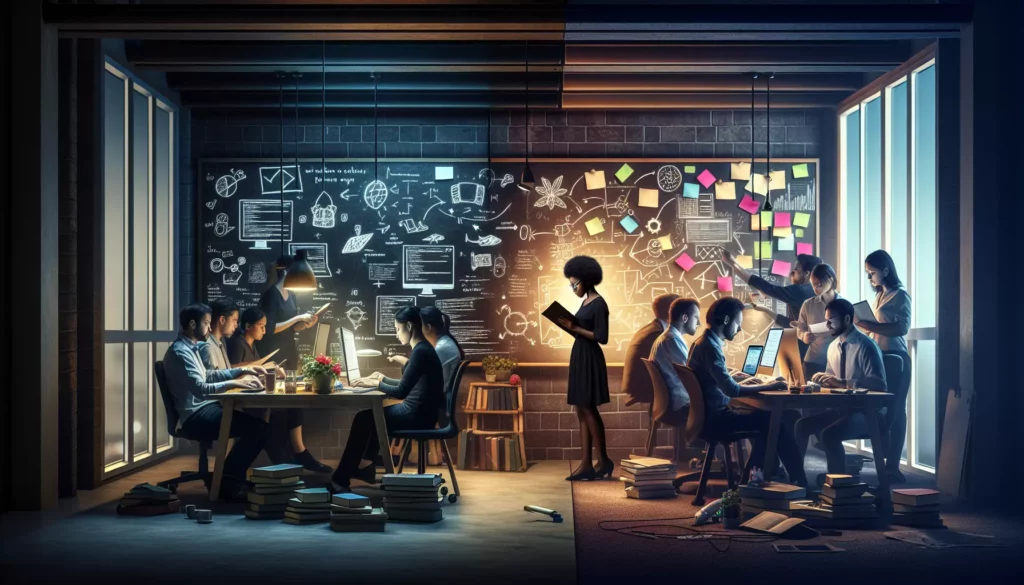
In the world of software development, problem-solving is a fundamental skill that every programmer must master. Whether you’re a beginner just starting your coding journey or an experienced developer preparing for technical interviews at major tech companies, the ability to solve complex problems is crucial. However, there’s a significant difference between solving problems on your own and tackling challenges as part of a team. In this article, we’ll explore why problem-solving in teams is different from solo coding and how you can excel in collaborative environments.
The Nature of Solo Coding
Before we dive into team problem-solving, let’s first understand the characteristics of solo coding:
1. Individual Pace and Style
When coding alone, you have the freedom to work at your own pace and follow your preferred problem-solving approach. You can take as much time as you need to think through a problem, experiment with different solutions, and implement your ideas without external pressure.
2. Complete Control
Solo coders have full control over their project. They make all the decisions, from choosing the programming language and framework to determining the overall architecture and coding style.
3. Limited Perspective
While working alone allows for focused concentration, it also means you’re limited to your own knowledge and experience. You might miss alternative approaches or fail to see potential pitfalls that others could easily spot.
4. Self-Reliance
When you encounter a problem during solo coding, you’re responsible for finding the solution. This often involves extensive research, trial and error, and sometimes struggling through challenging concepts on your own.
The Dynamics of Team Problem-Solving
Now, let’s examine how problem-solving changes when you’re working as part of a team:
1. Diverse Perspectives and Skill Sets
One of the most significant advantages of team problem-solving is the diversity of perspectives and skills that each member brings to the table. In a team, you have access to a collective pool of knowledge and experience, which can lead to more innovative and comprehensive solutions.
For example, consider a team working on optimizing a complex algorithm:
def optimize_algorithm(data):
# Team member A suggests a divide-and-conquer approach
result = divide_and_conquer(data)
# Team member B proposes using dynamic programming
result = apply_dynamic_programming(result)
# Team member C implements parallel processing
result = parallelize_computation(result)
return result
In this scenario, each team member contributes a unique optimization technique, resulting in a more efficient algorithm than any individual might have devised alone.
2. Collaborative Decision-Making
In a team setting, decisions are made collaboratively. This process often involves discussions, debates, and compromises. While it may take longer to reach a consensus, the resulting decisions are usually more well-rounded and consider multiple viewpoints.
3. Code Reviews and Quality Assurance
Team environments typically involve code reviews, where other developers examine and provide feedback on your code. This process helps catch bugs, improve code quality, and ensure adherence to best practices and coding standards.
Here’s an example of how a code review might lead to improvements:
// Original code
function calculateTotal(items) {
let total = 0;
for (let i = 0; i < items.length; i++) {
total += items[i].price;
}
return total;
}
// After code review
function calculateTotal(items) {
return items.reduce((total, item) => total + item.price, 0);
}
In this case, a team member suggested using the reduce method for a more concise and functional approach.
4. Specialization and Division of Labor
Teams often divide tasks based on individual strengths and expertise. This specialization allows for more efficient problem-solving, as each team member can focus on the areas where they excel.
5. Communication Challenges
While team collaboration brings many benefits, it also introduces communication challenges. Misunderstandings can occur, and conveying complex ideas or technical concepts to team members with different backgrounds can be difficult.
Key Differences Between Solo and Team Problem-Solving
Now that we’ve explored the characteristics of both solo and team problem-solving, let’s highlight the key differences:
1. Speed vs. Thoroughness
Solo coding often allows for quicker initial progress, as there’s no need to coordinate with others or wait for approvals. However, team problem-solving, while potentially slower at the outset, often results in more thorough and well-vetted solutions.
2. Flexibility vs. Consistency
Individual coders have the flexibility to change direction quickly or try unconventional approaches. Teams, on the other hand, need to maintain consistency in coding style, naming conventions, and overall architecture, which can sometimes limit creativity but ensures a more maintainable codebase.
3. Learning Curve
In solo projects, you learn primarily through self-study and trial and error. Team environments offer opportunities for peer learning, mentorship, and exposure to diverse coding practices and problem-solving techniques.
4. Accountability
When coding alone, you’re accountable only to yourself. In a team, you’re responsible for meeting deadlines, maintaining code quality, and ensuring your work integrates smoothly with that of your teammates.
5. Scope and Complexity
Team projects often tackle larger, more complex problems that would be difficult or impossible for a single developer to handle. This allows for the creation of more ambitious and feature-rich applications.
Strategies for Effective Team Problem-Solving
To excel in collaborative problem-solving environments, consider the following strategies:
1. Improve Communication Skills
Clear and effective communication is crucial in team settings. Practice articulating your ideas, asking questions, and providing constructive feedback. Use tools like diagrams, pseudocode, or simple examples to convey complex concepts.
2. Embrace Version Control
Familiarize yourself with version control systems like Git. These tools are essential for managing code contributions from multiple team members and tracking changes over time.
Here’s a basic Git workflow that teams often use:
// Create a new branch for your feature
git checkout -b feature/new-algorithm
// Make changes and commit them
git add .
git commit -m "Implement new sorting algorithm"
// Push your changes to the remote repository
git push origin feature/new-algorithm
// Create a pull request for team review
// After approval, merge into the main branch
git checkout main
git merge feature/new-algorithm
3. Practice Active Listening
When team members are sharing ideas or explaining concepts, practice active listening. This means fully concentrating on what is being said, asking clarifying questions, and providing thoughtful responses.
4. Be Open to Feedback
In team environments, your code will be reviewed by others. Learn to accept constructive criticism gracefully and use it as an opportunity for growth and improvement.
5. Develop Collaborative Problem-Solving Techniques
Familiarize yourself with collaborative problem-solving methods like pair programming, brainstorming sessions, and code walkthroughs. These techniques can help teams tackle complex problems more effectively.
6. Stay Organized
Use project management tools and maintain clear documentation to keep track of tasks, deadlines, and team decisions. This helps ensure everyone is on the same page and working towards common goals.
7. Cultivate a Growth Mindset
Approach team problem-solving as an opportunity to learn from others and expand your skills. Be open to new ideas and willing to adapt your approach based on team input.
The Impact of Team Problem-Solving on Career Development
While solo coding skills are important, the ability to work effectively in a team is crucial for career advancement in the tech industry. Here’s how team problem-solving can impact your career:
1. Preparation for Professional Environments
Most software development jobs involve working in teams. Experience with collaborative problem-solving prepares you for real-world work environments and makes you a more attractive candidate to potential employers.
2. Leadership Opportunities
As you become proficient in team problem-solving, you may have opportunities to take on leadership roles, such as team lead or project manager. These positions often require strong collaborative and communication skills.
3. Broader Skill Set
Working in teams exposes you to different coding styles, problem-solving approaches, and technologies. This broader skill set makes you more versatile and valuable as a developer.
4. Networking
Collaborative environments provide opportunities to build professional relationships and expand your network within the tech community.
Balancing Solo and Team Problem-Solving Skills
While team problem-solving is crucial in professional settings, it’s important to maintain a balance between collaborative and individual skills. Here’s how you can do that:
1. Personal Projects
Continue working on personal coding projects to keep your individual problem-solving skills sharp and explore areas of personal interest.
2. Online Coding Challenges
Participate in online coding challenges or competitive programming contests to practice solving problems under time constraints and compare your solutions with others.
3. Contribute to Open Source
Contributing to open-source projects allows you to experience both solo and collaborative coding. You’ll work on individual contributions while also interacting with the project’s community.
4. Mentoring and Teaching
Offering to mentor junior developers or teach coding skills can help reinforce your individual knowledge while also improving your ability to communicate technical concepts effectively.
Conclusion
Problem-solving in teams is fundamentally different from solo coding, offering both unique challenges and significant benefits. While individual coding skills remain important, the ability to collaborate effectively, communicate clearly, and contribute to team problem-solving efforts is crucial for success in the professional world of software development.
As you continue your coding journey, whether you’re using platforms like AlgoCademy to improve your algorithmic thinking or preparing for technical interviews at major tech companies, remember to cultivate both your individual and collaborative problem-solving skills. Embrace opportunities to work in team settings, learn from your peers, and contribute your unique perspective to collective efforts.
By mastering both solo and team problem-solving, you’ll become a more well-rounded, adaptable, and valuable developer, ready to tackle any challenge that comes your way in the ever-evolving world of technology.