The Coder’s Bestiary: Personifying Bugs and Errors for Easier Debugging
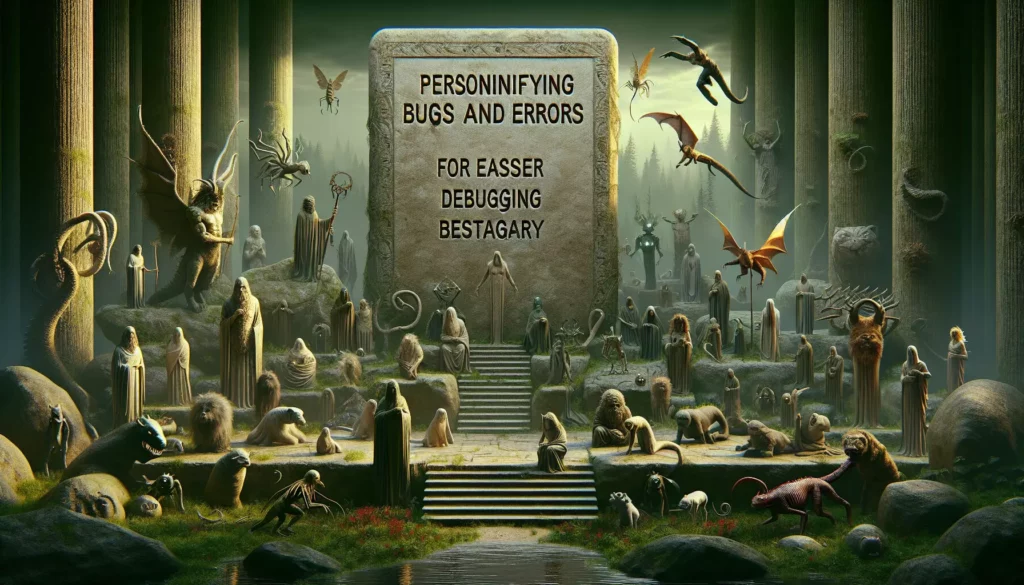
In the vast digital wilderness of code, lurk creatures both fascinating and frustrating. These elusive beasts, known to programmers as bugs and errors, can turn a smooth coding journey into a treacherous expedition. But fear not, intrepid coder! By learning to identify and understand these digital fauna, you can transform the debugging process from a dreaded chore into an exciting safari.
Welcome to “The Coder’s Bestiary,” where we’ll explore the diverse ecosystem of programming pitfalls by personifying common bugs and errors. This unique approach will not only make debugging more engaging but also help you remember and recognize these issues more easily in your future coding adventures.
1. The Syntax Sloth: Slow and Sloppy
Our journey begins with the notoriously lazy Syntax Sloth. This creature is known for its slow movement and tendency to hang around in the most unexpected places of your code.
Habitat:
The Syntax Sloth can be found lounging in nearly any part of your codebase, but it has a particular fondness for dangling from misplaced brackets, semicolons, and quotation marks.
Behavior:
True to its name, the Syntax Sloth moves at a glacial pace, often causing your entire program to grind to a halt. It’s not uncommon to find this creature sleeping on the job, completely unaware of the chaos it’s causing.
How to spot it:
Look for tell-tale signs like red squiggly lines in your IDE or compiler errors that point to specific lines. The Syntax Sloth often leaves behind a trail of cryptic messages like “unexpected token” or “missing semicolon.”
How to tame it:
The key to dealing with a Syntax Sloth is patience and attention to detail. Carefully review your code, line by line, and make sure all your punctuation is in order. Most modern IDEs come equipped with “Sloth detectors” (also known as linters) that can help you spot these creatures before they cause too much trouble.
Example:
function greetUser(name) {
console.log("Hello, " + name) // Oops! Missing semicolon
return "Greeting complete"
}
In this case, the Syntax Sloth is hanging out at the end of the console.log
line, where a semicolon should be. While JavaScript’s automatic semicolon insertion might let this slide, it’s best to evict the Sloth and add the semicolon for clarity and consistency.
2. The Null Pointer Ninja: Silent and Deadly
Stealthy and unpredictable, the Null Pointer Ninja is a formidable foe that can bring down even the most robust programs with its silent strikes.
Habitat:
This elusive creature prefers to hide in the shadows of uninitialized variables and objects. It’s particularly fond of lurking in functions that return values without proper null checks.
Behavior:
The Null Pointer Ninja strikes without warning, often causing your program to crash spectacularly. It’s known for its ability to slip past even the most vigilant programmers, lying in wait until the most inopportune moment to attack.
How to spot it:
Watch for error messages like “NullPointerException” or “Cannot read property ‘x’ of null”. These are clear signs that a Null Pointer Ninja has infiltrated your code.
How to tame it:
The best defense against Null Pointer Ninjas is a good offense. Implement robust null checks throughout your code, especially when dealing with function returns or accessing object properties. Some languages also offer null-safe operators or optional chaining to help keep these ninjas at bay.
Example:
function getUserData(userId) {
// Assume this function might return null if user not found
return database.findUser(userId);
}
function displayUserName(userId) {
let user = getUserData(userId);
console.log(user.name); // Danger! Null Pointer Ninja attack!
}
// A safer approach:
function displayUserName(userId) {
let user = getUserData(userId);
if (user && user.name) {
console.log(user.name);
} else {
console.log("User not found");
}
}
In the first version of displayUserName
, we’re vulnerable to a Null Pointer Ninja attack if getUserData
returns null. The second version includes proper checks to prevent this sneaky creature from causing havoc.
3. The Infinite Loop Leviathan: The Endless Devourer
Behold the Infinite Loop Leviathan, a monstrous creature with an insatiable appetite for computational resources. This behemoth can swallow your program whole, trapping it in an endless cycle of repetition.
Habitat:
The Infinite Loop Leviathan thrives in poorly constructed loops, particularly those lacking proper exit conditions. It’s often found in recursive functions without base cases or while loops with faulty termination logic.
Behavior:
Once unleashed, the Infinite Loop Leviathan will consume all available resources, causing your program to hang indefinitely. It’s known for its ability to make time stand still, at least from your computer’s perspective.
How to spot it:
Signs of an Infinite Loop Leviathan include unresponsive programs, rapidly increasing memory usage, and CPUs running at 100% for extended periods. In some cases, you might see your program spitting out the same output endlessly.
How to tame it:
To subdue an Infinite Loop Leviathan, ensure all your loops have clear and achievable exit conditions. For recursive functions, always include a base case. It’s also wise to implement timeout mechanisms for potentially long-running operations.
Example:
function countDown(n) {
console.log(n);
if (n > 0) {
countDown(n - 1);
}
}
countDown(5); // This is fine
function countUp(n) {
console.log(n);
countUp(n + 1); // Uh oh, Infinite Loop Leviathan spotted!
}
countUp(1); // This will run forever
In the countDown
function, we have a proper base case that prevents the Infinite Loop Leviathan from appearing. However, countUp
lacks this safeguard, allowing the creature to run rampant.
4. The Race Condition Rabbit: Fast and Unpredictable
Quick and elusive, the Race Condition Rabbit is a tricky creature that thrives in the world of concurrent and parallel programming. Its unpredictable nature can lead to baffling bugs that seem to appear and disappear at random.
Habitat:
Race Condition Rabbits are commonly found in multi-threaded environments, asynchronous operations, and any situation where the sequence of events can vary between program executions.
Behavior:
These speedy creatures dart in and out of your code, causing seemingly random failures or inconsistent results. What works perfectly in one run might crash spectacularly in the next, all thanks to the capricious nature of the Race Condition Rabbit.
How to spot it:
Look for bugs that only appear intermittently, especially in multi-threaded or asynchronous code. If your program works 99% of the time but occasionally produces unexpected results or crashes, you might be dealing with a Race Condition Rabbit.
How to tame it:
Catching a Race Condition Rabbit requires patience and the right tools. Use synchronization mechanisms like locks, semaphores, or atomic operations to control access to shared resources. In asynchronous programming, properly manage your promises or callbacks to ensure operations occur in the intended order.
Example:
let sharedCounter = 0;
function incrementCounter() {
// Race Condition Rabbit alert!
let temp = sharedCounter;
temp = temp + 1;
sharedCounter = temp;
}
// In a multi-threaded environment, calling this function
// from multiple threads can lead to unexpected results
// A safer approach using atomic operations:
let safeCounter = new Atomic(0);
function safeIncrement() {
safeCounter.incrementAndGet();
}
In the first example, if multiple threads call incrementCounter
simultaneously, they might read the same initial value, leading to lost updates. The second example uses atomic operations to ensure that the increment happens as an indivisible unit, preventing the Race Condition Rabbit from interfering.
5. The Memory Leak Moth: The Silent Resource Devourer
Fluttering quietly in the background, the Memory Leak Moth slowly but surely consumes your program’s resources. This insidious creature can go unnoticed for long periods, gradually degrading your application’s performance.
Habitat:
Memory Leak Moths are often found in long-running applications, especially those that create and destroy objects frequently. They’re particularly fond of environments with manual memory management, but can also appear in garbage-collected languages.
Behavior:
The Memory Leak Moth feeds on unreleased resources, slowly growing larger over time. As it consumes more memory, your application becomes sluggish and may eventually crash when available memory is exhausted.
How to spot it:
Watch for gradually increasing memory usage over time, especially in long-running applications. Tools like task manager, activity monitor, or specialized memory profilers can help you track the Memory Leak Moth’s trail.
How to tame it:
To combat Memory Leak Moths, ensure you’re properly releasing resources when they’re no longer needed. In manual memory management languages, this means carefully balancing allocations and deallocations. In garbage-collected languages, be mindful of holding references to objects you no longer need.
Example:
class ResourceHog {
constructor() {
this.data = new Array(1000000).fill(0);
}
}
function createAndForget() {
let hog = new ResourceHog();
// Oops! We created a hog but never let it go
// Memory Leak Moth is happy here
}
setInterval(createAndForget, 1000); // This will slowly eat up memory
// A better approach:
function createAndRelease() {
let hog = new ResourceHog();
// Do something with hog
hog = null; // Explicitly remove the reference
}
In the first example, we’re repeatedly creating ResourceHog
objects without ever releasing them, providing a feast for Memory Leak Moths. The second example explicitly removes the reference, allowing the garbage collector to reclaim the memory.
6. The Off-By-One Owl: Wise But Slightly Misaligned
Perched on the edges of your arrays and loops, the Off-By-One Owl appears wise but often leads programmers astray with its slightly skewed perspective.
Habitat:
Off-By-One Owls are commonly found in array operations, loop boundaries, and any situation involving indexing or counting. They have a particular fondness for zero-indexed collections.
Behavior:
These creatures cause subtle errors by consistently being just one step ahead or behind where they should be. They’re known for causing array index out of bounds errors or loops that process one too many or too few items.
How to spot it:
Look for unexpected behavior at the boundaries of your operations. If your code works fine for most cases but fails for the first or last element of a collection, you might be dealing with an Off-By-One Owl.
How to tame it:
To outsmart the Off-By-One Owl, always double-check your loop conditions and array indices. Be especially careful when dealing with inclusive vs. exclusive ranges, and remember that most programming languages use zero-based indexing for arrays.
Example:
function printArrayElements(arr) {
for (let i = 1; i <= arr.length; i++) {
console.log(arr[i]); // Off-By-One Owl alert!
}
}
// This will miss the first element and try to access one past the end
// A correct version:
function printArrayElementsCorrectly(arr) {
for (let i = 0; i < arr.length; i++) {
console.log(arr[i]);
}
}
In the first example, the Off-By-One Owl has caused us to start at index 1 (missing the first element) and continue until we’re one past the end of the array. The corrected version properly handles zero-based indexing and the array’s length.
7. The Floating Point Ferret: Precision’s Playful Predator
Darting through the realm of decimal numbers, the Floating Point Ferret is a mischievous creature that delights in confounding programmers with its imprecise nature.
Habitat:
Floating Point Ferrets are found wherever decimal numbers are used, especially in financial calculations, scientific computing, and any situation requiring high precision.
Behavior:
These playful creatures introduce small inaccuracies into calculations, leading to results that are close to, but not quite, what you expect. They’re notorious for making seemingly simple comparisons fail in mysterious ways.
How to spot it:
Watch for unexpected results in decimal calculations, especially when comparing floating-point numbers for equality. If 0.1 + 0.2 doesn’t quite equal 0.3 in your program, you’ve likely encountered a Floating Point Ferret.
How to tame it:
To handle Floating Point Ferrets, avoid direct equality comparisons for floating-point numbers. Instead, check if the difference between two numbers is within an acceptable margin of error. For financial calculations, consider using decimal arithmetic libraries that provide exact decimal representations.
Example:
let a = 0.1;
let b = 0.2;
let c = 0.3;
if (a + b === c) {
console.log("Math works!");
} else {
console.log("Floating Point Ferret strikes again!");
}
// This will likely print "Floating Point Ferret strikes again!"
// A better approach:
function areFloatsEqual(a, b, epsilon = 0.0001) {
return Math.abs(a - b) < epsilon;
}
if (areFloatsEqual(a + b, c)) {
console.log("Close enough for floating point work!");
}
In the first example, the Floating Point Ferret causes the equality check to fail due to the imprecise nature of floating-point representation. The second approach uses a tolerance value (epsilon) to check if the numbers are close enough to be considered equal.
8. The Scope Spectre: The Invisible Variable Thief
Lurking in the shadows between functions and blocks, the Scope Spectre is a ghostly entity that confounds programmers by making variables disappear or behave unexpectedly.
Habitat:
Scope Spectres haunt the boundaries between different scopes in your code. They’re particularly active in languages with complex scoping rules or in codebases that make heavy use of closures and nested functions.
Behavior:
These ethereal beings can make variables seem to vanish into thin air or take on unexpected values. They’re known for causing confusion when variables with the same name exist in different scopes.
How to spot it:
If you find yourself scratching your head over variables that don’t seem to have the values you expect, or if you’re getting “undefined” errors for variables you’re sure you defined, you might be dealing with a Scope Spectre.
How to tame it:
To exorcise Scope Spectres, develop a clear understanding of your language’s scoping rules. Be careful with variable naming to avoid shadowing, and consider using block-scoped declarations (like let
and const
in JavaScript) instead of function-scoped ones.
Example:
let x = 10;
function outer() {
console.log(x); // Scope Spectre alert!
let x = 20;
console.log(x);
}
outer();
// This will log: undefined, 20
// A clearer approach:
let globalX = 10;
function outerClear() {
console.log(globalX); // 10
let localX = 20;
console.log(localX); // 20
}
outerClear();
In the first example, the Scope Spectre causes the first console.log
to print undefined
due to the temporal dead zone created by the later declaration of x
. The second approach avoids this by using distinct names for global and local variables.
9. The Type Coercion Chameleon: The Shape-Shifting Trickster
Master of disguise and transformation, the Type Coercion Chameleon is a creature that can change the very nature of your data when you least expect it.
Habitat:
Type Coercion Chameleons are most commonly found in dynamically-typed languages, particularly those with implicit type conversion. They’re especially active in JavaScript, where type coercion is a fundamental part of the language.
Behavior:
These shape-shifting creatures can transform data from one type to another in the blink of an eye. They’re known for turning strings into numbers, booleans into strings, and generally causing confusion when different types interact.
How to spot it:
Watch for unexpected results when comparing or operating on values of different types. If you find yourself wondering why “2” + 2 equals “22” instead of 4, you’ve likely encountered a Type Coercion Chameleon.
How to tame it:
To handle Type Coercion Chameleons, always be explicit about your type conversions. Use strict equality operators (=== in JavaScript) instead of loose equality (==). When performing operations, consider explicitly converting your data to the desired type first.
Example:
// Type Coercion Chameleon at work:
console.log(1 == "1"); // true
console.log(1 + "1"); // "11"
console.log(true + true); // 2
// A more predictable approach:
console.log(1 === Number("1")); // true
console.log(1 + parseInt("1")); // 2
console.log(Number(true) + Number(true)); // 2
In the first set of examples, the Type Coercion Chameleon causes some surprising results through implicit type conversion. The second set shows how to achieve more predictable results by being explicit about type conversions.
10. The Dependency Dragon: The Library-Hoarding Beast
Towering over your project, the Dependency Dragon is a massive creature that grows larger with every library and framework you add to your codebase.
Habitat:
Dependency Dragons are found in the depths of your project’s node_modules folder or wherever your project’s dependencies are stored. They’re particularly common in modern web development ecosystems.
Behavior:
These creatures grow by consuming external libraries and frameworks. As they get larger, they can slow down your build processes, increase your deployment size, and introduce potential security vulnerabilities.
How to spot it:
Signs of a Dependency Dragon include bloated project sizes, long build times, and a package.json file that scrolls for miles. If you find yourself installing a new package for every small task, you might be feeding a Dependency Dragon.
How to tame it:
To keep your Dependency Dragon in check, regularly audit your dependencies. Remove unused packages, and consider if you really need a full library for simple tasks. Use tools like npm-check or Depcheck to identify unused dependencies.
Example:
// package.json feeding a Dependency Dragon
{
"dependencies": {
"lodash": "^4.17.21",
"moment": "^2.29.1",
"axios": "^0.21.1",
"react": "^17.0.2",
"react-dom": "^17.0.2",
"redux": "^4.1.0",
"react-redux": "^7.2.4",
"styled-components": "^5.3.0",
// ... many more dependencies
}
}
// A leaner approach
{
"dependencies": {
"react": "^17.0.2",
"react-dom": "^17.0.2"
},
"devDependencies": {
"date-fns": "^2.22.1" // Lighter alternative to moment
}
}
The first example shows a package.json file that’s feeding a large Dependency Dragon. The second example demonstrates a more streamlined approach, using only necessary dependencies and opting for lighter alternatives where possible.
Conclusion: Mastering the Art of Bug Hunting
As we conclude our journey through the Coder’s Bestiary, remember that these creatures, while formidable, are not unbeatable. Each bug and error, from the lazy Syntax Sloth to the massive Dependency Dragon, can be tamed with the right knowledge and tools.
By personifying these common programming pitfalls, we hope to have made the debugging process more engaging and memorable. The next time you encounter one of these digital beasts in your code, you’ll be better equipped to identify and handle it.
Remember, every experienced programmer has faced these creatures countless times. What sets apart the coding masters is not an ability to avoid bugs entirely, but the skill to efficiently identify, understand, and resolve them when they appear.
So, intrepid coder, arm yourself with this knowledge and venture forth into your next programming adventure. May your debugger be sharp, your patience be long, and your code be ever bug-free!
Happy coding, and happy bug hunting!