Learning to Code Through Origami: Folding Your Way to Better Algorithms
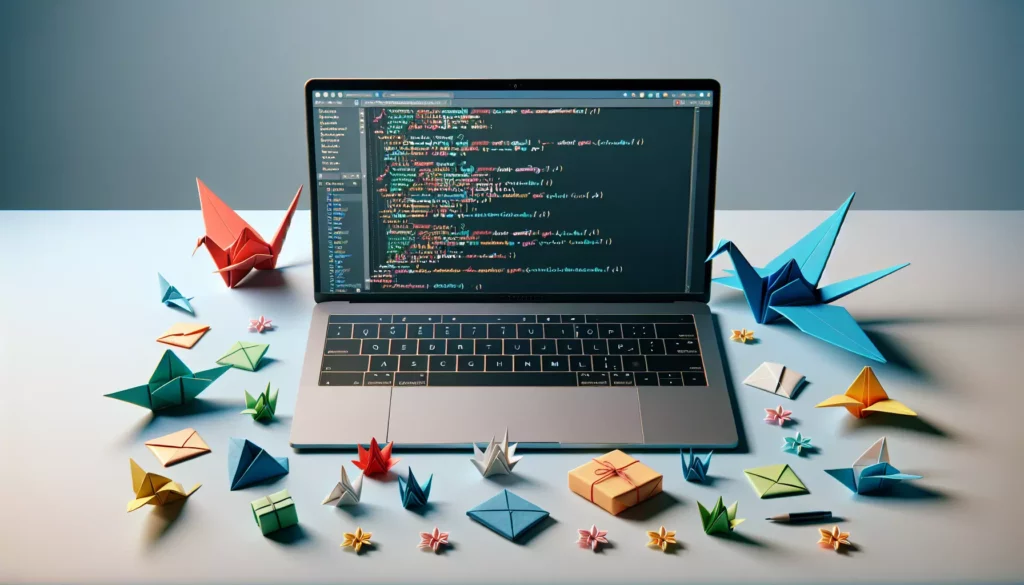
In the ever-evolving world of technology, learning to code has become an essential skill. But what if we told you that the ancient art of paper folding could be your secret weapon in mastering algorithms and improving your programming skills? Welcome to the fascinating intersection of origami and coding, where the delicate folds of paper can unfold the complexities of computer science.
The Unexpected Connection: Origami and Coding
At first glance, origami and coding might seem worlds apart. One involves physical manipulation of paper, while the other deals with abstract lines of code. However, these two disciplines share more common ground than you might think. Both require:
- Precision and attention to detail
- Following step-by-step instructions
- Problem-solving and logical thinking
- Creativity and innovation
- The ability to visualize end results
These shared attributes make origami an excellent tool for developing the mental skills necessary for coding. Let’s dive deeper into how folding paper can help you become a better programmer.
Algorithmic Thinking in Origami
An algorithm is a step-by-step procedure for solving a problem or accomplishing a task. In coding, algorithms are the backbone of every program. Origami, surprisingly, follows a similar pattern. Each origami model has its own algorithm – a series of precise folds that transform a flat sheet into a three-dimensional object.
Consider the process of folding a simple paper airplane:
- Start with a rectangular sheet of paper
- Fold the paper in half lengthwise, then unfold
- Fold the top corners down to the center crease
- Fold the top edges down to meet the bottom edge of the previous folds
- Fold the entire plane in half
- Fold down the wings
This sequence of steps is, in essence, an algorithm. It’s a repeatable process that produces consistent results. By practicing origami, you’re training your mind to think in algorithmic patterns, which is crucial in coding.
Debugging: The Art of Unfolding and Refolding
In programming, debugging is the process of identifying and fixing errors in code. It’s a critical skill that often requires patience and methodical thinking. Origami offers a tangible way to practice these debugging skills.
When you make a mistake in origami, you often need to unfold your work, identify where the error occurred, and then refold from that point. This process mirrors debugging in coding:
- Identifying the problem (a misaligned fold or a bug in the code)
- Tracing back through the steps to find the source of the error
- Correcting the mistake and proceeding from that point
- Testing the result to ensure the problem is resolved
By practicing origami, you’re honing your ability to methodically troubleshoot problems – a skill that directly translates to debugging code.
Modular Programming and Modular Origami
Modular programming is a software design technique that emphasizes separating the functionality of a program into independent, interchangeable modules. This approach makes code more manageable, reusable, and easier to debug. Interestingly, there’s a branch of origami that follows a similar principle: modular origami.
In modular origami, complex structures are created by combining multiple identical units. Each unit is folded separately and then assembled to create the final model. This process closely resembles modular programming:
- Each origami unit is like a function or class in programming
- The way units connect represents the interfaces between modules
- The final assembly is akin to how different modules come together to form a complete program
By practicing modular origami, you can gain insights into the principles of modular programming and how to break down complex problems into manageable, reusable components.
Recursion: The Fractal Nature of Origami
Recursion is a fundamental concept in computer science where a function calls itself to solve a problem. It’s a powerful technique for solving complex problems by breaking them down into simpler, self-similar sub-problems. Origami, particularly in its more advanced forms, often incorporates recursive patterns.
Consider the Koch snowflake, a fractal that can be created through origami:
- Start with an equilateral triangle
- Fold each side into thirds
- Fold the middle third outward to create a smaller equilateral triangle
- Repeat steps 2-3 for each new side created
This process can theoretically continue infinitely, creating increasingly complex patterns. It’s a perfect example of recursion in action. By folding fractal origami models, you’re physically engaging with the concept of recursion, making it easier to understand and apply in your coding.
Spatial Reasoning and Data Structures
Origami requires strong spatial reasoning skills. You need to visualize how a flat sheet of paper will transform into a three-dimensional object through a series of folds. This ability to mentally manipulate objects and understand their spatial relationships is incredibly valuable in programming, especially when working with complex data structures.
Consider these parallels:
- Folding a flat sheet into a box is similar to creating a 3D array in programming
- Creating multiple interconnected units in modular origami is akin to working with linked lists or trees
- The way an origami model unfolds and refolds can be compared to stack and queue operations
By improving your spatial reasoning through origami, you’re indirectly enhancing your ability to work with and visualize complex data structures in your code.
Optimization: Minimizing Folds, Maximizing Efficiency
In both origami and coding, there’s often more than one way to achieve the desired result. However, finding the most efficient solution is key. In origami, this might mean creating a model with the fewest possible folds. In coding, it’s about writing algorithms that use the least amount of computational resources.
The pursuit of elegance and efficiency in origami can translate directly to coding practices:
- Identifying unnecessary steps and eliminating them
- Finding clever shortcuts that achieve the same result with less effort
- Balancing complexity and functionality
- Striving for clean, readable solutions
By practicing origami with an eye for optimization, you’re training yourself to approach coding problems with the same mindset of efficiency and elegance.
Pattern Recognition and Algorithm Design
Successful origami artists and programmers share a crucial skill: the ability to recognize and utilize patterns. In origami, certain folding sequences appear across multiple models. Recognizing these patterns allows you to tackle new, more complex models more easily.
Similarly, in programming, recognizing common patterns in problems can help you design more effective algorithms. Many coding challenges can be solved by applying or adapting known algorithmic patterns, such as:
- Divide and conquer
- Dynamic programming
- Greedy algorithms
- Backtracking
By honing your pattern recognition skills through origami, you’re indirectly improving your ability to identify and apply algorithmic patterns in your coding projects.
Implementing Origami Principles in Code
Now that we’ve explored the theoretical connections between origami and coding, let’s look at a practical example of how origami principles can be implemented in code. We’ll create a simple Python program that simulates the folding of a paper airplane.
class PaperAirplane:
def __init__(self):
self.state = "flat sheet"
self.folds = 0
def fold_in_half(self):
if self.state == "flat sheet":
print("Folding paper in half lengthwise")
self.state = "folded in half"
self.folds += 1
else:
print("Cannot fold in half at this stage")
def fold_corners(self):
if self.state == "folded in half":
print("Folding top corners to center crease")
self.state = "corners folded"
self.folds += 1
else:
print("Cannot fold corners at this stage")
def fold_edges(self):
if self.state == "corners folded":
print("Folding top edges to meet bottom edge")
self.state = "edges folded"
self.folds += 1
else:
print("Cannot fold edges at this stage")
def fold_in_half_again(self):
if self.state == "edges folded":
print("Folding entire plane in half")
self.state = "folded in half again"
self.folds += 1
else:
print("Cannot fold in half at this stage")
def fold_wings(self):
if self.state == "folded in half again":
print("Folding down the wings")
self.state = "complete"
self.folds += 1
else:
print("Cannot fold wings at this stage")
def status(self):
print(f"Current state: {self.state}")
print(f"Number of folds: {self.folds}")
# Let's create and fold our paper airplane
airplane = PaperAirplane()
airplane.fold_in_half()
airplane.fold_corners()
airplane.fold_edges()
airplane.fold_in_half_again()
airplane.fold_wings()
airplane.status()
This code demonstrates several key concepts that bridge origami and programming:
- State Management: The
state
variable keeps track of the current folding stage, much like how we need to keep track of the current state of our origami model. - Sequential Steps: Each method represents a specific fold, and they must be executed in the correct order, mirroring the step-by-step nature of origami instructions.
- Error Handling: The code checks the current state before performing each fold, preventing incorrect sequences – similar to how we might catch ourselves before making an incorrect fold in origami.
- Modularity: Each folding step is encapsulated in its own method, allowing for easy reuse and modification – akin to the modular nature of origami units.
Origami Coding Challenges
To further reinforce the connection between origami and coding, here are a few coding challenges inspired by origami concepts:
- Recursive Snowflake: Implement a function that generates the Koch snowflake fractal to a specified depth of recursion.
- Origami Simulator: Expand on the paper airplane example to create a more general origami simulator that can handle different models and folding sequences.
- Crease Pattern Analyzer: Develop an algorithm that can analyze an origami crease pattern and determine if it’s flat-foldable.
- Modular Origami Generator: Create a program that generates instructions for modular origami structures based on user input (number of units, connection points, etc.).
These challenges encourage you to think about geometry, recursion, state management, and algorithmic thinking – all crucial skills in both origami and coding.
Conclusion: Unfolding Your Coding Potential
As we’ve explored throughout this article, the connection between origami and coding runs deep. The skills you develop through paper folding – precision, patience, spatial reasoning, and algorithmic thinking – directly translate to the world of programming. By incorporating origami into your learning routine, you’re not just creating beautiful paper models; you’re sculpting your mind to think like a programmer.
Remember, the journey to becoming a proficient coder is much like creating a complex origami model. It starts with simple folds (basic programming concepts) and gradually builds up to more intricate designs (advanced algorithms and data structures). Each fold, each line of code, brings you closer to your goal.
So, the next time you’re struggling with a coding problem, why not take a break and fold some paper? You might just find that the solution unfolds before your eyes, crisp and clean as a perfectly executed origami model.
Happy folding, and happy coding!