The Bartender’s Guide to Data Structures: Mixing the Perfect Algorithm Cocktail
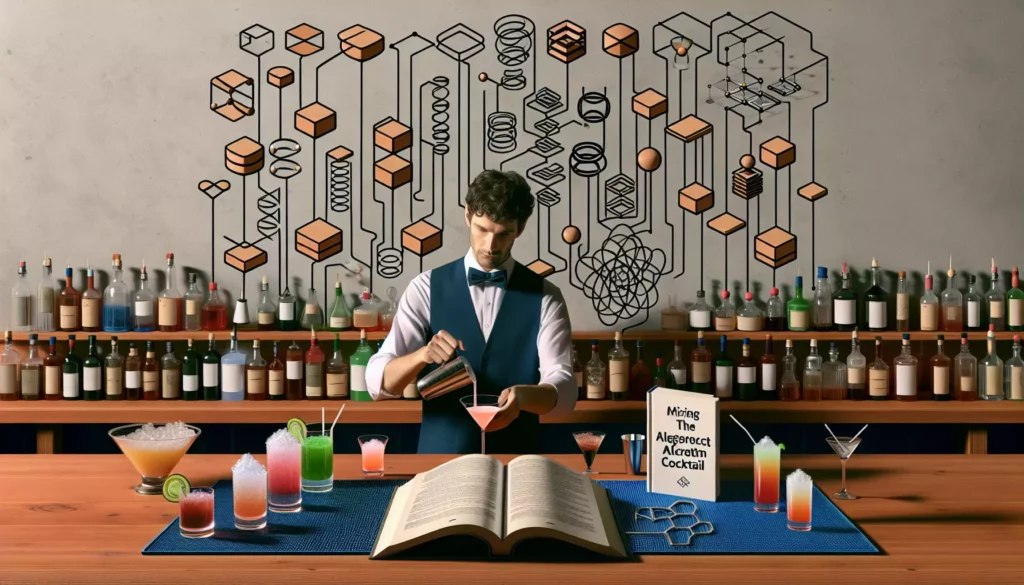
Welcome to the AlgoCademy Bar, where we serve up the finest algorithm cocktails in town! Today, we’re going to explore the world of data structures through the lens of a skilled bartender. Just as a mixologist combines various ingredients to create the perfect drink, a programmer uses different data structures to craft efficient and elegant algorithms. So, grab a seat at the bar, and let’s dive into the art of mixing code!
The Basics: Understanding Your Ingredients
Before we start mixing our algorithm cocktails, let’s familiarize ourselves with the basic ingredients – the fundamental data structures that form the backbone of computer science.
1. Arrays: The Classic Shooter
Arrays are like a row of shot glasses lined up on the bar. They’re simple, straightforward, and perfect for quick access. Each element in an array is stored in a contiguous memory location, making it easy to retrieve any item if you know its position.
// Declaring and initializing an array in Java
int[] shots = {1, 2, 3, 4, 5};
// Accessing an element
int thirdShot = shots[2]; // Remember, indexing starts at 0!
Arrays are great for situations where you know the size of your data in advance and need quick access to elements by their index. However, they’re not so flexible when it comes to adding or removing elements, especially in the middle of the array.
2. Linked Lists: The Daisy Chain
Imagine a string of paper umbrellas, each linked to the next – that’s your linked list. Each element (or node) in a linked list contains data and a reference to the next node.
class Node {
int data;
Node next;
Node(int d) {
data = d;
next = null;
}
}
Linked lists are excellent for situations where you need to frequently add or remove elements, especially at the beginning or end of the list. They’re not as good for random access, though – you’ll need to traverse the list from the beginning to find a specific element.
3. Stacks: The Tower of Champagne Glasses
Picture a tower of champagne glasses at a wedding. You can only add or remove glasses from the top – that’s exactly how a stack works. It follows the Last-In-First-Out (LIFO) principle.
import java.util.Stack;
Stack<String> champagneTower = new Stack<>();
champagneTower.push("Top Glass");
champagneTower.push("Middle Glass");
champagneTower.push("Bottom Glass");
String lastAdded = champagneTower.pop(); // Returns "Bottom Glass"
Stacks are perfect for scenarios like undo operations, parsing expressions, or managing function calls in programming languages.
4. Queues: The Bar Line
A queue is like the line of patrons waiting to order at a busy bar. The first person to arrive is the first to be served – this is the First-In-First-Out (FIFO) principle.
import java.util.LinkedList;
import java.util.Queue;
Queue<String> barLine = new LinkedList<>();
barLine.add("Alice");
barLine.add("Bob");
barLine.add("Charlie");
String firstInLine = barLine.remove(); // Returns "Alice"
Queues are ideal for managing tasks that need to be processed in a specific order, like print job spooling or handling requests in a web server.
Advanced Mixology: Complex Data Structures
Now that we’ve covered the basics, let’s move on to some more sophisticated structures – the premium spirits of our algorithm bar, if you will.
5. Trees: The Cocktail Menu
Think of a tree data structure as your cocktail menu. It starts with a root (the menu cover), branches out into categories (spirits, wines, beers), and then into individual drinks (the leaves).
class TreeNode {
String data;
List<TreeNode> children;
TreeNode(String data) {
this.data = data;
this.children = new ArrayList<>();
}
}
Trees are excellent for representing hierarchical data. Binary trees, a special type of tree where each node has at most two children, are particularly useful in search algorithms and expression parsing.
6. Graphs: The Social Network
Imagine mapping out all the connections between patrons at your bar – who knows whom, who’s buying drinks for whom. That’s essentially what a graph does. It represents a set of objects (vertices) and their relationships (edges).
class Graph {
private Map<Integer, List<Integer>> adjacencyList;
public Graph() {
adjacencyList = new HashMap<>();
}
public void addEdge(int source, int destination) {
adjacencyList.putIfAbsent(source, new ArrayList<>());
adjacencyList.get(source).add(destination);
}
}
Graphs are incredibly versatile and can be used to model everything from social networks to transportation systems to computer networks.
7. Hash Tables: The Inventory System
A hash table is like the bar’s inventory system. Each bottle has a unique code (the key) that maps to its location in the storeroom (the value). This allows for quick retrieval of items.
import java.util.HashMap;
HashMap<String, Integer> inventory = new HashMap<>();
inventory.put("Vodka", 50);
inventory.put("Gin", 30);
inventory.put("Rum", 40);
int vodkaCount = inventory.get("Vodka"); // Returns 50
Hash tables offer constant-time complexity for insertions and lookups on average, making them excellent for scenarios where quick access to data is crucial.
Mixing It Up: Choosing the Right Data Structure
Now that we’ve explored our ingredients, how do we choose which ones to use in our algorithm cocktails? Here are some factors to consider:
1. Time Complexity: Speed of Service
Different data structures have different time complexities for various operations. For instance:
- Arrays offer O(1) access time but O(n) insertion/deletion time for arbitrary positions.
- Linked lists provide O(1) insertion/deletion at the ends but O(n) access time.
- Hash tables offer O(1) average-case time for insertions, deletions, and lookups.
Choose the structure that optimizes the operations you’ll be performing most frequently.
2. Space Complexity: Bar Capacity
Consider how much memory your chosen data structure will consume. Arrays, for example, require contiguous memory allocation, which might not be feasible for very large datasets. Linked structures like trees and graphs can be more memory-efficient for sparse data.
3. Functionality: Special Orders
What specific operations does your algorithm need to perform? If you need to frequently add or remove elements from both ends of a collection, a deque (double-ended queue) might be your best bet. If you need to maintain a sorted collection with fast insertions and deletions, a balanced binary search tree like a Red-Black tree could be ideal.
4. Implementation Complexity: Bartending Skills
Some data structures are simpler to implement than others. While a basic array or linked list is relatively straightforward, implementing a balanced tree or an efficient hash table requires more skill and care. Consider your own expertise and the maintainability of your code when choosing a data structure.
Crafting Your Signature Cocktails: Algorithm Design Patterns
Now that we’ve covered our ingredients and how to choose them, let’s look at some classic “cocktail recipes” – algorithm design patterns that combine data structures in clever ways.
1. The Two-Pointer Technique: The Perfect Blend
This technique involves using two pointers to traverse a data structure, often moving at different speeds or in different directions. It’s particularly useful for array and linked list problems.
public boolean isPalindrome(String s) {
int left = 0;
int right = s.length() - 1;
while (left < right) {
if (s.charAt(left) != s.charAt(right)) {
return false;
}
left++;
right--;
}
return true;
}
This algorithm checks if a string is a palindrome by comparing characters from both ends, moving towards the center.
2. Sliding Window: The Tasting Flight
The sliding window technique is like serving a tasting flight of cocktails. You maintain a “window” of elements and slide it over your data structure, updating the window as you go.
public int maxSumSubarray(int[] nums, int k) {
int maxSum = 0;
int windowSum = 0;
for (int i = 0; i < nums.length; i++) {
windowSum += nums[i];
if (i >= k - 1) {
maxSum = Math.max(maxSum, windowSum);
windowSum -= nums[i - (k - 1)];
}
}
return maxSum;
}
This algorithm finds the maximum sum of a subarray of size k in an array.
3. Divide and Conquer: The Cocktail Party
Divide and conquer algorithms break a problem into smaller subproblems, solve them, and then combine the results. It’s like organizing a large cocktail party by dividing guests into smaller groups.
public int[] mergeSort(int[] arr) {
if (arr.length <= 1) {
return arr;
}
int mid = arr.length / 2;
int[] left = Arrays.copyOfRange(arr, 0, mid);
int[] right = Arrays.copyOfRange(arr, mid, arr.length);
return merge(mergeSort(left), mergeSort(right));
}
private int[] merge(int[] left, int[] right) {
int[] result = new int[left.length + right.length];
int i = 0, j = 0, k = 0;
while (i < left.length && j < right.length) {
if (left[i] <= right[j]) {
result[k++] = left[i++];
} else {
result[k++] = right[j++];
}
}
while (i < left.length) {
result[k++] = left[i++];
}
while (j < right.length) {
result[k++] = right[j++];
}
return result;
}
This is an implementation of the merge sort algorithm, a classic example of divide and conquer.
4. Dynamic Programming: The Mixologist’s Recipe Book
Dynamic programming is like a mixologist’s recipe book, where complex cocktails are broken down into simpler components, and the results are stored for future use. It’s all about avoiding redundant work by storing and reusing intermediate results.
public int fibonacci(int n) {
if (n <= 1) {
return n;
}
int[] fib = new int[n + 1];
fib[0] = 0;
fib[1] = 1;
for (int i = 2; i <= n; i++) {
fib[i] = fib[i - 1] + fib[i - 2];
}
return fib[n];
}
This dynamic programming approach to calculating Fibonacci numbers is much more efficient than the naive recursive approach.
Garnishing Your Cocktails: Optimizing Your Algorithms
Once you’ve mixed your algorithm cocktail, it’s time to add the finishing touches. Here are some tips for optimizing your algorithms:
1. Space-Time Tradeoffs: The Premium Shelf
Sometimes, you can trade memory for speed (or vice versa). For example, caching results of expensive computations can dramatically speed up your algorithm at the cost of using more memory. It’s like stocking premium spirits – they take up more shelf space but allow you to serve high-quality drinks faster.
2. Amortized Analysis: The Happy Hour Strategy
Some data structures, like dynamic arrays, have operations that are occasionally expensive but cheap on average. Understanding amortized analysis can help you make better decisions about when to use these structures. It’s like running a happy hour – you might lose money on some drinks, but you make up for it in volume.
3. Algorithmic Paradigms: Mixology Techniques
Familiarize yourself with various algorithmic paradigms like greedy algorithms, backtracking, and branch and bound. These are like advanced mixology techniques that can be applied to a wide range of problems.
4. Profiling and Benchmarking: Taste Testing
Always measure the performance of your algorithms in real-world scenarios. What looks good on paper might not always perform well in practice. It’s like taste-testing your cocktails before serving them to customers.
The Last Call: Continuous Learning and Practice
As with bartending, mastering the art of algorithm design and data structures takes time, practice, and a willingness to learn. Here are some final tips to help you on your journey:
1. Study the Classics
Familiarize yourself with classic algorithms and data structures. They form the foundation of computer science and appear frequently in coding interviews.
2. Practice, Practice, Practice
Solve coding problems regularly. Platforms like AlgoCademy offer a wide range of problems to test your skills and learn new techniques.
3. Analyze and Reflect
After solving a problem, take time to analyze your solution. Could it be optimized further? Are there alternative approaches? This reflection will deepen your understanding and improve your problem-solving skills.
4. Stay Updated
The field of computer science is constantly evolving. Stay updated with new algorithms, data structures, and best practices. Attend conferences, read research papers, and engage with the coding community.
5. Teach Others
Teaching is one of the best ways to solidify your own understanding. Share your knowledge with others, participate in coding forums, or start a blog to explain complex concepts in simple terms.
Conclusion: Cheers to Algorithmic Thinking!
As we close our AlgoCademy Bar for the night, remember that becoming a master algorithm mixologist is a journey, not a destination. Each problem you solve, each data structure you implement, and each algorithm you optimize adds another recipe to your cocktail book of coding knowledge.
The world of algorithms and data structures is vast and fascinating, offering endless opportunities for creativity and problem-solving. Whether you’re preparing for a coding interview, working on a complex software project, or simply enjoying the intellectual challenge, the skills you develop here will serve you well throughout your programming career.
So, raise a glass to the art of algorithmic thinking! May your code be efficient, your data structures be elegant, and your solutions be as smooth and satisfying as a perfectly mixed cocktail. Cheers, and happy coding!