The Role of Empathy in Software Development: Building Better Code and Stronger Teams
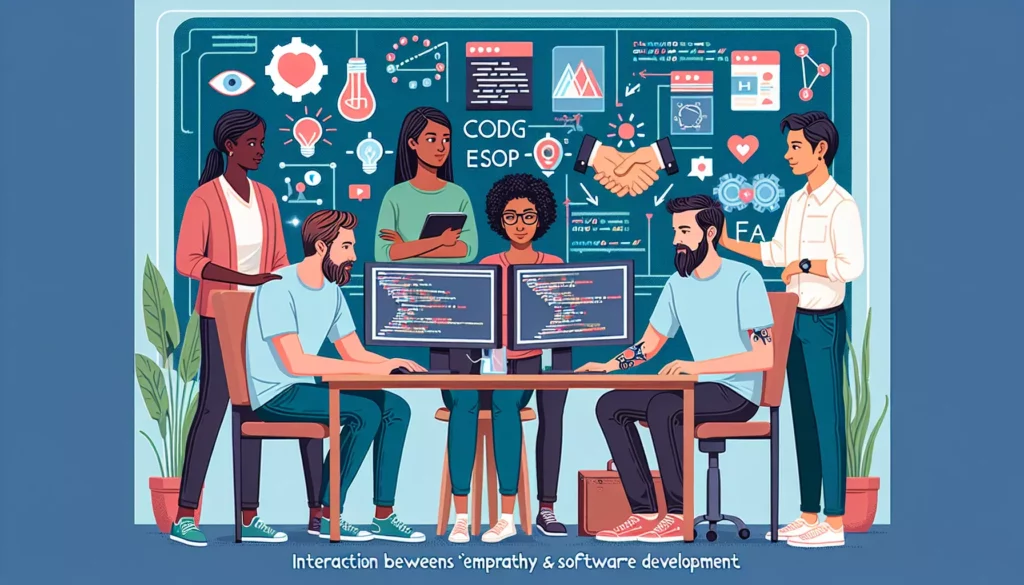
In the world of software development, technical skills often take center stage. We focus on mastering programming languages, understanding complex algorithms, and staying up-to-date with the latest frameworks and tools. However, there’s a crucial skill that’s sometimes overlooked but can make a significant difference in the success of software projects and teams: empathy.
Empathy, the ability to understand and share the feelings of another, might seem out of place in the logical, code-driven world of software development. Yet, it plays a vital role in creating better software, fostering stronger teams, and ultimately delivering more value to users. In this article, we’ll explore the importance of empathy in software development and how it can be cultivated and applied in various aspects of the development process.
Why Empathy Matters in Software Development
Before we dive into the specific applications of empathy in software development, let’s consider why it’s so important:
- User-Centered Design: Empathy helps developers understand and anticipate user needs, leading to more intuitive and user-friendly software.
- Effective Collaboration: In a team environment, empathy fosters better communication and cooperation among developers, designers, and other stakeholders.
- Problem-Solving: Understanding different perspectives can lead to more creative and comprehensive solutions to complex problems.
- Code Quality: Empathetic developers are more likely to write clean, maintainable code that considers future developers and team members.
- Career Growth: Soft skills like empathy are increasingly valued in the tech industry, particularly for leadership roles.
Now that we understand the importance of empathy, let’s explore how it can be applied in various aspects of software development.
Empathy in User Experience (UX) Design
One of the most obvious applications of empathy in software development is in user experience design. Developers who can put themselves in the users’ shoes are better equipped to create interfaces and features that truly meet user needs and preferences.
Practical Applications:
- User Research: Conduct interviews, surveys, and usability tests to understand user pain points and desires.
- Persona Creation: Develop detailed user personas to represent different user types and their unique needs.
- Accessibility Considerations: Design with empathy for users with different abilities and needs.
- Intuitive Navigation: Create navigation structures that align with users’ mental models and expectations.
Consider this example of empathetic design in action:
<!-- Example of empathetic design for a login form -->
<form id="login-form">
<label for="username">Username or Email</label>
<input type="text" id="username" name="username" required>
<label for="password">Password</label>
<input type="password" id="password" name="password" required>
<div class="password-help">
<a href="#" id="show-password">Show password</a>
<a href="#" id="forgot-password">Forgot password?</a>
</div>
<button type="submit">Log In</button>
</form>
In this example, we’ve considered user needs by:
- Allowing login with either username or email, accommodating different user preferences
- Providing a “Show password” option for users who want to verify their input
- Including a prominently placed “Forgot password” link for easy access
Empathy in Code Writing and Documentation
Empathy isn’t just about understanding end-users; it’s also about considering fellow developers who will read, maintain, and build upon your code. Writing empathetic code means creating clear, well-structured, and well-documented solutions that are easy for others to understand and work with.
Practices for Empathetic Coding:
- Clear Naming Conventions: Use descriptive and meaningful names for variables, functions, and classes.
- Comprehensive Comments: Provide context and explanations for complex logic or non-obvious decisions.
- Consistent Formatting: Follow established style guides to maintain consistency across the codebase.
- Modular Design: Structure code in a way that’s easy to understand, test, and modify.
- Thorough Documentation: Write clear README files, API documentation, and inline comments.
Here’s an example of empathetic code vs. non-empathetic code:
// Non-empathetic code
function calc(a, b, c) {
return a ? b + c : b - c;
}
// Empathetic code
/**
* Calculates the result based on a condition and two values.
* @param {boolean} isAddition - Determines whether to add or subtract.
* @param {number} firstValue - The first value in the calculation.
* @param {number} secondValue - The second value in the calculation.
* @returns {number} The result of the calculation.
*/
function calculateConditionalResult(isAddition, firstValue, secondValue) {
if (isAddition) {
return firstValue + secondValue;
} else {
return firstValue - secondValue;
}
}
The empathetic version provides clear naming, a descriptive comment explaining the function’s purpose, and a more readable structure. This makes it much easier for other developers to understand and use the function correctly.
Empathy in Team Collaboration
Software development is rarely a solitary activity. Most projects involve collaboration among team members with diverse backgrounds, skills, and perspectives. Empathy plays a crucial role in fostering effective teamwork and communication.
Ways to Practice Empathy in Team Settings:
- Active Listening: Pay attention to your colleagues’ ideas and concerns, and make an effort to understand their viewpoints.
- Constructive Feedback: Provide feedback in a way that is helpful and considerate of the recipient’s feelings and efforts.
- Inclusivity: Create an environment where all team members feel valued and comfortable sharing their ideas.
- Flexibility: Be open to different working styles and approaches to problem-solving.
- Recognition: Acknowledge and appreciate the contributions of your team members.
Consider this scenario: You’re reviewing a pull request from a junior developer. Here are two possible approaches:
// Non-empathetic code review comment
This implementation is inefficient and doesn't follow our coding standards. Rewrite it.
// Empathetic code review comment
Thank you for your work on this feature. I see some areas where we can improve efficiency and align better with our coding standards. Here are some specific suggestions:
1. Consider using a for...of loop instead of a traditional for loop for better readability.
2. We typically use camelCase for variable names in our project. Could you update 'user_name' to 'userName'?
3. The function 'processData' is quite long. Let's discuss how we might break it down into smaller, more focused functions.
Let me know if you'd like to pair program to work through these changes together!
The empathetic approach provides specific, constructive feedback while acknowledging the developer’s efforts and offering support. This fosters a positive learning environment and strengthens team relationships.
Empathy in Problem-Solving and Debugging
When it comes to solving complex problems or debugging tricky issues, empathy can be a powerful tool. By putting yourself in the shoes of the user experiencing the bug, or imagining how different components of a system interact, you can often uncover solutions more quickly and effectively.
Empathetic Approaches to Problem-Solving:
- User Perspective: Try to recreate the user’s experience and environment when addressing bugs or usability issues.
- System Empathy: Visualize how different parts of the system interact and affect each other.
- Cross-Functional Understanding: Consider the perspectives of other roles (e.g., design, QA, operations) when approaching problems.
- Error Message Empathy: Write clear, helpful error messages that guide users towards solutions.
Let’s look at an example of how empathy can improve error handling:
// Non-empathetic error handling
if (error) {
throw new Error("Invalid input");
}
// Empathetic error handling
if (error) {
if (error.code === "INVALID_EMAIL") {
throw new Error("The email address you entered is not valid. Please check for typos and try again.");
} else if (error.code === "PASSWORD_TOO_SHORT") {
throw new Error("Your password must be at least 8 characters long. Please choose a longer password and try again.");
} else {
throw new Error("An unexpected error occurred. Please try again later or contact support if the problem persists.");
}
}
The empathetic version provides specific, actionable information to the user, helping them understand and resolve the issue more easily.
Cultivating Empathy in Software Development
Empathy is a skill that can be developed and strengthened over time. Here are some strategies for cultivating empathy in your software development practice:
- Practice Active Listening: In meetings and discussions, focus on truly understanding others’ perspectives before formulating your response.
- Seek Diverse Perspectives: Actively seek input from team members with different backgrounds and expertise.
- User Testing and Feedback: Regularly engage with users through testing sessions, surveys, and feedback channels.
- Cross-Functional Collaboration: Work closely with designers, product managers, and other roles to broaden your understanding of the product and its users.
- Mindfulness and Self-Reflection: Practice being aware of your own emotions and biases, which can help you better understand others.
- Read Widely: Explore literature, psychology, and other fields that can enhance your understanding of human behavior and experiences.
- Role-Playing Exercises: Simulate user scenarios or team conflicts to practice empathetic problem-solving.
Overcoming Challenges to Empathy in Software Development
While the benefits of empathy in software development are clear, there can be challenges to implementing empathetic practices. Here are some common obstacles and strategies to overcome them:
1. Time Pressure
Challenge: Tight deadlines can make it tempting to skip user research or write quick, unclear code.
Solution: Emphasize that empathetic practices often save time in the long run by reducing rework and user issues. Incorporate empathy-driven practices into your standard workflow so they become second nature.
2. Technical Focus
Challenge: Some developers may view soft skills like empathy as less important than technical skills.
Solution: Highlight how empathy enhances technical work, leading to better architecture decisions, more intuitive interfaces, and more maintainable code. Share success stories that demonstrate the impact of empathetic development.
3. Remote Work
Challenge: Remote work can make it harder to build connections and understand team members’ perspectives.
Solution: Use video calls when possible, schedule regular check-ins, and create opportunities for informal team interactions. Utilize collaborative tools that help convey tone and context in written communication.
4. Diverse User Base
Challenge: It can be difficult to empathize with a diverse user base with varying needs and backgrounds.
Solution: Conduct thorough user research, create detailed personas, and involve a diverse group of testers and stakeholders in the development process. Consider accessibility needs from the start of the project.
The Future of Empathy in Software Development
As the field of software development continues to evolve, the importance of empathy is likely to grow. Here are some trends and considerations for the future:
1. AI and Empathy
As artificial intelligence becomes more prevalent in software development, there will be new challenges and opportunities related to empathy. Developers will need to consider how to imbue AI systems with empathetic responses and ensure that AI-driven development tools don’t lose the human touch.
2. Empathy in Tech Education
We may see increased emphasis on empathy and other soft skills in computer science education and coding bootcamps. This could include courses on user psychology, team dynamics, and empathetic design principles.
3. Empathy Metrics
Organizations might start to develop metrics and KPIs related to empathy in software development. This could include measures of user satisfaction, code readability, or team collaboration effectiveness.
4. Cross-Cultural Empathy
As software development becomes increasingly global, there will be a growing need for cross-cultural empathy. Developers will need to consider diverse cultural perspectives in both user experience design and team collaboration.
Conclusion
Empathy is not just a “nice-to-have” soft skill in software development; it’s a crucial component that can significantly impact the success of projects, teams, and entire organizations. By understanding and considering the perspectives of users, team members, and other stakeholders, developers can create more intuitive, efficient, and valuable software solutions.
From user experience design to code writing, from team collaboration to problem-solving, empathy touches every aspect of the software development process. By cultivating empathy and integrating it into our daily practices, we can become not just better coders, but better collaborators, innovators, and technology leaders.
As you continue your journey in software development, whether you’re just starting out or you’re a seasoned professional, remember to balance your technical skills with empathetic practices. Seek to understand your users, write code with future developers in mind, listen actively to your team members, and approach problems from multiple perspectives. In doing so, you’ll not only create better software but also contribute to a more inclusive, collaborative, and innovative tech industry.
The code you write tells a story. Make sure it’s one that speaks to the hearts and minds of your users and fellow developers alike. In the end, the most powerful programs are those that successfully bridge the gap between human needs and technological solutions – and empathy is the key to building that bridge.