Pair Programming Across Time Zones: Collaborating with Your Past and Future Selves
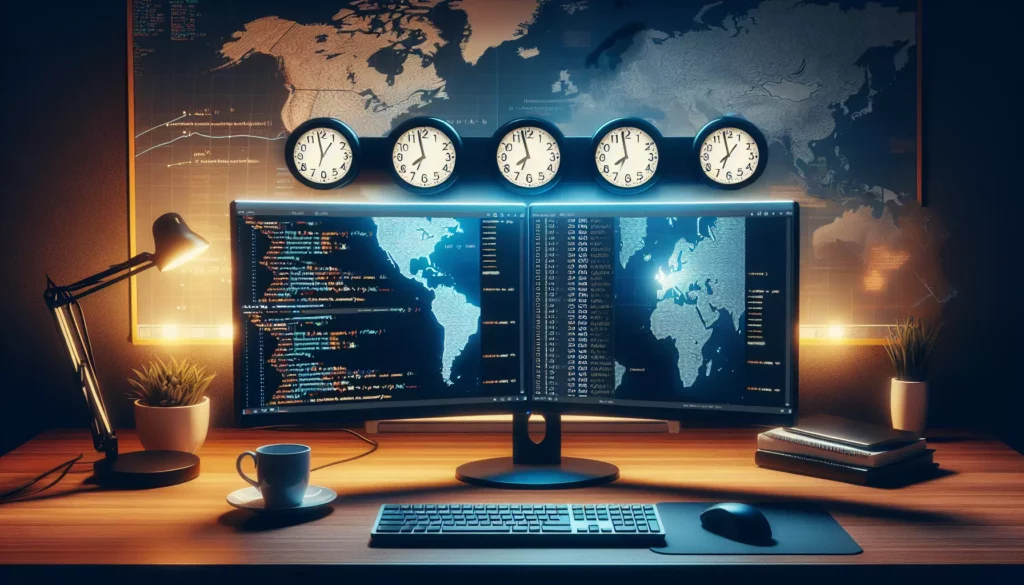
In the ever-evolving landscape of software development, pair programming has emerged as a powerful technique to enhance code quality, knowledge sharing, and team collaboration. But what if we told you that you could engage in a unique form of pair programming that transcends not just geographical boundaries, but temporal ones as well? Welcome to the concept of “Pair Programming Across Time Zones: Collaborating with Your Past and Future Selves” – a revolutionary approach to coding that can supercharge your development skills and productivity.
Understanding Traditional Pair Programming
Before we dive into this time-bending concept, let’s briefly recap what traditional pair programming entails. Pair programming is a software development technique where two programmers work together at one workstation. One, the “driver,” writes code while the other, the “observer” or “navigator,” reviews each line of code as it’s typed in. The two programmers switch roles frequently.
This collaborative approach offers several benefits:
- Improved code quality
- Faster problem-solving
- Knowledge sharing
- Reduced errors
- Enhanced team communication
While these advantages are significant, pair programming across time zones with your past and future selves takes these benefits to a whole new level.
The Concept of Time-Shifted Pair Programming
Time-shifted pair programming is a metaphorical concept that encourages developers to view their coding journey as a collaborative effort between their past, present, and future selves. This approach leverages the power of documentation, version control, and intentional coding practices to create a dialogue across different points in time.
Collaborating with Your Past Self
When you sit down to code, you’re not starting from scratch. You’re building upon the foundation laid by your past self. This collaboration involves:
- Reading and understanding your own code: Treat the code written by your past self as if it were written by a different programmer. This perspective can help you identify areas for improvement and maintain a critical eye.
- Learning from past mistakes: Analyze bugs, inefficiencies, or design flaws in your previous code to avoid repeating them.
- Appreciating growth: Recognize how your coding skills have improved over time, boosting your confidence and motivation.
Collaborating with Your Future Self
Every line of code you write today is a message to your future self. This forward-thinking collaboration involves:
- Writing clear, self-documenting code: Use meaningful variable names, write descriptive comments, and structure your code logically to make it easier for your future self to understand and maintain.
- Creating comprehensive documentation: Document your thought process, design decisions, and any complex algorithms to provide context for your future self.
- Planning for extensibility: Design your code with future enhancements in mind, making it easier for your future self to build upon your work.
Implementing Time-Shifted Pair Programming
Now that we understand the concept, let’s explore how to implement this unique form of pair programming in your daily coding practice.
1. Embrace Version Control
Version control systems like Git are your time machine. They allow you to travel back in time to any point in your project’s history. To maximize the benefits of time-shifted pair programming:
- Commit frequently with meaningful commit messages
- Use branches to experiment with new ideas
- Regularly review your commit history to understand the evolution of your code
Here’s an example of a good commit message:
feat: Add user authentication module
- Implement JWT-based authentication
- Create login and registration endpoints
- Add password hashing using bcrypt
- Write unit tests for auth functions
Closes #123
2. Write Self-Documenting Code
Your code should speak for itself. Use clear and descriptive names for variables, functions, and classes. Here’s an example of poorly documented code versus well-documented code:
Poor example:
function f(x, y) {
return x * y + 100;
}
Better example:
function calculateTotalPrice(basePrice, taxRate) {
const TAX_INCLUSIVE_MARKUP = 100;
return basePrice * (1 + taxRate) + TAX_INCLUSIVE_MARKUP;
}
3. Use Comments Wisely
While self-documenting code is ideal, sometimes complex algorithms or business logic require additional explanation. Use comments to explain the “why” behind your code, not just the “what”. For example:
// Implement the Sieve of Eratosthenes algorithm to find prime numbers
// Time complexity: O(n log log n)
function findPrimes(max) {
// Implementation details...
}
4. Maintain a Coding Journal
Keep a daily or weekly log of your coding activities. This journal can include:
- Challenges you faced and how you overcame them
- New concepts or techniques you learned
- Ideas for future improvements or features
- Reflections on your coding process and productivity
This journal becomes a valuable resource for your future self to understand your thought process and decision-making.
5. Regularly Refactor Your Code
Refactoring is like having a conversation with your past self. It’s an opportunity to improve upon your previous work based on new knowledge and experience. Set aside time regularly to review and refactor your code. This practice not only improves code quality but also reinforces learning and helps you internalize best practices.
6. Use TODO Comments
TODO comments are a great way to communicate with your future self. They highlight areas that need improvement or features that should be implemented later. For example:
// TODO: Optimize this function for better performance
function slowOperation() {
// Current implementation...
}
// TODO: Add input validation to prevent SQL injection
function queryDatabase(userInput) {
// Current implementation...
}
7. Write Tests for Your Future Self
Unit tests serve as executable documentation for your code. They describe how your functions should behave and provide examples of how to use them. Writing comprehensive tests is like leaving a roadmap for your future self. It makes it easier to understand the intended behavior of your code and catch regressions when making changes.
describe('calculateTotalPrice', () => {
it('should calculate the total price with tax and markup', () => {
expect(calculateTotalPrice(100, 0.1)).toBe(210);
});
it('should handle zero base price', () => {
expect(calculateTotalPrice(0, 0.1)).toBe(100);
});
// More test cases...
});
Benefits of Time-Shifted Pair Programming
Adopting this approach to coding offers numerous benefits:
1. Improved Code Quality
By consistently writing code with your future self in mind, you’ll naturally produce cleaner, more maintainable code. This reduces technical debt and makes your codebase more resilient to changes over time.
2. Enhanced Learning and Skill Development
Regularly reviewing and reflecting on your past code accelerates your learning process. You’ll quickly identify areas for improvement and reinforce good coding practices.
3. Better Project Management
Time-shifted pair programming encourages you to think ahead and plan for future developments. This forward-thinking approach can lead to better project architecture and more efficient development cycles.
4. Increased Productivity
Well-documented, self-explanatory code saves time in the long run. You’ll spend less time trying to decipher old code and more time building new features.
5. Improved Problem-Solving Skills
By treating your past code as if it were written by someone else, you develop a more critical and analytical approach to problem-solving. This skill is invaluable in collaborative environments and technical interviews.
Challenges and How to Overcome Them
While time-shifted pair programming offers many benefits, it’s not without its challenges:
1. Time Constraints
Challenge: Taking the time to write detailed documentation and self-explanatory code can feel like it slows down development in the short term.
Solution: Remember that this investment pays off in the long run. Start small by incorporating these practices gradually, and you’ll soon find that they become second nature and actually speed up your overall development process.
2. Overcoming the Curse of Knowledge
Challenge: As you become more familiar with your code, it can be difficult to identify areas that might be confusing to your future self or others.
Solution: Regularly step away from your code and return to it with fresh eyes. Also, consider explaining your code to someone else (or rubber duck debugging) to identify areas that need more clarity.
3. Balancing Detail and Brevity
Challenge: It’s easy to go overboard with comments and documentation, which can clutter your code and potentially become outdated.
Solution: Focus on writing self-documenting code first, and use comments to explain complex logic or provide context that can’t be easily inferred from the code itself. Regularly review and update your documentation as part of your refactoring process.
4. Maintaining Consistency
Challenge: Consistently applying these practices across all your projects and over time can be difficult.
Solution: Develop a personal coding standard or checklist. Use linters and code formatters to enforce consistent style. Consider setting up pre-commit hooks to ensure your commits meet certain quality standards.
Tools and Techniques to Enhance Time-Shifted Pair Programming
Several tools and techniques can support and enhance your time-shifted pair programming practice:
1. Code Analysis Tools
Static code analysis tools can help you identify potential issues in your code that you might have missed. Tools like ESLint for JavaScript, Pylint for Python, or SonarQube for multiple languages can act as an additional pair of eyes reviewing your code.
2. Documentation Generators
Tools like JSDoc for JavaScript, Sphinx for Python, or Doxygen for multiple languages can generate documentation from your code comments. This encourages you to write better comments and provides a convenient way to review your code’s documentation.
3. Code Visualization Tools
Tools that visualize your code structure, such as CodeSee or Sourcetrail, can help you understand complex codebases more easily. This is particularly useful when you’re revisiting old projects or trying to understand the broader context of a piece of code.
4. Time Management Techniques
Techniques like the Pomodoro Technique can help you balance focused coding time with regular breaks for reflection and review. This can enhance your ability to switch between your “present” and “future” programmer mindsets.
5. Code Review Tools
Even if you’re working solo, using code review tools like GitHub’s Pull Requests or GitLab’s Merge Requests can help you review your own code more effectively. You can leave comments for your future self and create a documented history of your decision-making process.
Integrating Time-Shifted Pair Programming with AlgoCademy
AlgoCademy’s focus on coding education and programming skills development makes it an ideal platform to practice and refine your time-shifted pair programming skills. Here’s how you can leverage AlgoCademy’s features to enhance this practice:
1. Interactive Coding Tutorials
Use AlgoCademy’s interactive coding tutorials to experiment with different coding styles and documentation techniques. Try solving the same problem multiple times, each time focusing on a different aspect of time-shifted pair programming (e.g., self-documenting code, comprehensive comments, test-driven development).
2. AI-Powered Assistance
Leverage AlgoCademy’s AI-powered assistance as a stand-in for your future self. Ask it to review your code, suggest improvements, or explain complex sections. This can help you develop a more critical eye for your own code.
3. Problem-Solving Focus
AlgoCademy’s emphasis on algorithmic thinking and problem-solving aligns well with the reflective nature of time-shifted pair programming. As you work through problems, document your thought process and solution strategy. Revisit these notes later to see how your problem-solving approach has evolved.
4. Technical Interview Preparation
As you prepare for technical interviews using AlgoCademy’s resources, practice explaining your code as if you were presenting it to an interviewer. This exercise in clear communication will also benefit your future self when revisiting your code.
5. Progress Tracking
Use AlgoCademy’s progress tracking features to maintain a log of your learning journey. This serves as a valuable reference for your future self, allowing you to see how far you’ve come and identify areas for further improvement.
Conclusion: Embracing Continuous Growth and Collaboration
Time-shifted pair programming is more than just a coding technique; it’s a mindset that promotes continuous learning, self-reflection, and intentional growth. By viewing your coding journey as a collaboration between your past, present, and future selves, you create a feedback loop that constantly pushes you to improve.
This approach aligns perfectly with AlgoCademy’s mission of fostering coding education and skills development. As you progress from beginner-level coding to tackling complex algorithmic challenges, the habits formed through time-shifted pair programming will serve you well. You’ll develop cleaner, more maintainable code, enhance your problem-solving skills, and cultivate a deep understanding of your own learning process.
Remember, every line of code you write today is a message to your future self. Make it clear, make it thoughtful, and make it a stepping stone to becoming the programmer you aspire to be. By mastering the art of collaborating across time zones with yourself, you’ll not only become a better programmer but also a more effective collaborator in any team setting.
So, the next time you sit down to code, take a moment to greet your past self with gratitude and your future self with consideration. Embrace the power of time-shifted pair programming, and watch as your skills and your codebase evolve in ways you never thought possible.