The Coder’s Bucket List: 50 Programming Challenges to Complete Before Your Next Interview
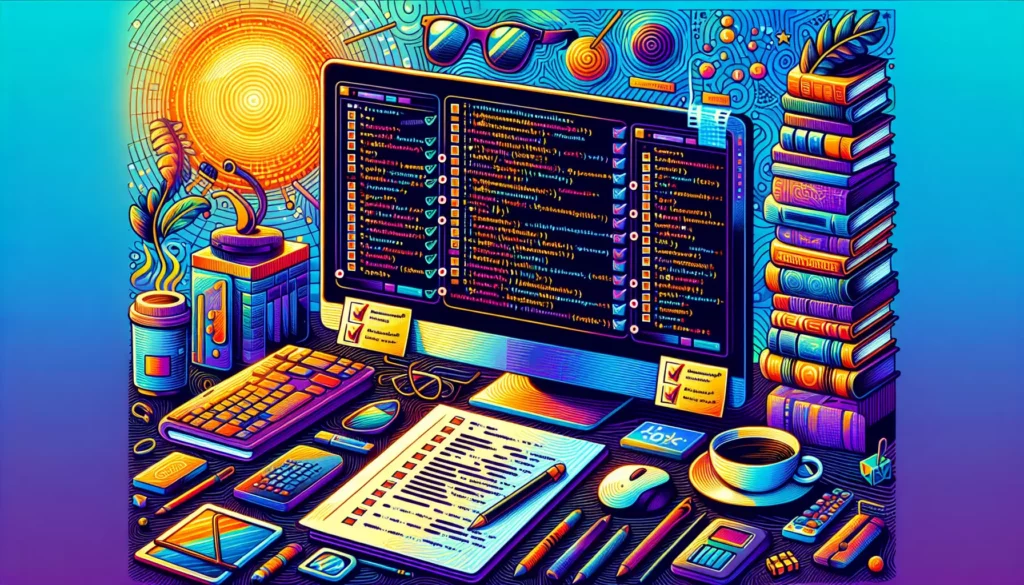
As a programmer, continuous learning and skill improvement are crucial for staying competitive in the ever-evolving tech industry. Whether you’re a beginner looking to solidify your foundation or an experienced developer aiming to sharpen your skills for your next big interview, tackling a variety of programming challenges can significantly boost your abilities and confidence. In this comprehensive guide, we’ve curated a list of 50 programming challenges that every coder should attempt before their next interview, especially if you’re eyeing positions at major tech companies like FAANG (Facebook, Amazon, Apple, Netflix, Google).
Why Complete Programming Challenges?
Before we dive into the list, let’s understand why working on programming challenges is beneficial:
- Skill Enhancement: Regular practice helps refine your coding skills and problem-solving abilities.
- Interview Preparation: Many of these challenges are similar to questions asked in technical interviews.
- Algorithmic Thinking: You’ll develop a stronger grasp of algorithms and data structures.
- Time Management: Working on timed challenges improves your ability to code efficiently under pressure.
- Diverse Knowledge: Exposure to various problem types broadens your programming knowledge.
The 50 Programming Challenges
1. Array Manipulation
- Two Sum: Find two numbers in an array that add up to a specific target.
- Rotate Array: Rotate an array to the right by k steps.
- Remove Duplicates: Remove duplicates from a sorted array in-place.
- Merge Sorted Arrays: Merge two sorted arrays into a single sorted array.
- Maximum Subarray: Find the contiguous subarray with the largest sum.
2. String Manipulation
- Reverse String: Reverse a string in-place.
- Valid Palindrome: Determine if a string is a valid palindrome.
- String to Integer (atoi): Implement the atoi function.
- Longest Substring Without Repeating Characters: Find the length of the longest substring without repeating characters.
- Valid Anagram: Determine if two strings are anagrams of each other.
3. Linked Lists
- Reverse Linked List: Reverse a singly linked list.
- Detect Cycle: Determine if a linked list has a cycle.
- Merge Two Sorted Lists: Merge two sorted linked lists into one sorted list.
- Remove Nth Node From End: Remove the nth node from the end of a linked list.
- Palindrome Linked List: Determine if a linked list is a palindrome.
4. Trees and Graphs
- Maximum Depth of Binary Tree: Find the maximum depth of a binary tree.
- Validate Binary Search Tree: Determine if a binary tree is a valid BST.
- Symmetric Tree: Check if a binary tree is a mirror of itself.
- Binary Tree Level Order Traversal: Traverse a binary tree in level order.
- Graph Valid Tree: Determine if an undirected graph is a valid tree.
5. Dynamic Programming
- Climbing Stairs: Count the number of ways to climb n stairs.
- Coin Change: Find the fewest number of coins that you need to make up an amount.
- Longest Increasing Subsequence: Find the length of the longest increasing subsequence.
- House Robber: Determine the maximum amount of money you can rob from houses.
- Edit Distance: Find the minimum number of operations to convert one string to another.
6. Sorting and Searching
- Merge Sort: Implement the merge sort algorithm.
- Quick Sort: Implement the quick sort algorithm.
- Binary Search: Implement binary search on a sorted array.
- Search in Rotated Sorted Array: Search for a target value in a rotated sorted array.
- Find Median of Two Sorted Arrays: Find the median of two sorted arrays.
7. Stack and Queue
- Valid Parentheses: Determine if the input string has valid parentheses.
- Implement Queue using Stacks: Implement a first-in-first-out (FIFO) queue using only two stacks.
- Min Stack: Design a stack that supports push, pop, top, and retrieving the minimum element in constant time.
- Evaluate Reverse Polish Notation: Evaluate the value of an arithmetic expression in Reverse Polish Notation.
- Sliding Window Maximum: Find the maximum element in each sliding window of size k.
8. Bit Manipulation
- Single Number: Find the single element in an array where every element appears twice except for one.
- Number of 1 Bits: Count the number of ‘1’ bits in an integer.
- Reverse Bits: Reverse the bits of a given 32-bit unsigned integer.
- Power of Two: Determine if a given integer is a power of two.
- Bitwise AND of Numbers Range: Find the bitwise AND of all numbers in a given range.
9. Math and Logic Puzzles
- Fizz Buzz: Print numbers from 1 to n, but for multiples of 3 print “Fizz”, for multiples of 5 print “Buzz”, and for multiples of both 3 and 5 print “FizzBuzz”.
- Power Function: Implement pow(x, n), which calculates x raised to the power n.
- Excel Sheet Column Number: Given a column title as it appears in an Excel sheet, return its corresponding column number.
- Happy Number: Determine if a number is “happy”.
- Sqrt(x): Implement int sqrt(int x) without using any built-in exponent function or operator.
10. System Design and OOP
- Design a Parking Lot: Design a system to manage a multi-level parking lot.
- Implement LRU Cache: Design and implement a data structure for Least Recently Used (LRU) cache.
- Design Twitter: Design a simplified version of Twitter.
- Design a Chess Game: Design the classes and methods for a chess game.
- Design a File System: Design an in-memory file system.
How to Approach These Challenges
Now that you have the list, here’s how to make the most of these programming challenges:
- Start with the basics: If you’re a beginner, start with the easier challenges in each category and gradually move to more complex ones.
- Time yourself: Set a time limit for each challenge to simulate interview conditions.
- Write clean code: Focus on writing clean, readable, and efficient code.
- Test your solutions: Always test your code with various input cases, including edge cases.
- Analyze time and space complexity: For each solution, consider its time and space complexity.
- Learn from others: After solving a problem, look at other solutions to learn different approaches.
- Revisit challenges: Periodically revisit challenges you’ve completed to reinforce your understanding.
Using AlgoCademy to Master These Challenges
AlgoCademy is an excellent platform to work on these programming challenges and prepare for technical interviews. Here’s how you can leverage AlgoCademy’s features:
- Interactive Tutorials: Use AlgoCademy’s step-by-step tutorials to learn the concepts behind each challenge.
- AI-Powered Assistance: When you’re stuck, utilize the AI assistant to get hints or explanations.
- Practice Environment: Use the built-in code editor to implement your solutions and test them in real-time.
- Progress Tracking: Keep track of the challenges you’ve completed and identify areas for improvement.
- Community Support: Engage with the AlgoCademy community to discuss different approaches and learn from peers.
Sample Challenge: Two Sum
Let’s take a closer look at one of the challenges from our list: Two Sum. This is a classic problem often asked in coding interviews.
Problem Statement:
Given an array of integers nums
and an integer target
, return indices of the two numbers such that they add up to target
. You may assume that each input would have exactly one solution, and you may not use the same element twice.
Example:
Input: nums = [2,7,11,15], target = 9
Output: [0,1]
Explanation: Because nums[0] + nums[1] == 9, we return [0, 1].
Solution in Python:
class Solution:
def twoSum(self, nums: List[int], target: int) -> List[int]:
num_dict = {}
for i, num in enumerate(nums):
complement = target - num
if complement in num_dict:
return [num_dict[complement], i]
num_dict[num] = i
return [] # No solution found
Explanation:
- We use a dictionary (hash map) to store the numbers we’ve seen so far and their indices.
- For each number, we calculate its complement (target – num).
- If the complement is in the dictionary, we’ve found our pair and return their indices.
- If not, we add the current number and its index to the dictionary.
- This solution has a time complexity of O(n) and space complexity of O(n).
Conclusion
Completing these 50 programming challenges will significantly improve your coding skills and prepare you for technical interviews, especially for positions at major tech companies. Remember, the key to mastering these challenges is consistent practice and a willingness to learn from each problem you solve.
As you work through this bucket list, take advantage of resources like AlgoCademy to guide your learning journey. With its interactive tutorials, AI-powered assistance, and comprehensive practice environment, AlgoCademy can help you tackle these challenges more effectively and efficiently.
Don’t be discouraged if you find some problems difficult at first. Programming skill development is a gradual process, and each challenge you attempt, regardless of whether you solve it immediately, contributes to your growth as a developer. Keep coding, stay curious, and enjoy the journey of becoming a better programmer!