Coding with Constraints: Solving Problems Using Only 10 Distinct Characters
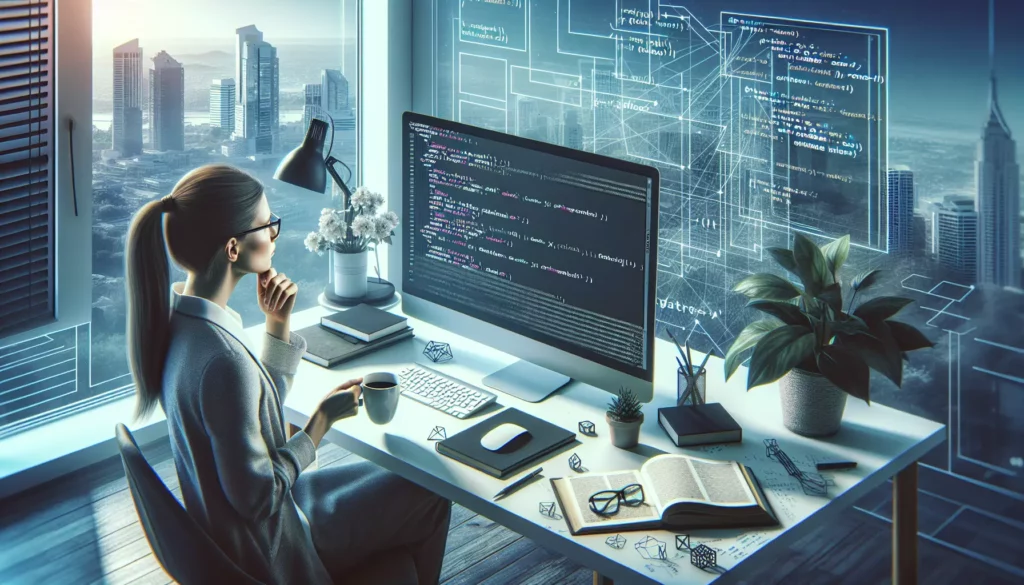
In the world of programming, constraints often lead to creativity and innovation. One intriguing challenge that has gained popularity among coding enthusiasts is the art of solving problems using only a limited set of characters. In this blog post, we’ll explore the fascinating realm of coding with constraints, specifically focusing on solving problems using only 10 distinct characters. This challenge not only tests your problem-solving skills but also pushes you to think outside the box and come up with ingenious solutions.
The 10-Character Challenge
The premise of this challenge is simple yet profound: solve coding problems using only 10 distinct characters. This constraint forces programmers to be creative and efficient in their approach to problem-solving. It’s a great exercise for both beginners and experienced coders alike, as it encourages thinking about programming concepts in a new light.
Let’s start by defining our set of 10 characters. For this challenge, we’ll use the following:
[ ] + - . , > <
These characters are chosen because they form the basis of a minimalist programming language called Brainfuck. While we won’t be programming in Brainfuck directly, understanding its principles can help us approach this challenge more effectively.
Understanding the Basics
Before we dive into solving problems, let’s break down what each of these characters typically represents in this constrained environment:
[
and]
: Used for loops+
and-
: Increment and decrement values.
: Output a value,
: Input a value>
and<
: Move the pointer right or left
With these characters, we can create a simple virtual machine with a memory array and a pointer. The challenge is to manipulate this environment to solve various programming problems.
Problem 1: Hello, World!
Let’s start with the classic “Hello, World!” program. In our constrained environment, we need to output the ASCII values corresponding to each character in the phrase.
++++++++[>++++[>++>+++>+++>+<<<<-]>+>+>->>+[<]<-]>>.>---.+++++++..+++.>>.<-.<.+++.------.--------.>>+.>++.
This code might look cryptic, but let’s break it down:
- We start by setting up a loop to initialize some values in our memory array.
- Then, we move the pointer to different cells, incrementing or decrementing values as needed.
- Finally, we output the ASCII values corresponding to “Hello, World!” using the
.
operator.
While this may seem complex, it demonstrates the power of working within constraints. We’ve managed to output a string using only our 10 allowed characters!
Problem 2: Adding Two Numbers
Now, let’s try something a bit more mathematical. We’ll create a program that adds two single-digit numbers input by the user.
,>,<[>+<-]>.
Here’s how this program works:
,>,
: Input two numbers, moving the pointer to the right after the first input<
: Move the pointer back to the first input[>+<-]
: Add the value of the first cell to the second cell, zeroing out the first cell>.
: Move to the result cell and output the sum
This compact program demonstrates how we can perform basic arithmetic operations within our constraints.
Problem 3: Fibonacci Sequence
Let’s up the ante with a more complex problem: generating the Fibonacci sequence. We’ll aim to output the first 10 Fibonacci numbers.
++++++++++>+>+[<<[>>+<<-]>[>+<<+>-]>[<+>-]<.>]
This program does the following:
- Initialize the first two Fibonacci numbers (1 and 1)
- Enter a loop that calculates the next Fibonacci number
- Output each number in the sequence
- Continue until we’ve output 10 numbers
While this program is more complex, it showcases how we can implement more advanced algorithms using our limited character set.
The Benefits of Constraint-Based Coding
You might be wondering, “Why would anyone want to code like this?” While it’s true that you wouldn’t use this approach for real-world applications, there are several benefits to engaging in constraint-based coding exercises:
1. Improved Problem-Solving Skills
When you’re limited to a small set of tools, you’re forced to think creatively about how to solve problems. This can help you develop new problem-solving strategies that you can apply in other areas of programming.
2. Better Understanding of Low-Level Operations
Working with such a limited set of operations gives you a deeper appreciation for how computers work at a low level. You’ll gain insights into memory management, pointer manipulation, and how higher-level programming constructs are built from simpler operations.
3. Code Optimization
When you’re working with constraints, every character counts. This mindset can help you write more efficient code even when you’re not under such strict limitations.
4. Learning New Programming Paradigms
This style of coding is similar to certain esoteric programming languages and can introduce you to new ways of thinking about code structure and execution.
Challenges for You to Try
Now that you’ve seen a few examples, why not try some challenges yourself? Here are a few problems you can attempt to solve using only our 10 allowed characters:
- Create a program that outputs the squares of numbers from 1 to 10.
- Implement a simple “echo” program that repeats the user’s input.
- Write a program that checks if a number is prime.
- Create a basic calculator that can add, subtract, multiply, or divide two numbers.
Remember, the goal is not just to solve the problem, but to do so within the constraints of our 10-character limit.
Tools and Resources
If you’re interested in exploring this style of programming further, here are some resources you might find helpful:
- Online Interpreters: There are several online interpreters available that allow you to run Brainfuck code (which is compatible with our 10-character constraint). These can be useful for testing and debugging your programs.
- Code Converters: Some tools can convert regular code into Brainfuck-style code, which can help you understand how to approach problems within these constraints.
- Community Forums: Websites like Stack Overflow and Reddit have communities dedicated to esoteric programming languages and coding challenges, where you can find inspiration and ask for help.
Expanding Your Horizons
While the 10-character challenge is a great starting point, there are many other constraint-based coding challenges you can explore:
1. Code Golf
Code Golf is a type of recreational computer programming competition in which participants strive to achieve the shortest possible source code that implements a certain algorithm. It’s similar to our 10-character challenge but allows the use of all characters, focusing instead on minimizing the total code length.
2. Esoteric Programming Languages
There are many esoteric programming languages designed with various constraints or unique paradigms. Some popular ones include:
- Piet: A language where programs look like abstract paintings
- Whitespace: A language that uses only spaces, tabs, and line feeds
- Shakespeare: A language where programs are written in the form of Shakespearean plays
3. One-Liners
Challenge yourself to solve problems using only a single line of code. This is popular in languages like Python and Perl, which have powerful one-liner capabilities.
Integrating Constraints into Your Learning Journey
As you progress in your coding education, you might wonder how exercises like the 10-character challenge fit into your overall learning journey. Here are some ways you can integrate constraint-based coding into your skill development:
1. Algorithmic Thinking
Constraint-based coding forces you to break down problems into their most fundamental operations. This process enhances your algorithmic thinking skills, which are crucial for solving complex programming problems and acing technical interviews.
2. Language Agnostic Skills
The problem-solving skills you develop through these challenges are language-agnostic. They can be applied regardless of the programming language you’re using, making you a more versatile programmer.
3. Interview Preparation
While you won’t be asked to code with just 10 characters in a job interview, the mental agility and creative problem-solving skills you develop can be invaluable. Many technical interviews involve solving problems with constraints, and this practice can help you approach these challenges with confidence.
4. Understanding Compiler Design
Working with a minimal set of operations can give you insights into how compilers and interpreters work. This knowledge can be beneficial if you ever delve into language design or low-level programming.
The Bigger Picture: Constraint as a Catalyst for Innovation
The concept of using constraints to drive innovation isn’t unique to programming. In many fields, constraints have led to groundbreaking innovations:
- In Art: The limited color palettes of early video games led to iconic, recognizable character designs.
- In Engineering: The space constraints in smartphones have driven incredible miniaturization in electronics.
- In Literature: Constraints like the 17-syllable structure of haiku have produced powerful, evocative poetry.
By embracing constraints in coding, you’re participating in a broader tradition of using limitations as a springboard for creativity and innovation.
Conclusion: Embracing the Challenge
Coding with constraints, such as using only 10 distinct characters, is more than just a quirky challenge. It’s a powerful tool for developing your problem-solving skills, deepening your understanding of programming concepts, and fostering creativity in your approach to coding.
As you continue your journey in programming, consider incorporating constraint-based challenges into your practice routine. Whether you’re preparing for technical interviews, looking to improve your algorithmic thinking, or simply wanting to stretch your coding muscles in new ways, these exercises can provide valuable insights and skills.
Remember, the goal isn’t to write production code this way, but to use these exercises as a means of expanding your programming horizons. By pushing the boundaries of what’s possible with limited resources, you’ll become a more resourceful, creative, and skilled programmer.
So, are you ready to take on the 10-character challenge? Grab your keyboard, pick a problem, and see what you can create with just [ ] + - . , > <
. Happy coding!