The Coder’s Museum: Curating a Personal Exhibition of Your Most Elegant Solutions
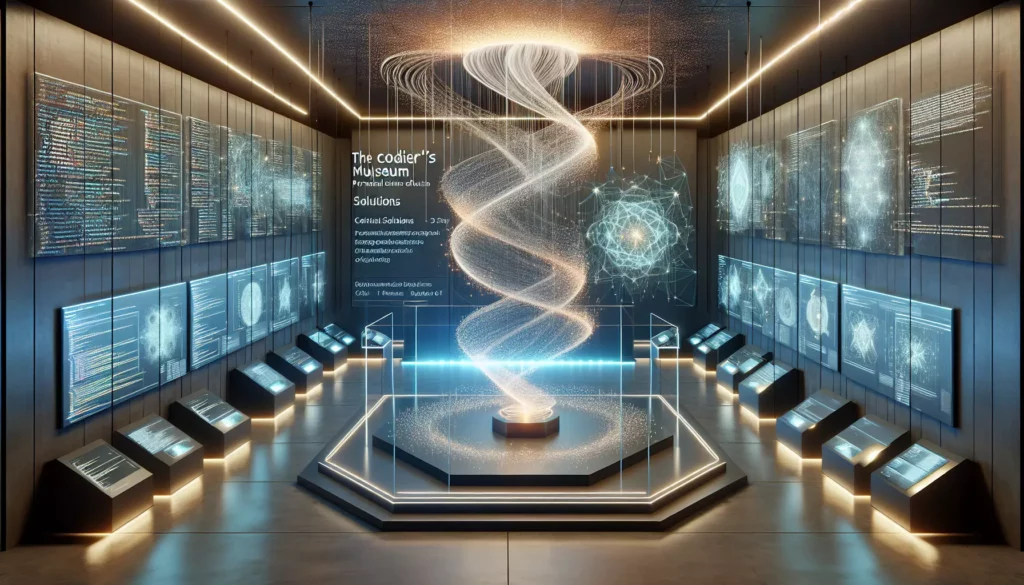
In the vast world of programming, every coder has their masterpieces – those elegant solutions that stand out as pinnacles of creativity, efficiency, and ingenuity. Just as artists curate galleries to showcase their finest works, programmers can benefit immensely from creating their own “Coder’s Museum” – a carefully curated collection of their most impressive code snippets, algorithms, and projects. This practice not only serves as a testament to your growth and achievements but also as a powerful tool for learning, reflection, and professional development.
The Importance of Code Curation
Before we dive into the how-to’s of building your Coder’s Museum, let’s explore why this practice is so valuable:
- Reflection and Growth: Reviewing past code allows you to see how far you’ve come and identify areas for improvement.
- Portfolio Building: A curated collection serves as an impressive showcase of your skills for potential employers or clients.
- Learning Resource: Your elegant solutions can be revisited and adapted for future projects.
- Motivation Boost: Seeing your best work can inspire confidence and drive to tackle new challenges.
- Knowledge Sharing: Your curated code can be a valuable resource for other developers in your community.
Setting Up Your Coder’s Museum
Creating your personal code exhibition doesn’t have to be complicated. Here are some steps to get you started:
1. Choose Your Platform
Select a platform that suits your needs and preferences. Some popular options include:
- GitHub Gists: Perfect for sharing individual code snippets and small scripts.
- Personal Blog: Ideal for in-depth explanations and discussions about your code.
- GitHub Repository: Great for larger projects and collaborative work.
- CodePen: Excellent for front-end demos and interactive examples.
2. Establish Categories
Organize your code into meaningful categories. This could be based on:
- Programming languages (Python, JavaScript, Java, etc.)
- Problem domains (Algorithms, Data Structures, Web Development, etc.)
- Project types (Personal Projects, Work Samples, Code Challenges, etc.)
- Complexity levels (Beginner, Intermediate, Advanced)
3. Select Your Best Work
Choose code samples that truly represent your skills and problem-solving abilities. Look for:
- Elegant and efficient solutions
- Creative approaches to complex problems
- Well-documented and readable code
- Implementations of advanced concepts or algorithms
4. Document and Explain
For each piece in your museum, provide context and explanation:
- Describe the problem or challenge the code addresses
- Explain your approach and why you chose it
- Highlight any particularly clever or efficient parts of your solution
- Discuss what you learned from creating this piece of code
Curating Your Collection: What to Include
Now that you have a framework for your Coder’s Museum, let’s explore the types of code exhibits you might want to include:
1. Algorithmic Masterpieces
Showcase your problem-solving skills with elegant implementations of classic algorithms. For example, a highly optimized sorting algorithm:
def quick_sort(arr):
if len(arr) <= 1:
return arr
pivot = arr[len(arr) // 2]
left = [x for x in arr if x < pivot]
middle = [x for x in arr if x == pivot]
right = [x for x in arr if x > pivot]
return quick_sort(left) + middle + quick_sort(right)
# Example usage
unsorted_list = [3, 6, 8, 10, 1, 2, 1]
sorted_list = quick_sort(unsorted_list)
print(sorted_list) # Output: [1, 1, 2, 3, 6, 8, 10]
Explain why you chose this implementation, its time complexity, and any optimizations you made.
2. Data Structure Innovations
Include any custom data structures you’ve created or interesting uses of existing ones. For instance, a memory-efficient implementation of a Trie for autocomplete functionality:
class TrieNode:
def __init__(self):
self.children = {}
self.is_end_of_word = False
class Trie:
def __init__(self):
self.root = TrieNode()
def insert(self, word):
node = self.root
for char in word:
if char not in node.children:
node.children[char] = TrieNode()
node = node.children[char]
node.is_end_of_word = True
def search(self, prefix):
node = self.root
for char in prefix:
if char not in node.children:
return []
node = node.children[char]
return self._dfs(node, prefix)
def _dfs(self, node, prefix):
results = []
if node.is_end_of_word:
results.append(prefix)
for char, child in node.children.items():
results.extend(self._dfs(child, prefix + char))
return results
# Example usage
trie = Trie()
words = ["apple", "app", "apricot", "banana", "bat"]
for word in words:
trie.insert(word)
print(trie.search("ap")) # Output: ['app', 'apple', 'apricot']
Discuss the advantages of using a Trie for this purpose and how it compares to other data structures.
3. Design Pattern Implementations
Demonstrate your understanding of software design principles with clean implementations of design patterns. Here’s an example of the Observer pattern:
from abc import ABC, abstractmethod
class Subject:
def __init__(self):
self._observers = []
self._state = None
def attach(self, observer):
self._observers.append(observer)
def detach(self, observer):
self._observers.remove(observer)
def notify(self):
for observer in self._observers:
observer.update(self._state)
def set_state(self, state):
self._state = state
self.notify()
class Observer(ABC):
@abstractmethod
def update(self, state):
pass
class ConcreteObserverA(Observer):
def update(self, state):
print(f"ConcreteObserverA: Reacted to the event. New state: {state}")
class ConcreteObserverB(Observer):
def update(self, state):
print(f"ConcreteObserverB: Reacted to the event. New state: {state}")
# Example usage
subject = Subject()
observer_a = ConcreteObserverA()
observer_b = ConcreteObserverB()
subject.attach(observer_a)
subject.attach(observer_b)
subject.set_state("New State")
# Output:
# ConcreteObserverA: Reacted to the event. New state: New State
# ConcreteObserverB: Reacted to the event. New state: New State
Explain how this pattern promotes loose coupling and when it’s appropriate to use.
4. Efficient Data Processing
Include examples of how you’ve optimized data processing tasks. For instance, a parallel processing solution using Python’s multiprocessing module:
import multiprocessing
import time
def process_chunk(chunk):
return [x * x for x in chunk]
def parallel_processing(data, num_processes):
pool = multiprocessing.Pool(processes=num_processes)
chunk_size = len(data) // num_processes
chunks = [data[i:i + chunk_size] for i in range(0, len(data), chunk_size)]
results = pool.map(process_chunk, chunks)
return [item for sublist in results for item in sublist]
if __name__ == '__main__':
data = list(range(10000000))
start_time = time.time()
result_serial = [x * x for x in data]
end_time = time.time()
print(f"Serial processing time: {end_time - start_time:.2f} seconds")
start_time = time.time()
result_parallel = parallel_processing(data, 4)
end_time = time.time()
print(f"Parallel processing time: {end_time - start_time:.2f} seconds")
assert result_serial == result_parallel, "Results don't match!"
Discuss the performance improvements achieved and potential use cases for this approach.
5. Creative Problem-Solving
Highlight instances where you’ve come up with unique solutions to challenging problems. For example, a recursive solution to generate all valid parentheses combinations:
def generate_parenthesis(n):
def backtrack(s='', left=0, right=0):
if len(s) == 2 * n:
result.append(s)
return
if left < n:
backtrack(s + '(', left + 1, right)
if right < left:
backtrack(s + ')', left, right + 1)
result = []
backtrack()
return result
# Example usage
n = 3
valid_combinations = generate_parenthesis(n)
print(valid_combinations)
# Output: ['((()))', '(()())', '(())()', '()(())', '()()()']
Explain the thought process behind this solution and how it efficiently generates all valid combinations.
6. API Design and Implementation
Showcase your ability to design clean and intuitive APIs. Here’s an example of a simple RESTful API using Flask:
from flask import Flask, jsonify, request
app = Flask(__name__)
# In-memory storage for simplicity
tasks = []
@app.route('/tasks', methods=['GET'])
def get_tasks():
return jsonify({'tasks': tasks})
@app.route('/tasks', methods=['POST'])
def create_task():
if not request.json or 'title' not in request.json:
return jsonify({'error': 'Bad request'}), 400
task = {
'id': len(tasks) + 1,
'title': request.json['title'],
'description': request.json.get('description', ""),
'done': False
}
tasks.append(task)
return jsonify({'task': task}), 201
@app.route('/tasks/<int:task_id>', methods=['GET'])
def get_task(task_id):
task = next((task for task in tasks if task['id'] == task_id), None)
if task is None:
return jsonify({'error': 'Task not found'}), 404
return jsonify({'task': task})
@app.route('/tasks/<int:task_id>', methods=['PUT'])
def update_task(task_id):
task = next((task for task in tasks if task['id'] == task_id), None)
if task is None:
return jsonify({'error': 'Task not found'}), 404
task['title'] = request.json.get('title', task['title'])
task['description'] = request.json.get('description', task['description'])
task['done'] = request.json.get('done', task['done'])
return jsonify({'task': task})
@app.route('/tasks/<int:task_id>', methods=['DELETE'])
def delete_task(task_id):
task = next((task for task in tasks if task['id'] == task_id), None)
if task is None:
return jsonify({'error': 'Task not found'}), 404
tasks.remove(task)
return jsonify({'result': True})
if __name__ == '__main__':
app.run(debug=True)
Discuss the principles of RESTful design you’ve applied and how this API could be extended or improved.
Maintaining and Evolving Your Coder’s Museum
Your Coder’s Museum should be a living, breathing entity that grows and evolves with your skills. Here are some tips for maintaining and improving your collection:
1. Regular Updates
Set aside time periodically (e.g., monthly or quarterly) to review and update your museum. Add new pieces that represent your latest achievements and remove or update older ones that no longer meet your standards.
2. Seek Feedback
Share your museum with peers, mentors, or the coding community. Their feedback can provide valuable insights and help you identify areas for improvement.
3. Refactor and Optimize
As you learn new techniques and best practices, revisit your older code samples. Refactor and optimize them to reflect your current skill level and coding standards.
4. Add Context and Learnings
For each piece in your museum, consider adding a section on what you’ve learned since creating it. This can showcase your growth and provide valuable insights to others.
5. Explore New Territories
Challenge yourself to add exhibits from new areas of programming or problem domains. This can help broaden your skills and make your museum more diverse and interesting.
Leveraging Your Coder’s Museum for Career Growth
Your carefully curated collection of code can be a powerful asset in your professional journey. Here are some ways to leverage your Coder’s Museum:
1. Job Applications
Include a link to your museum in your resume or cover letter. This gives potential employers a concrete demonstration of your skills and coding style.
2. Technical Interviews
Use examples from your museum to discuss your problem-solving approach during interviews. This can help you articulate your thought process and showcase your best work.
3. Networking
Share your museum at coding meetups or conferences. It can serve as a great conversation starter and help you connect with like-minded professionals.
4. Blogging and Content Creation
Use your curated code samples as the basis for blog posts or tutorials. This can help establish you as a thought leader in your areas of expertise.
5. Open Source Contributions
Your elegant solutions might be valuable to open source projects. Use your museum as a springboard for contributing to the wider coding community.
Conclusion: Your Code, Your Legacy
Creating and maintaining a Coder’s Museum is more than just a showcase of your technical skills – it’s a reflection of your journey as a programmer, a tool for continuous learning, and a platform for sharing knowledge with others. By carefully curating your most elegant solutions, you not only create a valuable resource for yourself but also contribute to the broader programming community.
Remember, every great coder started somewhere, and your museum is a testament to how far you’ve come and the exciting places you’re yet to go. So start building your Coder’s Museum today, and let your code tell the story of your growth, creativity, and passion for programming.
As you continue to learn and grow, your museum will evolve, becoming an ever more impressive collection of your achievements. Who knows? The elegant solutions you craft today might inspire the next generation of coders tomorrow. Happy coding, and may your Coder’s Museum always be a source of pride and inspiration!