The Storyteller’s Code: Crafting Compelling Narratives in Your GitHub Commits
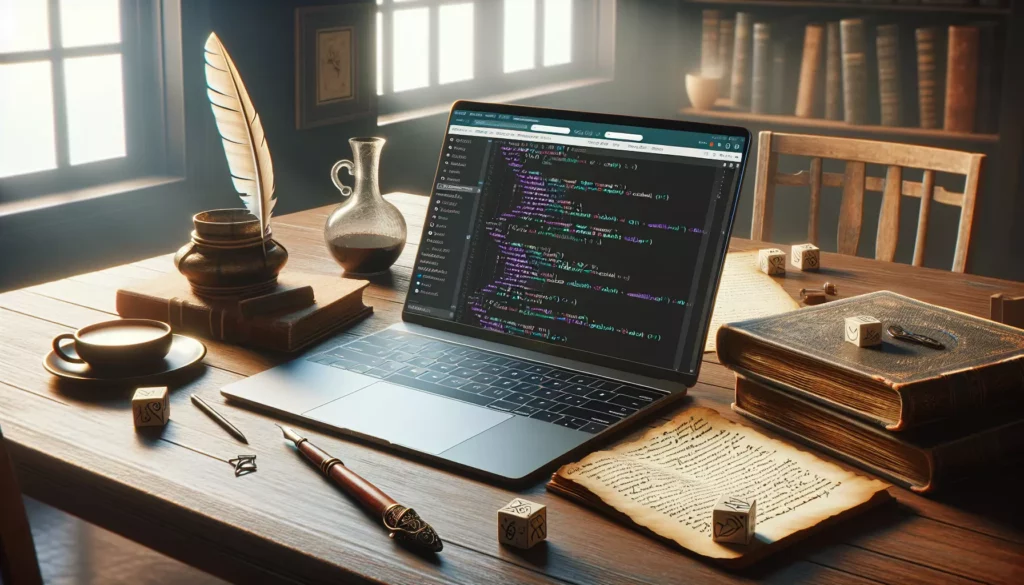
In the vast realm of software development, where lines of code weave intricate digital tapestries, there lies an often-overlooked art form: the commit message. These brief snippets of text, accompanying each change in your codebase, are more than mere documentation—they are the storytellers of your project’s evolution. Welcome to “The Storyteller’s Code,” where we’ll explore the art and science of crafting compelling narratives in your GitHub commits.
The Importance of Commit Messages in Coding Education
Before we dive into the intricacies of writing effective commit messages, let’s consider why this skill is crucial, especially in the context of coding education and programming skills development.
For learners navigating the complex world of coding, from beginner-level tutorials to advanced algorithmic challenges, understanding the history and reasoning behind code changes is invaluable. Well-crafted commit messages serve as breadcrumbs, guiding both the author and future readers through the labyrinth of development decisions.
In platforms like AlgoCademy, which focus on interactive coding tutorials and preparing for technical interviews, the ability to read and write clear commit messages can significantly enhance the learning experience. It’s not just about writing code; it’s about communicating the thought process behind the code—a skill highly valued in collaborative environments and technical interviews alike.
The Anatomy of a Great Commit Message
A well-structured commit message typically consists of three main parts:
- Subject Line: A concise summary of the change (50 characters or less)
- Blank Line: Separating the subject from the body
- Body: A more detailed explanation of the what and why of the change (if needed)
Let’s break these down and explore best practices for each component.
1. The Subject Line: Your Commit’s Headline
The subject line is the most critical part of your commit message. It’s what appears in the git log and should provide a clear, concise summary of the change. Here are some guidelines for crafting an effective subject line:
- Keep it short: Aim for 50 characters or less. This ensures readability in various Git tools.
- Use the imperative mood: Write as if you’re giving a command. For example, “Fix bug” instead of “Fixed bug” or “Fixes bug”.
- Capitalize the first letter: Start with a capital letter for consistency.
- Don’t end with a period: It’s a title, not a sentence.
- Be specific: Provide enough context to understand the change at a glance.
Here are some examples of good subject lines:
Add login functionality to user dashboard
Optimize database queries for faster load times
Refactor calculateTotal() method for better readability
Fix off-by-one error in pagination logic
2. The Blank Line: The Unsung Hero
The blank line between the subject and body might seem trivial, but it serves an important purpose. It separates the summary from the detailed explanation, making the commit message more readable and allowing tools to display the subject line separately from the body.
3. The Body: Diving Deeper
Not all commits require a body, but for complex changes or those that need additional context, the body is your canvas to paint a more detailed picture. Here are some tips for writing an effective commit body:
- Explain the what and why, not the how: The code itself shows how the change was made. Use the body to explain what was changed and why it was necessary.
- Use bullet points for clarity: If you’re listing multiple points, bullet points can make the message more readable.
- Wrap lines at 72 characters: This ensures good readability across various tools.
- Reference relevant issues or pull requests: If the commit is related to a specific issue or PR, mention it.
Here’s an example of a commit message with a body:
Implement caching for frequently accessed data
- Add Redis caching layer to store user profiles
- Reduce database load during peak hours
- Improve response time for profile page by 60%
Resolves: #123
See also: #456, #789
The Art of Storytelling in Commits
Now that we’ve covered the structure, let’s explore how to infuse your commit messages with narrative flair. Remember, you’re not just documenting changes; you’re telling the story of your project’s evolution.
1. Provide Context
Good stories don’t exist in a vacuum, and neither should your commits. Provide context that helps readers understand the bigger picture. For example:
Update user authentication flow
As part of our security overhaul (see #234), we're
implementing multi-factor authentication. This commit
adds the initial framework for SMS-based 2FA.
2. Explain the Reasoning
Don’t just state what you did; explain why you did it. This is especially important for non-obvious changes or decisions that might be questioned later. For instance:
Replace custom sorting algorithm with built-in method
Our custom QuickSort implementation was causing
performance issues with large datasets. Switching to
the language's built-in sorting method provides better
performance and reduces maintenance overhead.
3. Describe the Impact
When applicable, mention the impact of your changes. This could include performance improvements, bug fixes, or new features. For example:
Optimize image loading process
- Implement lazy loading for images
- Compress images on upload
These changes reduce initial page load time by 40%
and decrease bandwidth usage by approximately 2 MB
per page view.
4. Use a Consistent Style
Consistency in your commit messages helps create a coherent narrative throughout your project’s history. Consider establishing a commit message template or guidelines for your team or personal projects. For example:
[Type]: Short description
Longer description if necessary. Keep it wrapped to
72 characters. Use bullet points if needed:
- Point 1
- Point 2
[Type] can be:
- Feature: A new feature
- Fix: A bug fix
- Docs: Documentation changes
- Style: Changes that do not affect the meaning of the code
- Refactor: Code change that neither fixes a bug nor adds a feature
- Test: Adding missing tests or correcting existing tests
- Chore: Changes to the build process or auxiliary tools
Advanced Techniques for Commit Storytelling
As you become more comfortable with basic commit message practices, consider these advanced techniques to elevate your commit storytelling:
1. Link Related Commits
For complex features or bug fixes that span multiple commits, create a narrative thread by referencing related commits. This helps readers understand the progression of changes. For example:
Implement user profile page (1/3)
This is the first in a series of commits to implement
the user profile feature. This commit sets up the
basic route and controller structure.
See also: [link to next commit]
2. Use Emojis (Judiciously)
Emojis can add visual flair to your commit messages and quickly convey the nature of the change. However, use them sparingly and consistently. Some teams adopt conventions like:
✨ Add new feature: dark mode toggle
🛠Fix login button not responding on mobile
📚 Update API documentation
🔧 Refactor database connection logic
3. Include Before and After Comparisons
For significant changes, especially those affecting performance or user experience, consider including before and after comparisons in your commit message. This provides tangible evidence of improvement. For instance:
Optimize database query for user search
Before: Avg. query time 1.2s
After: Avg. query time 0.3s
Implemented indexing on frequently searched fields
and refactored the query to use more efficient joins.
4. Reference External Resources
If your change is based on external research, a specific algorithm, or a design pattern, include references in your commit message. This provides valuable context and learning opportunities for others (and your future self). For example:
Implement Levenshtein distance algorithm for fuzzy search
Based on the algorithm described in:
https://en.wikipedia.org/wiki/Levenshtein_distance
This implementation improves search accuracy for
misspelled product names by 35%.
Common Pitfalls to Avoid
Even with the best intentions, it’s easy to fall into bad habits when writing commit messages. Here are some common pitfalls to watch out for:
1. Vague or Uninformative Messages
Avoid commit messages that don’t provide any real information about the change. Messages like “Fix stuff”, “Update”, or “WIP” (Work in Progress) are not helpful and should be avoided.
2. Focusing on the “How” Instead of the “What” and “Why”
Remember, the code itself shows how you made the change. Your commit message should focus on what was changed and why it was necessary.
3. Including Temporary or Debug Code
Avoid committing temporary or debug code. If you must commit unfinished work, clearly label it as such in the commit message and explain why it’s being committed in an unfinished state.
4. Combining Multiple Unrelated Changes
Each commit should represent a single logical change. If you find yourself writing “and” in your commit message, it might be a sign that you should split the commit into multiple, more focused commits.
5. Neglecting to Update the Commit Message After Amending Changes
If you use git commit --amend
to make changes to your commit, don’t forget to update the commit message if necessary to reflect the amended changes.
Tools and Techniques for Better Commit Messages
To help you craft better commit messages and maintain consistency, consider using these tools and techniques:
1. Git Hooks
Git hooks are scripts that Git executes before or after events such as commit, push, and receive. You can use a pre-commit hook to enforce commit message standards. For example, you could create a hook that checks the commit message format and rejects the commit if it doesn’t meet your standards.
Here’s a simple example of a pre-commit hook that checks the commit message length:
#!/bin/sh
commit_msg_file=$1
commit_msg=$(cat "$commit_msg_file")
if [ ${#commit_msg} -gt 50 ]; then
echo "Commit message is too long (${#commit_msg} characters). Max length is 50 characters."
exit 1
fi
2. Commit Templates
Git allows you to set up commit message templates. These can serve as a reminder of your commit message structure and prompt you to include all necessary information. To set up a commit template:
- Create a file (e.g.,
.gitmessage
) with your template:
Subject line (50 chars or less)
More detailed explanation, if necessary. Wrap it to
72 characters. Consider including:
- Why was this change necessary?
- How does it address the issue?
- What side effects does this change have?
Include any relevant issue numbers.
Resolves: #123
See also: #456, #789
- Set the template in your Git config:
git config --global commit.template ~/.gitmessage
3. Conventional Commits
Conventional Commits is a specification for adding human and machine-readable meaning to commit messages. It provides an easy set of rules for creating an explicit commit history, which makes it easier to write automated tools on top of. The basic structure is:
<type>[optional scope]: <description>
[optional body]
[optional footer(s)]
Where type
can be:
- feat: A new feature
- fix: A bug fix
- docs: Documentation only changes
- style: Changes that do not affect the meaning of the code
- refactor: A code change that neither fixes a bug nor adds a feature
- perf: A code change that improves performance
- test: Adding missing tests or correcting existing tests
- chore: Changes to the build process or auxiliary tools
4. IDE Integration
Many Integrated Development Environments (IDEs) offer features to help with commit messages. For example:
- Visual Studio Code: Extensions like “Conventional Commits” can provide templates and validation for commit messages.
- IntelliJ IDEA: Offers a built-in spell-checker for commit messages and can be configured to show a character count.
- GitKraken: Provides a commit message editor with a character count and the ability to set up templates.
The Impact of Good Commit Messages on Collaboration and Learning
In the context of coding education and skill development, the importance of well-crafted commit messages cannot be overstated. They serve multiple crucial purposes:
1. Enhancing Code Review Processes
Clear, descriptive commit messages make code reviews more efficient and effective. Reviewers can quickly understand the context and purpose of changes, allowing them to focus on the implementation details and potential issues.
2. Facilitating Knowledge Transfer
In educational settings or when onboarding new team members, a well-documented commit history serves as a valuable learning resource. It provides insights into the decision-making process, problem-solving approaches, and the evolution of the codebase.
3. Improving Debugging and Troubleshooting
When issues arise, a detailed commit history can be invaluable in tracking down the source of problems. Good commit messages can help pinpoint when and why specific changes were made, speeding up the debugging process.
4. Encouraging Best Practices
The practice of writing thoughtful commit messages encourages developers to think more critically about their changes. It promotes smaller, more focused commits and clearer reasoning about code modifications.
5. Preparing for Technical Interviews
For those preparing for technical interviews, especially with major tech companies, the ability to articulate changes and decisions clearly is a valuable skill. Writing good commit messages is excellent practice for explaining technical concepts concisely and effectively.
Conclusion: Elevating Your Coding Narrative
As we conclude our journey through “The Storyteller’s Code,” remember that every commit message you write is an opportunity to contribute to your project’s narrative. Whether you’re a beginner working through coding tutorials or an experienced developer preparing for technical interviews, the art of crafting compelling commit messages is a skill that will serve you well throughout your coding career.
By following the guidelines and techniques we’ve explored—from structuring your messages effectively to providing context and explaining the reasoning behind your changes—you’ll create a more transparent, collaborative, and educational development process. Your commit history will become not just a record of changes, but a rich tapestry of decisions, improvements, and learning experiences.
As you continue to develop your coding skills and tackle increasingly complex challenges, let your commit messages tell the story of your growth and the evolution of your projects. In doing so, you’ll not only become a better developer but also a more effective communicator and collaborator—skills that are invaluable in any technical role.
Remember, every line of code has a story to tell. As the author of that story, it’s up to you to make it compelling, informative, and worthy of the amazing work you’re doing. Happy coding, and may your commit messages always be clear, concise, and illuminating!