The Coder’s Family Tree: Tracing Your Programming Lineage Through Languages and Influences
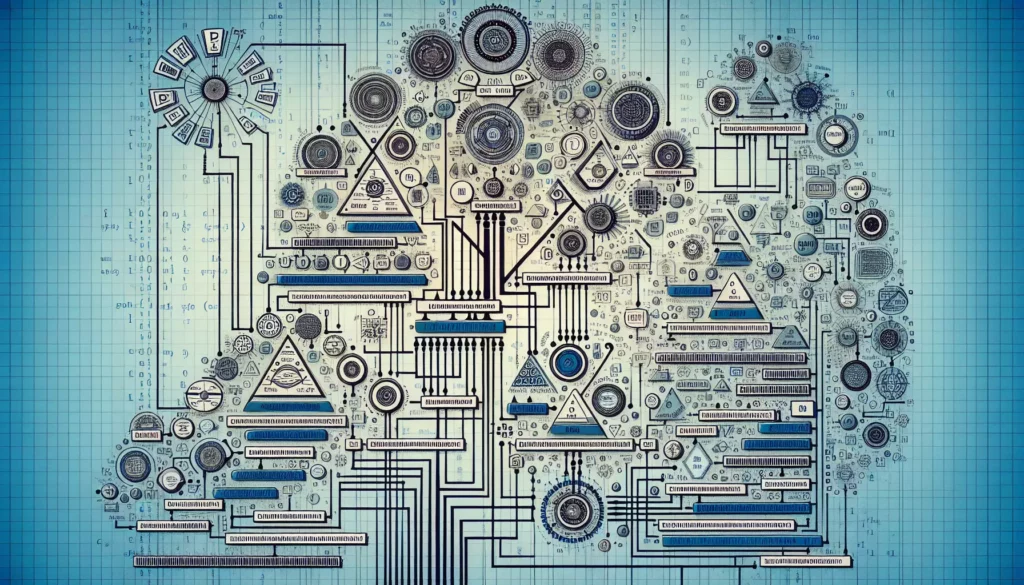
As a programmer, have you ever wondered about your coding ancestry? Just as we can trace our family lineage through generations, we can also explore the rich history and interconnections of programming languages. In this deep dive, we’ll embark on a journey through the coder’s family tree, uncovering the influences, innovations, and evolution that have shaped the programming landscape we know today.
The Roots: Early Programming Languages
To understand our coding lineage, we must first look at the roots of modern programming. These early languages laid the foundation for everything that followed:
1. Assembly Language (1940s)
At the very base of our family tree, we find Assembly language. This low-level language was the first step away from programming directly in machine code. While it’s still used today for specific tasks, Assembly served as the springboard for higher-level languages.
2. FORTRAN (1957)
FORTRAN (FORmula TRANslation) was one of the first high-level programming languages. Developed by IBM, it was designed for scientific and engineering calculations. FORTRAN introduced many concepts we still use today, such as variables, expressions, and subroutines.
3. COBOL (1959)
COBOL (Common Business-Oriented Language) was created to standardize business data processing. It introduced the concept of structured programming and emphasized readability with its English-like syntax.
4. LISP (1958)
LISP (LISt Processor) brought functional programming to the forefront. It introduced crucial concepts like tree data structures, automatic storage management, and dynamic typing.
The Trunk: Foundational Languages
As we move up our family tree, we encounter languages that formed the trunk from which many modern languages branched:
1. ALGOL (1958)
ALGOL (ALGOrithmic Language) was influential in the development of many subsequent languages. It introduced block structure and the concept of scope, which are fundamental to modern programming.
2. C (1972)
C, developed by Dennis Ritchie at Bell Labs, is perhaps one of the most influential languages in our family tree. Its efficiency and power made it ideal for system programming, and its syntax has influenced countless languages that followed.
// Hello World in C
#include <stdio.h>
int main() {
printf("Hello, World!\n");
return 0;
}
3. Smalltalk (1980)
Smalltalk pioneered the object-oriented programming paradigm. It introduced concepts like objects, classes, and inheritance, which have become staples in modern programming.
The Branches: Modern Language Families
From these foundational languages, several distinct branches emerged, each with its own philosophy and strengths:
1. The C Family
C++ (1979), Objective-C (1984), and Java (1995) all descended from C. Each built upon C’s syntax while introducing new features:
- C++ added object-oriented features to C
- Objective-C blended Smalltalk’s object-oriented concepts with C
- Java aimed for platform independence with its “write once, run anywhere” philosophy
// Hello World in Java
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
2. The Scripting Language Branch
This branch includes languages designed for rapid development and ease of use:
- Python (1991): Known for its readability and versatility
- Ruby (1995): Emphasizes simplicity and productivity
- JavaScript (1995): Originally for web browsers, now used server-side as well
# Hello World in Python
print("Hello, World!")
3. The Functional Programming Branch
Descending from LISP, this branch includes languages that emphasize immutable data and functions as first-class citizens:
- Haskell (1990): A purely functional language
- Scala (2004): Combines functional and object-oriented paradigms
- Clojure (2007): A modern LISP dialect for the Java Virtual Machine
-- Hello World in Haskell
main :: IO ()
main = putStrLn "Hello, World!"
The Leaves: Modern and Specialized Languages
At the tips of our branches, we find the most recent additions to our family tree:
1. Go (2009)
Developed by Google, Go aims to combine the ease of programming of an interpreted, dynamically typed language with the efficiency and safety of a statically typed, compiled language.
// Hello World in Go
package main
import "fmt"
func main() {
fmt.Println("Hello, World!")
}
2. Rust (2010)
Rust focuses on safety and concurrency, aiming to be a safer alternative to C++ for systems programming.
// Hello World in Rust
fn main() {
println!("Hello, World!");
}
3. Swift (2014)
Developed by Apple as a replacement for Objective-C, Swift aims to be safer and more concise while maintaining interoperability with existing Objective-C code.
// Hello World in Swift
print("Hello, World!")
4. Kotlin (2011)
Designed to interoperate with Java while addressing some of its pain points, Kotlin has gained popularity, especially in Android development.
// Hello World in Kotlin
fun main() {
println("Hello, World!")
}
Cross-Pollination: How Languages Influence Each Other
Just as in nature, our programming family tree isn’t strictly hierarchical. Languages often influence each other across branches:
1. JavaScript and Java
Despite the similar names, JavaScript and Java are quite different. However, JavaScript’s syntax was influenced by Java, leading to some superficial similarities.
2. Python and Ruby
These two languages, while distinct, have influenced each other over the years. Both emphasize readability and have similar philosophies about making programming enjoyable.
3. C# and Java
C# was Microsoft’s answer to Java, and the two languages have continued to evolve in parallel, often adopting similar features.
The Ecosystem: Beyond Languages
Our family tree isn’t just about languages. It also includes the rich ecosystem of tools, frameworks, and paradigms that have evolved alongside them:
1. Integrated Development Environments (IDEs)
IDEs like Visual Studio, IntelliJ IDEA, and Eclipse have evolved to support multiple languages and provide powerful development tools.
2. Frameworks and Libraries
Frameworks like React for JavaScript, Django for Python, and Spring for Java have become integral parts of modern development.
3. Version Control Systems
Tools like Git have revolutionized how we collaborate on code, becoming an essential part of every programmer’s toolkit.
Tracing Your Own Coding Lineage
Now that we’ve explored the broader family tree, it’s time to consider your own coding lineage. Here are some questions to ponder:
1. What was your first programming language?
For many, this might be a scripting language like Python or JavaScript. For others, it might be a more traditional language like C++ or Java.
2. Which languages have influenced your coding style?
Even if you primarily use one language, you may find that concepts from other languages have shaped how you approach problems.
3. What paradigms do you prefer?
Are you more comfortable with object-oriented programming, functional programming, or a mix of both? This can often be traced back to the languages you’ve used most.
4. How has your “lineage” evolved over time?
Consider how your language preferences and programming style have changed as you’ve gained experience and explored different parts of the family tree.
The Future: New Branches on the Horizon
As technology continues to evolve, new branches are constantly being added to our family tree:
1. Quantum Computing Languages
Languages like Q# are emerging to tackle the unique challenges of quantum computing.
2. AI and Machine Learning Focused Languages
While not entirely new languages, extensions and libraries for existing languages are evolving to better support AI and machine learning tasks.
3. Domain-Specific Languages (DSLs)
More specialized languages are being developed for specific domains, from financial modeling to bioinformatics.
Conclusion: Embracing Your Coding Heritage
Understanding the coder’s family tree isn’t just an academic exercise. It provides valuable context for the tools we use every day and can inform our choices as we continue to grow as programmers.
By tracing the lineage of programming languages, we gain appreciation for the innovations that have shaped our field. We see how ideas have evolved, been refined, and sometimes come full circle. This knowledge can help us make informed decisions about which languages to learn, which paradigms to explore, and how to approach problem-solving in our own code.
Moreover, understanding our coding lineage connects us to the broader community of developers past and present. It reminds us that we’re part of a continuum of innovation and creativity that stretches back decades and will continue into the future.
As you continue your coding journey, take time to explore different branches of the family tree. Each language and paradigm offers unique perspectives that can enrich your skills and broaden your understanding of programming as a whole.
Remember, the best programmers are those who can adapt and learn from various sources. By embracing the diversity of our coding heritage, we position ourselves to be more versatile, creative, and effective developers.
So, the next time you write a line of code, take a moment to appreciate the rich history behind it. You’re not just writing a program; you’re adding your own leaf to the ever-growing coder’s family tree.
Further Exploration
If you’re inspired to dive deeper into the history and connections between programming languages, here are some resources to explore:
- “The Evolution of Programming Languages” by ACM (Association for Computing Machinery)
- “Programming Language Pragmatics” by Michael L. Scott
- The Computer History Museum’s online exhibitions on software and programming languages
- Language-specific documentation and histories, often found on official language websites
Remember, every time you learn a new language or concept, you’re not just adding to your skill set – you’re connecting yourself to a rich tapestry of computing history and innovation. Happy coding, and may your branch of the family tree continue to grow and flourish!