The Philosopher Coder: Examining the Existential Implications of Recursion
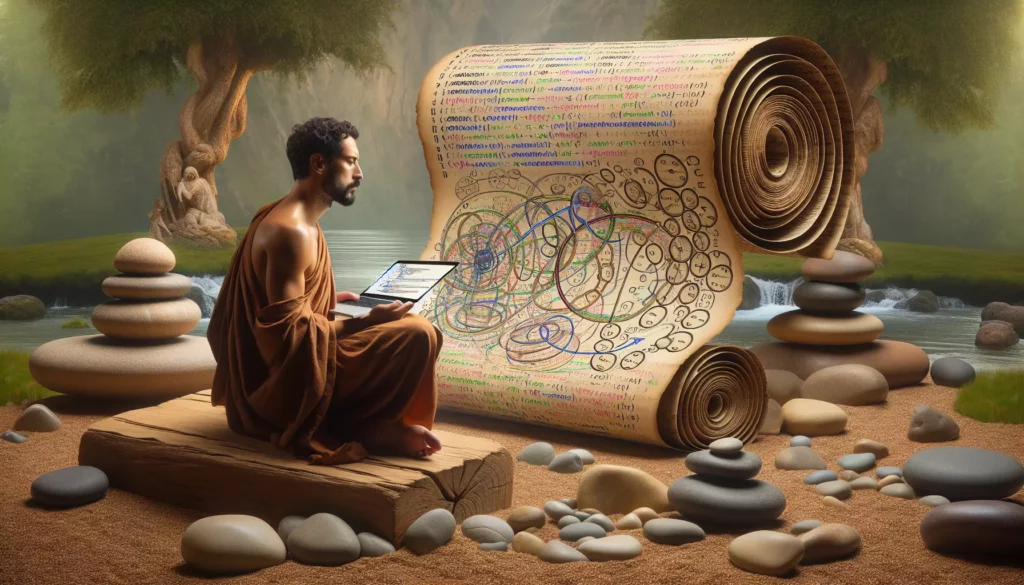
In the vast landscape of computer science and programming, few concepts are as intellectually stimulating and philosophically rich as recursion. As we delve into the world of coding education and algorithmic thinking, it’s crucial to understand not just the technical aspects of recursion, but also its profound implications on how we perceive reality, problem-solving, and even our own existence. This exploration is particularly relevant for those embarking on their coding journey, whether through platforms like AlgoCademy or preparing for technical interviews at major tech companies.
What is Recursion?
Before we dive into the philosophical depths, let’s establish a clear understanding of recursion in programming terms. Recursion is a method of solving problems by breaking them down into smaller, similar subproblems. In coding, this typically involves a function that calls itself with a modified input, continuing until it reaches a base case that doesn’t require further recursion.
Here’s a simple example of a recursive function in Python that calculates the factorial of a number:
def factorial(n):
if n == 0 or n == 1:
return 1
else:
return n * factorial(n - 1)
This function demonstrates the key elements of recursion:
- A base case (when n is 0 or 1) that doesn’t require further recursion
- A recursive case that calls the function with a modified input (n – 1)
- Progress towards the base case with each recursive call
The Philosophical Implications of Recursion
Now that we have a basic understanding of recursion in programming, let’s explore its deeper, philosophical implications. The concept of recursion extends far beyond the realm of coding and touches upon fundamental questions about existence, knowledge, and reality.
1. Infinite Regress and the Nature of Reality
Recursion in programming often leads us to contemplate the concept of infinite regress in philosophy. Infinite regress refers to a series of propositions that require an infinite chain of justification, with each step requiring further explanation. This concept is closely related to foundationalism in epistemology, which seeks to find a basis for knowledge that doesn’t require further justification.
In the context of recursion, we can draw parallels to the way recursive functions call themselves repeatedly. Just as a poorly designed recursive function might lead to a stack overflow, philosophical infinite regress can lead to logical dead ends. This prompts us to question the nature of reality itself: Is there a foundational “base case” to our existence, or is reality inherently recursive?
2. Self-Reference and Consciousness
Recursion inherently involves self-reference, as functions call themselves. This concept of self-reference has profound implications when we consider consciousness and self-awareness. Douglas Hofstadter, in his seminal work “Gödel, Escher, Bach: An Eternal Golden Braid,” explores how self-reference and recursion might be fundamental to consciousness itself.
Consider this recursive definition of consciousness:
def consciousness(self):
if not self.is_aware():
return False
else:
return self.think_about(consciousness(self))
This pseudo-code, while not practically implementable, illustrates the recursive nature of self-awareness. Our ability to think about our own thinking process is a form of mental recursion that may be at the core of what we consider consciousness.
3. Problem-Solving and Reductionism
The recursive approach to problem-solving in programming mirrors philosophical reductionism, the idea that complex systems can be understood by breaking them down into simpler, more fundamental parts. This approach has been influential in various fields, from physics to cognitive science.
However, just as there are limitations to recursion in programming (e.g., stack overflow), there are also critiques of reductionism in philosophy. Some argue that certain phenomena, particularly in fields like biology and psychology, exhibit emergent properties that cannot be fully explained by their constituent parts alone.
4. Time and Causality
Recursion often involves a form of time travel in computation, where we dive deeper into a problem before resolving earlier stages. This concept can lead us to philosophical questions about the nature of time and causality. Is time truly linear, or is there a recursive element to it?
Consider the concept of retrocausality in quantum physics, where future events might influence the past. This mind-bending idea shares some conceptual similarities with how recursive functions operate, resolving later calls before earlier ones complete.
Practical Applications of Recursive Thinking
While these philosophical musings are intellectually stimulating, it’s essential to ground our discussion in practical applications. Understanding recursion and its implications can significantly enhance problem-solving skills, particularly in the context of coding education and technical interview preparation.
1. Algorithmic Problem-Solving
Many complex algorithmic problems are most elegantly solved using recursive approaches. Classic examples include:
- Tree traversal algorithms
- Divide-and-conquer algorithms like QuickSort
- Dynamic programming problems
- Backtracking algorithms for combinatorial problems
Let’s look at a simple example of a recursive solution for generating all permutations of a string:
def generate_permutations(s):
if len(s) == 1:
return [s]
perms = []
for i in range(len(s)):
char = s[i]
remaining_chars = s[:i] + s[i+1:]
for perm in generate_permutations(remaining_chars):
perms.append(char + perm)
return perms
# Example usage
print(generate_permutations("abc"))
# Output: ['abc', 'acb', 'bac', 'bca', 'cab', 'cba']
This recursive solution elegantly breaks down the problem into smaller subproblems, demonstrating the power of recursive thinking in algorithmic design.
2. System Design and Architecture
Recursive thinking can be invaluable in system design and software architecture. Many complex systems can be modeled using recursive structures, such as:
- File systems (directories containing subdirectories)
- Organizational hierarchies
- Nested comment systems in social media platforms
- Fractal-based algorithms for graphics and simulation
Understanding recursive patterns can help in designing more flexible and scalable systems, a crucial skill for software engineers, especially those aiming for positions at major tech companies.
3. Machine Learning and AI
Recursive neural networks (RNNs) and their variants have been crucial in advancing natural language processing and other sequential data processing tasks. The ability to process data with a variable input size and maintain a form of memory makes these architectures powerful tools in the AI toolkit.
Moreover, the concept of recursion is fundamental to many AI algorithms, including:
- Minimax algorithm for game-playing AI
- Recursive feature elimination in machine learning
- Hierarchical clustering algorithms
Challenges and Limitations of Recursion
While recursion is a powerful concept, it’s important to understand its limitations and challenges, both in programming and in philosophical thinking.
1. Stack Overflow and Resource Limitations
In programming, deep recursive calls can lead to stack overflow errors. This limitation reminds us of the physical constraints of our computing systems and, by extension, the potential limitations of our own cognitive processes.
To mitigate this, techniques like tail recursion optimization and iterative approaches are often employed. Here’s an example of tail recursion optimization for the factorial function:
def factorial_tail_recursive(n, accumulator=1):
if n == 0 or n == 1:
return accumulator
else:
return factorial_tail_recursive(n - 1, n * accumulator)
2. Readability and Maintainability
While recursive solutions can be elegant, they can also be difficult to understand and maintain, especially for complex problems. This challenge in recursion mirrors the difficulty in comprehending deeply nested or self-referential concepts in philosophy.
3. Performance Considerations
In some cases, recursive solutions may be less efficient than their iterative counterparts. This reminds us that the most philosophically satisfying solution may not always be the most practical one.
Recursion in Learning and Education
The concept of recursion has significant implications for learning and education, particularly in the context of coding education platforms like AlgoCademy.
1. Metacognition and Learning Strategies
Understanding recursion can enhance metacognition – the awareness and understanding of one’s own thought processes. Just as a recursive function calls itself, learners can benefit from recursively examining their own learning processes, leading to more effective study strategies.
2. Problem Decomposition
Recursive thinking encourages breaking down complex problems into smaller, manageable parts. This skill is invaluable not just in coding, but in all areas of problem-solving and critical thinking.
3. Algorithmic Thinking
Mastering recursion is a key step in developing strong algorithmic thinking skills. It encourages a deeper understanding of problem structures and solution patterns, essential for success in technical interviews and software development careers.
Ethical Considerations and Societal Impact
As we delve deeper into the implications of recursion, it’s crucial to consider its ethical dimensions and potential societal impacts.
1. AI and Recursive Systems
As AI systems become more complex and potentially recursive in nature (e.g., self-improving AI), we must grapple with ethical questions about control, accountability, and the nature of intelligence itself.
2. Privacy and Data Processing
Recursive algorithms can be powerful tools for data processing and analysis. However, they also raise concerns about privacy and data exploitation, especially when dealing with nested or hierarchical personal data.
3. Cognitive Enhancement and Transhumanism
Understanding recursion and its role in consciousness could potentially lead to new approaches in cognitive enhancement. This touches upon transhumanist ideas and the ethical implications of augmenting human cognition.
Conclusion: The Recursive Nature of Understanding
As we conclude our exploration of the philosophical implications of recursion, we find ourselves in a meta-recursive situation. Our understanding of recursion deepens our ability to think recursively, which in turn enhances our grasp of the concept itself.
For aspiring programmers and computer scientists, particularly those using platforms like AlgoCademy to prepare for technical interviews at major tech companies, understanding recursion is more than just a technical skill. It’s a gateway to a deeper understanding of problem-solving, consciousness, and the nature of reality itself.
As you continue your coding journey, remember that each recursive function you write is not just a piece of code, but a small mirror reflecting the intricate, self-referential nature of existence. Embrace the recursive nature of learning, where each new understanding builds upon and refines previous knowledge.
In the end, the true power of recursion lies not just in its ability to solve complex computational problems, but in its capacity to reshape our understanding of the world and our place within it. As philosopher-coders, we stand at the intersection of technology and metaphysics, using our understanding of recursion to probe the deepest questions of existence while building the digital future.