The Importance of Consistent Hashing in Distributed Systems
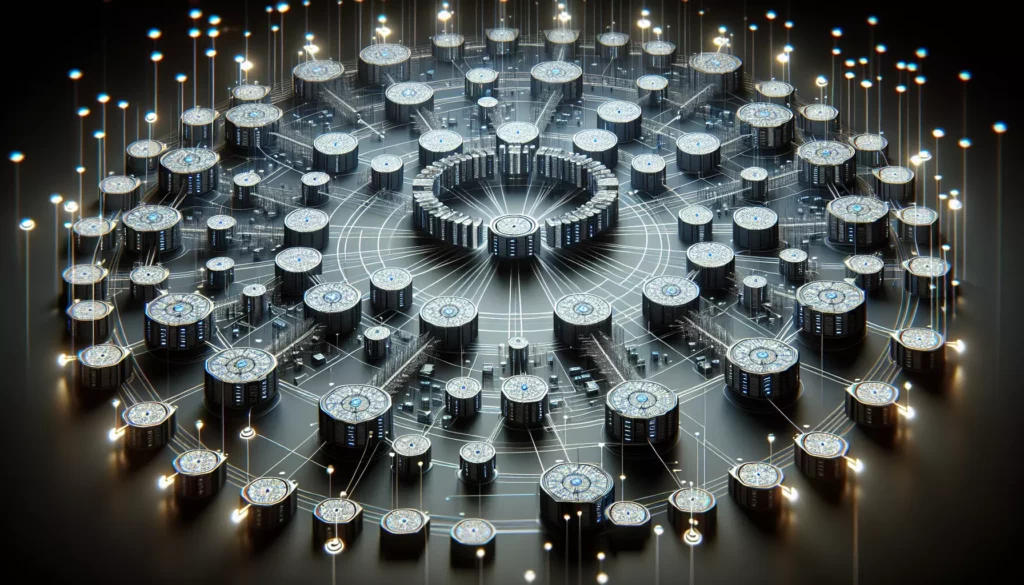
In the world of distributed systems, efficient data management and load balancing are crucial for maintaining performance and scalability. One technique that has become increasingly important in this context is consistent hashing. This powerful algorithm plays a vital role in modern distributed systems, from content delivery networks to large-scale databases. In this comprehensive guide, we’ll explore the concept of consistent hashing, its importance, and how it’s implemented in various distributed systems.
What is Consistent Hashing?
Consistent hashing is a distributed hashing scheme that provides a way to distribute data across multiple nodes in a system, while minimizing the amount of data that needs to be moved when nodes are added or removed. It was first introduced in 1997 by David Karger et al. in their paper “Consistent Hashing and Random Trees: Distributed Caching Protocols for Relieving Hot Spots on the World Wide Web”.
The primary goal of consistent hashing is to balance the load across nodes in a distributed system and ensure that when the number of nodes changes, only a small fraction of keys need to be remapped. This is in contrast to traditional hash-based data distribution methods, where a change in the number of nodes can lead to a significant redistribution of data.
How Does Consistent Hashing Work?
To understand consistent hashing, let’s break down its key components and mechanisms:
1. Hash Ring
Consistent hashing uses the concept of a hash ring. Imagine a circular ring where the values range from 0 to 2^32 – 1 (assuming a 32-bit hash function). Both the nodes in the system and the keys to be stored are mapped onto this ring using a hash function.
2. Mapping Nodes
Each node in the system is assigned a position on the ring by hashing its identifier (e.g., IP address or name). These positions are distributed around the ring.
3. Mapping Keys
When a key needs to be stored or retrieved, it is also hashed to determine its position on the ring.
4. Key Assignment
To determine which node should store a particular key, we move clockwise from the key’s position on the ring until we encounter a node. This node becomes responsible for storing the key.
5. Virtual Nodes
To improve load balancing, each physical node can be represented by multiple virtual nodes on the ring. This helps distribute the keys more evenly across the system.
Implementing Consistent Hashing
Let’s look at a basic implementation of consistent hashing in Python to illustrate the concept:
import hashlib
class ConsistentHash:
def __init__(self, nodes=None, virtual_nodes=100):
self.virtual_nodes = virtual_nodes
self.ring = {}
self.sorted_keys = []
if nodes:
for node in nodes:
self.add_node(node)
def add_node(self, node):
for i in range(self.virtual_nodes):
key = self._hash(f"{node}:{i}")
self.ring[key] = node
self.sorted_keys.append(key)
self.sorted_keys.sort()
def remove_node(self, node):
for i in range(self.virtual_nodes):
key = self._hash(f"{node}:{i}")
del self.ring[key]
self.sorted_keys.remove(key)
def get_node(self, key):
if not self.ring:
return None
hash_key = self._hash(key)
for node_key in self.sorted_keys:
if node_key >= hash_key:
return self.ring[node_key]
return self.ring[self.sorted_keys[0]]
def _hash(self, key):
return hashlib.md5(key.encode()).hexdigest()
# Usage example
nodes = ["node1", "node2", "node3"]
ch = ConsistentHash(nodes)
print(ch.get_node("key1")) # Output: node2
print(ch.get_node("key2")) # Output: node1
print(ch.get_node("key3")) # Output: node3
ch.add_node("node4")
print(ch.get_node("key1")) # Output: node2 (unchanged)
print(ch.get_node("key2")) # Output: node1 (unchanged)
print(ch.get_node("key3")) # Output: node3 (unchanged)
print(ch.get_node("key4")) # Output: node4 (new key assigned to new node)
This implementation demonstrates the basic principles of consistent hashing, including the use of virtual nodes to improve load balancing.
Importance of Consistent Hashing in Distributed Systems
Consistent hashing offers several key benefits that make it crucial for distributed systems:
1. Scalability
Consistent hashing allows distributed systems to scale horizontally with minimal disruption. When new nodes are added to the system, only a fraction of the keys need to be remapped, rather than triggering a complete redistribution of data.
2. Load Balancing
By using virtual nodes, consistent hashing can achieve a more even distribution of data across nodes, even when the nodes have different capacities or when the number of nodes changes.
3. Fault Tolerance
When a node fails or is removed from the system, only the keys mapped to that node need to be redistributed. This minimizes the impact of node failures on the overall system.
4. Reduced Data Movement
Compared to traditional hash-based distribution methods, consistent hashing significantly reduces the amount of data that needs to be moved when the topology of the system changes.
5. Predictable Key Locations
Consistent hashing provides a deterministic way to locate keys, which can improve read and write performance in distributed systems.
Applications of Consistent Hashing
Consistent hashing finds applications in various distributed systems and scenarios:
1. Distributed Caches
Systems like Memcached and Redis use consistent hashing to distribute cached data across multiple nodes. This ensures that cache hits remain high even as nodes are added or removed from the cluster.
2. Content Delivery Networks (CDNs)
CDNs use consistent hashing to determine which edge server should serve content for a particular user request. This helps in load balancing and efficient content delivery.
3. Distributed Databases
NoSQL databases like Apache Cassandra and Amazon DynamoDB use consistent hashing to distribute data across nodes in a cluster. This allows for efficient data partitioning and load balancing.
4. Load Balancers
Consistent hashing can be used in load balancers to distribute incoming requests across multiple backend servers while maintaining session affinity.
5. Distributed File Systems
Systems like HDFS (Hadoop Distributed File System) use consistent hashing to determine the placement of data blocks across multiple nodes.
Challenges and Considerations
While consistent hashing offers numerous benefits, there are some challenges and considerations to keep in mind:
1. Hotspots
Even with virtual nodes, it’s possible for certain keys to become hotspots, leading to uneven load distribution. Techniques like dynamic load balancing may be necessary to address this issue.
2. Node Heterogeneity
In systems where nodes have different capacities, additional mechanisms may be needed to ensure that more powerful nodes receive a proportionally larger share of the data.
3. Data Replication
Consistent hashing doesn’t inherently handle data replication. Additional logic is needed to ensure data is replicated across multiple nodes for fault tolerance.
4. Consistency Models
In distributed databases, consistent hashing needs to be combined with appropriate consistency models to ensure data integrity across nodes.
Advanced Techniques and Variations
As distributed systems have evolved, several advanced techniques and variations of consistent hashing have emerged:
1. Jump Consistent Hash
Introduced by Google, Jump Consistent Hash is a fast, memory-efficient algorithm that doesn’t require the maintenance of a ring data structure. It’s particularly useful for systems with a large number of buckets.
2. Rendezvous Hashing
Also known as Highest Random Weight (HRW) hashing, this technique provides a different approach to distributed hashing that can be more suitable for certain use cases.
3. Bounded Load Consistent Hashing
This variation aims to provide stronger guarantees on load balancing by ensuring that no server is assigned more than a certain fraction above the average load.
4. Consistent Hashing with Bounded Loads
Introduced by researchers at Microsoft, this technique combines consistent hashing with a mechanism to enforce an upper bound on the load of any server.
Implementing Advanced Consistent Hashing Techniques
Let’s look at an example of implementing Jump Consistent Hash in Python:
def jump_consistent_hash(key, num_buckets):
b = -1
j = 0
while j < num_buckets:
b = j
key = ((key * 2862933555777941757) + 1) & 0xffffffffffffffff
j = int((b + 1) * (float(1 << 31) / float((key >> 33) + 1)))
return b
# Usage example
print(jump_consistent_hash(123456, 10)) # Output: 5
print(jump_consistent_hash(789012, 10)) # Output: 2
print(jump_consistent_hash(345678, 10)) # Output: 0
This implementation of Jump Consistent Hash provides a fast and memory-efficient way to map keys to buckets without the need for a ring data structure.
Best Practices for Using Consistent Hashing
When implementing consistent hashing in your distributed systems, consider the following best practices:
1. Use Virtual Nodes
Implement virtual nodes to improve load balancing, especially when dealing with a small number of physical nodes.
2. Choose an Appropriate Hash Function
Select a hash function that provides a uniform distribution of keys across the hash space. Cryptographic hash functions like MD5 or SHA-1 are often good choices.
3. Monitor and Adjust
Regularly monitor the distribution of data across nodes and be prepared to adjust the number of virtual nodes or implement additional load balancing techniques if hotspots occur.
4. Consider Node Weights
If your system has nodes with different capacities, implement a weighting mechanism to ensure that more powerful nodes receive a proportionally larger share of the data.
5. Plan for Replication
Design your system to handle data replication alongside consistent hashing to ensure fault tolerance and data availability.
6. Test Thoroughly
Thoroughly test your consistent hashing implementation under various scenarios, including node additions, removals, and failures.
Future Trends in Consistent Hashing
As distributed systems continue to evolve, we can expect to see further developments in consistent hashing and related techniques:
1. Machine Learning Integration
Machine learning algorithms may be used to predict and optimize data distribution in consistent hashing systems, potentially leading to more efficient load balancing.
2. Quantum-Resistant Hashing
As quantum computing advances, there may be a need for quantum-resistant hash functions in consistent hashing implementations to ensure long-term security.
3. Edge Computing Adaptations
Consistent hashing techniques may need to be adapted for edge computing scenarios, where data locality and network latency play crucial roles.
4. Blockchain Integration
Consistent hashing could find new applications in blockchain systems for efficient data partitioning and consensus mechanisms.
Conclusion
Consistent hashing has become an indispensable technique in the world of distributed systems. Its ability to efficiently distribute data across nodes while minimizing redistribution during topology changes makes it a cornerstone of many modern distributed architectures. From content delivery networks to distributed databases, consistent hashing plays a crucial role in ensuring scalability, load balancing, and fault tolerance.
As you develop your skills in distributed systems and prepare for technical interviews, particularly for major tech companies, understanding consistent hashing and its applications is essential. It’s not just a theoretical concept but a practical tool that you’ll likely encounter and implement in real-world systems.
By mastering consistent hashing and staying abreast of its advanced techniques and future trends, you’ll be well-equipped to design and maintain robust, scalable distributed systems. Whether you’re building the next big cloud service or optimizing an existing distributed database, the principles of consistent hashing will serve as a valuable foundation for your work in the ever-evolving landscape of distributed computing.