The Archaeologist Coder: Unearthing the Ancient Origins of Modern Algorithms
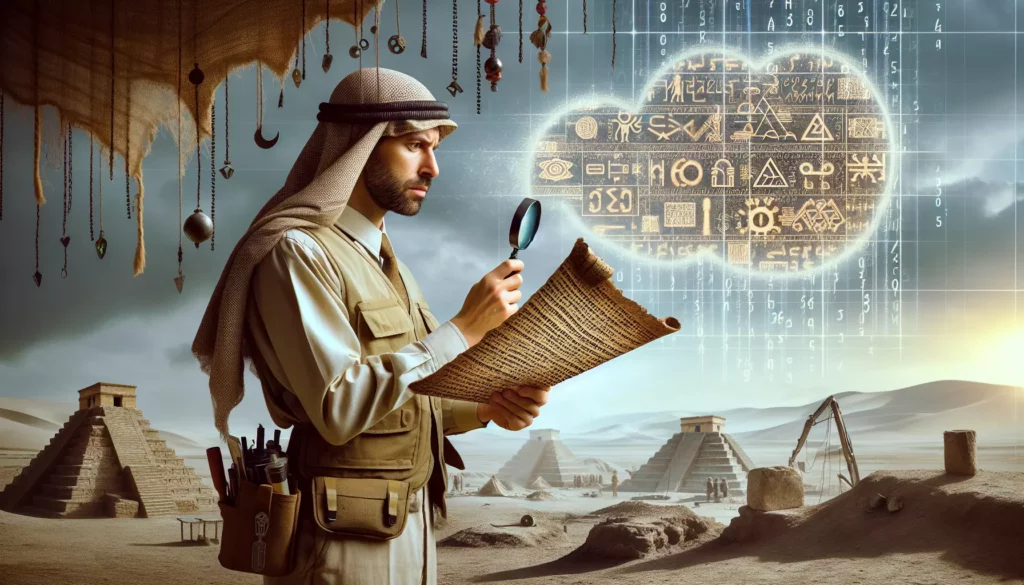
In the fast-paced world of modern technology, it’s easy to forget that many of the algorithms we use today have roots that stretch back thousands of years. Just as archaeologists unearth ancient artifacts to understand our past, we can dig into the history of algorithms to gain a deeper appreciation for the foundations of computer science. This exploration not only satisfies our curiosity but also enriches our understanding of coding principles, making us better programmers in the process.
At AlgoCademy, we believe that understanding the historical context of algorithms can significantly enhance a programmer’s ability to grasp complex concepts and develop innovative solutions. This knowledge forms an integral part of our comprehensive coding education, which aims to take learners from beginner levels all the way to mastering the skills needed for technical interviews at major tech companies.
In this extensive blog post, we’ll embark on a journey through time, exploring the ancient origins of modern algorithms. We’ll uncover how ancient civilizations laid the groundwork for computational thinking, and how these early ideas evolved into the sophisticated algorithms that power our digital world today. By the end of this article, you’ll have a newfound appreciation for the historical depth of computer science and how it can inform your coding practice.
The Babylonian Roots of Numerical Algorithms
Our journey begins in ancient Mesopotamia, where the Babylonians developed some of the earliest known numerical algorithms. Around 1800 BCE, Babylonian mathematicians were already using sophisticated methods to solve quadratic equations and calculate square roots.
The Babylonian Square Root Method
One of the most fascinating algorithms from this era is the Babylonian method for calculating square roots. This method is remarkably similar to what we now know as the Newton-Raphson method for finding roots of equations. Here’s how it works:
- Start with an initial guess for the square root of a number N.
- Improve the guess by taking the average of the guess and N divided by the guess.
- Repeat step 2 until the desired accuracy is achieved.
In modern Python, we might implement this algorithm like this:
def babylonian_sqrt(n, tolerance=0.0001):
x = n # Initial guess
while True:
next_x = 0.5 * (x + n / x)
if abs(next_x - x) < tolerance:
return next_x
x = next_x
# Example usage
print(babylonian_sqrt(2)) # Output: ~1.4142135623730951
This ancient algorithm demonstrates that iterative methods and the concept of convergence were understood thousands of years before the advent of modern computers. It’s a testament to the timelessness of good algorithmic thinking.
Euclidean Algorithm: The Granddaddy of All Algorithms
Moving forward in time to ancient Greece, we encounter what is often considered the oldest algorithm still in common use today: the Euclidean algorithm. Described by the Greek mathematician Euclid in his work “Elements” around 300 BCE, this algorithm efficiently computes the greatest common divisor (GCD) of two numbers.
How the Euclidean Algorithm Works
The Euclidean algorithm is based on a simple principle: the greatest common divisor of two numbers doesn’t change if the smaller number is subtracted from the larger number. By repeatedly applying this principle, we can reduce the problem to finding the GCD of smaller and smaller pairs of numbers until we reach a pair where one number divides the other evenly.
Here’s a modern implementation of the Euclidean algorithm in Python:
def euclidean_gcd(a, b):
while b != 0:
a, b = b, a % b
return a
# Example usage
print(euclidean_gcd(48, 18)) # Output: 6
The elegance and efficiency of this algorithm have stood the test of time. It’s still used today in various applications, from cryptography to computer graphics. Understanding the Euclidean algorithm provides insight into the principles of recursion and the power of reducing complex problems to simpler ones—concepts that are fundamental to modern algorithm design.
The Sieve of Eratosthenes: Ancient Prime Number Generation
Another gem from ancient Greece is the Sieve of Eratosthenes, an efficient algorithm for finding all prime numbers up to a given limit. Developed by Eratosthenes of Cyrene around 240 BCE, this algorithm demonstrates early understanding of optimization and the power of elimination in problem-solving.
How the Sieve Works
The Sieve of Eratosthenes works by iteratively marking the multiples of each prime number as composite (not prime), starting from 2. The numbers that remain unmarked are prime. Here’s a step-by-step process:
- Create a list of consecutive integers from 2 to the given limit, n.
- Start with the smallest number in the list (2).
- Mark all multiples of this number up to n as composite.
- Find the next unmarked number in the list and repeat step 3.
- Repeat until you’ve processed all numbers up to √n.
Let’s implement this ancient algorithm in Python:
def sieve_of_eratosthenes(n):
primes = [True] * (n + 1)
primes[0] = primes[1] = False
for i in range(2, int(n**0.5) + 1):
if primes[i]:
for j in range(i*i, n+1, i):
primes[j] = False
return [i for i in range(n+1) if primes[i]]
# Example usage
print(sieve_of_eratosthenes(30))
# Output: [2, 3, 5, 7, 11, 13, 17, 19, 23, 29]
This algorithm is remarkably efficient for its age and demonstrates sophisticated concepts like space-time tradeoffs and the importance of preprocessing in algorithm design. These are ideas that continue to be crucial in modern computer science and are emphasized in AlgoCademy’s curriculum.
Ancient Chinese Algorithms: The Rod Calculus
While the Western world was developing its mathematical traditions, ancient China was nurturing its own rich algorithmic heritage. One of the most significant contributions from this tradition is the rod calculus, a system of arithmetic using counting rods that led to the development of efficient algorithms for multiplication and division.
The Chinese Remainder Theorem
A particularly notable algorithm that emerged from the Chinese mathematical tradition is the Chinese Remainder Theorem (CRT). This theorem, which appears in the writings of Sun Tzu (not to be confused with the military strategist) around 100 CE, provides a solution to a system of simultaneous linear congruences with coprime moduli.
The CRT has numerous applications in modern computer science, including cryptography, parallel computation, and error correction in digital communication. Here’s a simple implementation of the Chinese Remainder Theorem in Python:
def chinese_remainder_theorem(n, a):
total = 0
product = math.prod(n)
for n_i, a_i in zip(n, a):
p = product // n_i
total += a_i * mod_inverse(p, n_i) * p
return total % product
def mod_inverse(a, m):
def egcd(a, b):
if a == 0:
return b, 0, 1
else:
g, y, x = egcd(b % a, a)
return g, x - (b // a) * y, y
g, x, _ = egcd(a, m)
if g != 1:
raise Exception('Modular inverse does not exist')
else:
return x % m
# Example usage
n = [3, 5, 7]
a = [2, 3, 2]
print(chinese_remainder_theorem(n, a)) # Output: 23
This algorithm demonstrates the power of modular arithmetic and how ancient mathematicians were able to solve complex problems with elegant solutions. The concepts behind the Chinese Remainder Theorem continue to be relevant in modern computer science, particularly in areas like distributed computing and cryptography.
The Islamic Golden Age: Algebra and Algorithms
As we move into the medieval period, we encounter the Islamic Golden Age, a time of great mathematical and scientific advancement. The very word “algorithm” comes from the name of the Persian mathematician Al-Khwarizmi, who lived in the 9th century CE.
Al-Khwarizmi’s Contribution to Algebra
Al-Khwarizmi’s most famous work, “The Compendious Book on Calculation by Completion and Balancing,” introduced systematic solutions for linear and quadratic equations. His approach laid the foundation for algebra as we know it today and introduced step-by-step problem-solving methods that are at the heart of algorithmic thinking.
One of Al-Khwarizmi’s methods for solving quadratic equations can be seen as an early precursor to modern root-finding algorithms. Here’s a Python implementation of a simple quadratic equation solver inspired by Al-Khwarizmi’s work:
import math
def solve_quadratic(a, b, c):
discriminant = b**2 - 4*a*c
if discriminant < 0:
return None
elif discriminant == 0:
return -b / (2*a)
else:
return ((-b + math.sqrt(discriminant)) / (2*a),
(-b - math.sqrt(discriminant)) / (2*a))
# Example usage
print(solve_quadratic(1, 5, 6)) # Output: (-2.0, -3.0)
This method, while simple by today’s standards, represents a significant step in the development of algorithmic problem-solving. It shows how mathematical procedures can be systematized into repeatable steps—a core principle of algorithm design.
The Renaissance and Beyond: Algorithms in the Modern Era
As we move into the Renaissance and the early modern period, we see a rapid acceleration in the development of mathematical and algorithmic thinking. This era saw the birth of many algorithms that are still in use today.
Newton’s Method: Iterative Approximation
One of the most important algorithms to emerge from this period is Newton’s method for finding roots of equations. Developed by Isaac Newton in the late 17th century, this method is a powerful tool for numerical analysis and is still widely used in various fields of science and engineering.
Newton’s method is an iterative algorithm that uses the derivative of a function to find better approximations of its roots. Here’s a Python implementation:
def newton_method(f, df, x0, epsilon=1e-7, max_iter=100):
x = x0
for _ in range(max_iter):
fx = f(x)
if abs(fx) < epsilon:
return x
dfx = df(x)
if dfx == 0:
return None # Derivative is zero, method fails
x = x - fx / dfx
return None # Max iterations reached, method fails
# Example: Finding the square root of 2
f = lambda x: x**2 - 2
df = lambda x: 2*x
result = newton_method(f, df, 1)
print(f"Square root of 2: {result}")
# Output: Square root of 2: 1.4142135623730951
Newton’s method demonstrates the power of iterative refinement, a concept that is central to many modern optimization algorithms. It also showcases the importance of calculus in algorithm design, a theme that continues to be relevant in advanced areas of computer science like machine learning and computer graphics.
The Birth of Modern Computer Science
As we approach the 20th century, we begin to see the emergence of what we now recognize as modern computer science. This era saw the development of formal logic, the concept of computability, and the first mechanical and electronic computers.
Alan Turing and the Foundations of Computation
No discussion of the history of algorithms would be complete without mentioning Alan Turing. His work in the 1930s on the concept of a “Universal Turing Machine” laid the theoretical foundation for all modern computing. Turing’s ideas about computability and the nature of algorithms continue to influence computer science to this day.
One of Turing’s most famous contributions is the concept of the Turing machine, a theoretical model of computation. While we can’t implement a true Turing machine in Python (as it requires infinite memory), we can create a simplified version to illustrate the concept:
class SimpleTuringMachine:
def __init__(self, tape, initial_state, final_states, transitions):
self.tape = list(tape)
self.head = 0
self.state = initial_state
self.final_states = final_states
self.transitions = transitions
def step(self):
if self.state in self.final_states:
return False
current_symbol = self.tape[self.head]
if (self.state, current_symbol) not in self.transitions:
return False
new_state, new_symbol, direction = self.transitions[(self.state, current_symbol)]
self.tape[self.head] = new_symbol
self.state = new_state
self.head += 1 if direction == 'R' else -1
return True
def run(self):
while self.step():
pass
return ''.join(self.tape)
# Example: A Turing machine that replaces '0's with '1's
tm = SimpleTuringMachine(
tape='00011010',
initial_state='q0',
final_states={'qf'},
transitions={
('q0', '0'): ('q0', '1', 'R'),
('q0', '1'): ('q0', '1', 'R'),
('q0', ''): ('qf', '', 'R')
}
)
result = tm.run()
print(f"Result: {result}")
# Output: Result: 11111111
This simple Turing machine demonstrates the core ideas of state-based computation and the manipulation of symbols on a tape. These concepts are fundamental to our understanding of what can be computed and how, forming the basis of algorithmic complexity theory and the design of programming languages.
The Digital Age: Algorithms in the Computer Era
With the advent of electronic computers in the mid-20th century, the development and application of algorithms exploded. This era saw the birth of many classic algorithms that are now staples of computer science education.
Sorting Algorithms: From Bubble Sort to QuickSort
Sorting algorithms are among the most studied in computer science, and their development illustrates the evolution of algorithmic thinking in the digital age. Let’s look at two sorting algorithms: the simple but inefficient Bubble Sort, and the more sophisticated QuickSort.
Bubble Sort, while not efficient for large datasets, is easy to understand and implement:
def bubble_sort(arr):
n = len(arr)
for i in range(n):
for j in range(0, n - i - 1):
if arr[j] > arr[j + 1]:
arr[j], arr[j + 1] = arr[j + 1], arr[j]
return arr
# Example usage
print(bubble_sort([64, 34, 25, 12, 22, 11, 90]))
# Output: [11, 12, 22, 25, 34, 64, 90]
In contrast, QuickSort, developed by Tony Hoare in 1959, uses a divide-and-conquer approach to achieve much better average-case performance:
def quicksort(arr):
if len(arr) <= 1:
return arr
pivot = arr[len(arr) // 2]
left = [x for x in arr if x < pivot]
middle = [x for x in arr if x == pivot]
right = [x for x in arr if x > pivot]
return quicksort(left) + middle + quicksort(right)
# Example usage
print(quicksort([64, 34, 25, 12, 22, 11, 90]))
# Output: [11, 12, 22, 25, 34, 64, 90]
The contrast between these two algorithms illustrates how algorithmic thinking evolved to handle larger datasets more efficiently, a crucial development as the amount of data processed by computers grew exponentially.
Modern Algorithmic Breakthroughs
In recent decades, we’ve seen remarkable advances in algorithmic design, often driven by the needs of emerging technologies. Let’s look at a few examples of modern algorithmic breakthroughs.
PageRank: The Algorithm that Powered Google
Developed by Larry Page and Sergey Brin in 1996, PageRank revolutionized web search by providing a way to rank web pages based on their importance. Here’s a simplified implementation of the PageRank algorithm:
import numpy as np
def pagerank(graph, damping=0.85, epsilon=1e-8):
n = len(graph)
# Create adjacency matrix
A = np.array([[1 if j in graph[i] else 0 for j in range(n)] for i in range(n)])
# Normalize adjacency matrix
out_degree = np.sum(A, axis=1)
A_hat = (A / out_degree[:, np.newaxis]).T
# Initialize PageRank vector
pr = np.ones(n) / n
while True:
pr_next = (1 - damping) / n + damping * A_hat @ pr
if np.sum(np.abs(pr_next - pr)) < epsilon:
return pr_next
pr = pr_next
# Example usage
graph = {0: [1, 2], 1: [2], 2: [0, 1]}
result = pagerank(graph)
print(f"PageRank: {result}")
# Output: PageRank: [0.38461538 0.30769231 0.30769231]
PageRank demonstrates how graph theory and linear algebra can be combined to solve real-world problems, a theme that continues in many modern algorithms.
Machine Learning Algorithms: Neural Networks and Beyond
The field of machine learning has produced some of the most impactful algorithms of the 21st century. While a full implementation of a neural network is beyond the scope of this article, we can look at a simple example of a machine learning algorithm: linear regression using gradient descent.
import numpy as np
def linear_regression(X, y, learning_rate=0.01, iterations=1000):
m, n = X.shape
theta = np.zeros(n)
for _ in range(iterations):
h = np.dot(X, theta)
gradient = np.dot(X.T, (h - y)) / m
theta -= learning_rate * gradient
return theta
# Example usage
X = np.array([[1, 1], [1, 2], [1, 3]])
y = np.array([1, 2, 3])
theta = linear_regression(X, y)
print(f"Theta: {theta}")
# Output: Theta: [0. 1.]
This simple example illustrates key concepts in machine learning, such as iterative optimization and the use of gradients to guide learning. These ideas form the foundation for more complex algorithms used in deep learning and artificial intelligence.
The Future of Algorithms
As we look to the future, the field of algorithm design continues to evolve rapidly. Emerging areas like quantum computing promise to revolutionize our approach to certain classes of problems, while the growing importance of AI and machine learning is driving the development of new types of algorithms.
Quantum Algorithms: A New Frontier
Quantum algorithms represent a fundamentally different approach to computation, leveraging the principles of quantum mechanics to solve certain problems exponentially faster than classical algorithms. While we can’t run true quantum algorithms on classical computers, we can simulate some aspects of quantum computation. Here’s a simple example of a quantum coin flip simulation:
import numpy as np
def quantum_coin_flip():
# Create a superposition state
state = np.array([1/np.sqrt(2), 1/np.sqrt(2)])
# Measure the state
measurement = np.random.choice(['Heads', 'Tails'], p=state**2)
return measurement
# Example usage
results = [quantum_coin_flip() for _ in range(1000)]
heads_count = results.count('Heads')
tails_count = results.count('Tails')
print(f"Heads: {heads_count}, Tails: {tails_count}")
# Output will be approximately: Heads: 500, Tails: 500
While this is a very simple example, it illustrates the probabilistic nature of quantum computation and how it differs from classical deterministic algorithms.
Conclusion: The Timeless Nature of Algorithmic Thinking
As we’ve seen in this journey through the history of algorithms, from ancient Babylonian mathematics to quantum computing, the fundamental principles of algorithmic thinking have remained remarkably consistent. The ability to break down complex problems into step-by-step procedures, to optimize for efficiency, and to leverage mathematical principles to solve real-world problems are skills that have been valuable for millennia and will continue to be crucial in the future.
At AlgoCademy, we believe that understanding this historical context enriches our approach to coding education. By studying the evolution of algorithms, we gain insights that can inform our problem-solving strategies and deepen our appreciation for the elegance of well-designed algorithms.
As you continue your journey in coding and computer science, we encourage you to keep this historical perspective in mind. Remember that every line of code you write is part of a tradition that stretches back thousands of years. By understanding where we’ve come from, we’re better equipped to innovate and push the boundaries of what’s possible in the world of algorithms and computation.
Whether you’re just starting out in coding or preparing for technical interviews at top tech companies, the ability to think algorithmically is a skill that will serve you well. At AlgoCademy, we’re committed to helping you develop this skill, providing you with the tools, resources, and guidance you need to excel in the ever-evolving world of computer science.
So, the next time you’re tackling a complex coding problem or designing a new algorithm, take a moment to appreciate the rich history behind your work. You’re not just a coder; you’re part of a long line of algorithmic thinkers stretching back to the dawn of civilization. Embrace this legacy, learn from it, and use it to fuel your own innovations in the exciting world of algorithms and computer science.