Cooking Up Code: Learning Algorithms Through Culinary Analogies
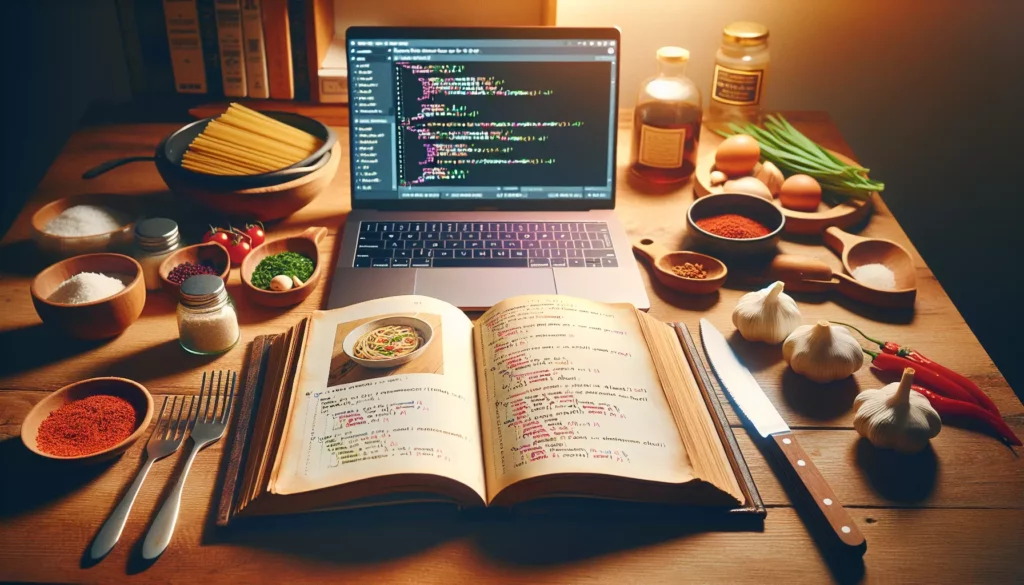
In the world of programming, algorithms are the secret ingredients that make our digital creations come to life. Much like a chef crafting a delectable dish, a programmer weaves together lines of code to create something functional and elegant. At AlgoCademy, we believe that learning algorithms doesn’t have to be a dry, abstract process. Instead, we can spice things up by drawing parallels between coding and cooking. In this post, we’ll explore how culinary analogies can help demystify complex algorithmic concepts and make your journey from coding novice to interview-ready professional more palatable.
The Kitchen as Your Coding Environment
Before we dive into specific algorithms, let’s set the stage by comparing your coding environment to a well-equipped kitchen. Just as a chef needs the right tools and ingredients to create culinary masterpieces, a programmer requires the proper setup to craft efficient code.
Essential Tools
- Cutting Board (IDE): Your Integrated Development Environment is like a cutting board, providing a clean, organized space to prepare your code ingredients.
- Knives (Text Editor): A good text editor is as crucial as a sharp knife, allowing you to slice and dice your code with precision.
- Pots and Pans (Compilers/Interpreters): These are the vessels that transform your raw code into executable programs, much like pots and pans turn raw ingredients into finished dishes.
- Measuring Cups (Debugger): A debugger helps you measure and adjust your code, ensuring everything is in the right proportion.
Ingredients (Data Structures)
In cooking, ingredients are the building blocks of any recipe. In programming, data structures serve a similar purpose:
- Arrays (Spice Rack): Organized, indexed collections of similar items.
- Linked Lists (Ingredient Chain): A series of connected elements, each pointing to the next.
- Stacks (Pancake Stack): Last-in, first-out structure, perfect for tracking function calls or undo operations.
- Queues (Buffet Line): First-in, first-out structure, ideal for managing tasks or requests in order.
- Trees (Family Recipe Book): Hierarchical structure with branches, great for representing relationships.
- Hash Tables (Spice Cabinet): Quick access to items based on unique keys.
Now that we’ve set up our kitchen, let’s start cooking up some algorithmic dishes!
Appetizers: Simple Sorting Algorithms
Just as appetizers prepare your palate for the main course, simple sorting algorithms lay the groundwork for more complex computational concepts. Let’s explore a few sorting methods through culinary lenses.
Bubble Sort: The Rising Soufflé
Imagine you’re making a cheese soufflé. As it bakes, the air bubbles rise to the top, carrying lighter ingredients with them. This is similar to how Bubble Sort works:
- Start at the bottom of your unsorted list.
- Compare adjacent elements, swapping them if they’re in the wrong order.
- Repeat this process, allowing the “bubbles” (larger elements) to rise to the top.
- Continue until no more swaps are needed.
Here’s a simple implementation of Bubble Sort in Python:
def bubble_sort(arr):
n = len(arr)
for i in range(n):
for j in range(0, n-i-1):
if arr[j] > arr[j+1]:
arr[j], arr[j+1] = arr[j+1], arr[j]
return arr
# Example usage
unsorted_list = [64, 34, 25, 12, 22, 11, 90]
sorted_list = bubble_sort(unsorted_list)
print(sorted_list)
While Bubble Sort is easy to understand, it’s not the most efficient for large datasets. It’s like hand-whipping cream instead of using an electric mixer – it gets the job done, but there are faster methods available.
Selection Sort: Plating a Salad
Picture yourself assembling a colorful salad. You scan your ingredients, select the smallest vegetable, and place it on the plate. Then you repeat the process until all vegetables are arranged. This is essentially how Selection Sort operates:
- Find the smallest element in the unsorted portion of the list.
- Swap it with the first unsorted element.
- Move the boundary between sorted and unsorted sections one element to the right.
- Repeat until the entire list is sorted.
Here’s a Python implementation of Selection Sort:
def selection_sort(arr):
n = len(arr)
for i in range(n):
min_idx = i
for j in range(i+1, n):
if arr[j] < arr[min_idx]:
min_idx = j
arr[i], arr[min_idx] = arr[min_idx], arr[i]
return arr
# Example usage
unsorted_list = [64, 25, 12, 22, 11]
sorted_list = selection_sort(unsorted_list)
print(sorted_list)
Selection Sort, like carefully plating a salad, ensures that each element finds its proper place. However, for large datasets, more efficient methods are preferred.
Main Course: Divide and Conquer Algorithms
As we move to more complex algorithms, let’s explore the “divide and conquer” strategy. In cooking, this might be likened to preparing a multi-course meal, where tasks are divided among kitchen staff to increase efficiency.
Merge Sort: The Buffet Line Organizer
Imagine you’re managing a large buffet. To serve guests efficiently, you divide them into smaller groups, organize each group, and then merge them back into a single, orderly line. This is the essence of Merge Sort:
- Divide the unsorted list into n sublists, each containing one element.
- Repeatedly merge sublists to produce new sorted sublists until there is only one sublist remaining.
Here’s how you might implement Merge Sort in Python:
def merge_sort(arr):
if len(arr) > 1:
mid = len(arr) // 2
L = arr[:mid]
R = arr[mid:]
merge_sort(L)
merge_sort(R)
i = j = k = 0
while i < len(L) and j < len(R):
if L[i] < R[j]:
arr[k] = L[i]
i += 1
else:
arr[k] = R[j]
j += 1
k += 1
while i < len(L):
arr[k] = L[i]
i += 1
k += 1
while j < len(R):
arr[k] = R[j]
j += 1
k += 1
return arr
# Example usage
unsorted_list = [38, 27, 43, 3, 9, 82, 10]
sorted_list = merge_sort(unsorted_list)
print(sorted_list)
Merge Sort is like an efficient buffet line organizer, handling large groups with ease and ensuring everyone gets their food in order.
Quick Sort: The Mise en Place Master
In professional kitchens, chefs use a technique called “mise en place,” which means “everything in its place.” This involves organizing and arranging ingredients before cooking begins. Quick Sort follows a similar principle:
- Choose a ‘pivot’ element from the array.
- Partition the other elements into two sub-arrays, according to whether they are less than or greater than the pivot.
- Recursively sort the sub-arrays.
Here’s a Python implementation of Quick Sort:
def quick_sort(arr):
if len(arr) <= 1:
return arr
else:
pivot = arr[len(arr) // 2]
left = [x for x in arr if x < pivot]
middle = [x for x in arr if x == pivot]
right = [x for x in arr if x > pivot]
return quick_sort(left) + middle + quick_sort(right)
# Example usage
unsorted_list = [3, 6, 8, 10, 1, 2, 1]
sorted_list = quick_sort(unsorted_list)
print(sorted_list)
Quick Sort, like a chef with excellent mise en place, efficiently organizes elements around a pivot, leading to a well-sorted result.
Side Dish: Search Algorithms
No meal is complete without side dishes, and no discussion of algorithms is complete without touching on search algorithms. Let’s explore a couple through our culinary lens.
Linear Search: The Pantry Scan
Imagine you’re looking for a specific spice in an unorganized pantry. You’d likely scan each item one by one until you find what you need. This is essentially how Linear Search works:
- Start at the beginning of the list.
- Compare each element with the target value.
- If a match is found, return the index.
- If the end of the list is reached without finding a match, return -1 or a “not found” message.
Here’s a simple implementation of Linear Search in Python:
def linear_search(arr, target):
for i in range(len(arr)):
if arr[i] == target:
return i
return -1
# Example usage
spice_rack = ["Salt", "Pepper", "Cumin", "Paprika", "Turmeric"]
target_spice = "Cumin"
result = linear_search(spice_rack, target_spice)
print(f"{target_spice} is at index {result}")
Linear Search is straightforward but can be time-consuming for large datasets, much like searching through a disorganized pantry.
Binary Search: The Cookbook Index
Now, imagine you’re looking up a recipe in a well-organized cookbook with an alphabetical index. You’d start in the middle, determine if your recipe comes before or after, and then repeat the process in the correct half. This is how Binary Search operates:
- Start with the middle element of the sorted list.
- If the target value is equal to the middle element, we’re done.
- If the target value is less than the middle element, repeat the search on the left half.
- If the target value is greater than the middle element, repeat the search on the right half.
Here’s a Python implementation of Binary Search:
def binary_search(arr, target):
left = 0
right = len(arr) - 1
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1
# Example usage
sorted_recipes = ["Apple Pie", "Beef Stew", "Chicken Curry", "Pasta Carbonara", "Zucchini Bread"]
target_recipe = "Chicken Curry"
result = binary_search(sorted_recipes, target_recipe)
print(f"{target_recipe} is at index {result}")
Binary Search, like using a well-organized cookbook index, is extremely efficient for large, sorted datasets.
Dessert: Dynamic Programming
As we reach the sweet conclusion of our meal, let’s indulge in the concept of Dynamic Programming. In the culinary world, this might be compared to creating a complex dessert with multiple components that can be prepared independently and combined in various ways.
Fibonacci Sequence: The Layer Cake
Consider building a multi-layered cake where each layer’s size depends on the two layers beneath it. This is similar to how the Fibonacci sequence works, where each number is the sum of the two preceding ones. Dynamic Programming can optimize this process:
def fibonacci(n):
if n <= 1:
return n
fib = [0] * (n + 1)
fib[1] = 1
for i in range(2, n + 1):
fib[i] = fib[i-1] + fib[i-2]
return fib[n]
# Example usage
n = 10
result = fibonacci(n)
print(f"The {n}th Fibonacci number is {result}")
This approach, like preparing cake layers in advance, saves time by storing and reusing previously calculated values.
Knapsack Problem: The Picnic Basket Optimizer
Imagine you’re packing a picnic basket with a weight limit. You want to maximize the total value of items you can carry. This is the essence of the Knapsack Problem, which can be solved efficiently using Dynamic Programming:
def knapsack(W, wt, val, n):
K = [[0 for x in range(W + 1)] for x in range(n + 1)]
for i in range(n + 1):
for w in range(W + 1):
if i == 0 or w == 0:
K[i][w] = 0
elif wt[i-1] <= w:
K[i][w] = max(val[i-1] + K[i-1][w-wt[i-1]], K[i-1][w])
else:
K[i][w] = K[i-1][w]
return K[n][W]
# Example usage
val = [60, 100, 120] # values of items
wt = [10, 20, 30] # weights of items
W = 50 # maximum weight capacity
n = len(val)
result = knapsack(W, wt, val, n)
print(f"Maximum value that can be carried: {result}")
This algorithm, like an expert picnic packer, efficiently determines the optimal combination of items to maximize value within the given constraints.
The Recipe for Success: Practice and Application
As with any skill, mastering algorithms requires practice and real-world application. At AlgoCademy, we believe in learning by doing. Here are some tips to help you on your journey:
- Start Small: Begin with simple algorithms and gradually work your way up to more complex ones.
- Visualize: Use diagrams or animations to understand how algorithms work step-by-step.
- Analyze: Study the time and space complexity of different algorithms to understand their efficiency.
- Implement: Write code for these algorithms in your preferred programming language.
- Solve Problems: Apply these algorithms to solve real-world coding challenges and interview questions.
- Experiment: Try modifying algorithms or combining them to solve more complex problems.
Remember, just as a chef doesn’t become a master overnight, becoming proficient in algorithms takes time and dedication. But with each problem you solve, you’re adding another recipe to your coding cookbook.
Conclusion: From Kitchen to Coding, and Beyond
As we’ve seen, the world of algorithms shares many parallels with the culinary arts. Both require creativity, precision, and a deep understanding of how different elements work together. By viewing algorithms through this culinary lens, we hope to have made these concepts more accessible and engaging.
At AlgoCademy, we’re committed to helping you develop your algorithmic thinking and problem-solving skills. Whether you’re a beginner just starting out or preparing for technical interviews at top tech companies, our platform provides the ingredients and recipes you need to succeed.
So, the next time you’re faced with a coding challenge, remember: you’re not just writing code, you’re crafting a digital delicacy. Bon appétit and happy coding!