How to Design a Real-Time Chat Application in a System Design Interview
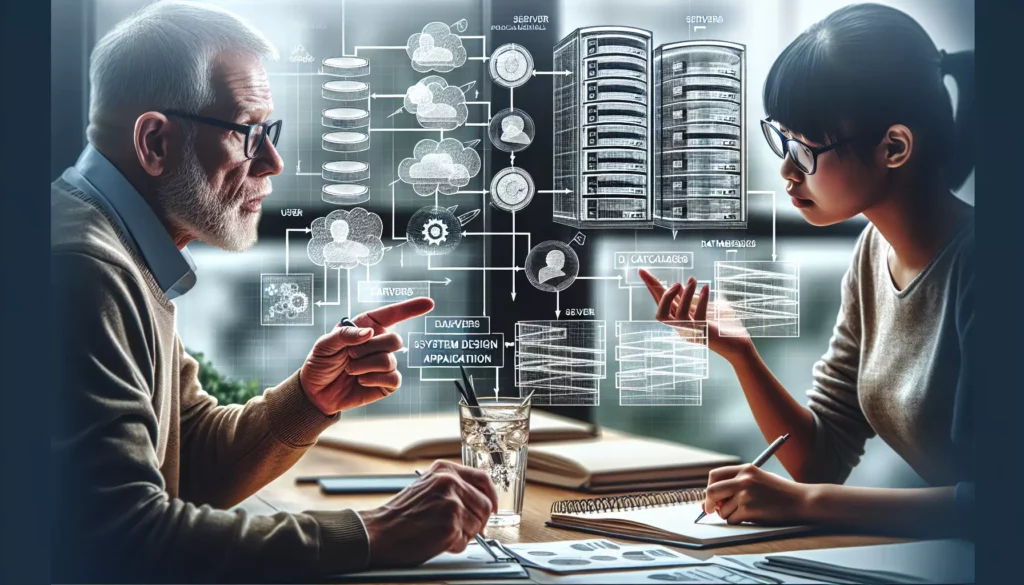
System design interviews are a crucial part of the hiring process for software engineering positions, especially at major tech companies. One common scenario that candidates might encounter is designing a real-time chat application. This task tests your ability to architect scalable, efficient, and robust systems. In this comprehensive guide, we’ll walk through the process of designing a real-time chat application, covering all the essential components and considerations you should address in a system design interview.
1. Understanding the Requirements
Before diving into the design, it’s crucial to clarify the requirements and constraints of the system. Here are some questions you should ask the interviewer:
- What is the scale of the system? (e.g., number of users, messages per day)
- What features are required? (e.g., one-on-one chats, group chats, file sharing)
- Are there any specific performance requirements? (e.g., message delivery latency)
- What are the supported platforms? (e.g., web, mobile, desktop)
- Are there any security or privacy considerations?
- Is offline message support required?
For this example, let’s assume we’re designing a system that supports:
- 100 million daily active users
- One-on-one and group chats
- Text messages and file sharing (images, documents)
- Web and mobile platforms
- Message delivery latency of less than 100ms
- Offline message support
2. High-Level System Design
With the requirements in mind, let’s outline a high-level architecture for our real-time chat application:
2.1. Client-Side Components
- Web application (React, Angular, or Vue.js)
- Mobile applications (iOS and Android)
- Desktop applications (optional)
2.2. Server-Side Components
- Load Balancer
- Chat Servers
- Authentication Service
- User Service
- Message Service
- Notification Service
- File Storage Service
- Database (for user data, chat history)
- Cache (for frequently accessed data)
- Message Queue
3. Detailed Component Design
3.1. Client-Side Design
The client-side applications should handle:
- User interface for chat conversations
- Real-time message sending and receiving
- Local caching of recent messages
- File upload and download
- Offline support and message synchronization
For real-time communication, we’ll use WebSockets, which provide full-duplex communication channels over a single TCP connection. This allows for efficient, low-latency message delivery.
3.2. Load Balancer
The load balancer distributes incoming traffic across multiple chat servers to ensure high availability and optimal resource utilization. We can use a Layer 7 (application layer) load balancer like NGINX or HAProxy, which can make routing decisions based on the content of the request.
3.3. Chat Servers
Chat servers handle WebSocket connections from clients and manage real-time message delivery. They should be stateless to allow for easy scaling. We can use Node.js with the Socket.IO library for implementing WebSocket servers.
3.4. Authentication Service
This service handles user authentication and authorization. It can use JSON Web Tokens (JWT) for secure, stateless authentication. The authentication flow would be:
- User logs in with credentials
- Authentication service verifies credentials and generates a JWT
- Client stores the JWT and includes it in subsequent requests
- Chat servers validate the JWT for each WebSocket connection and message
3.5. User Service
The User Service manages user profiles, friend lists, and online status. It can be implemented as a RESTful API using a framework like Express.js (Node.js) or Django (Python).
3.6. Message Service
This service is responsible for persisting messages and retrieving chat history. It should handle:
- Storing new messages in the database
- Retrieving chat history for users
- Message pagination for efficient loading of large conversations
3.7. Notification Service
The Notification Service handles push notifications for offline users or users with closed applications. It can integrate with platforms like Firebase Cloud Messaging (FCM) for Android and Apple Push Notification Service (APNS) for iOS.
3.8. File Storage Service
For handling file uploads and downloads, we can use a cloud storage solution like Amazon S3 or Google Cloud Storage. This service should:
- Generate pre-signed URLs for secure file uploads
- Store file metadata (e.g., file name, size, type) in the database
- Provide secure download links for shared files
3.9. Database
For our chat application, we’ll use a combination of relational and NoSQL databases:
- Relational Database (e.g., PostgreSQL): For storing user profiles, friend relationships, and other structured data.
- NoSQL Database (e.g., Cassandra or MongoDB): For storing chat messages, which can handle high write throughput and horizontal scaling.
Here’s a simplified schema for the relational database:
CREATE TABLE users (
id SERIAL PRIMARY KEY,
username VARCHAR(50) UNIQUE NOT NULL,
email VARCHAR(100) UNIQUE NOT NULL,
password_hash VARCHAR(255) NOT NULL,
created_at TIMESTAMP DEFAULT CURRENT_TIMESTAMP
);
CREATE TABLE friendships (
id SERIAL PRIMARY KEY,
user_id INTEGER REFERENCES users(id),
friend_id INTEGER REFERENCES users(id),
status VARCHAR(20) NOT NULL,
created_at TIMESTAMP DEFAULT CURRENT_TIMESTAMP,
UNIQUE(user_id, friend_id)
);
CREATE TABLE chat_rooms (
id SERIAL PRIMARY KEY,
name VARCHAR(100),
type VARCHAR(20) NOT NULL,
created_at TIMESTAMP DEFAULT CURRENT_TIMESTAMP
);
CREATE TABLE chat_room_members (
id SERIAL PRIMARY KEY,
chat_room_id INTEGER REFERENCES chat_rooms(id),
user_id INTEGER REFERENCES users(id),
joined_at TIMESTAMP DEFAULT CURRENT_TIMESTAMP,
UNIQUE(chat_room_id, user_id)
);
For the NoSQL database storing messages, we can use a schema like this:
{
"message_id": "uuid",
"chat_room_id": "integer",
"sender_id": "integer",
"content": "string",
"timestamp": "datetime",
"type": "string",
"file_metadata": {
"file_name": "string",
"file_size": "integer",
"file_type": "string",
"file_url": "string"
}
}
3.10. Cache
To improve performance and reduce database load, we’ll use a distributed cache like Redis. The cache can store:
- User sessions
- Recent messages for active conversations
- User online status
3.11. Message Queue
A message queue like Apache Kafka or RabbitMQ can be used to handle asynchronous tasks and ensure reliable message delivery. It can be used for:
- Buffering messages for offline users
- Handling push notifications
- Processing file uploads
4. Data Flow
Let’s walk through the data flow for sending and receiving messages:
4.1. Sending a Message
- User A sends a message through the client application.
- The message is sent to a chat server via WebSocket.
- The chat server validates the user’s JWT and checks permissions.
- The message is published to a Kafka topic for processing.
- The Message Service consumes the message from Kafka and stores it in the database.
- If the recipient (User B) is online, the message is sent to their WebSocket connection.
- If User B is offline, the message is queued for delivery when they come online.
- The Notification Service sends a push notification to User B’s device.
4.2. Receiving a Message
- User B’s client receives the message via WebSocket or retrieves it upon reconnection.
- The client updates the UI to display the new message.
- The client sends a delivery receipt to the server.
- The server updates the message status in the database.
5. Scalability and Performance Optimizations
To ensure our chat application can handle 100 million daily active users and maintain low latency, we need to implement several optimizations:
5.1. Horizontal Scaling
All components of our system should be designed for horizontal scaling:
- Chat servers can be scaled out behind the load balancer.
- Database sharding can be implemented to distribute data across multiple nodes.
- Cache clusters can be expanded to handle increased load.
5.2. Message Fanout
For group chats, we can implement a message fanout system:
- When a message is sent to a group, it’s published to a fanout exchange in RabbitMQ.
- Each online group member has a queue bound to this exchange.
- The message is automatically copied to all bound queues.
- Chat servers consume messages from these queues and send them to the respective WebSocket connections.
This approach reduces the load on chat servers and ensures efficient message delivery to large groups.
5.3. Caching Strategy
Implement a multi-level caching strategy:
- Client-side cache: Store recent messages and user data locally.
- CDN: Use a Content Delivery Network to cache static assets and file downloads.
- Server-side cache: Use Redis to cache frequently accessed data, such as user profiles and active conversations.
5.4. Database Optimizations
- Implement database indexing for frequently queried fields.
- Use database connection pooling to reduce connection overhead.
- Implement read replicas for the relational database to offload read operations.
5.5. Message Compression
Compress messages before sending them over the network to reduce bandwidth usage and improve transmission speed. You can use algorithms like gzip for text compression.
6. Handling Edge Cases
6.1. Network Disconnections
To handle temporary network disconnections:
- Implement a reconnection strategy with exponential backoff on the client-side.
- Use client-side message queuing to store messages sent while offline.
- Implement a message synchronization protocol to reconcile missed messages upon reconnection.
6.2. Message Ordering
To ensure correct message ordering:
- Assign a timestamp and sequence number to each message at the server level.
- Implement a message buffering system on the client to handle out-of-order message delivery.
- Use the sequence numbers to display messages in the correct order, even if they arrive out of sequence.
6.3. Large Group Chats
For very large group chats (e.g., thousands of members):
- Implement a separate service for handling large group messages.
- Use a pub/sub system like Redis to efficiently broadcast messages to all online group members.
- Implement lazy loading of group members and messages to improve client performance.
7. Security Considerations
To ensure the security and privacy of our chat application:
- Implement end-to-end encryption for messages using protocols like Signal Protocol.
- Use HTTPS for all client-server communications.
- Implement rate limiting to prevent abuse and DDoS attacks.
- Use secure WebSocket connections (WSS) for real-time communication.
- Implement proper input validation and sanitization to prevent injection attacks.
- Use secure file upload mechanisms, including virus scanning for shared files.
- Implement proper access controls and permissions for group chats and file sharing.
8. Monitoring and Logging
To ensure the health and performance of our chat application:
- Implement comprehensive logging across all services.
- Use a centralized log management system like ELK stack (Elasticsearch, Logstash, Kibana) for log analysis.
- Set up real-time monitoring and alerting for key metrics such as message delivery latency, server CPU usage, and database performance.
- Implement distributed tracing to identify performance bottlenecks across services.
Conclusion
Designing a real-time chat application for a system design interview requires careful consideration of scalability, performance, and reliability. By following the approach outlined in this guide, you’ll be well-prepared to tackle this challenge in your next interview.
Remember to:
- Start by clarifying requirements and constraints.
- Design a scalable architecture with clear separation of concerns.
- Consider real-time communication protocols like WebSockets.
- Plan for data persistence, caching, and message queuing.
- Address scalability through horizontal scaling and optimizations.
- Handle edge cases and implement security measures.
- Plan for monitoring and ongoing maintenance.
By demonstrating a thorough understanding of these concepts and your ability to design a complex, scalable system, you’ll significantly improve your chances of success in a system design interview.