The Coder’s Lexicon: Creating a Personal Dictionary of Programming Concepts
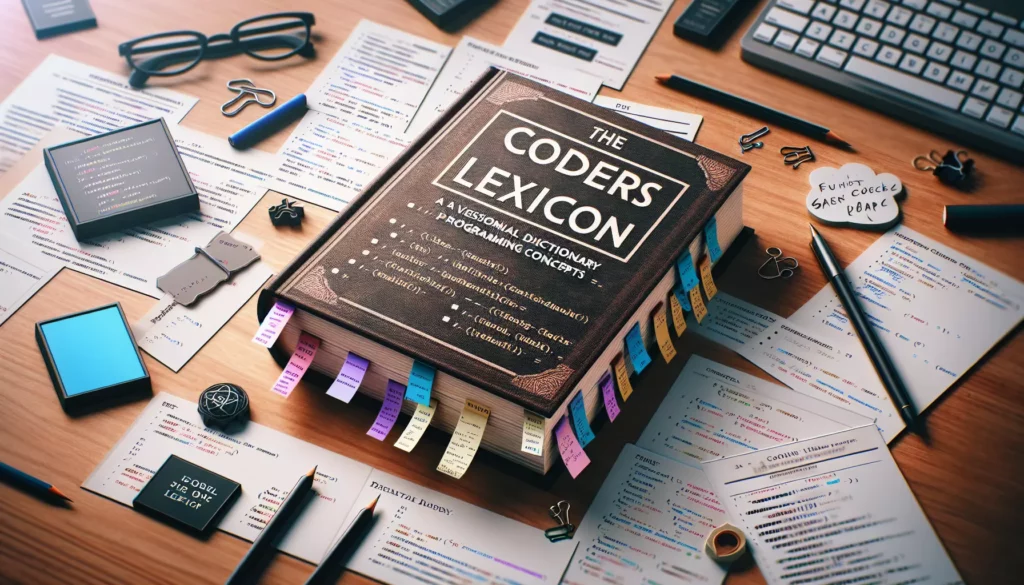
In the ever-evolving world of programming, staying on top of various concepts, terminologies, and best practices can be a daunting task. Whether you’re a beginner just starting your coding journey or an experienced developer looking to expand your knowledge, creating a personal dictionary of programming concepts can be an invaluable tool. This comprehensive guide will walk you through the process of building your own coder’s lexicon, helping you to organize, retain, and apply programming knowledge more effectively.
Why Create a Personal Programming Dictionary?
Before we dive into the how-to, let’s explore the benefits of maintaining your own programming dictionary:
- Improved retention: Writing down concepts in your own words enhances understanding and memory.
- Quick reference: Having a personalized resource allows for rapid recall during coding sessions or interviews.
- Continuous learning: The act of curating and updating your lexicon keeps you engaged with new concepts and technologies.
- Customized knowledge base: Your dictionary reflects your unique learning path and areas of expertise.
- Interview preparation: A well-maintained lexicon can be an excellent study aid for technical interviews, especially for positions at major tech companies.
Getting Started: Choosing Your Format
The first step in creating your coder’s lexicon is deciding on the format. There are several options to consider, each with its own advantages:
1. Digital Note-Taking Apps
Apps like Evernote, OneNote, or Notion offer flexible organization and easy searchability. They allow you to create nested notebooks, add tags, and include multimedia elements like code snippets or diagrams.
2. Personal Wiki
Setting up a personal wiki using platforms like TiddlyWiki or DokuWiki can provide a more structured approach. Wikis excel at creating interconnected pages, making it easy to link related concepts.
3. GitHub Repository
For those comfortable with version control, a GitHub repository can be an excellent choice. It allows for easy collaboration, version tracking, and the ability to share your lexicon with others.
4. Traditional Notebook
Some prefer the tactile experience of writing by hand. A physical notebook can be a great option for those who find they retain information better through handwriting.
5. Flashcard Apps
Apps like Anki or Quizlet are particularly useful for memorization and quick review, especially when preparing for interviews.
Choose the format that best suits your learning style and workflow. You may even decide to use a combination of these methods for different purposes.
Structuring Your Coder’s Lexicon
Once you’ve chosen your format, it’s time to think about how to structure your dictionary. A well-organized lexicon will make it easier to add new entries and find information quickly. Here’s a suggested structure:
1. Main Categories
Start by creating broad categories to organize your entries. Some examples include:
- Programming Languages (e.g., Python, JavaScript, Java)
- Data Structures
- Algorithms
- Design Patterns
- Web Development
- Database Concepts
- DevOps and Deployment
- Software Architecture
- Testing and Debugging
- Version Control
2. Subcategories
Within each main category, create subcategories for more specific topics. For example, under “Algorithms,” you might have:
- Sorting Algorithms
- Searching Algorithms
- Graph Algorithms
- Dynamic Programming
3. Individual Entries
For each concept or term, create an entry that includes:
- Term or Concept Name: The main keyword or phrase
- Definition: A clear, concise explanation in your own words
- Examples: Code snippets or use cases that illustrate the concept
- Related Concepts: Links or references to other entries in your lexicon
- Resources: Links to articles, videos, or documentation for further learning
- Personal Notes: Any insights, mnemonics, or tips you’ve found helpful
Populating Your Lexicon: Where to Start
With your structure in place, it’s time to start adding entries to your coder’s lexicon. Here are some strategies to help you begin:
1. Start with the Basics
Begin by adding fundamental concepts that form the foundation of programming. These might include:
- Variables and data types
- Control structures (if statements, loops)
- Functions and methods
- Object-oriented programming principles
- Basic data structures (arrays, lists, dictionaries)
2. Add Concepts as You Learn
As you encounter new concepts in your coding journey, add them to your lexicon. This could be through:
- Online courses or tutorials
- Programming books
- Coding challenges or projects
- Work experiences
3. Review Common Interview Topics
If you’re preparing for technical interviews, especially for positions at major tech companies, focus on adding entries related to common interview topics:
- Time and space complexity analysis
- Advanced data structures (e.g., trees, graphs, heaps)
- Sorting and searching algorithms
- Dynamic programming
- System design principles
4. Explore Language-Specific Features
For each programming language you work with, create entries for language-specific features and best practices. For example, in Python, you might include:
- List comprehensions
- Decorators
- Context managers
- Generator expressions
5. Incorporate Industry Trends
Stay current by adding entries related to emerging technologies and methodologies:
- Machine learning and AI concepts
- Cloud computing platforms
- Containerization and orchestration
- Agile and DevOps practices
Making the Most of Your Coder’s Lexicon
Creating your lexicon is just the beginning. To maximize its value, consider these tips:
1. Regular Review and Updates
Set aside time periodically to review and update your entries. This helps reinforce your knowledge and ensures your lexicon remains current.
2. Active Application
Whenever you’re working on a coding project or solving a problem, refer to your lexicon. Try to apply the concepts you’ve documented in practical scenarios.
3. Collaborative Learning
If you’re using a shareable format like a GitHub repository, consider collaborating with peers. Sharing knowledge and perspectives can enrich your learning experience.
4. Link to External Resources
For each entry, include links to high-quality external resources. This could include official documentation, well-regarded tutorials, or insightful articles.
5. Use Visualization
Where appropriate, include diagrams, flowcharts, or mind maps to visually represent complex concepts. Tools like draw.io or Mermaid can be helpful for creating these visuals.
6. Practice Active Recall
Regularly test yourself on the concepts in your lexicon. This could be through flashcards, coding challenges, or explaining concepts to others.
Example Entry: Binary Search
To illustrate how an entry in your coder’s lexicon might look, here’s an example for the concept of Binary Search:
<h3>Binary Search</h3>
<p><strong>Definition:</strong> Binary search is an efficient algorithm for finding a target value within a sorted array. It works by repeatedly dividing the search interval in half.</p>
<p><strong>Key Characteristics:</strong></p>
<ul>
<li>Time Complexity: O(log n)</li>
<li>Space Complexity: O(1) for iterative implementation</li>
<li>Requires a sorted array</li>
</ul>
<p><strong>Example Implementation (Python):</strong></p>
<pre><code>def binary_search(arr, target):
left, right = 0, len(arr) - 1
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1 # Target not found
# Example usage
sorted_array = [1, 3, 5, 7, 9, 11, 13, 15]
target = 7
result = binary_search(sorted_array, target)
print(f"Target {target} found at index: {result}")
</code></pre>
<p><strong>Related Concepts:</strong></p>
<ul>
<li>Linear Search</li>
<li>Divide and Conquer Algorithms</li>
<li>Time Complexity Analysis</li>
</ul>
<p><strong>Resources:</strong></p>
<ul>
<li><a href="https://www.geeksforgeeks.org/binary-search/">GeeksforGeeks: Binary Search</a></li>
<li><a href="https://www.khanacademy.org/computing/computer-science/algorithms/binary-search/a/binary-search">Khan Academy: Binary Search</a></li>
</ul>
<p><strong>Personal Notes:</strong> Remember, binary search is like finding a name in a phone book - you always start in the middle and eliminate half the remaining options with each step. Key to many coding interview questions!</p>
Leveraging Your Lexicon for Interview Preparation
As you build your coder’s lexicon, you’re not just creating a reference tool; you’re developing a powerful resource for interview preparation, especially for positions at major tech companies. Here’s how to leverage your lexicon effectively:
1. Focus on High-Priority Topics
Identify and prioritize topics that frequently appear in technical interviews. These often include:
- Data Structures (arrays, linked lists, trees, graphs, hash tables)
- Algorithms (sorting, searching, graph traversal, dynamic programming)
- System Design concepts
- Object-Oriented Programming principles
- Time and space complexity analysis
2. Create Problem-Solving Entries
For each major algorithm or data structure, include entries that outline common problem-solving patterns. For example:
- Two-pointer technique for array problems
- Sliding window approach for substring problems
- BFS vs DFS for graph traversal
- Recursion and memoization for dynamic programming
3. Develop Cheat Sheets
Create condensed versions of key entries that serve as quick reference guides. These can be particularly useful for last-minute review before an interview.
4. Practice Explaining Concepts
Use your lexicon entries as prompts for verbal explanations. Practice articulating complex concepts clearly and concisely, as you might need to do in an interview setting.
5. Link to Practice Problems
For each concept, include links to related practice problems from platforms like LeetCode, HackerRank, or AlgoCademy. This helps bridge the gap between theory and practical application.
6. Review Company-Specific Information
If you’re targeting specific companies, create entries that cover their tech stacks, products, and any publicly known engineering challenges they face.
Continuous Improvement of Your Coder’s Lexicon
Your coder’s lexicon should be a living document that grows and evolves with your knowledge and experience. Here are some strategies for continuous improvement:
1. Regular Audits
Periodically review your lexicon to identify areas that need updating or expansion. Technology moves quickly, so ensure your entries remain current.
2. Feedback Loop
If you’re sharing your lexicon with peers or mentors, encourage feedback. Fresh perspectives can help you identify gaps or areas for improvement.
3. Integrate New Learning
Whenever you learn something new – whether from a project, a course, or a conference – make it a habit to add it to your lexicon.
4. Refine Based on Interview Experiences
After each technical interview, reflect on the questions you were asked and the concepts discussed. Use these experiences to refine and expand your lexicon entries.
5. Cross-Pollinate Knowledge
Look for connections between different areas of your lexicon. Understanding how various concepts interrelate can deepen your overall comprehension.
Conclusion: Your Personal Path to Programming Mastery
Creating and maintaining a personal coder’s lexicon is more than just an organizational exercise; it’s a powerful tool for continuous learning and professional growth. By systematically documenting, reviewing, and expanding your programming knowledge, you’re building a solid foundation for success in your coding journey.
Whether you’re preparing for technical interviews at top tech companies, working on personal projects, or simply striving to become a better programmer, your lexicon will serve as a trusted companion. It’s a reflection of your unique learning path and a testament to your growing expertise.
Remember, the process of creating your lexicon is just as valuable as the end product. Each entry you add, each concept you clarify, and each connection you make contributes to your deeper understanding of the vast and exciting world of programming.
So, start building your coder’s lexicon today. Begin with the basics, expand as you learn, and watch as your personal dictionary becomes an indispensable asset in your programming toolkit. Happy coding, and may your lexicon guide you to new heights in your programming career!