Coding in Reverse: Solving LeetCode Problems Starting from the End
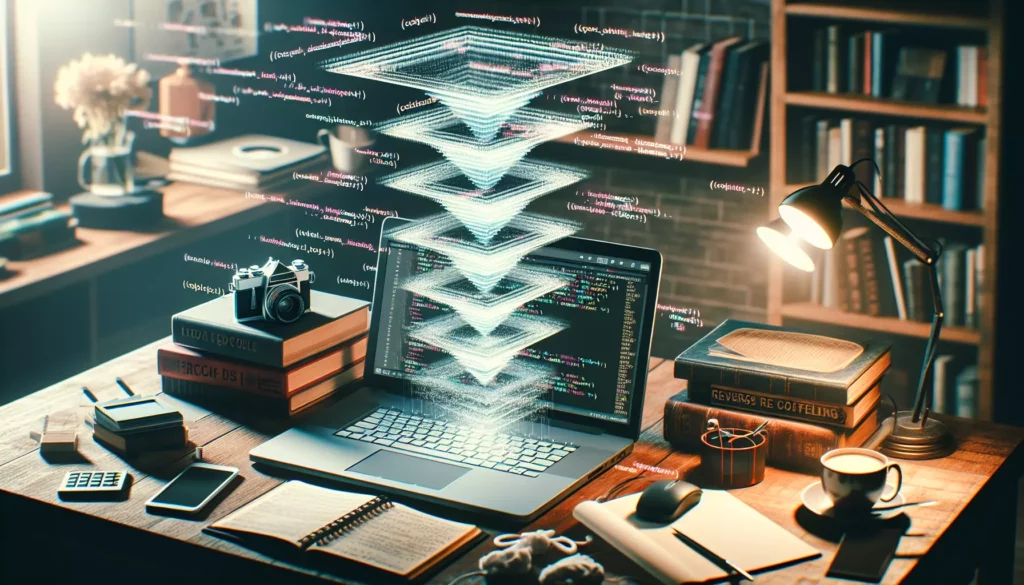
In the world of coding interviews and algorithmic problem-solving, LeetCode has become a go-to platform for developers looking to sharpen their skills. However, the traditional approach of tackling these problems from the beginning can sometimes lead to mental blocks or inefficient solutions. This is where the concept of “coding in reverse” comes into play – a strategy that involves starting from the desired end result and working backwards to construct the solution.
Understanding the “Coding in Reverse” Approach
The “coding in reverse” technique is not about writing code from right to left or bottom to top. Instead, it’s a problem-solving strategy that encourages developers to begin with the end goal in mind and reverse-engineer the solution. This approach can be particularly effective for complex LeetCode problems where the path to the solution isn’t immediately apparent.
Benefits of Reverse Problem-Solving
- Clearer problem understanding
- Simplified solution design
- Improved efficiency in reaching the optimal solution
- Enhanced ability to handle edge cases
- Better alignment with the expected output
Step-by-Step Guide to Coding in Reverse
Let’s break down the process of coding in reverse into actionable steps:
1. Analyze the Expected Output
Begin by thoroughly examining the expected output of the problem. What exactly should your function or algorithm produce? Understanding the end result is crucial for reverse-engineering the solution.
2. Identify the Final State
Determine what the final state of your data structures or variables should be just before returning the result. This step helps you visualize the solution’s endpoint.
3. Work Backwards
Starting from the final state, think about what steps or transformations are necessary to reach that point. What operations need to be performed on the input data?
4. Outline the Solution
Create a high-level outline of your solution, starting from the end and moving towards the beginning. This outline serves as a roadmap for your code implementation.
5. Implement in Reverse Order
Begin coding the solution, starting with the final steps and gradually moving towards the initial input processing. This approach ensures that you’re always working towards a known state.
6. Refine and Optimize
Once you have a working solution, refine and optimize it. Look for opportunities to improve efficiency or simplify the code.
Practical Example: Reverse Coding a LeetCode Problem
Let’s apply the reverse coding technique to a classic LeetCode problem: “Reverse a Linked List” (Problem 206).
Problem Statement:
Given the head of a singly linked list, reverse the list, and return the reversed list.
Expected Output:
A linked list with its nodes in reverse order.
Reverse Coding Approach:
- Analyze the Expected Output: We need to return the head of the reversed list.
- Identify the Final State: The last node of the original list should become the head of the reversed list.
- Work Backwards: We need to change each node’s next pointer to its previous node.
- Outline the Solution:
- Start with the last node as the new head
- For each node, set its next node’s next pointer to itself
- Continue until we reach the original head
- Set the original head’s next pointer to null
- Implement in Reverse Order: We’ll use a three-pointer approach to reverse the links.
Implementation:
class Solution {
public ListNode reverseList(ListNode head) {
ListNode prev = null;
ListNode current = head;
ListNode next = null;
while (current != null) {
next = current.next; // Store next node
current.next = prev; // Reverse the link
prev = current; // Move prev one step forward
current = next; // Move current one step forward
}
return prev; // prev is now the head of the reversed list
}
}
By starting from the end state (the reversed list) and working backwards, we’ve created a solution that efficiently reverses the linked list in-place.
Advanced Reverse Coding Techniques
As you become more comfortable with the basic reverse coding approach, you can apply more advanced techniques to tackle complex LeetCode problems:
1. State Transition Mapping
For problems involving state changes or transformations, create a map of the desired state transitions working backwards from the final state. This technique is particularly useful for dynamic programming problems.
2. Reverse Simulation
In problems that involve simulations or step-by-step processes, try simulating the process in reverse. This can often lead to insights about optimizations or shortcuts in the forward solution.
3. Output-Driven Input Construction
For problems where you need to construct or manipulate input to achieve a specific output, start by analyzing the properties of the desired output and work backwards to determine the necessary input characteristics.
4. Reverse Induction
In mathematical or logical problems, use reverse induction by assuming the final state or solution and working backwards to prove or construct the initial conditions that lead to that solution.
Common LeetCode Problem Types and Reverse Coding Strategies
Different types of LeetCode problems can benefit from specific reverse coding strategies. Let’s explore some common problem categories and how to approach them in reverse:
1. Array Manipulation Problems
Reverse Strategy: Start by visualizing the final array state and work backwards to determine the necessary transformations.
Example Problem: “Product of Array Except Self” (Problem 238)
Reverse Approach: Begin with the final product array and consider how each element contributes to the products of others. This leads to the realization that you can use prefix and suffix products.
2. String Problems
Reverse Strategy: Analyze the desired output string characteristics and work backwards to determine the necessary string operations.
Example Problem: “Longest Palindromic Substring” (Problem 5)
Reverse Approach: Start by considering what makes a substring palindromic, then work backwards to find the center(s) of potential palindromes and expand outwards.
3. Tree and Graph Problems
Reverse Strategy: Visualize the desired tree or graph structure and work backwards to determine the necessary traversal or construction steps.
Example Problem: “Construct Binary Tree from Inorder and Postorder Traversal” (Problem 106)
Reverse Approach: Start from the root (last element in postorder) and recursively construct right and left subtrees by analyzing the traversal arrays from right to left.
4. Dynamic Programming Problems
Reverse Strategy: Begin with the final state or solution and work backwards to build the dp table or recurrence relation.
Example Problem: “Coin Change” (Problem 322)
Reverse Approach: Start from the target amount and work backwards to find the minimum number of coins needed for each smaller amount.
Challenges and Limitations of Reverse Coding
While reverse coding can be a powerful problem-solving technique, it’s important to be aware of its challenges and limitations:
1. Overcomplication
Sometimes, working backwards can lead to overcomplicated solutions for simple problems. It’s important to recognize when a straightforward approach might be more appropriate.
2. Time Complexity Considerations
Reverse-engineered solutions might not always yield the most time-efficient algorithms. Be mindful of the time complexity requirements of the problem.
3. Difficulty with Certain Problem Types
Some problems, particularly those involving real-time processing or streaming data, may not lend themselves well to reverse coding approaches.
4. Mental Shift Required
Reverse coding requires a different mental model, which can be challenging for developers accustomed to traditional problem-solving approaches. It may take practice to become proficient in this technique.
Integrating Reverse Coding into Your Problem-Solving Toolkit
To effectively use reverse coding in your LeetCode problem-solving arsenal:
1. Practice Regularly
Dedicate time to solving problems using the reverse coding approach. Start with simpler problems and gradually move to more complex ones.
2. Analyze Official Solutions
After solving a problem, study the official solution and try to understand how it could be derived using a reverse coding approach.
3. Combine with Other Techniques
Use reverse coding in conjunction with other problem-solving strategies like divide and conquer, greedy algorithms, or dynamic programming.
4. Teach and Explain
Try explaining your reverse coding solutions to others. Teaching reinforces your understanding and helps identify areas for improvement.
Conclusion: Mastering the Art of Reverse Coding
Coding in reverse is more than just a novel approach to solving LeetCode problems – it’s a powerful cognitive tool that can reshape how you approach algorithmic challenges. By starting from the desired outcome and working backwards, you often gain insights that might be missed when approaching the problem conventionally.
As you integrate this technique into your problem-solving toolkit, you’ll likely find that certain problems become more intuitive and that your overall coding efficiency improves. Remember, the goal isn’t to use reverse coding for every problem, but to have it as a valuable option when traditional approaches fall short.
Mastering reverse coding takes practice and patience. As you apply this technique to more LeetCode problems, you’ll develop a keener sense of when and how to use it most effectively. This skill will not only help you in coding interviews but will also enhance your general problem-solving abilities in software development.
So the next time you’re faced with a challenging LeetCode problem, consider flipping your perspective and starting from the end. You might just find that the solution was hiding in plain sight, waiting for you to approach it from a different angle.