The Forgetful Programmer: Techniques for Remembering What You Just Wrote
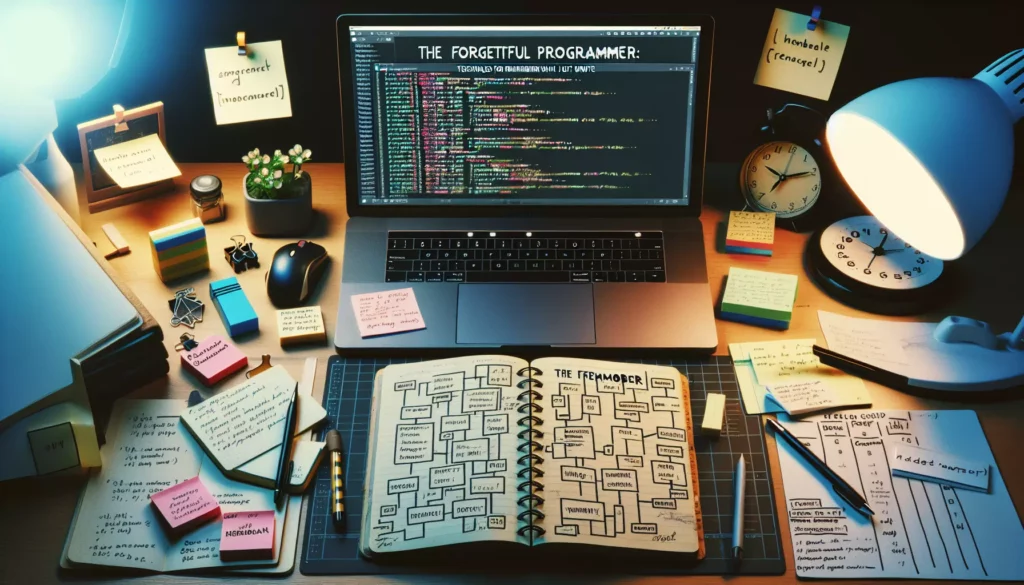
As programmers, we’ve all been there. You’re deep in the flow, writing line after line of code, solving complex problems, and feeling like a coding superhero. Then, suddenly, you need to revisit a function you wrote just an hour ago, and… blank. You can’t remember how it works or why you wrote it that way. Welcome to the world of the forgetful programmer!
In this comprehensive guide, we’ll explore various techniques and strategies to help you remember what you’ve written, improve your code retention, and ultimately become a more efficient and effective programmer. Whether you’re a beginner just starting your coding journey or an experienced developer looking to sharpen your skills, these tips will help you combat the dreaded “code amnesia” and boost your productivity.
1. Write Clear and Descriptive Comments
One of the simplest yet most effective ways to remember your code is to write clear and descriptive comments. Comments serve as a roadmap for your future self (and other developers) to understand the logic and purpose behind your code.
Best Practices for Writing Comments:
- Explain the “why” behind your code, not just the “what”
- Use consistent formatting and style
- Keep comments up-to-date as your code evolves
- Avoid redundant or obvious comments
- Use inline comments sparingly for complex logic
Here’s an example of good commenting practice:
// Calculate the Fibonacci sequence up to n terms
// Using dynamic programming to optimize performance
function fibonacci(n) {
// Initialize an array to store Fibonacci numbers
let fib = [0, 1];
// Generate Fibonacci numbers iteratively
for (let i = 2; i < n; i++) {
// Each number is the sum of the two preceding ones
fib[i] = fib[i-1] + fib[i-2];
}
return fib;
}
2. Use Meaningful Variable and Function Names
Choosing descriptive and meaningful names for your variables and functions can significantly improve code readability and make it easier to remember what each component does. Avoid single-letter variables (except for simple loop counters) and opt for names that clearly describe the purpose or content of the variable or function.
Tips for Naming Variables and Functions:
- Use camelCase for variables and functions in most programming languages
- Be specific and avoid ambiguity
- Use verbs for function names (e.g., calculateTotal, fetchUserData)
- Use nouns or adjectives for variable names (e.g., userAge, isActive)
- Avoid abbreviations unless they’re widely understood
Compare these two code snippets:
// Poor naming
function f(x, y) {
return x * y;
}
// Good naming
function calculateRectangleArea(width, height) {
return width * height;
}
3. Break Down Complex Functions
Large, complex functions can be difficult to understand and remember. By breaking them down into smaller, more focused functions, you make your code more modular, easier to read, and simpler to maintain. This approach, known as the Single Responsibility Principle, is a key concept in clean code practices.
Benefits of Breaking Down Functions:
- Improved readability and maintainability
- Easier debugging and testing
- Increased code reusability
- Better organization of logic
Here’s an example of refactoring a complex function into smaller, more manageable pieces:
// Before: Complex, hard-to-remember function
function processUserData(userData) {
// Validate user input
if (!userData.name || !userData.email || !userData.age) {
throw new Error("Invalid user data");
}
// Format user name
const formattedName = userData.name.trim().toLowerCase().split(' ').map(word => word.charAt(0).toUpperCase() + word.slice(1)).join(' ');
// Validate email
const emailRegex = /^[^\s@]+@[^\s@]+\.[^\s@]+$/;
if (!emailRegex.test(userData.email)) {
throw new Error("Invalid email address");
}
// Calculate user category based on age
let category;
if (userData.age < 18) {
category = "Junior";
} else if (userData.age < 60) {
category = "Adult";
} else {
category = "Senior";
}
return {
name: formattedName,
email: userData.email,
age: userData.age,
category: category
};
}
// After: Broken down into smaller, easier-to-remember functions
function validateUserData(userData) {
if (!userData.name || !userData.email || !userData.age) {
throw new Error("Invalid user data");
}
}
function formatName(name) {
return name.trim().toLowerCase().split(' ')
.map(word => word.charAt(0).toUpperCase() + word.slice(1))
.join(' ');
}
function validateEmail(email) {
const emailRegex = /^[^\s@]+@[^\s@]+\.[^\s@]+$/;
if (!emailRegex.test(email)) {
throw new Error("Invalid email address");
}
}
function calculateUserCategory(age) {
if (age < 18) return "Junior";
if (age < 60) return "Adult";
return "Senior";
}
function processUserData(userData) {
validateUserData(userData);
const formattedName = formatName(userData.name);
validateEmail(userData.email);
const category = calculateUserCategory(userData.age);
return {
name: formattedName,
email: userData.email,
age: userData.age,
category: category
};
}
4. Use Version Control Systems
Version control systems like Git are invaluable tools for programmers. They not only help you track changes and collaborate with others but also serve as a memory aid. By making frequent, small commits with descriptive messages, you create a detailed history of your code that you can refer back to when you need to remember why you made certain changes.
Best Practices for Using Version Control:
- Make small, focused commits
- Write clear and descriptive commit messages
- Use branches for different features or experiments
- Review your commit history regularly
- Use tags to mark important milestones
Example of a good commit message:
Fix bug in user authentication process
- Add email validation before sending reset password link
- Implement rate limiting to prevent brute force attacks
- Update error messages for better user feedback
5. Document Your Code
While comments are great for explaining small sections of code, proper documentation is essential for understanding the bigger picture. Documentation can include README files, API documentation, and inline documentation for functions and classes.
Types of Documentation to Consider:
- README files: Provide an overview of your project, setup instructions, and basic usage
- API documentation: Describe the inputs, outputs, and behavior of your functions and classes
- Architecture documentation: Explain the overall structure and design of your project
- Inline documentation: Use tools like JSDoc or Sphinx to generate documentation from your code comments
Here’s an example of using JSDoc for inline documentation:
/**
* Calculates the area of a circle.
*
* @param {number} radius - The radius of the circle.
* @returns {number} The area of the circle.
* @throws {Error} If the radius is negative.
*/
function calculateCircleArea(radius) {
if (radius < 0) {
throw new Error("Radius cannot be negative");
}
return Math.PI * radius * radius;
}
6. Implement a Consistent Coding Style
Adhering to a consistent coding style makes your code more readable and easier to remember. Whether you’re working on a personal project or collaborating with a team, having a set of style guidelines can significantly improve code quality and maintainability.
Elements of a Coding Style Guide:
- Indentation and whitespace usage
- Naming conventions for variables, functions, and classes
- File organization and module structure
- Comment style and frequency
- Code formatting (e.g., line length, bracket placement)
Consider using linting tools like ESLint for JavaScript or Black for Python to enforce a consistent style automatically.
7. Practice Regular Code Reviews
Code reviews, whether done by yourself or with peers, can help reinforce your understanding of the code you’ve written. By explaining your code to others or critically examining it yourself, you’re more likely to remember the reasoning behind your implementation choices.
Tips for Effective Code Reviews:
- Review code in small chunks rather than large batches
- Focus on both the overall design and the implementation details
- Ask questions about unclear parts of the code
- Suggest improvements and alternatives
- Use code review tools to track comments and changes
8. Utilize Code Visualization Tools
Sometimes, seeing your code structure visually can help you better understand and remember its organization. Various tools can generate diagrams or visual representations of your code, making it easier to grasp complex relationships between different components.
Popular Code Visualization Tools:
- Draw.io: Create flowcharts and diagrams
- PlantUML: Generate UML diagrams from text descriptions
- CodeSee: Visualize codebases and track changes over time
- Sourcetrail: Create interactive, searchable graphs of your codebase
9. Implement Test-Driven Development (TDD)
Test-Driven Development is a software development approach where you write tests before implementing the actual code. This practice not only helps ensure code quality but also serves as a form of documentation and a memory aid for understanding the expected behavior of your code.
Benefits of TDD for Code Memory:
- Forces you to think about the desired outcome before writing code
- Provides a clear specification of what your code should do
- Helps you catch and remember edge cases
- Makes it easier to refactor code without breaking functionality
Here’s a simple example of TDD in JavaScript using the Jest testing framework:
// Test file: calculator.test.js
const { add } = require('./calculator');
describe('Calculator', () => {
test('adds two numbers correctly', () => {
expect(add(2, 3)).toBe(5);
expect(add(-1, 1)).toBe(0);
expect(add(0, 0)).toBe(0);
});
});
// Implementation file: calculator.js
function add(a, b) {
return a + b;
}
module.exports = { add };
10. Keep a Coding Journal or Blog
Maintaining a coding journal or blog can be an excellent way to reinforce your understanding of the code you write. By explaining your thought process, challenges faced, and solutions implemented, you create a personal knowledge base that you can refer back to when needed.
Ideas for Your Coding Journal:
- Document interesting problems you’ve solved
- Explain new concepts or techniques you’ve learned
- Reflect on your coding process and areas for improvement
- Keep track of useful resources and tools
- Share your experiences and insights with others
11. Use Code Snippets and Templates
Creating a library of reusable code snippets and templates can help you remember common patterns and implementations. Most modern code editors support snippet functionality, allowing you to quickly insert pre-defined code blocks.
Tips for Managing Code Snippets:
- Organize snippets by language and functionality
- Use descriptive names for easy recall
- Include comments explaining usage and any required modifications
- Regularly review and update your snippet library
- Consider using snippet management tools like Gist or SnippetsLab
12. Leverage IDE Features
Modern Integrated Development Environments (IDEs) offer numerous features that can help you navigate and understand your code better. Familiarize yourself with these features to boost your productivity and code recall.
Useful IDE Features:
- Code navigation (e.g., “Go to Definition”, “Find All References”)
- Refactoring tools
- Code completion and suggestions
- Integrated debugging tools
- Code outline and structure views
13. Practice Spaced Repetition
Spaced repetition is a learning technique that involves reviewing information at increasing intervals. Apply this concept to your coding practice by regularly revisiting and refactoring old projects or reviewing code you wrote in the past.
Implementing Spaced Repetition for Coding:
- Set reminders to review code from past projects
- Maintain a list of important concepts and review them periodically
- Use flashcard apps like Anki to remember coding patterns and syntax
- Participate in coding challenges to reinforce your skills
14. Teach Others
One of the most effective ways to remember something is to teach it to someone else. Look for opportunities to explain your code or share your knowledge with fellow programmers. This could be through mentoring, giving presentations, or contributing to open-source projects.
Ways to Share Your Knowledge:
- Participate in code review sessions
- Write technical blog posts or tutorials
- Contribute to documentation for open-source projects
- Answer questions on platforms like Stack Overflow
- Give talks at local meetups or conferences
Conclusion
Remembering what you’ve written is a crucial skill for any programmer. By implementing these techniques and making them a part of your regular coding practice, you’ll not only improve your ability to recall and understand your code but also become a more efficient and effective developer.
Remember, becoming a “less forgetful” programmer is an ongoing process. It requires consistent effort and a willingness to reflect on and improve your coding practices. As you apply these techniques, you’ll likely discover additional strategies that work well for you. Don’t be afraid to experiment and adapt these methods to suit your personal coding style and workflow.
By investing time in improving your code memory and understanding, you’re setting yourself up for long-term success in your programming career. Whether you’re preparing for technical interviews, working on complex projects, or collaborating with a team, these skills will serve you well and help you become a more confident and capable programmer.
So, the next time you find yourself staring at a piece of code you wrote, wondering what on earth you were thinking, remember these techniques. With practice and persistence, you’ll be well on your way to conquering code amnesia and becoming a more memorable programmer!