Why Knowledge of Caching Strategies Is Important for System Design
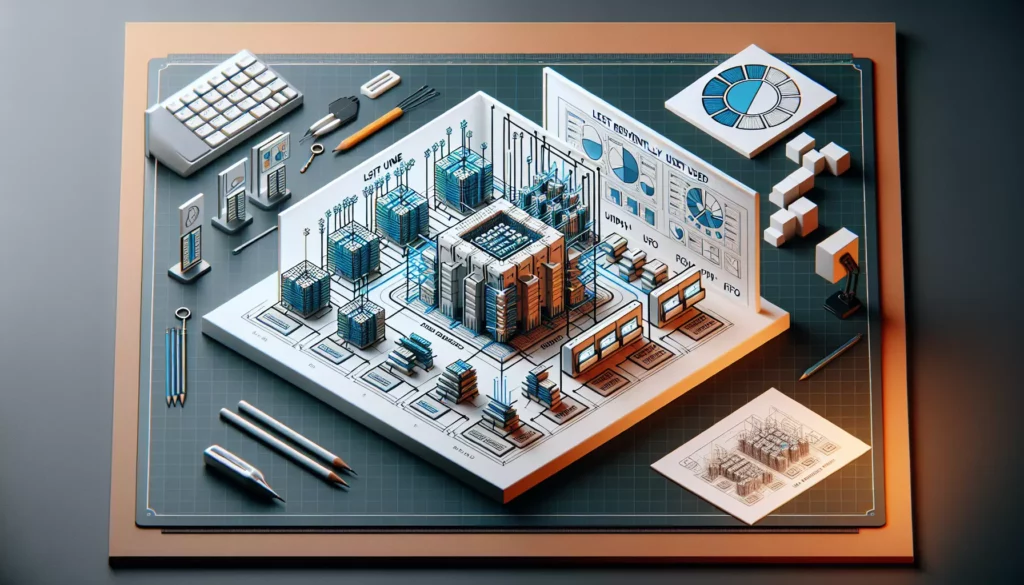
In the world of software engineering and system design, caching strategies play a crucial role in optimizing performance, reducing latency, and improving overall user experience. As developers progress from basic coding skills to more advanced concepts, understanding caching becomes increasingly important, especially when preparing for technical interviews at major tech companies. In this comprehensive guide, we’ll explore why knowledge of caching strategies is essential for system design and how it can impact your career as a software engineer.
1. The Fundamentals of Caching
Before diving into the importance of caching strategies, let’s review the basics of caching:
1.1 What is Caching?
Caching is the process of storing frequently accessed data in a temporary storage location for quick retrieval. Instead of fetching the data from its original source every time it’s needed, the system can access the cached copy, significantly reducing response times and computational overhead.
1.2 Types of Caches
There are several types of caches used in system design:
- In-memory cache: Stores data in the application’s memory for ultra-fast access
- Distributed cache: Spreads cached data across multiple nodes in a network
- Database cache: Caches query results to reduce database load
- CDN cache: Stores static content closer to end-users for faster delivery
- Browser cache: Stores web resources locally on the user’s device
2. The Impact of Caching on System Performance
Understanding caching strategies is crucial because of their significant impact on system performance:
2.1 Reduced Latency
Caching can dramatically reduce the time it takes to retrieve data. For example, consider a social media application that needs to display user profiles:
// Without caching
function getUserProfile(userId) {
return database.query("SELECT * FROM users WHERE id = ?", userId);
}
// With caching
const cache = new Map();
function getUserProfile(userId) {
if (cache.has(userId)) {
return cache.get(userId);
}
const profile = database.query("SELECT * FROM users WHERE id = ?", userId);
cache.set(userId, profile);
return profile;
}
In this example, subsequent requests for the same user profile will be served from the cache, significantly reducing response times.
2.2 Improved Scalability
Caching helps systems handle increased load by reducing the strain on backend resources. This is particularly important for large-scale applications that need to serve millions of users simultaneously.
2.3 Cost Reduction
By reducing the need for expensive computations or database queries, caching can lead to significant cost savings in terms of server resources and database licenses.
3. Common Caching Strategies
To effectively implement caching in system design, it’s essential to understand various caching strategies:
3.1 Cache-Aside (Lazy Loading)
In this strategy, the application first checks the cache for requested data. If it’s not found (a cache miss), the data is retrieved from the database and then stored in the cache for future use.
function getData(key) {
let data = cache.get(key);
if (data === null) {
data = database.query(key);
cache.set(key, data);
}
return data;
}
3.2 Write-Through
This strategy updates both the cache and the database simultaneously when data is modified. It ensures consistency but may introduce additional latency for write operations.
function updateData(key, value) {
database.update(key, value);
cache.set(key, value);
}
3.3 Write-Behind (Write-Back)
In this approach, data is written to the cache immediately but only periodically flushed to the database. This can improve write performance but risks data loss if the system fails before the data is persisted.
const writeQueue = [];
function updateData(key, value) {
cache.set(key, value);
writeQueue.push({ key, value });
}
setInterval(() => {
while (writeQueue.length > 0) {
const { key, value } = writeQueue.shift();
database.update(key, value);
}
}, 5000); // Flush to database every 5 seconds
3.4 Read-Through
Similar to cache-aside, but the caching layer is responsible for loading data from the database on a cache miss, rather than the application code.
4. Caching in Distributed Systems
As systems scale, distributed caching becomes increasingly important. Knowledge of distributed caching strategies is crucial for designing large-scale applications:
4.1 Consistent Hashing
Consistent hashing is a technique used to distribute cached data across multiple nodes in a way that minimizes reorganization when nodes are added or removed.
class ConsistentHash {
constructor(nodes, virtualNodes = 100) {
this.nodes = new Map();
this.keys = [];
for (let node of nodes) {
this.addNode(node, virtualNodes);
}
}
addNode(node, virtualNodes) {
for (let i = 0; i < virtualNodes; i++) {
let key = this.hash(`${node}:${i}`);
this.nodes.set(key, node);
this.keys.push(key);
}
this.keys.sort((a, b) => a - b);
}
removeNode(node, virtualNodes) {
for (let i = 0; i < virtualNodes; i++) {
let key = this.hash(`${node}:${i}`);
this.nodes.delete(key);
this.keys = this.keys.filter(k => k !== key);
}
}
getNode(key) {
if (this.nodes.size === 0) return null;
let hash = this.hash(key);
let node = this.findNode(hash);
return this.nodes.get(node);
}
findNode(hash) {
let i = this.keys.findIndex(k => k >= hash);
return i === -1 ? this.keys[0] : this.keys[i];
}
hash(key) {
// Simple hash function for demonstration
let total = 0;
for (let i = 0; i < key.length; i++) {
total += key.charCodeAt(i);
}
return total % 1000;
}
}
4.2 Cache Replication
Replicating cache data across multiple nodes can improve availability and read performance but requires careful management of consistency.
4.3 Cache Partitioning
Partitioning involves dividing the cache data across multiple nodes based on a partitioning strategy, such as range partitioning or hash partitioning.
5. Cache Eviction Policies
Understanding cache eviction policies is crucial for managing limited cache space effectively:
5.1 Least Recently Used (LRU)
LRU discards the least recently used items first. It’s a popular choice for many caching scenarios.
class LRUCache {
constructor(capacity) {
this.capacity = capacity;
this.cache = new Map();
}
get(key) {
if (!this.cache.has(key)) return -1;
const value = this.cache.get(key);
this.cache.delete(key);
this.cache.set(key, value);
return value;
}
put(key, value) {
if (this.cache.has(key)) {
this.cache.delete(key);
}
this.cache.set(key, value);
if (this.cache.size > this.capacity) {
const oldestKey = this.cache.keys().next().value;
this.cache.delete(oldestKey);
}
}
}
5.2 Least Frequently Used (LFU)
LFU removes the items that are used least frequently when the cache reaches its capacity.
5.3 Time-To-Live (TTL)
TTL assigns an expiration time to each cache entry, automatically removing items after they expire.
6. Challenges in Caching
While caching offers significant benefits, it also introduces challenges that system designers must address:
6.1 Cache Invalidation
Keeping cached data in sync with the source of truth is a common challenge. Strategies like time-based expiration, version tagging, and explicit invalidation can help manage this issue.
6.2 Cache Coherence
In distributed systems, maintaining consistency across multiple cache instances can be complex. Techniques like cache invalidation protocols and eventual consistency models are used to address this challenge.
6.3 Cold Start
When a cache is empty (e.g., after a system restart), it can lead to increased load on backend systems. Strategies like pre-warming the cache or using fallback mechanisms can mitigate this issue.
7. Caching in Different Layers of the System
Understanding how caching can be applied at different layers of a system is crucial for comprehensive system design:
7.1 Client-Side Caching
Implementing caching in web browsers or mobile apps can significantly improve user experience by reducing network requests.
// Example of client-side caching using the browser's Cache API
async function fetchWithCache(url) {
const cache = await caches.open('my-cache');
const cachedResponse = await cache.match(url);
if (cachedResponse) {
return cachedResponse;
}
const response = await fetch(url);
cache.put(url, response.clone());
return response;
}
7.2 CDN Caching
Content Delivery Networks (CDNs) cache static assets closer to end-users, reducing latency for geographically distributed applications.
7.3 Application-Level Caching
Caching within the application code, such as memoization of expensive computations or caching of database query results.
7.4 Database Caching
Many databases have built-in caching mechanisms that can be leveraged to improve query performance.
8. Monitoring and Optimizing Cache Performance
To effectively use caching in system design, it’s important to monitor and optimize cache performance:
8.1 Cache Hit Ratio
Monitoring the cache hit ratio (percentage of requests served from the cache) can help identify opportunities for optimization.
8.2 Cache Warm-Up Time
Understanding how long it takes for a cache to become effective after a cold start can inform strategies for managing system restarts or scaling events.
8.3 Cache Size and Memory Usage
Balancing cache size with available memory resources is crucial for optimal performance.
9. Caching in Real-World Scenarios
Let’s explore how caching strategies are applied in real-world scenarios:
9.1 E-commerce Product Catalog
In an e-commerce application, product details can be cached to reduce database load and improve page load times. However, care must be taken to invalidate the cache when product information is updated.
9.2 Social Media Feed
Social media platforms often use a combination of caching strategies to serve user feeds quickly while maintaining reasonable freshness of content.
9.3 Video Streaming Services
Video streaming platforms use extensive caching, including CDN caching and client-side caching, to deliver smooth playback experiences to users worldwide.
10. Caching in Technical Interviews
For those preparing for technical interviews, especially at major tech companies, understanding caching strategies is crucial:
10.1 System Design Questions
Caching often plays a key role in system design interview questions. Being able to discuss when and how to implement caching can significantly strengthen your solutions.
10.2 Performance Optimization
Interviewers may ask how you would optimize a given system, and caching is often a key component of such optimizations.
10.3 Trade-off Discussions
Being able to discuss the trade-offs involved in different caching strategies (e.g., consistency vs. performance) demonstrates a deep understanding of system design principles.
Conclusion
Knowledge of caching strategies is undeniably important for system design. It enables developers to create high-performance, scalable systems that can handle large amounts of traffic efficiently. From improving response times and reducing server load to optimizing costs and enhancing user experience, caching touches nearly every aspect of modern software architecture.
As you progress in your coding education and prepare for technical interviews, make sure to invest time in understanding and practicing various caching techniques. This knowledge will not only help you design better systems but also demonstrate your ability to think critically about performance and scalability – skills highly valued by top tech companies.
Remember, while caching is powerful, it’s not a silver bullet. Each caching strategy comes with its own set of trade-offs and challenges. The key is to understand these nuances and apply caching judiciously based on the specific requirements of your system. With a solid grasp of caching strategies, you’ll be well-equipped to tackle complex system design problems and excel in your software engineering career.