How to Design a Scalable Web Application for an Interview: A Comprehensive Guide
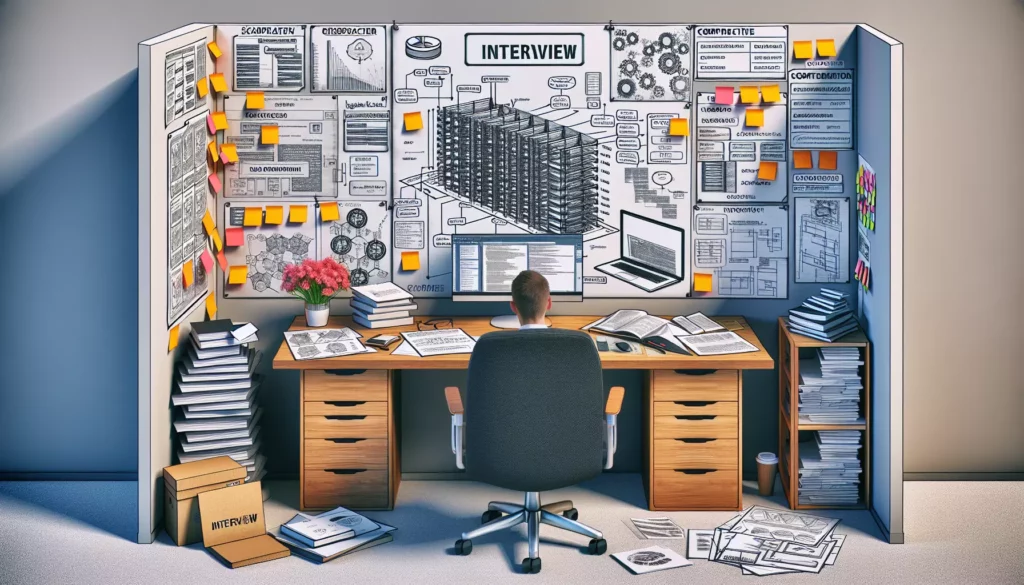
In today’s competitive tech landscape, designing scalable web applications is a crucial skill that many employers look for, especially during technical interviews. Whether you’re interviewing for a position at a FAANG company (Facebook, Amazon, Apple, Netflix, Google) or any other tech firm, understanding the principles of scalable web application design can set you apart from other candidates. This comprehensive guide will walk you through the process of designing a scalable web application, providing you with the knowledge and confidence to ace your next interview.
Table of Contents
- Understanding Scalability
- Key Principles of Scalable Web Application Design
- Choosing the Right Architecture
- Database Design and Optimization
- Caching Strategies
- Load Balancing and Traffic Management
- Microservices Architecture
- API Design for Scalability
- Monitoring and Logging
- Security Considerations
- Leveraging Cloud Services
- Case Study: Designing a Scalable Social Media Platform
- Interview Tips and Common Questions
- Conclusion
1. Understanding Scalability
Before diving into the specifics of designing a scalable web application, it’s essential to understand what scalability means in the context of web development. Scalability refers to a system’s ability to handle increased load without compromising performance or requiring a complete redesign.
There are two main types of scalability:
- Vertical Scalability (Scaling Up): This involves adding more resources (CPU, RAM, etc.) to a single server to increase its capacity.
- Horizontal Scalability (Scaling Out): This involves adding more servers to distribute the load across multiple machines.
In most modern web applications, horizontal scalability is preferred due to its flexibility and cost-effectiveness. However, a well-designed system often incorporates both vertical and horizontal scaling strategies.
2. Key Principles of Scalable Web Application Design
When designing a scalable web application, keep these fundamental principles in mind:
- Statelessness: Design your application to be stateless, where each request contains all the information needed to process it. This allows for easier horizontal scaling.
- Loose Coupling: Minimize dependencies between components to allow for independent scaling and easier maintenance.
- Asynchronous Processing: Use asynchronous communication and processing to improve responsiveness and resource utilization.
- Caching: Implement caching at various levels to reduce database load and improve response times.
- Data Partitioning: Distribute data across multiple servers to improve performance and scalability.
- Fault Tolerance: Design your system to handle failures gracefully and continue operating in degraded states.
- Automation: Automate deployment, scaling, and monitoring processes to reduce manual intervention and improve reliability.
3. Choosing the Right Architecture
Selecting the appropriate architecture is crucial for building a scalable web application. Here are some popular architectural patterns to consider:
3.1 N-Tier Architecture
N-Tier architecture separates an application into multiple logical layers, typically including:
- Presentation Layer (UI)
- Application Layer (Business Logic)
- Data Access Layer
- Database Layer
This separation allows for independent scaling of each layer and improved maintainability.
3.2 Microservices Architecture
Microservices architecture breaks down an application into small, independent services that communicate via APIs. This approach offers several benefits for scalability:
- Independent scaling of services
- Easier deployment and maintenance
- Technology diversity (different services can use different tech stacks)
- Improved fault isolation
3.3 Event-Driven Architecture
Event-driven architecture focuses on producing, detecting, and reacting to events. This pattern is particularly useful for building scalable, loosely coupled systems that can handle high volumes of real-time data.
4. Database Design and Optimization
Efficient database design is crucial for building scalable web applications. Consider the following strategies:
4.1 Database Sharding
Sharding involves partitioning data across multiple database servers. This horizontal partitioning can significantly improve performance and scalability by distributing the load across multiple machines.
4.2 Indexing
Properly indexed databases can dramatically improve query performance. Analyze your most common queries and create appropriate indexes to speed up data retrieval.
4.3 Denormalization
While normalization is important for data integrity, strategic denormalization can improve read performance by reducing the need for complex joins.
4.4 NoSQL Databases
Consider using NoSQL databases for specific use cases that require high scalability and flexibility, such as handling large volumes of unstructured data or real-time analytics.
5. Caching Strategies
Implementing effective caching strategies can significantly improve the performance and scalability of your web application. Consider the following caching levels:
5.1 Client-Side Caching
Utilize browser caching and local storage to reduce server requests for static assets and frequently accessed data.
5.2 CDN Caching
Use Content Delivery Networks (CDNs) to cache and serve static content from geographically distributed servers, reducing latency and server load.
5.3 Application-Level Caching
Implement in-memory caching solutions like Redis or Memcached to store frequently accessed data and reduce database load.
5.4 Database Caching
Utilize database query caching and result set caching to improve query performance and reduce load on the database server.
6. Load Balancing and Traffic Management
Load balancing is essential for distributing traffic across multiple servers and ensuring high availability. Consider the following load balancing techniques:
6.1 Round Robin
Distribute requests evenly across all available servers in a circular order.
6.2 Least Connections
Route new requests to the server with the least number of active connections.
6.3 IP Hash
Use the client’s IP address to determine which server to route the request to, ensuring that a client always connects to the same server.
6.4 Application-Aware Load Balancing
Implement more sophisticated load balancing algorithms that take into account factors like server health, response time, and resource utilization.
7. Microservices Architecture
Microservices architecture is a powerful approach for building scalable web applications. Here are some key considerations when implementing microservices:
7.1 Service Decomposition
Break down your application into small, focused services that can be developed, deployed, and scaled independently.
7.2 Inter-Service Communication
Choose appropriate communication patterns for your microservices, such as REST APIs, gRPC, or message queues.
7.3 Service Discovery
Implement service discovery mechanisms to allow services to locate and communicate with each other dynamically.
7.4 API Gateway
Use an API gateway to handle cross-cutting concerns like authentication, rate limiting, and request routing.
8. API Design for Scalability
Well-designed APIs are crucial for building scalable web applications. Consider the following best practices:
8.1 RESTful Design
Follow RESTful principles to create intuitive, scalable APIs that are easy to consume and maintain.
8.2 Versioning
Implement API versioning to allow for backwards compatibility and smooth transitions when introducing changes.
8.3 Rate Limiting
Implement rate limiting to prevent abuse and ensure fair usage of your API resources.
8.4 Pagination
Use pagination for large result sets to improve performance and reduce server load.
9. Monitoring and Logging
Effective monitoring and logging are essential for maintaining and scaling web applications. Consider the following aspects:
9.1 Application Performance Monitoring (APM)
Implement APM tools to track performance metrics, identify bottlenecks, and optimize your application.
9.2 Centralized Logging
Use centralized logging solutions to aggregate logs from multiple services and servers for easier troubleshooting and analysis.
9.3 Alerting
Set up alerts for critical issues and performance thresholds to enable proactive problem resolution.
9.4 Tracing
Implement distributed tracing to track requests across multiple services and identify performance issues in complex systems.
10. Security Considerations
Security is a crucial aspect of scalable web application design. Consider the following security measures:
10.1 Authentication and Authorization
Implement robust authentication and authorization mechanisms to protect user data and system resources.
10.2 HTTPS
Use HTTPS to encrypt all communication between clients and servers.
10.3 Input Validation
Implement thorough input validation to prevent common security vulnerabilities like SQL injection and cross-site scripting (XSS).
10.4 Rate Limiting and DDoS Protection
Implement rate limiting and DDoS protection measures to safeguard your application against abuse and attacks.
11. Leveraging Cloud Services
Cloud services can greatly simplify the process of building and scaling web applications. Consider the following cloud services:
11.1 Serverless Computing
Use serverless platforms like AWS Lambda or Azure Functions to build highly scalable, event-driven applications without managing infrastructure.
11.2 Managed Databases
Utilize managed database services like Amazon RDS or Google Cloud SQL to simplify database management and scaling.
11.3 Container Orchestration
Use container orchestration platforms like Kubernetes or Amazon ECS to manage and scale containerized applications.
11.4 Auto-scaling
Leverage cloud auto-scaling features to automatically adjust resources based on demand.
12. Case Study: Designing a Scalable Social Media Platform
Let’s apply the principles we’ve discussed to design a scalable social media platform. Here’s a high-level overview of the architecture:
12.1 Frontend
- Single-page application (SPA) built with React or Vue.js
- Served via CDN for global distribution and caching
12.2 Backend Services
- User Service: Handles user authentication and profile management
- Post Service: Manages creation and retrieval of user posts
- Timeline Service: Aggregates and serves user timelines
- Notification Service: Manages and delivers user notifications
12.3 Data Storage
- User data: Relational database (e.g., PostgreSQL) with read replicas
- Posts: NoSQL database (e.g., Cassandra) for high write throughput
- Media files: Object storage (e.g., Amazon S3) with CDN integration
12.4 Caching
- Redis for caching user sessions, timelines, and frequently accessed data
12.5 Message Queue
- Apache Kafka for handling events and asynchronous processing
12.6 Load Balancing
- Application load balancer to distribute traffic across backend services
12.7 Monitoring and Logging
- ELK stack (Elasticsearch, Logstash, Kibana) for centralized logging
- Prometheus and Grafana for monitoring and alerting
This architecture allows for independent scaling of different components, efficient data storage and retrieval, and high availability through redundancy and load balancing.
13. Interview Tips and Common Questions
When discussing scalable web application design in an interview, keep these tips in mind:
- Start with a high-level overview of the system architecture before diving into specific components.
- Discuss trade-offs between different design choices and explain your reasoning.
- Be prepared to estimate system capacity and resource requirements.
- Demonstrate knowledge of current industry trends and best practices.
Common interview questions on scalable web application design include:
- How would you design a URL shortening service like bit.ly?
- How would you implement a real-time chat application that can support millions of users?
- How would you design a system to handle millions of concurrent video streams?
- How would you design a distributed cache for a large-scale web application?
When answering these questions, focus on demonstrating your understanding of scalability principles, your ability to break down complex problems, and your knowledge of relevant technologies and design patterns.
14. Conclusion
Designing scalable web applications is a complex but essential skill for modern software engineers. By understanding and applying the principles and techniques discussed in this guide, you’ll be well-equipped to tackle scalability challenges in your projects and impress interviewers with your knowledge.
Remember that scalability is not just about handling large amounts of traffic or data; it’s about creating flexible, maintainable systems that can adapt to changing requirements and grow with your business. As you continue to learn and gain experience, you’ll develop an intuition for scalable design that will serve you well throughout your career.
Keep practicing, stay up-to-date with emerging technologies and best practices, and don’t hesitate to dive deep into specific areas that interest you. With dedication and continuous learning, you’ll be well on your way to mastering the art of scalable web application design.