Why Dynamic Programming Is a Common Focus in Interviews
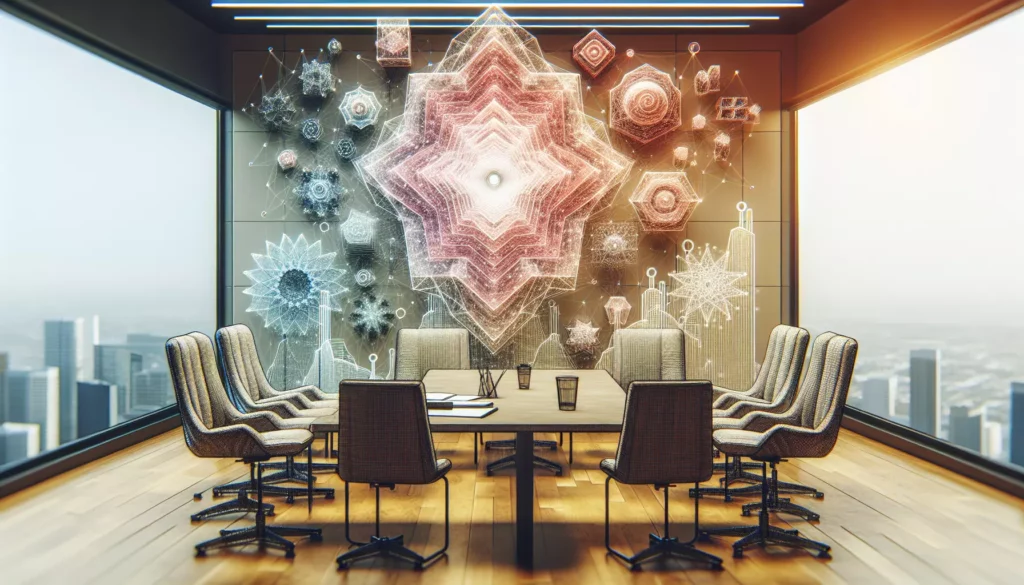
In the world of coding interviews, particularly those conducted by major tech companies like FAANG (Facebook, Amazon, Apple, Netflix, and Google), dynamic programming (DP) has emerged as a crucial topic that candidates are expected to master. This article delves into the reasons why dynamic programming is so frequently emphasized in technical interviews and why it’s an essential skill for aspiring software engineers to develop.
Understanding Dynamic Programming
Before we explore why dynamic programming is a common focus in interviews, let’s briefly recap what dynamic programming is and how it works.
Dynamic programming is an algorithmic paradigm that solves complex problems by breaking them down into simpler subproblems. It is a method for solving optimization problems by caching solutions to overlapping subproblems, thus avoiding redundant computations. The key idea behind dynamic programming is to store the results of subproblems so that we don’t have to recompute them when needed later.
The two main properties that a problem must have for dynamic programming to be applicable are:
- Optimal substructure: An optimal solution to the problem contains optimal solutions to subproblems.
- Overlapping subproblems: The problem can be broken down into subproblems which are reused several times.
Why Interviewers Love Dynamic Programming Questions
Now that we have a basic understanding of dynamic programming, let’s explore why it’s such a popular topic in coding interviews:
1. Demonstrates Problem-Solving Skills
Dynamic programming questions are excellent for assessing a candidate’s problem-solving abilities. These problems often require a deep understanding of the problem statement, the ability to break down complex issues into manageable subproblems, and the skill to recognize patterns and relationships between these subproblems.
When a candidate successfully solves a DP problem, it shows that they can:
- Analyze problems thoroughly
- Identify recursive relationships
- Optimize solutions by avoiding redundant computations
- Think critically about space and time complexity
2. Tests Algorithmic Thinking
Dynamic programming is a powerful algorithmic technique that goes beyond simple brute-force approaches. By asking DP questions, interviewers can gauge a candidate’s ability to think algorithmically and come up with efficient solutions to complex problems.
Solving DP problems requires:
- Recognizing when to apply dynamic programming
- Formulating recurrence relations
- Deciding between top-down (memoization) and bottom-up (tabulation) approaches
- Optimizing space complexity through state reduction techniques
3. Evaluates Code Optimization Skills
Dynamic programming often leads to significant improvements in time complexity compared to naive recursive solutions. Interviewers use DP questions to assess a candidate’s ability to optimize code and improve algorithmic efficiency.
For example, consider the Fibonacci sequence problem:
def fibonacci(n):
if n <= 1:
return n
return fibonacci(n-1) + fibonacci(n-2)
This naive recursive solution has a time complexity of O(2^n). However, with dynamic programming, we can optimize it to O(n):
def fibonacci_dp(n):
if n <= 1:
return n
dp = [0] * (n + 1)
dp[1] = 1
for i in range(2, n + 1):
dp[i] = dp[i-1] + dp[i-2]
return dp[n]
By asking candidates to optimize such solutions, interviewers can evaluate their ability to improve code performance dramatically.
4. Assesses Analytical and Mathematical Skills
Many dynamic programming problems have a strong mathematical foundation. They often involve concepts from combinatorics, probability, or graph theory. By posing DP questions, interviewers can assess a candidate’s analytical and mathematical prowess.
For instance, the “Coin Change” problem is a classic DP question that requires both mathematical intuition and programming skills:
def coin_change(coins, amount):
dp = [float('inf')] * (amount + 1)
dp[0] = 0
for coin in coins:
for x in range(coin, amount + 1):
dp[x] = min(dp[x], dp[x - coin] + 1)
return dp[amount] if dp[amount] != float('inf') else -1
This problem requires candidates to think about the mathematical relationship between different coin denominations and the target amount, while also implementing an efficient algorithm.
5. Tests Ability to Handle Complex Problems
Dynamic programming problems are often more complex than simple data structure manipulations or basic algorithmic questions. They require candidates to juggle multiple concepts simultaneously, such as recursion, memoization, and state transitions.
By presenting DP problems, interviewers can evaluate how well candidates handle complexity and whether they can break down intricate problems into manageable components.
6. Reveals Coding Style and Clean Code Practices
Dynamic programming solutions often involve multiple steps: identifying the base case, formulating the recurrence relation, and implementing the solution (either top-down or bottom-up). This multi-step process allows interviewers to assess a candidate’s coding style, including:
- Code organization and structure
- Variable naming conventions
- Comment clarity and documentation
- Ability to write modular and reusable code
For example, consider this well-structured solution to the “Longest Common Subsequence” problem:
def longest_common_subsequence(text1, text2):
m, n = len(text1), len(text2)
dp = [[0] * (n + 1) for _ in range(m + 1)]
# Fill the dp table
for i in range(1, m + 1):
for j in range(1, n + 1):
if text1[i-1] == text2[j-1]:
dp[i][j] = dp[i-1][j-1] + 1
else:
dp[i][j] = max(dp[i-1][j], dp[i][j-1])
# Reconstruct the LCS
lcs = []
i, j = m, n
while i > 0 and j > 0:
if text1[i-1] == text2[j-1]:
lcs.append(text1[i-1])
i -= 1
j -= 1
elif dp[i-1][j] > dp[i][j-1]:
i -= 1
else:
j -= 1
return ''.join(reversed(lcs))
This solution demonstrates clean code practices, clear variable names, and a logical structure that separates the DP table filling from the LCS reconstruction.
7. Evaluates Time and Space Complexity Analysis
Dynamic programming solutions often involve trade-offs between time and space complexity. Interviewers use DP questions to assess a candidate’s ability to analyze and optimize both time and space complexity.
For example, the “0/1 Knapsack” problem can be solved using different approaches with varying space complexities:
# 2D DP solution (O(n*W) time and space)
def knapsack_2d(values, weights, capacity):
n = len(values)
dp = [[0] * (capacity + 1) for _ in range(n + 1)]
for i in range(1, n + 1):
for w in range(1, capacity + 1):
if weights[i-1] <= w:
dp[i][w] = max(dp[i-1][w], values[i-1] + dp[i-1][w-weights[i-1]])
else:
dp[i][w] = dp[i-1][w]
return dp[n][capacity]
# 1D DP solution (O(n*W) time, O(W) space)
def knapsack_1d(values, weights, capacity):
n = len(values)
dp = [0] * (capacity + 1)
for i in range(n):
for w in range(capacity, weights[i] - 1, -1):
dp[w] = max(dp[w], values[i] + dp[w - weights[i]])
return dp[capacity]
By discussing these different approaches, candidates can demonstrate their understanding of space-time trade-offs and their ability to optimize solutions based on specific constraints.
Real-World Applications of Dynamic Programming
Another reason why dynamic programming is a common focus in interviews is its wide range of real-world applications. Companies often use DP in their products and services, making it an essential skill for software engineers. Some practical applications include:
1. Resource Allocation and Optimization
Dynamic programming is frequently used in resource allocation problems, such as:
- Optimizing cloud computing resource allocation
- Managing inventory and supply chain logistics
- Scheduling tasks in operating systems
2. Natural Language Processing
DP algorithms are used in various NLP tasks, including:
- Speech recognition
- Machine translation
- Text summarization
3. Bioinformatics
Dynamic programming is crucial in bioinformatics for tasks such as:
- Sequence alignment (e.g., DNA, RNA, or protein sequences)
- Gene prediction
- Protein folding simulation
4. Financial Modeling
DP is used in finance for various purposes, including:
- Option pricing
- Portfolio optimization
- Risk management
5. Computer Graphics
Dynamic programming finds applications in computer graphics, such as:
- Image segmentation
- Curve fitting
- 3D rendering optimizations
How to Prepare for Dynamic Programming Interview Questions
Given the importance of dynamic programming in coding interviews, it’s crucial for candidates to prepare thoroughly. Here are some tips to help you master DP:
1. Understand the Fundamentals
Start by gaining a solid understanding of the core concepts of dynamic programming, including:
- Optimal substructure
- Overlapping subproblems
- Memoization vs. tabulation
- State transition
2. Practice Classic DP Problems
Familiarize yourself with common DP problems, such as:
- Fibonacci sequence
- Longest Common Subsequence
- Knapsack problem
- Coin Change
- Edit Distance
- Longest Increasing Subsequence
3. Learn to Recognize DP Patterns
Identify common patterns in DP problems, such as:
- Linear DP
- Matrix DP
- Interval DP
- Tree DP
- State compression DP
4. Practice Formulating Recurrence Relations
Work on your ability to formulate recurrence relations, which are the heart of dynamic programming solutions. For example, the recurrence relation for the Fibonacci sequence is:
F(n) = F(n-1) + F(n-2), where F(0) = 0 and F(1) = 1
5. Implement Both Top-Down and Bottom-Up Approaches
Practice implementing both memoization (top-down) and tabulation (bottom-up) approaches for DP problems. Understanding the pros and cons of each method will help you choose the best approach for different scenarios.
6. Analyze Time and Space Complexity
For each DP solution you implement, make sure to analyze its time and space complexity. This will help you understand the efficiency of your solutions and identify areas for optimization.
7. Use Visualization Tools
Utilize visualization tools to help you understand how DP algorithms work. Websites like VisuAlgo offer interactive visualizations of various algorithms, including some DP problems.
8. Solve Problems on Coding Platforms
Practice solving DP problems on coding platforms like LeetCode, HackerRank, or CodeForces. These platforms offer a wide range of problems with varying difficulty levels, allowing you to gradually build your skills.
9. Participate in Mock Interviews
Engage in mock interviews with friends, mentors, or through online platforms. This will help you get comfortable explaining your thought process and implementing DP solutions under time pressure.
10. Review and Reflect
After solving each DP problem, take time to review your solution and reflect on what you’ve learned. Consider alternative approaches and optimizations you could have used.
Conclusion
Dynamic programming is a common focus in coding interviews for good reasons. It allows interviewers to assess a candidate’s problem-solving skills, algorithmic thinking, code optimization abilities, and capacity to handle complex problems. Moreover, DP has numerous real-world applications across various industries, making it an essential skill for software engineers.
By understanding why DP is emphasized in interviews and following the preparation tips outlined in this article, you can improve your chances of success in technical interviews, particularly those conducted by major tech companies. Remember that mastering dynamic programming is not just about memorizing solutions to specific problems, but about developing a problem-solving mindset that can be applied to a wide range of algorithmic challenges.
As you continue your journey in software engineering and interview preparation, keep in mind that dynamic programming is just one of many important topics. A well-rounded understanding of data structures, algorithms, system design, and other computer science fundamentals will be crucial for your success in technical interviews and your career as a software engineer.