How to Solve Coding Interview Problems Using Recursion
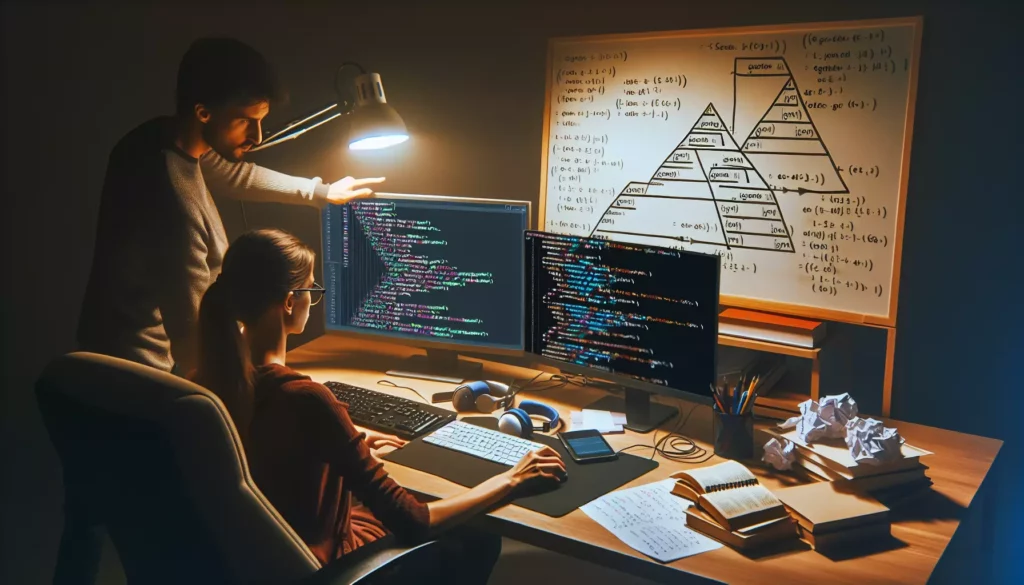
Recursion is a powerful problem-solving technique that’s often used in coding interviews, especially at top tech companies like FAANG (Facebook, Amazon, Apple, Netflix, Google). Understanding and mastering recursion can significantly boost your chances of success in these high-stakes interviews. In this comprehensive guide, we’ll explore how to approach and solve coding interview problems using recursion, providing you with the tools and strategies you need to excel.
What is Recursion?
Before diving into problem-solving strategies, let’s quickly review what recursion is. Recursion is a programming technique where a function calls itself to solve a problem. It’s based on the principle of breaking down a complex problem into smaller, more manageable subproblems. The function continues to call itself with these smaller subproblems until it reaches a base case, which is a simple enough problem that can be solved directly without further recursion.
The general structure of a recursive function looks like this:
def recursive_function(parameters):
if base_case_condition:
return base_case_solution
else:
return recursive_function(modified_parameters)
Why is Recursion Important in Coding Interviews?
Recursion is a favorite topic among interviewers for several reasons:
- It demonstrates your ability to think algorithmically and break down complex problems.
- Many problems, especially those involving trees, graphs, and divide-and-conquer algorithms, have elegant recursive solutions.
- Understanding recursion often indicates a deeper grasp of programming concepts.
- It can lead to more concise and readable code for certain types of problems.
Steps to Solve Recursive Problems in Coding Interviews
When faced with a problem that might be solved recursively, follow these steps:
1. Identify the Base Case
The base case is the simplest form of the problem that can be solved directly. It’s crucial to identify this correctly as it’s what stops the recursion. For example, in a factorial function, the base case is when n = 0 or 1, as factorial(0) = factorial(1) = 1.
2. Define the Recursive Case
This is where you break down the problem into a smaller subproblem. You need to ensure that each recursive call moves closer to the base case. In the factorial example, we can define n! as n * (n-1)!.
3. Ensure the Recursion Terminates
Make sure your recursive calls will eventually reach the base case. If not, you’ll end up with infinite recursion and a stack overflow.
4. Combine the Results
Determine how to combine the results of the recursive calls to solve the original problem.
Common Recursive Patterns in Coding Interviews
Several recursive patterns frequently appear in coding interviews. Familiarizing yourself with these can help you recognize when to apply recursion and how to structure your solution.
1. Linear Recursion
In linear recursion, the function makes one recursive call each time it’s invoked. Examples include calculating factorial, Fibonacci numbers, or traversing a linked list.
Here’s an example of calculating factorial using linear recursion:
def factorial(n):
if n == 0 or n == 1:
return 1
else:
return n * factorial(n - 1)
2. Binary Recursion
Binary recursion involves making two recursive calls in each function invocation. This is common in problems involving binary trees or divide-and-conquer algorithms.
Here’s an example of binary recursion to calculate Fibonacci numbers:
def fibonacci(n):
if n <= 1:
return n
else:
return fibonacci(n - 1) + fibonacci(n - 2)
3. Tail Recursion
In tail recursion, the recursive call is the last operation in the function. This can be optimized by many compilers to use constant stack space.
Here’s the factorial function rewritten with tail recursion:
def factorial_tail(n, accumulator=1):
if n == 0 or n == 1:
return accumulator
else:
return factorial_tail(n - 1, n * accumulator)
4. Mutual Recursion
Mutual recursion involves two or more functions calling each other recursively. This is less common but can be useful in certain scenarios.
Here’s an example of mutual recursion to determine if a number is even or odd:
def is_even(n):
if n == 0:
return True
else:
return is_odd(n - 1)
def is_odd(n):
if n == 0:
return False
else:
return is_even(n - 1)
Common Recursive Problems in Coding Interviews
Let’s look at some common recursive problems you might encounter in coding interviews and how to approach them.
1. Tree Traversal
Tree traversal is a classic recursive problem. Here’s an example of in-order traversal of a binary tree:
class TreeNode:
def __init__(self, val=0, left=None, right=None):
self.val = val
self.left = left
self.right = right
def inorder_traversal(root):
if not root:
return []
return inorder_traversal(root.left) + [root.val] + inorder_traversal(root.right)
2. Merge Sort
Merge sort is a divide-and-conquer algorithm that uses recursion:
def merge_sort(arr):
if len(arr) <= 1:
return arr
mid = len(arr) // 2
left = merge_sort(arr[:mid])
right = merge_sort(arr[mid:])
return merge(left, right)
def merge(left, right):
result = []
i, j = 0, 0
while i < len(left) and j < len(right):
if left[i] < right[j]:
result.append(left[i])
i += 1
else:
result.append(right[j])
j += 1
result.extend(left[i:])
result.extend(right[j:])
return result
3. Tower of Hanoi
The Tower of Hanoi is a classic problem that’s elegantly solved with recursion:
def tower_of_hanoi(n, source, auxiliary, destination):
if n == 1:
print(f"Move disk 1 from {source} to {destination}")
return
tower_of_hanoi(n-1, source, destination, auxiliary)
print(f"Move disk {n} from {source} to {destination}")
tower_of_hanoi(n-1, auxiliary, source, destination)
4. Generating Subsets
Generating all subsets of a set is another common recursive problem:
def generate_subsets(nums):
def backtrack(start, current):
result.append(current[:])
for i in range(start, len(nums)):
current.append(nums[i])
backtrack(i + 1, current)
current.pop()
result = []
backtrack(0, [])
return result
Tips for Solving Recursive Problems in Interviews
Here are some tips to help you tackle recursive problems effectively in coding interviews:
1. Trust the Recursion
One of the biggest challenges with recursion is trusting that it will work. Don’t try to follow the entire recursive process in your head. Instead, focus on ensuring that:
- The base case is correct
- The recursive case breaks down the problem correctly
- The recursive calls move towards the base case
2. Start with the Base Case
Always start by defining the base case. This is the foundation of your recursive function and will prevent infinite recursion.
3. Think About the Current Step
Focus on what needs to happen at the current step, assuming the recursive calls will correctly solve the smaller subproblems.
4. Avoid Redundant Calculations
Some recursive solutions, like the naive Fibonacci implementation, can be very inefficient due to redundant calculations. Consider using memoization or dynamic programming to optimize these cases.
5. Consider the Call Stack
Remember that each recursive call adds a new frame to the call stack. For problems with deep recursion, consider iterative solutions or tail recursion to avoid stack overflow.
6. Practice, Practice, Practice
The key to mastering recursion is practice. Solve a variety of recursive problems to build your intuition and problem-solving skills.
Common Pitfalls to Avoid
When working with recursion, be aware of these common pitfalls:
1. Infinite Recursion
Ensure your recursive calls always move towards the base case. Infinite recursion will cause a stack overflow error.
2. Missing Base Case
Always define a base case. Without it, your recursion will never terminate.
3. Incorrect Base Case
An incorrect base case can lead to wrong results or infinite recursion. Double-check your base cases.
4. Unnecessary Recursion
Sometimes, an iterative solution might be simpler or more efficient. Don’t force recursion if it’s not the best approach.
5. Inefficient Recursion
Be mindful of the time and space complexity of your recursive solution. Some naive recursive implementations can be extremely inefficient.
Advanced Recursive Techniques
As you become more comfortable with basic recursion, you can explore more advanced techniques:
1. Memoization
Memoization involves caching the results of expensive function calls to avoid redundant calculations. This can significantly improve the efficiency of recursive algorithms.
Here’s an example of memoized Fibonacci:
def fibonacci_memo(n, memo={}):
if n in memo:
return memo[n]
if n <= 1:
return n
memo[n] = fibonacci_memo(n-1, memo) + fibonacci_memo(n-2, memo)
return memo[n]
2. Tail Call Optimization
Tail call optimization is a technique where the compiler optimizes recursive calls that are in tail position (the last operation in the function). This can prevent stack overflow for deep recursion.
3. Continuation-Passing Style
Continuation-passing style is a programming technique where the control is passed explicitly in the form of a continuation. This can be used to implement recursion in a way that’s amenable to tail call optimization.
Conclusion
Mastering recursion is a crucial skill for acing coding interviews, especially at top tech companies. By understanding the core concepts, recognizing common patterns, and practicing with a variety of problems, you can develop the confidence and skill to tackle recursive problems effectively.
Remember, the key to recursion is breaking down complex problems into simpler subproblems, defining clear base cases, and trusting the recursive process. With practice, you’ll find that many problems that seem complex at first glance can be elegantly solved using recursion.
As you prepare for your coding interviews, make sure to practice a wide range of recursive problems. Platforms like AlgoCademy offer a wealth of resources, including interactive coding tutorials and AI-powered assistance, to help you hone your recursion skills and prepare for technical interviews at major tech companies.
Happy coding, and best of luck with your interviews!