The Importance of Practicing Common Interview Questions
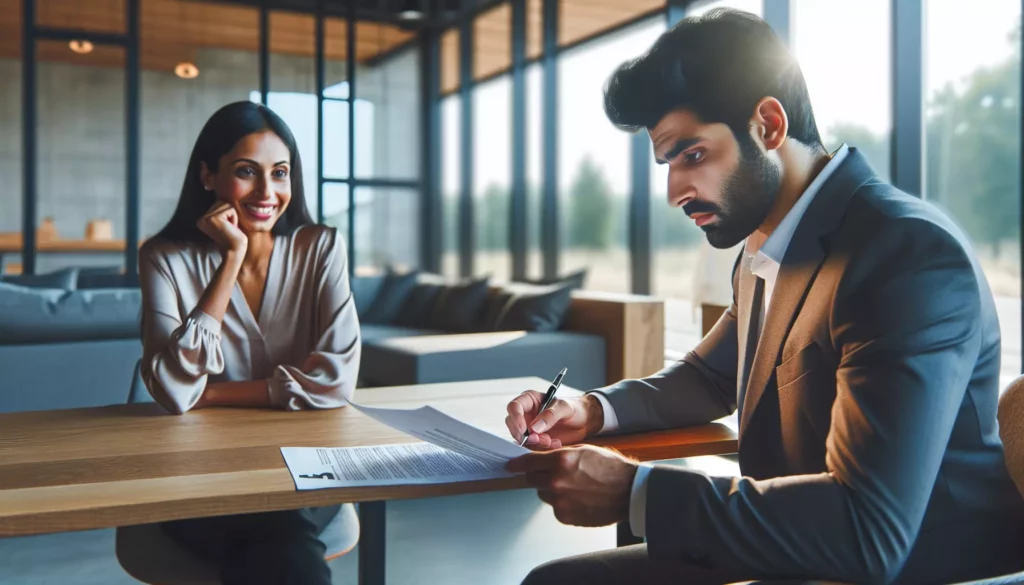
In the competitive world of tech, landing your dream job often hinges on your performance during technical interviews. Whether you’re aiming for a position at a FAANG company (Facebook, Amazon, Apple, Netflix, Google) or any other tech giant, one thing is certain: preparation is key. A crucial aspect of this preparation involves practicing common interview questions. In this comprehensive guide, we’ll explore why this practice is so important and how it can significantly boost your chances of success.
Why Practicing Common Interview Questions Matters
Before diving into the specifics, let’s understand why dedicating time to practice common interview questions is a game-changer for aspiring developers:
- Builds Confidence: Familiarity with common questions reduces anxiety and boosts confidence during the actual interview.
- Improves Problem-Solving Skills: Regular practice enhances your ability to approach and solve complex problems efficiently.
- Reveals Knowledge Gaps: Working through various questions helps identify areas where you need to strengthen your understanding.
- Enhances Communication Skills: Practicing verbalization of your thought process improves your ability to explain complex concepts clearly.
- Saves Time During Interviews: Familiarity with common patterns allows you to tackle questions more quickly, leaving time for more challenging problems.
Common Types of Interview Questions
To make the most of your practice sessions, it’s essential to understand the different types of questions you might encounter. Here are some common categories:
1. Data Structure Questions
These questions test your understanding of fundamental data structures like arrays, linked lists, trees, and graphs. For example:
- Implement a stack using two queues
- Reverse a linked list
- Find the height of a binary tree
- Detect a cycle in a directed graph
2. Algorithm Questions
Algorithm questions assess your ability to design efficient solutions to computational problems. Common topics include:
- Sorting and searching algorithms
- Dynamic programming
- Greedy algorithms
- Backtracking
3. System Design Questions
For more senior positions, you might encounter system design questions that test your ability to architect large-scale systems. Examples include:
- Design a URL shortening service like bit.ly
- Create a basic version of Twitter
- Design a parking lot system
4. Coding Implementation Questions
These questions require you to write actual code to solve a given problem. They test both your problem-solving skills and your ability to translate ideas into working code. For instance:
- Implement a function to check if a string is a palindrome
- Write a program to find the nth Fibonacci number
- Implement a basic calculator that can perform addition, subtraction, multiplication, and division
Strategies for Effective Practice
Now that we understand the types of questions you might face, let’s explore some strategies to make your practice sessions more effective:
1. Create a Study Plan
Develop a structured study plan that covers all the essential topics. Allocate specific time slots for different types of questions and ensure you’re giving adequate attention to each area.
2. Use Online Platforms
Leverage online coding platforms like LeetCode, HackerRank, or AlgoCademy. These platforms offer a wide range of problems, often categorized by difficulty and topic, making it easier to focus on specific areas of improvement.
3. Time Your Practice Sessions
Many technical interviews have time constraints. Practice solving problems within a set time limit to improve your speed and efficiency.
4. Implement Solutions from Scratch
While it’s tempting to look at solutions when stuck, try to implement solutions from scratch as much as possible. This builds your problem-solving muscles and helps you internalize different approaches.
5. Review and Reflect
After solving a problem, take time to review your solution. Ask yourself:
- Is this the most efficient solution?
- Can I explain my approach clearly?
- Are there any edge cases I haven’t considered?
6. Practice Explaining Your Thought Process
In many interviews, the interviewer is as interested in your thought process as they are in your final solution. Practice explaining your approach out loud as you solve problems.
7. Collaborate with Others
Join coding communities or find a study buddy. Explaining concepts to others and hearing different perspectives can deepen your understanding and expose you to new problem-solving approaches.
Common Pitfalls to Avoid
While practicing common interview questions is crucial, there are some pitfalls you should be aware of:
1. Memorizing Solutions
While it’s important to recognize patterns, avoid simply memorizing solutions. Interviewers can often tell when a candidate is reciting a memorized answer rather than truly understanding the problem.
2. Neglecting Fundamentals
Don’t get so caught up in solving complex problems that you neglect the basics. Make sure you have a solid grasp of fundamental concepts like time and space complexity, basic data structures, and core algorithms.
3. Ignoring Soft Skills
Technical skills are crucial, but don’t forget about soft skills. Practice communicating clearly, handling pressure, and collaborating effectively.
4. Focusing Only on Coding
While coding questions are important, don’t neglect other aspects of the interview process, such as behavioral questions or system design for more senior roles.
Sample Practice Question: Two Sum
Let’s walk through a common interview question to illustrate how you might approach practice:
Problem: Given an array of integers nums
and an integer target
, return indices of the two numbers such that they add up to target
.
Example:
Input: nums = [2,7,11,15], target = 9
Output: [0,1]
Explanation: Because nums[0] + nums[1] == 9, we return [0, 1].
Approach:
- We can use a hash map to solve this problem efficiently.
- Iterate through the array once, for each element:
- Calculate the complement (target – current number)
- If the complement exists in the hash map, we’ve found our pair
- If not, add the current number and its index to the hash map
Python Implementation:
def two_sum(nums, target):
num_map = {}
for i, num in enumerate(nums):
complement = target - num
if complement in num_map:
return [num_map[complement], i]
num_map[num] = i
return [] # No solution found
# Test the function
print(two_sum([2,7,11,15], 9)) # Should print [0, 1]
Time Complexity: O(n), where n is the length of the input array.
Space Complexity: O(n) to store the hash map.
The Role of AI in Interview Preparation
As technology advances, AI is playing an increasingly significant role in interview preparation. Platforms like AlgoCademy are leveraging AI to provide personalized learning experiences and targeted practice sessions. Here’s how AI can enhance your interview preparation:
1. Personalized Problem Recommendations
AI algorithms can analyze your performance on practice problems and recommend questions that target your weak areas. This ensures that you’re always challenging yourself and improving where it matters most.
2. Real-time Feedback
Advanced AI systems can provide instant feedback on your code, pointing out potential optimizations, suggesting alternative approaches, and even catching subtle bugs that you might have missed.
3. Simulated Interview Experiences
Some platforms use AI to simulate realistic interview scenarios, complete with timed coding challenges and even virtual interviewers that can ask follow-up questions. This helps you get comfortable with the pressure and pacing of real interviews.
4. Adaptive Learning Paths
AI can create personalized learning paths that adapt to your progress. As you master certain concepts, the system can automatically introduce more advanced topics, ensuring that your learning remains challenging and relevant.
5. Natural Language Processing for Explanation Practice
Advanced AI systems can evaluate your verbal or written explanations of your problem-solving approach, helping you improve your ability to communicate technical concepts clearly.
Beyond Technical Questions: Holistic Interview Preparation
While mastering technical questions is crucial, it’s important to remember that interviews, especially at top tech companies, often involve more than just coding challenges. Here are some additional areas to focus on in your preparation:
1. Behavioral Questions
Many companies, including FAANG, place a high value on cultural fit and soft skills. Prepare for questions like:
- “Tell me about a time when you faced a significant challenge in a project.”
- “How do you handle disagreements with team members?”
- “Describe a situation where you had to meet a tight deadline.”
Practice using the STAR method (Situation, Task, Action, Result) to structure your responses to these types of questions.
2. Company Research
Demonstrate your genuine interest in the company by researching:
- The company’s products and services
- Recent news or developments
- The company’s culture and values
- Potential challenges or opportunities in their industry
3. Questions for the Interviewer
Prepare thoughtful questions to ask your interviewer. This shows your engagement and helps you gather valuable information about the role and company. Some examples:
- “What does success look like in this role?”
- “Can you tell me about the team I’d be working with?”
- “What are the biggest challenges facing the department right now?”
4. Mock Interviews
Conduct mock interviews with friends, mentors, or through professional services. This helps you:
- Get comfortable with the interview format
- Practice explaining your thought process out loud
- Receive feedback on your communication and problem-solving approach
Maintaining Long-term Growth
While intense preparation leading up to interviews is important, it’s equally crucial to maintain a mindset of continuous learning and growth. Here are some strategies for long-term success:
1. Stay Current with Technology Trends
The tech industry evolves rapidly. Make it a habit to:
- Read tech blogs and news sites
- Attend conferences or webinars
- Experiment with new technologies in personal projects
2. Contribute to Open Source Projects
Contributing to open source not only improves your skills but also demonstrates your ability to work on real-world projects and collaborate with other developers.
3. Build a Strong Online Presence
Maintain an active GitHub profile, contribute to tech forums, or write a blog about your coding journey. This can make you stand out to potential employers.
4. Network
Build relationships within the tech community. Attend meetups, join online forums, and connect with professionals in your field. Networking can lead to opportunities and valuable insights.
5. Continuous Learning
Set aside time regularly to learn new skills or deepen existing ones. This could involve taking online courses, working through coding challenges, or exploring new programming languages.
Conclusion
Practicing common interview questions is an essential step in preparing for technical interviews, especially when aiming for positions at top tech companies. It builds confidence, sharpens problem-solving skills, and helps you identify areas for improvement. By combining structured practice with a holistic approach to interview preparation and a commitment to long-term growth, you’ll be well-equipped to tackle even the most challenging technical interviews.
Remember, the goal isn’t just to memorize solutions, but to develop a deep understanding of fundamental concepts and problem-solving strategies. With consistent practice and the right mindset, you’ll be well on your way to acing your next technical interview and landing your dream job in the tech industry.
Happy coding, and best of luck with your interview preparation!