How to Master Data Structures for Coding Interviews: A Comprehensive Guide
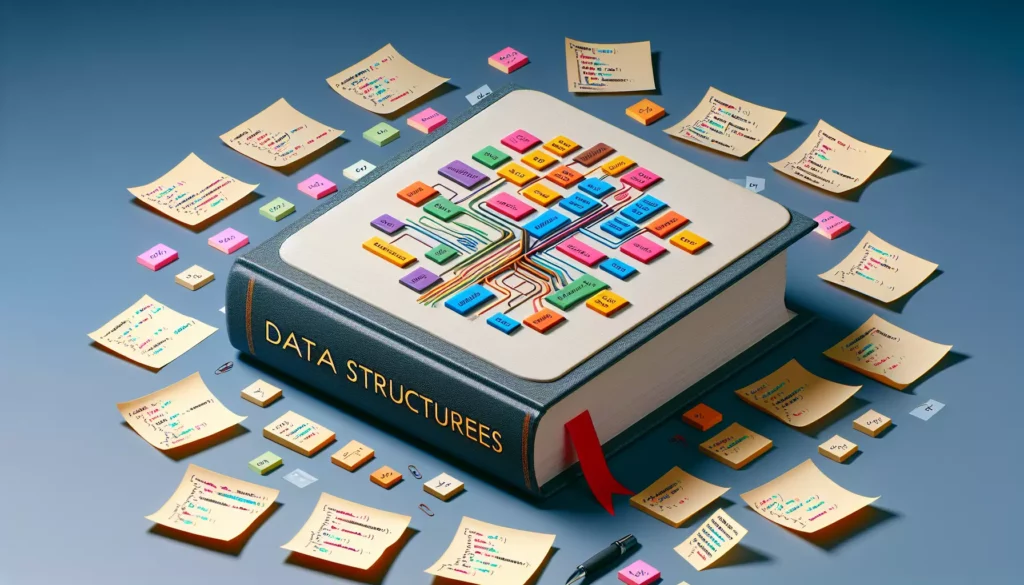
Data structures are the building blocks of efficient algorithms and are crucial for success in coding interviews. Whether you’re aiming for a position at a FAANG company (Facebook, Amazon, Apple, Netflix, Google) or any other tech giant, mastering data structures is non-negotiable. In this comprehensive guide, we’ll explore the most important data structures, their applications, and strategies to help you excel in your coding interviews.
Table of Contents
- Introduction to Data Structures
- Arrays and Dynamic Arrays
- Linked Lists
- Stacks and Queues
- Hash Tables
- Trees and Binary Search Trees
- Heaps
- Graphs
- Advanced Data Structures
- Practice Strategies
- Interview Tips and Tricks
- Conclusion
1. Introduction to Data Structures
Data structures are specialized formats for organizing, processing, retrieving, and storing data. They provide a way to manage large amounts of data efficiently for uses such as large databases and internet indexing services. Understanding data structures is crucial because they are the foundation of efficient algorithms and software design.
The choice of data structure often impacts the efficiency of an algorithm. For instance, using an array for frequent insertions and deletions might be less efficient than using a linked list. Similarly, using a hash table for quick lookups is generally more efficient than using an unsorted array.
In coding interviews, you’ll often be asked to solve problems that require you to choose and implement the most appropriate data structure. This is why mastering various data structures is essential for interview success.
2. Arrays and Dynamic Arrays
Arrays are one of the most fundamental data structures. They store elements in contiguous memory locations, allowing for constant-time access to elements using their indices.
Key Characteristics of Arrays:
- Fixed size (for static arrays)
- Constant-time access to elements: O(1)
- Efficient for random access
- Poor for insertions and deletions, especially in the middle: O(n)
Dynamic arrays (like ArrayList in Java or vector in C++) can grow or shrink in size. They provide more flexibility than static arrays but may have occasional performance hits when resizing.
Common Array Operations and Their Time Complexities:
- Access: O(1)
- Search: O(n) for unsorted, O(log n) for sorted (using binary search)
- Insertion: O(n)
- Deletion: O(n)
Example: Implementing a Dynamic Array in Python
class DynamicArray:
def __init__(self):
self.array = []
self.size = 0
self.capacity = 1
def append(self, element):
if self.size == self.capacity:
self._resize(2 * self.capacity)
self.array[self.size] = element
self.size += 1
def _resize(self, new_capacity):
new_array = [None] * new_capacity
for i in range(self.size):
new_array[i] = self.array[i]
self.array = new_array
self.capacity = new_capacity
def __getitem__(self, index):
if 0 <= index < self.size:
return self.array[index]
raise IndexError('Index out of range')
def __len__(self):
return self.size
Arrays are often used in interview questions involving string manipulation, matrix operations, and as the underlying structure for other data structures like stacks and queues.
3. Linked Lists
Linked lists are linear data structures where elements are stored in nodes. Each node contains a data field and a reference (or link) to the next node in the sequence.
Key Characteristics of Linked Lists:
- Dynamic size
- Efficient insertions and deletions
- No random access; sequential access only
- Extra memory space for link
Types of Linked Lists:
- Singly Linked List: Each node has a link to the next node
- Doubly Linked List: Each node has links to both the next and previous nodes
- Circular Linked List: The last node points back to the first node
Common Linked List Operations and Their Time Complexities:
- Access: O(n)
- Search: O(n)
- Insertion at beginning: O(1)
- Insertion at end: O(n) for singly linked list, O(1) for doubly linked list with tail pointer
- Deletion: O(1) if we have a reference to the node to be deleted, otherwise O(n)
Example: Implementing a Singly Linked List in Python
class Node:
def __init__(self, data):
self.data = data
self.next = None
class LinkedList:
def __init__(self):
self.head = None
def append(self, data):
new_node = Node(data)
if not self.head:
self.head = new_node
return
current = self.head
while current.next:
current = current.next
current.next = new_node
def print_list(self):
current = self.head
while current:
print(current.data, end=' -> ')
current = current.next
print('None')
def delete_node(self, key):
current = self.head
if current and current.data == key:
self.head = current.next
return
prev = None
while current and current.data != key:
prev = current
current = current.next
if current is None:
return
prev.next = current.next
Linked lists are often used in interview questions involving list manipulation, implementing other data structures like stacks and queues, and problems that require frequent insertions or deletions.
4. Stacks and Queues
Stacks and queues are abstract data types that can be implemented using arrays or linked lists. They are fundamental in many algorithms and are often used in interview questions.
Stacks
A stack follows the Last-In-First-Out (LIFO) principle. The last element added to the stack will be the first one to be removed.
Key Operations:
- Push: Add an element to the top of the stack
- Pop: Remove the top element from the stack
- Peek or Top: Get the top element without removing it
- IsEmpty: Check if the stack is empty
Time Complexity:
- Push: O(1)
- Pop: O(1)
- Peek: O(1)
Example: Implementing a Stack in Python
class Stack:
def __init__(self):
self.items = []
def push(self, item):
self.items.append(item)
def pop(self):
if not self.is_empty():
return self.items.pop()
def peek(self):
if not self.is_empty():
return self.items[-1]
def is_empty(self):
return len(self.items) == 0
def size(self):
return len(self.items)
Queues
A queue follows the First-In-First-Out (FIFO) principle. The first element added to the queue will be the first one to be removed.
Key Operations:
- Enqueue: Add an element to the rear of the queue
- Dequeue: Remove the front element from the queue
- Front: Get the front element without removing it
- IsEmpty: Check if the queue is empty
Time Complexity:
- Enqueue: O(1)
- Dequeue: O(1)
- Front: O(1)
Example: Implementing a Queue in Python
from collections import deque
class Queue:
def __init__(self):
self.items = deque()
def enqueue(self, item):
self.items.append(item)
def dequeue(self):
if not self.is_empty():
return self.items.popleft()
def front(self):
if not self.is_empty():
return self.items[0]
def is_empty(self):
return len(self.items) == 0
def size(self):
return len(self.items)
Stacks and queues are often used in interview questions involving expression evaluation, graph traversals (DFS uses a stack, BFS uses a queue), and implementing other algorithms like undo functionality (stack) or managing tasks (queue).
5. Hash Tables
Hash tables, also known as hash maps or dictionaries, are data structures that implement an associative array abstract data type, a structure that can map keys to values. They use a hash function to compute an index into an array of buckets or slots, from which the desired value can be found.
Key Characteristics of Hash Tables:
- Fast lookups: Average case O(1) for search, insert, and delete
- Unordered
- Key-value pairs
- May have collisions that need to be handled
Common Hash Table Operations and Their Time Complexities:
- Insert: Average O(1), Worst O(n)
- Delete: Average O(1), Worst O(n)
- Search: Average O(1), Worst O(n)
Example: Using a Hash Table in Python
# In Python, dictionaries are implemented as hash tables
hash_table = {}
# Insertion
hash_table['key1'] = 'value1'
hash_table['key2'] = 'value2'
# Lookup
print(hash_table['key1']) # Output: value1
# Deletion
del hash_table['key2']
# Check if key exists
if 'key2' in hash_table:
print("Key exists")
else:
print("Key does not exist")
# Iteration
for key, value in hash_table.items():
print(f"{key}: {value}")
Hash tables are extremely useful in solving many types of coding problems efficiently. They are often used in interview questions involving:
- Finding pairs with a given sum in an array
- Detecting duplicates
- Implementing caches
- Counting frequencies of elements
6. Trees and Binary Search Trees
Trees are hierarchical data structures consisting of nodes connected by edges. A tree has a root node and every node has zero or more child nodes.
Key Characteristics of Trees:
- Hierarchical structure
- No cycles
- Can represent relationships between data points
Binary Trees
A binary tree is a tree data structure in which each node has at most two children, referred to as the left child and the right child.
Binary Search Trees (BST)
A binary search tree is a binary tree with the following properties:
- The left subtree of a node contains only nodes with keys less than the node’s key
- The right subtree of a node contains only nodes with keys greater than the node’s key
- Both the left and right subtrees must also be binary search trees
Common BST Operations and Their Time Complexities:
- Search: Average O(log n), Worst O(n)
- Insert: Average O(log n), Worst O(n)
- Delete: Average O(log n), Worst O(n)
Example: Implementing a Binary Search Tree in Python
class Node:
def __init__(self, key):
self.left = None
self.right = None
self.val = key
class BST:
def __init__(self):
self.root = None
def insert(self, key):
self.root = self._insert_recursive(self.root, key)
def _insert_recursive(self, root, key):
if root is None:
return Node(key)
if key < root.val:
root.left = self._insert_recursive(root.left, key)
else:
root.right = self._insert_recursive(root.right, key)
return root
def search(self, key):
return self._search_recursive(self.root, key)
def _search_recursive(self, root, key):
if root is None or root.val == key:
return root
if root.val < key:
return self._search_recursive(root.right, key)
return self._search_recursive(root.left, key)
def inorder_traversal(self):
self._inorder_recursive(self.root)
def _inorder_recursive(self, root):
if root:
self._inorder_recursive(root.left)
print(root.val, end=' ')
self._inorder_recursive(root.right)
Trees, especially binary search trees, are common in interview questions. They are used in scenarios such as:
- Implementing search algorithms
- Representing hierarchical data
- Expression parsing
- Implementing other data structures like heaps and tries
7. Heaps
A heap is a specialized tree-based data structure that satisfies the heap property. In a max heap, for any given node I, the value of I is greater than or equal to the values of its children. In a min heap, the value of I is less than or equal to the values of its children.
Key Characteristics of Heaps:
- Complete binary tree
- Efficient for finding the minimum or maximum element
- Often implemented using arrays
Common Heap Operations and Their Time Complexities:
- Insert: O(log n)
- Delete max/min: O(log n)
- Get max/min: O(1)
- Heapify: O(n)
Example: Implementing a Min Heap in Python
import heapq
class MinHeap:
def __init__(self):
self.heap = []
def parent(self, i):
return (i - 1) // 2
def left_child(self, i):
return 2 * i + 1
def right_child(self, i):
return 2 * i + 2
def insert(self, key):
heapq.heappush(self.heap, key)
def delete_min(self):
if self.heap:
return heapq.heappop(self.heap)
def get_min(self):
if self.heap:
return self.heap[0]
def heapify(self, arr):
self.heap = arr
heapq.heapify(self.heap)
# Usage
min_heap = MinHeap()
min_heap.insert(3)
min_heap.insert(2)
min_heap.insert(1)
min_heap.insert(5)
min_heap.insert(4)
print(min_heap.get_min()) # Output: 1
print(min_heap.delete_min()) # Output: 1
print(min_heap.get_min()) # Output: 2
Heaps are often used in interview questions involving:
- Priority queues
- Scheduling algorithms
- Finding the k-th largest/smallest element
- Implementing efficient sorting algorithms like Heap Sort
8. Graphs
Graphs are versatile data structures used to represent complex relationships and connections between objects. They consist of vertices (also called nodes) and edges that connect these vertices.
Key Characteristics of Graphs:
- Can represent a wide variety of real-world scenarios
- Can be directed (edges have a direction) or undirected
- Can be weighted (edges have associated costs) or unweighted
- Can be cyclic or acyclic
Common Graph Representations:
- Adjacency Matrix: A 2D array where matrix[i][j] represents an edge from vertex i to vertex j
- Adjacency List: An array of lists where each list describes the set of neighbors of a vertex
Common Graph Algorithms and Their Time Complexities:
- Breadth-First Search (BFS): O(V + E)
- Depth-First Search (DFS): O(V + E)
- Dijkstra’s Shortest Path: O((V + E) log V) with a binary heap
- Bellman-Ford Algorithm: O(VE)
- Floyd-Warshall Algorithm: O(V^3)
- Kruskal’s Minimum Spanning Tree: O(E log E) or O(E log V)
- Prim’s Minimum Spanning Tree: O((V + E) log V) with a binary heap
Example: Implementing a Graph using Adjacency List in Python
from collections import defaultdict
class Graph:
def __init__(self):
self.graph = defaultdict(list)
def add_edge(self, u, v):
self.graph[u].append(v)
def bfs(self, start):
visited = set()
queue = [start]
visited.add(start)
while queue:
vertex = queue.pop(0)
print(vertex, end=' ')
for neighbor in self.graph[vertex]:
if neighbor not in visited:
visited.add(neighbor)
queue.append(neighbor)
def dfs(self, start):
visited = set()
def dfs_util(vertex):
visited.add(vertex)
print(vertex, end=' ')
for neighbor in self.graph[vertex]:
if neighbor not in visited:
dfs_util(neighbor)
dfs_util(start)
# Usage
g = Graph()
g.add_edge(0, 1)
g.add_edge(0, 2)
g.add_edge(1, 2)
g.add_edge(2, 0)
g.add_edge(2, 3)
g.add_edge(3, 3)
print("BFS starting from vertex 2:")
g.bfs(2)
print("\nDFS starting from vertex 2:")
g.dfs(2)
Graphs are extensively used in interview questions, particularly for problems involving:
- Network flow
- Shortest path algorithms
- Connectivity problems
- Cycle detection
- Topological sorting
9. Advanced Data Structures
While the previously mentioned data structures form the core of most coding interviews, it’s beneficial to be familiar with some advanced data structures. These may not be as common in interviews, but understanding them can give you an edge and help solve complex problems more efficiently.
1. Trie (Prefix Tree)
A trie is an efficient information retrieval data structure. It’s particularly useful for tasks involving strings, such as autocomplete features or spell checkers.
Key Characteristics:
- Each node represents a character
- Paths from root to leaf represent complete words
- Efficient for prefix matching
Time Complexity:
- Insert: O(m), where m is the length of the string
- Search: O(m)
- Delete: O(m)
2. Segment Tree
A segment tree is a tree data structure used for storing information about intervals, or segments. It allows querying which of the stored segments contain a given point.
Key Characteristics:
- Efficient for range queries
- Supports updates in logarithmic time
Time Complexity:
- Build: O(n)
- Query: O(log n)
- Update: O(log n)
3. Fenwick Tree (Binary Indexed Tree)
A Fenwick tree or binary indexed tree is a data structure that can efficiently update elements and calculate prefix sums in a table of numbers.
Key Characteristics:
- More space-efficient than segment trees
- Efficient for calculating cumulative frequency
Time Complexity:
- Update: O(log n)
- Query: O(log n)
4. Disjoint Set (Union-Find)
A disjoint-set data structure, also known as a union–find data structure or merge–find set, is a data structure that stores a collection of disjoint (non-overlapping) sets.
Key Characteristics:
- Efficient for grouping elements into sets
- Useful for finding connected components in graphs
Time Complexity:
- Union: O(α(n)), where α(n) is the inverse Ackermann function
- Find: O(α(n))
5. Bloom Filter
A Bloom filter is a space-efficient probabilistic data structure used to test whether an element is a member of a set.
Key Characteristics:
- Space-efficient for representing sets
- Can have false positives, but no false negatives
Time Complexity:
- Insert: O(k), where k is the number of hash functions
- Lookup: O(k)
While these advanced data structures may not be as common in coding interviews, understanding them can help you solve certain problems more efficiently. They’re particularly useful in specific domains like string processing, range queries, and set operations.
10. Practice Strategies
Mastering data structures for coding interviews requires consistent practice and a structured approach. Here are some effective strategies to enhance your skills:
1. Consistent Daily Practice
- Set aside dedicated time each day for data structure problems
- Start with easier problems and gradually increase difficulty
- Use platforms like LeetCode, HackerRank, or CodeSignal for a variety of problems
2. Implement Data Structures from Scratch
- Build common data structures like linked lists, stacks, queues, and trees from scratch
- Implement their basic operations to understand their inner workings
3. Solve Problems Using Multiple Data Structures
- For each problem, try to solve it using different data structures
- Compare the time and space complexities of each solution
4. Focus on Understanding, Not Just Memorization
- Don’t just memorize solutions; understand why a particular data structure is suitable for a given problem
- Analyze the trade-offs between different data structures for each problem
5. Review and Reflect
- After solving a problem, review your solution and look for optimizations
- Study other people’s solutions to learn different approaches
6. Mock Interviews
- Practice with a friend or use platforms that offer mock interviews
- Get comfortable explaining your thought process out loud
7. Time Your Problem-Solving
- Practice solving problems within time constraints to simulate interview conditions
- Aim to reduce your solving time for common problem types
8. Study Real Interview Questions
- Look for interview experiences shared by others online
- Focus on questions from companies you’re interested in
9. Implement a Study Plan
- Create a structured study plan covering all major data structures
- Allocate more time to areas where you’re weaker
10. Use Visualization Tools
- Utilize online tools or draw diagrams to visualize data structures and algorithms
- This can help in better understanding complex operations
Remember, consistency is key. Regular practice over an extended period will yield better results than cramming right before an interview. As you practice, you’ll not only improve your problem-solving skills but also gain confidence in your abilities.
11. Interview Tips and Tricks
Mastering data structures is crucial, but performing well in a coding interview requires more than just technical knowledge. Here are some tips and tricks to help you excel:
1. Communicate Clearly
- Explain your thought process out loud as you work through the problem
- Clarify any assumptions you’re making about the problem
- Ask questions if you need more information
2. Start with a Brute Force Approach
- Begin by explaining the simplest solution you can think of
- Discuss its time and space complexity
- Use this as a starting point to develop more efficient solutions
3. Analyze Time and Space Complexity
- Always discuss the time and space complexity of your solution
- Be prepared to optimize your solution if asked
4. Use Appropriate Data Structures
- Choose the most suitable data structure for the problem
- Explain why you chose a particular data structure
5. Write Clean, Readable Code
- Use meaningful variable and function names
- Structure your code with proper indentation
- Add comments to explain complex parts of your code
6. Test Your Code
- After writing your solution, walk through it with a simple example
- Consider edge cases and how your code handles them
7. Be Open to Hints
- If you’re stuck, don’t be afraid to ask for hints
- Use hints as a guide, not a complete solution
8. Practice Good Time Management
- Keep an eye on the time during the interview
- If you’re spending too long on one part, consider moving on and coming back later
9. Stay Calm Under Pressure
- Take deep breaths if you feel stressed
- Remember that it’s okay to take a moment to think
10. Show Enthusiasm
- Demonstrate your passion for problem-solving and coding
- Show interest in learning about the company and the role
11. Handle Mistakes Gracefully
- If you realize you’ve made a mistake, acknowledge it and correct it
- Treat mistakes as learning opportunities
12. Ask Thoughtful Questions
- Prepare questions about the company, team, and role
- Show that you’ve done your research
Remember, interviewers are not just assessing your technical skills, but also your problem