The Ultimate Guide to Coding Interview Preparation
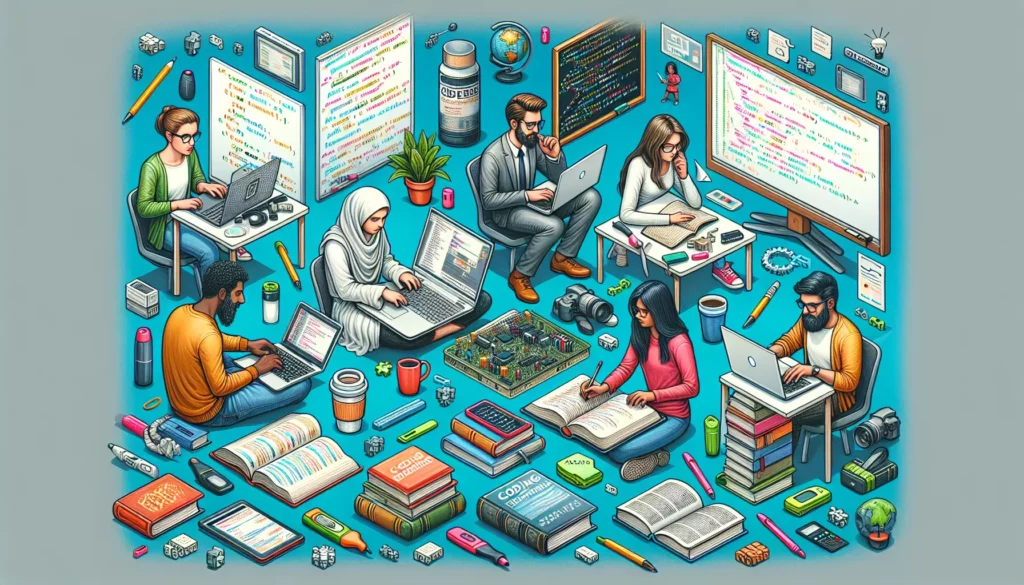
In today’s competitive tech landscape, acing your coding interview is crucial to landing your dream job at top companies like Google, Amazon, or Facebook. Whether you’re a fresh graduate or an experienced developer looking to switch roles, this comprehensive guide will equip you with the knowledge and strategies needed to excel in coding interviews. From mastering data structures and algorithms to honing your problem-solving skills, we’ll cover everything you need to know to prepare for success.
Table of Contents
- Understanding Coding Interviews
- Essential Topics to Master
- Problem-Solving Strategies
- Practice Resources and Platforms
- Conducting Mock Interviews
- Soft Skills and Communication
- Interview Day Preparation
- Post-Interview Follow-up
- Common Mistakes to Avoid
- Success Stories and Tips from Industry Professionals
1. Understanding Coding Interviews
Before diving into preparation strategies, it’s essential to understand what coding interviews entail and why companies use them. Coding interviews are designed to assess a candidate’s technical skills, problem-solving abilities, and cultural fit within the organization.
Types of Coding Interviews
- Phone Screenings: Initial technical assessments conducted over the phone or video call.
- Take-home Assignments: Coding projects to be completed within a specified timeframe.
- On-site Interviews: Multiple rounds of face-to-face interviews, including whiteboard coding sessions.
- Pair Programming: Collaborative coding sessions with an interviewer.
What Interviewers Look For
- Problem-solving skills
- Code quality and efficiency
- Communication and collaboration abilities
- Ability to handle pressure and think on your feet
- Knowledge of fundamental computer science concepts
2. Essential Topics to Master
To succeed in coding interviews, you need a strong foundation in core computer science concepts and data structures. Here are the key areas to focus on:
Data Structures
- Arrays and Strings
- Linked Lists
- Stacks and Queues
- Trees and Graphs
- Hash Tables
- Heaps
Algorithms
- Sorting (e.g., Quicksort, Mergesort)
- Searching (e.g., Binary Search)
- Graph Traversal (BFS, DFS)
- Dynamic Programming
- Greedy Algorithms
- Divide and Conquer
Time and Space Complexity Analysis
Understanding Big O notation and being able to analyze the efficiency of your solutions is crucial. Practice evaluating the time and space complexity of different algorithms and data structures.
Object-Oriented Programming (OOP) Concepts
- Encapsulation
- Inheritance
- Polymorphism
- Abstraction
System Design Basics
For more senior positions, having a grasp of system design principles is essential. Familiarize yourself with concepts like:
- Scalability
- Load Balancing
- Caching
- Database Sharding
3. Problem-Solving Strategies
Developing a systematic approach to problem-solving is key to success in coding interviews. Here’s a step-by-step strategy to tackle coding challenges:
- Understand the Problem: Carefully read the question and ask clarifying questions if needed.
- Identify the Inputs and Outputs: Determine what data you’re working with and what result is expected.
- Break Down the Problem: Divide the problem into smaller, manageable sub-problems.
- Consider Edge Cases: Think about potential edge cases and how to handle them.
- Develop a High-Level Approach: Outline your solution strategy before diving into coding.
- Implement the Solution: Write clean, readable code to implement your approach.
- Test and Debug: Run through test cases and fix any issues in your code.
- Optimize: Look for ways to improve the efficiency of your solution.
Example Problem-Solving Walkthrough
Let’s walk through a simple problem to illustrate this approach:
Problem: Given an array of integers, find two numbers such that they add up to a specific target number.
function twoSum(nums, target) {
const numMap = new Map();
for (let i = 0; i < nums.length; i++) {
const complement = target - nums[i];
if (numMap.has(complement)) {
return [numMap.get(complement), i];
}
numMap.set(nums[i], i);
}
return []; // No solution found
}
// Example usage
const nums = [2, 7, 11, 15];
const target = 9;
console.log(twoSum(nums, target)); // Output: [0, 1]
In this example, we use a hash map to store the numbers we’ve seen so far, allowing us to find the complement in constant time. This approach has a time complexity of O(n) and a space complexity of O(n).
4. Practice Resources and Platforms
Consistent practice is crucial for improving your coding skills and interview performance. Here are some excellent resources to help you prepare:
Online Platforms
- LeetCode: Offers a vast collection of coding problems with difficulty ratings and company tags.
- HackerRank: Provides coding challenges and competitions across various domains.
- CodeSignal: Features a mix of practice problems and real company coding assessments.
- AlgoExpert: Curated list of coding interview questions with video explanations.
Books
- “Cracking the Coding Interview” by Gayle Laakmann McDowell
- “Elements of Programming Interviews” by Adnan Aziz, Tsung-Hsien Lee, and Amit Prakash
- “Introduction to Algorithms” by Thomas H. Cormen, Charles E. Leiserson, Ronald L. Rivest, and Clifford Stein
Online Courses
- Coursera’s “Algorithms Specialization” by Stanford University
- edX’s “Introduction to Computer Science and Programming Using Python” by MIT
- Udacity’s “Data Structures and Algorithms Nanodegree”
5. Conducting Mock Interviews
Mock interviews are an excellent way to simulate the real interview experience and get valuable feedback. Here’s how to make the most of them:
Finding Mock Interview Partners
- Join coding interview preparation groups on platforms like Meetup or Facebook
- Use websites like Pramp or InterviewBit that offer peer-to-peer mock interviews
- Ask friends or colleagues in the tech industry to conduct mock interviews for you
Structuring Mock Interviews
- Set a specific time limit (e.g., 45 minutes)
- Choose a problem that mimics real interview questions
- Have the interviewer take notes on your performance
- Conduct a thorough debrief after the interview to discuss areas for improvement
Key Areas to Focus On During Mock Interviews
- Clear communication of your thought process
- Ability to handle hints and guidance
- Time management
- Code quality and organization
- Handling pressure and unexpected challenges
6. Soft Skills and Communication
While technical skills are crucial, soft skills play a significant role in your overall interview performance. Here are some key areas to focus on:
Effective Communication
- Clearly explain your thought process and approach to problems
- Ask clarifying questions when needed
- Use appropriate technical terminology
- Practice active listening
Collaboration
- Show willingness to consider alternative approaches
- Demonstrate teamwork skills during pair programming exercises
- Be open to feedback and suggestions
Handling Pressure
- Stay calm and composed when faced with challenging questions
- Practice deep breathing or other relaxation techniques
- Maintain a positive attitude throughout the interview
Cultural Fit
- Research the company’s values and culture
- Prepare examples that demonstrate alignment with the company’s mission
- Show enthusiasm for the role and the company
7. Interview Day Preparation
Proper preparation for the day of your interview can help you feel more confident and perform at your best. Here’s a checklist to ensure you’re ready:
Before the Interview
- Get a good night’s sleep
- Eat a healthy meal
- Review your resume and prepare to discuss your experiences
- Gather necessary materials (e.g., pen, notepad, water bottle)
- Test your computer and internet connection for virtual interviews
- Review the job description and company information
During the Interview
- Arrive early or log in to the virtual meeting room ahead of time
- Dress professionally, even for virtual interviews
- Maintain good posture and eye contact
- Take brief pauses to collect your thoughts when needed
- Ask thoughtful questions about the role and company
Technical Setup for Virtual Interviews
- Ensure your coding environment is set up and ready to go
- Have a backup internet connection (e.g., mobile hotspot) available
- Test your microphone and camera
- Choose a quiet, well-lit location for the interview
8. Post-Interview Follow-up
Your actions after the interview can leave a lasting impression and potentially influence the hiring decision. Here’s what to do:
Send a Thank-You Note
- Email a personalized thank-you note to your interviewers within 24 hours
- Express your appreciation for their time and reiterate your interest in the position
- Briefly mention a specific topic or insight from the interview to make your note memorable
Reflect on Your Performance
- Write down the questions you were asked and how you answered them
- Identify areas where you excelled and areas for improvement
- Research any topics or concepts you struggled with during the interview
Follow Up on Next Steps
- If you haven’t heard back within the specified timeframe, send a polite follow-up email
- Express your continued interest in the position and ask about the next steps in the process
9. Common Mistakes to Avoid
Being aware of common pitfalls can help you avoid them during your interview preparation and performance. Here are some mistakes to watch out for:
Technical Mistakes
- Jumping into coding without fully understanding the problem
- Overlooking edge cases or failing to validate input
- Writing overly complex solutions when simpler ones exist
- Ignoring time and space complexity considerations
- Not testing your code or handling error cases
Communication Mistakes
- Failing to explain your thought process as you work
- Being defensive or argumentative when receiving feedback
- Not asking clarifying questions when needed
- Rambling or providing unnecessarily long explanations
Behavioral Mistakes
- Showing a lack of enthusiasm or interest in the role
- Badmouthing previous employers or colleagues
- Demonstrating overconfidence or arrogance
- Failing to research the company or role adequately
Preparation Mistakes
- Focusing solely on memorizing solutions to specific problems
- Neglecting to practice coding on a whiteboard or in a shared online environment
- Not allocating enough time for consistent practice
- Ignoring the importance of soft skills and behavioral interview preparation
10. Success Stories and Tips from Industry Professionals
Learning from those who have successfully navigated the coding interview process can provide valuable insights and motivation. Here are some success stories and tips from industry professionals:
Sarah Chen, Software Engineer at Google
“The key to my success was consistent practice. I solved at least two LeetCode problems every day for three months leading up to my interviews. I also focused on understanding the underlying patterns in different types of problems, which helped me approach new challenges more effectively.”
Mike Johnson, Senior Developer at Amazon
“Don’t underestimate the importance of system design questions, especially for more senior roles. I spent a significant amount of time studying distributed systems concepts and practicing designing scalable architectures. This preparation was crucial in helping me land my current position.”
Emily Wong, Frontend Engineer at Facebook
“Mock interviews were a game-changer for me. They helped me get comfortable with the interview format and improved my ability to communicate my thought process clearly. I also learned to manage my time better during coding sessions.”
Additional Tips from Successful Candidates
- Focus on understanding core concepts rather than memorizing solutions
- Practice explaining your code and thought process out loud
- Don’t be afraid to ask for help or clarification during the interview
- Stay up-to-date with industry trends and new technologies
- Develop a growth mindset and view each interview as a learning opportunity
Conclusion
Preparing for coding interviews can be a challenging and time-consuming process, but with the right approach and dedication, you can significantly improve your chances of success. Remember that interview preparation is not just about memorizing solutions or cramming information; it’s about developing a problem-solving mindset and honing your skills over time.
By focusing on mastering core computer science concepts, practicing consistently, honing your communication skills, and learning from both successes and failures, you’ll be well-equipped to tackle even the most challenging coding interviews. Stay persistent, embrace the learning process, and approach each interview as an opportunity to showcase your skills and passion for technology.
As you embark on your journey to ace coding interviews, remember that every experienced developer has been in your shoes. With patience, hard work, and the strategies outlined in this guide, you’ll be well on your way to landing your dream job in the tech industry. Good luck, and happy coding!