Why Your Code Refactoring is Actually a Form of Time Travel
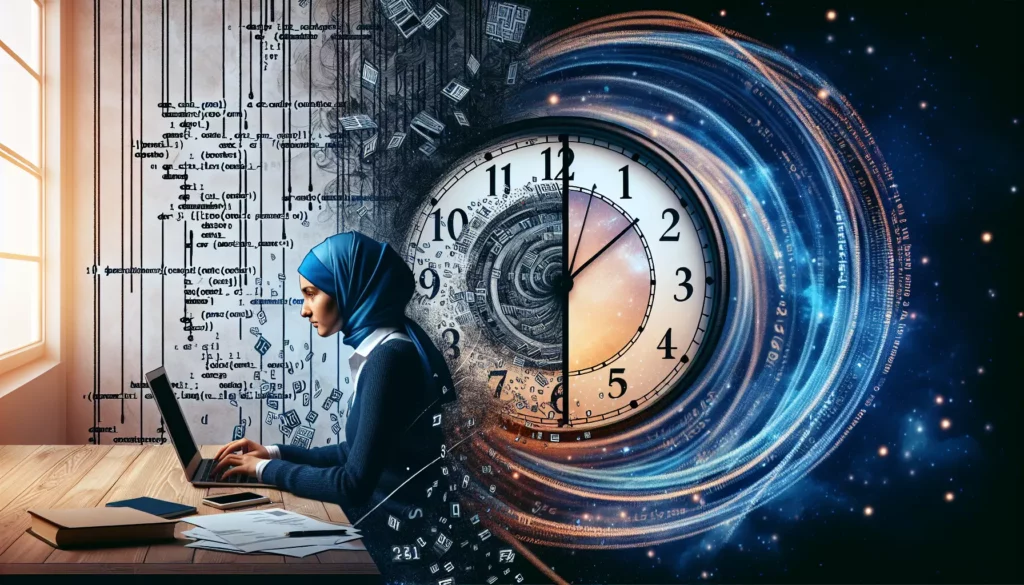
As software developers, we often find ourselves knee-deep in code, wrestling with complex algorithms and intricate data structures. But have you ever stopped to consider that when you’re refactoring code, you’re not just cleaning up lines of text—you’re actually engaging in a form of time travel? It might sound far-fetched, but stick with me, and I’ll show you how the art of refactoring is more akin to traversing the space-time continuum than you might think.
The Time Machine of Code
Imagine your codebase as a vast, sprawling city. Each function, each class, each module is a building in this metropolis of logic. Now, picture yourself as a time-traveling architect, equipped with the power to reshape this city not just in the present, but across its entire history and future. That’s essentially what you’re doing when you refactor code.
When you refactor, you’re not just changing the code as it exists now. You’re reaching back into the past, understanding the decisions and constraints that led to the current structure. At the same time, you’re projecting into the future, anticipating how your changes will affect the evolution of the codebase. It’s a delicate balance of respecting the past, improving the present, and safeguarding the future.
Traveling to the Past: Understanding Legacy Code
One of the first steps in any refactoring journey is to understand the existing code. This is where our time travel analogy really starts to take shape. As you dive into legacy code, you’re essentially traveling back in time, trying to get into the mindset of the original developers.
Consider this snippet of legacy JavaScript code:
function calculateTotal(items) {
var total = 0;
for (var i = 0; i < items.length; i++) {
total += items[i].price * items[i].quantity;
}
return total;
}
At first glance, it might seem straightforward. But as you examine it, you’re piecing together the context in which it was written. Why did they use a for
loop instead of reduce
? Was ES6 not widely adopted when this was written? You’re not just reading code; you’re archeologically reconstructing the technological landscape of the past.
Altering the Present: The Act of Refactoring
Now that you’ve understood the past, you’re ready to make changes in the present. This is where the real “time manipulation” occurs. You’re taking code shaped by past decisions and reshaping it based on current best practices and future needs.
Let’s refactor our previous example:
const calculateTotal = (items) =>
items.reduce((total, item) => total + item.price * item.quantity, 0);
In this refactored version, we’ve used ES6 syntax, arrow functions, and the reduce
method. We’ve essentially taken a piece of code from the past and updated it to modern standards. But we’re not just changing syntax; we’re changing the very fabric of how the code operates, potentially affecting its performance, readability, and maintainability.
Glimpsing the Future: Anticipating Code Evolution
But our time travel doesn’t stop at the present. Good refactoring also involves looking ahead, anticipating how the code might need to evolve in the future. This is where your experience as a developer really comes into play. You’re not just fixing what’s broken now; you’re setting up the codebase for success in scenarios that haven’t even occurred yet.
For instance, let’s take our refactored calculateTotal
function a step further:
const calculateTotal = (items, applyDiscount = false) => {
const rawTotal = items.reduce((total, item) => total + item.price * item.quantity, 0);
return applyDiscount ? rawTotal * 0.9 : rawTotal;
};
Now we’ve added a parameter for applying a discount. We didn’t need this functionality when we started, but by thinking ahead, we’ve made the function more flexible for future requirements. This is like planting seeds in the present that will grow into robust features in the future.
The Butterfly Effect in Code
In time travel stories, there’s often talk of the “butterfly effect”—the idea that small changes in the past can have massive repercussions in the future. The same is true in refactoring. A small change in a core function can ripple out, affecting numerous other parts of your application.
For example, imagine we decide to change how we represent prices in our system, moving from cents to dollars:
const calculateTotal = (items, applyDiscount = false) => {
const rawTotal = items.reduce((total, item) => total + (item.price / 100) * item.quantity, 0);
return applyDiscount ? rawTotal * 0.9 : rawTotal;
};
This small change—dividing the price by 100—could have far-reaching effects. Any code that interacts with this function now needs to be aware of this change. It’s like going back in time and making a tiny alteration that changes the course of history.
The Paradoxes of Refactoring
Just as time travel stories often grapple with paradoxes, refactoring has its own set of contradictions and challenges. One of the most common is the “refactoring paradox”: the idea that by trying to improve code, we might temporarily make it worse or introduce new bugs.
Consider this more complex refactoring:
class ShoppingCart {
constructor() {
this.items = [];
}
addItem(item) {
this.items.push(item);
}
calculateTotal(applyDiscount = false) {
const rawTotal = this.items.reduce((total, item) => total + (item.price / 100) * item.quantity, 0);
return applyDiscount ? rawTotal * 0.9 : rawTotal;
}
}
const cart = new ShoppingCart();
cart.addItem({ name: "Widget", price: 1000, quantity: 2 });
console.log(cart.calculateTotal()); // Outputs 20
In this refactoring, we’ve encapsulated our shopping cart logic into a class. This is generally a good practice, but it’s also changed how we interact with the code. Any part of our application that was using the old calculateTotal
function directly now needs to be updated. We’ve improved the code, but at the cost of potentially breaking existing functionality—a classic time travel paradox!
The Time Traveler’s Toolkit: Refactoring Techniques
Just as time travelers in science fiction have their tools and techniques, so do we as code refactorers. Let’s explore some of these techniques and how they relate to our time travel analogy.
1. Extract Method: Creating New Timelines
The extract method technique involves taking a piece of code and turning it into its own method. This is like creating a new timeline—a separate stream of logic that can be reused and modified independently.
class ShoppingCart {
// ... previous code ...
calculateSubtotal() {
return this.items.reduce((total, item) => total + (item.price / 100) * item.quantity, 0);
}
calculateTotal(applyDiscount = false) {
const rawTotal = this.calculateSubtotal();
return applyDiscount ? rawTotal * 0.9 : rawTotal;
}
}
By extracting the subtotal calculation into its own method, we’ve created a new “timeline” that can be used and modified independently of the total calculation.
2. Rename Method: Rewriting History
Renaming a method is like going back in time and changing how something was originally named. It doesn’t change the functionality, but it can greatly improve understanding and maintainability.
class ShoppingCart {
// ... previous code ...
applyDiscount(total, discountPercentage) {
return total * (1 - discountPercentage / 100);
}
calculateTotal(discountPercentage = 0) {
const rawTotal = this.calculateSubtotal();
return this.applyDiscount(rawTotal, discountPercentage);
}
}
We’ve renamed our discount application logic and made it more flexible. It’s as if we’ve gone back in time and given our past selves better naming conventions.
3. Move Method: Altering the Fabric of Space-Time
Moving a method from one class to another is like altering the very fabric of your code’s space-time. You’re deciding that a piece of functionality belongs somewhere else entirely.
class DiscountCalculator {
static apply(total, discountPercentage) {
return total * (1 - discountPercentage / 100);
}
}
class ShoppingCart {
// ... previous code ...
calculateTotal(discountPercentage = 0) {
const rawTotal = this.calculateSubtotal();
return DiscountCalculator.apply(rawTotal, discountPercentage);
}
}
We’ve moved the discount calculation to its own class. This is like deciding that a particular event in history actually belongs to a different timeline altogether.
The Ripple Effects: How Refactoring Changes Everything
When you refactor code, you’re not just changing isolated bits of functionality. You’re potentially altering the entire ecosystem of your application. Let’s explore some of these ripple effects.
Performance Implications
Refactoring can have significant impacts on performance. Sometimes, what seems like a cleaner, more elegant solution might actually be less efficient. For example:
// Original code
function findLargestNumber(numbers) {
let largest = numbers[0];
for (let i = 1; i < numbers.length; i++) {
if (numbers[i] > largest) {
largest = numbers[i];
}
}
return largest;
}
// Refactored code
const findLargestNumber = (numbers) => Math.max(...numbers);
The refactored version is certainly more concise, but for very large arrays, it could be less efficient due to the spread operator. It’s like changing a small event in the past that leads to unexpected consequences in the future.
Testing Implications
Refactoring often necessitates changes in your testing strategy. As you modify code, you might need to update existing tests or write new ones. This is like ensuring that your changes to the timeline don’t create paradoxes or unintended consequences.
describe('ShoppingCart', () => {
let cart;
beforeEach(() => {
cart = new ShoppingCart();
});
test('calculateTotal applies discount correctly', () => {
cart.addItem({ name: "Widget", price: 1000, quantity: 2 });
expect(cart.calculateTotal(10)).toBeCloseTo(18);
});
test('calculateSubtotal works correctly', () => {
cart.addItem({ name: "Widget", price: 1000, quantity: 2 });
expect(cart.calculateSubtotal()).toBe(20);
});
});
These tests ensure that our refactored code still behaves as expected, much like a time traveler might run simulations to ensure their changes don’t disrupt the timeline.
The Ethics of Code Time Travel
Just as time travel raises ethical questions in science fiction, refactoring presents its own ethical considerations in the world of software development.
Respecting the Past
When refactoring legacy code, it’s important to respect the decisions of past developers. They were working with the knowledge and constraints of their time. Before making sweeping changes, try to understand the context in which the original code was written.
Responsibility to the Future
As a refactorer, you have a responsibility to future developers (including your future self). Your changes should make the code more maintainable and extensible, not just different.
Balancing Progress and Stability
There’s often a tension between wanting to improve code and maintaining stability. Radical refactoring might improve code quality but could also introduce bugs or break existing functionality. It’s crucial to find the right balance.
Tools of the Time Traveling Developer
Just as time travelers need special equipment, developers engaged in refactoring have their own set of tools:
Version Control Systems
Git and other version control systems are like time machines for your code. They allow you to travel back to any point in your code’s history, create alternate timelines (branches), and even merge different timelines together.
IDE Refactoring Tools
Modern IDEs come with built-in refactoring tools that can automate many common refactoring tasks. These are like having a time machine with pre-programmed destinations.
Static Analysis Tools
Tools like ESLint for JavaScript or RuboCop for Ruby are like sensors that can detect anomalies in the code timeline, helping you identify areas that need refactoring.
Testing Frameworks
Robust testing frameworks are your safeguard against temporal paradoxes. They ensure that your changes don’t unintentionally alter the functionality of your code.
The Continuum of Code: Past, Present, and Future
As we wrap up our journey through the time-bending world of code refactoring, it’s worth reflecting on how this process connects the past, present, and future of our codebases.
Learning from the Past
Every piece of code has a history. By understanding this history—the decisions made, the constraints faced, the problems solved—we can make more informed decisions about how to improve it. This archaeological approach to code helps us avoid repeating past mistakes and builds on the accumulated wisdom of previous developers.
Improving the Present
Refactoring is fundamentally about improving the present state of our code. We’re taking what exists now and making it better—more efficient, more readable, more maintainable. This process of continuous improvement is what keeps our codebases healthy and our applications running smoothly.
Preparing for the Future
Perhaps most importantly, refactoring is about preparing our code for the future. We’re not just solving today’s problems; we’re setting up our codebase to be flexible and extensible for tomorrow’s challenges. Every time we refactor, we’re laying the groundwork for future features, optimizations, and innovations.
Conclusion: The Timeless Art of Refactoring
Refactoring, when viewed through the lens of time travel, becomes more than just a technical task. It’s a journey through the lifetime of your code, a delicate balance of respecting the past, improving the present, and preparing for the future.
As you embark on your next refactoring adventure, remember that you’re not just editing code—you’re traversing the timeline of your application. You’re the guardian of your code’s past, the architect of its present, and the visionary of its future.
So the next time you sit down to refactor, take a moment to appreciate the time-bending nature of what you’re about to do. You’re not just a developer; you’re a time traveler, shaping the destiny of your codebase one commit at a time.
Happy coding, and may your travels through the space-time continuum of code be fruitful and paradox-free!