The Art of Using Programming Languages to Create a New Spoken Language
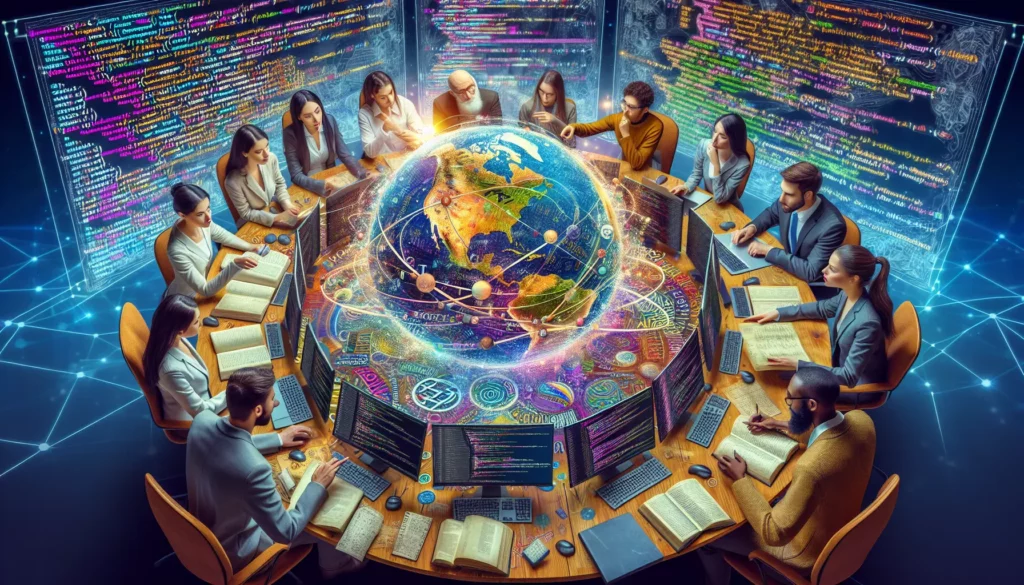
In the vast realm of coding education and programming skills development, we often focus on learning existing languages to communicate with machines. But what if we could use our programming knowledge to create an entirely new spoken language? This fascinating intersection of linguistics and computer science opens up a world of possibilities for language enthusiasts and programmers alike. In this article, we’ll explore how programming concepts can be applied to construct a new language, the benefits of such an endeavor, and how it relates to the broader field of coding education.
The Intersection of Programming and Linguistics
At first glance, programming languages and human languages might seem worlds apart. However, they share more similarities than one might think. Both are structured systems for communication, governed by rules and syntax. Just as programming languages have their grammar and vocabulary, so do spoken languages. This parallel makes it possible to apply programming principles to the creation of a new spoken language.
Key Concepts in Language Creation
- Syntax: The rules governing the structure of sentences
- Semantics: The meaning associated with words and phrases
- Phonology: The system of sounds used in the language
- Morphology: The structure and formation of words
- Pragmatics: The practical use of language in context
These linguistic concepts have analogues in programming, making it easier for those with a coding background to approach language creation systematically.
Applying Programming Concepts to Language Creation
Let’s explore how various programming concepts can be applied to the process of creating a new spoken language.
1. Variables and Data Types
In programming, variables are used to store and manipulate data. Similarly, in a constructed language, we can define different classes of words (nouns, verbs, adjectives, etc.) as our “data types.” Each word in the language would then be an instance of one of these types, with specific properties and behaviors.
class Noun:
def __init__(self, root, plural_suffix="s"):
self.root = root
self.plural_suffix = plural_suffix
def pluralize(self):
return self.root + self.plural_suffix
# Example usage
tree = Noun("tree")
print(tree.pluralize()) # Output: trees
In this example, we’ve created a simple class for nouns in our language, with a method for pluralization. This object-oriented approach allows us to easily manage and manipulate words in our constructed language.
2. Functions and Methods
Functions in programming can be likened to grammatical rules in language. We can create functions that handle various linguistic operations, such as conjugating verbs, declining nouns, or forming compound words.
def conjugate_verb(verb, tense, person, number):
if tense == "present":
if person == 3 and number == "singular":
return verb + "s"
return verb
# Example usage
print(conjugate_verb("run", "present", 3, "singular")) # Output: runs
print(conjugate_verb("run", "present", 1, "plural")) # Output: run
This function demonstrates a simple verb conjugation rule. More complex rules can be implemented to handle various tenses, moods, and aspects of verbs in the constructed language.
3. Control Structures
Control structures like if-else statements and loops can be used to implement more complex linguistic rules. For example, we can use conditional statements to handle irregular forms or exceptions in our language.
def pluralize(noun):
irregular_plurals = {
"child": "children",
"person": "people",
"mouse": "mice"
}
if noun in irregular_plurals:
return irregular_plurals[noun]
elif noun.endswith("y"):
return noun[:-1] + "ies"
else:
return noun + "s"
# Example usage
print(pluralize("child")) # Output: children
print(pluralize("city")) # Output: cities
print(pluralize("book")) # Output: books
This function demonstrates how we can handle both regular and irregular plural forms in our constructed language using control structures.
4. Data Structures
Various data structures can be employed to organize and store linguistic information efficiently. For instance, we can use dictionaries to store vocabulary, lists for grammatical categories, or trees to represent sentence structures.
vocabulary = {
"nouns": ["tree", "house", "book", "person"],
"verbs": ["run", "speak", "write", "think"],
"adjectives": ["big", "small", "red", "happy"]
}
class SentenceNode:
def __init__(self, word, part_of_speech):
self.word = word
self.part_of_speech = part_of_speech
self.children = []
# Example usage
root = SentenceNode("speaks", "verb")
subject = SentenceNode("person", "noun")
object = SentenceNode("language", "noun")
root.children = [subject, object]
def print_sentence(node, level=0):
print(" " * level + f"{node.word} ({node.part_of_speech})")
for child in node.children:
print_sentence(child, level + 1)
print_sentence(root)
This example shows how we can use a dictionary to store vocabulary and a tree structure to represent the hierarchical nature of sentences in our language.
Benefits of Creating a Language Using Programming Principles
Applying programming concepts to language creation offers several advantages:
- Consistency: Programming principles can help ensure that the language rules are applied consistently throughout the language.
- Scalability: As with software development, a well-structured language can be easily expanded and refined over time.
- Testability: We can create unit tests for our language rules, ensuring that they work as intended and catching any inconsistencies.
- Modularity: Different aspects of the language (phonology, morphology, syntax) can be developed as separate modules and integrated later.
- Automation: Many aspects of language generation, such as conjugation tables or vocabulary lists, can be automated using programming techniques.
Challenges in Programmatic Language Creation
While using programming principles to create a language offers many benefits, it also presents some challenges:
- Balancing Logic and Naturalness: Languages evolved naturally often have irregularities and exceptions that might not align with the logical structure of programming.
- Phonetic Considerations: Creating a phonetically pleasing and speakable language requires considerations that go beyond pure logic.
- Cultural Context: Languages are deeply tied to culture, and creating a language that feels authentic and usable requires more than just grammatical rules.
- Semantic Nuances: Capturing the full range of human expression and the subtle nuances of meaning can be challenging in a programmatically created language.
Tools and Resources for Language Creation
Several tools and resources can aid in the process of creating a new language using programming principles:
- Vulgar: A constructed language generator that creates a unique language with vocabulary, grammar, and phonology.
- Awkwords: A word generator that can be used to create consistent vocabulary based on defined patterns.
- Linguifex: A wiki for constructed languages, providing resources and a community for language creators.
- Natural Language Toolkit (NLTK): A Python library for working with human language data, which can be useful for analyzing and manipulating linguistic structures.
Practical Applications
Creating a new language using programming principles isn’t just an academic exercise. It has several practical applications:
- Fictional World-building: Authors and game developers can create rich, consistent languages for their fictional worlds.
- Language Learning Tools: The systematic approach can be used to develop new methods for teaching and learning languages.
- Linguistic Research: Constructed languages can serve as controlled environments for studying language acquisition and evolution.
- Communication Systems: In fields like cryptography or secure communications, constructed languages could provide new ways of encoding information.
- AI and NLP Development: Creating languages can provide insights into language structure that could be valuable in developing natural language processing systems.
Case Study: Lojban – A Logical Language
Lojban is an excellent example of a constructed language that incorporates logical principles similar to those found in programming. Created in 1987, Lojban aims to be culturally neutral and logically structured.
Key features of Lojban that align with programming concepts include:
- Unambiguous Grammar: Like a well-designed programming language, Lojban’s grammar is designed to be free from structural ambiguity.
- Predicate Structure: Sentences in Lojban are built around predicates, similar to functions in programming.
- Compound Words: Lojban allows for the systematic creation of new words, much like how new functions or classes can be created in programming.
Here’s a simple example of Lojban, with a breakdown of its structure:
mi prami do
I love you
mi = I (first-person pronoun)
prami = x1 loves x2 (predicate)
do = you (second-person pronoun)
This structure is reminiscent of function calls in programming, where ‘prami’ acts as the function and ‘mi’ and ‘do’ are its arguments.
Integrating Language Creation into Coding Education
The process of creating a new language using programming principles can be an excellent way to reinforce various coding concepts. Here are some ways to integrate language creation into coding education:
1. Object-Oriented Programming
Students can create classes for different parts of speech, implementing methods for various linguistic operations. This reinforces concepts like encapsulation, inheritance, and polymorphism.
class Word:
def __init__(self, root):
self.root = root
class Noun(Word):
def pluralize(self):
return self.root + "s"
class Verb(Word):
def conjugate(self, tense):
if tense == "past":
return self.root + "ed"
return self.root
# Example usage
tree = Noun("tree")
print(tree.pluralize()) # Output: trees
run = Verb("run")
print(run.conjugate("past")) # Output: runned
2. Data Structures and Algorithms
Implementing a language parser or generator can provide practical experience with various data structures (like trees for syntax parsing) and algorithms (like depth-first search for sentence generation).
class SyntaxTree:
def __init__(self, value):
self.value = value
self.children = []
def add_child(self, child):
self.children.append(child)
def generate_sentence(self):
sentence = [self.value]
for child in self.children:
sentence.extend(child.generate_sentence())
return sentence
# Example usage
root = SyntaxTree("S")
np = SyntaxTree("NP")
vp = SyntaxTree("VP")
root.add_child(np)
root.add_child(vp)
np.add_child(SyntaxTree("The"))
np.add_child(SyntaxTree("cat"))
vp.add_child(SyntaxTree("sleeps"))
print(" ".join(root.generate_sentence())) # Output: S NP The cat VP sleeps
3. Regular Expressions
Creating rules for word formation or sentence structure can provide an excellent opportunity to practice using regular expressions.
import re
def is_valid_word(word):
# Example rule: words must start with a consonant,
# end with a vowel, and be 3-8 characters long
pattern = r'^[^aeiou][a-z]{1,6}[aeiou]$'
return bool(re.match(pattern, word))
# Example usage
print(is_valid_word("hello")) # Output: True
print(is_valid_word("apple")) # Output: False
print(is_valid_word("try")) # Output: False
4. Functional Programming
Language rules can be implemented as pure functions, providing practice with functional programming concepts.
def pluralize(word):
return word + "s"
def past_tense(word):
return word + "ed"
def apply_rules(word, *rules):
return reduce(lambda w, rule: rule(w), rules, word)
# Example usage
print(apply_rules("walk", past_tense, pluralize)) # Output: walkeds
5. Database Design
Storing vocabulary and grammar rules can provide practice with database design and querying.
import sqlite3
conn = sqlite3.connect('language.db')
c = conn.cursor()
# Create tables
c.execute('''CREATE TABLE words
(id INTEGER PRIMARY KEY, word TEXT, part_of_speech TEXT)''')
c.execute('''CREATE TABLE rules
(id INTEGER PRIMARY KEY, rule_name TEXT, rule_function TEXT)''')
# Insert some data
c.execute("INSERT INTO words VALUES (1, 'run', 'verb')")
c.execute("INSERT INTO rules VALUES (1, 'past_tense', 'lambda w: w + "ed"')")
conn.commit()
conn.close()
Ethical Considerations in Language Creation
As with any powerful tool, the ability to create languages comes with ethical responsibilities. Here are some considerations:
- Inclusivity: Ensure that the created language doesn’t inadvertently exclude or marginalize any groups.
- Cultural Sensitivity: Be aware of cultural implications in language structure and vocabulary.
- Accessibility: Consider how the language can be made accessible to people with different abilities.
- Potential for Misuse: Be mindful of how the language could potentially be used for harmful purposes, such as exclusion or propaganda.
Future Directions
The intersection of programming and language creation offers exciting possibilities for the future:
- AI-assisted Language Creation: Machine learning algorithms could be used to generate more natural-sounding constructed languages.
- Virtual Reality Integration: Constructed languages could be deeply integrated into virtual worlds, enhancing immersion and interaction.
- Universal Translation: Principles from constructed languages could inform the development of more effective universal translation systems.
- Cognitive Science Research: Constructed languages could serve as controlled environments for studying language acquisition and processing.
Conclusion
The art of using programming languages to create a new spoken language is a fascinating blend of computer science, linguistics, and creativity. It offers a unique way to apply programming skills to a different domain, potentially enhancing both language learning and coding education.
For coding educators and students alike, language creation provides a novel and engaging way to practice various programming concepts. It encourages thinking about structure, rules, and systems in new ways, potentially leading to insights that can be applied back to traditional software development.
As we continue to explore the intersections between different fields of study, the combination of programming and linguistics stands out as a particularly fertile ground for innovation. Whether used for world-building, language learning, or pushing the boundaries of how we think about communication, the programmatic approach to language creation opens up exciting new possibilities.
In the spirit of AlgoCademy’s focus on coding education and skill development, we encourage readers to experiment with creating their own languages using the programming principles discussed in this article. Not only will it provide valuable practice in coding, but it may also offer new perspectives on the nature of language and communication itself.