What to Expect in a Database Design Interview
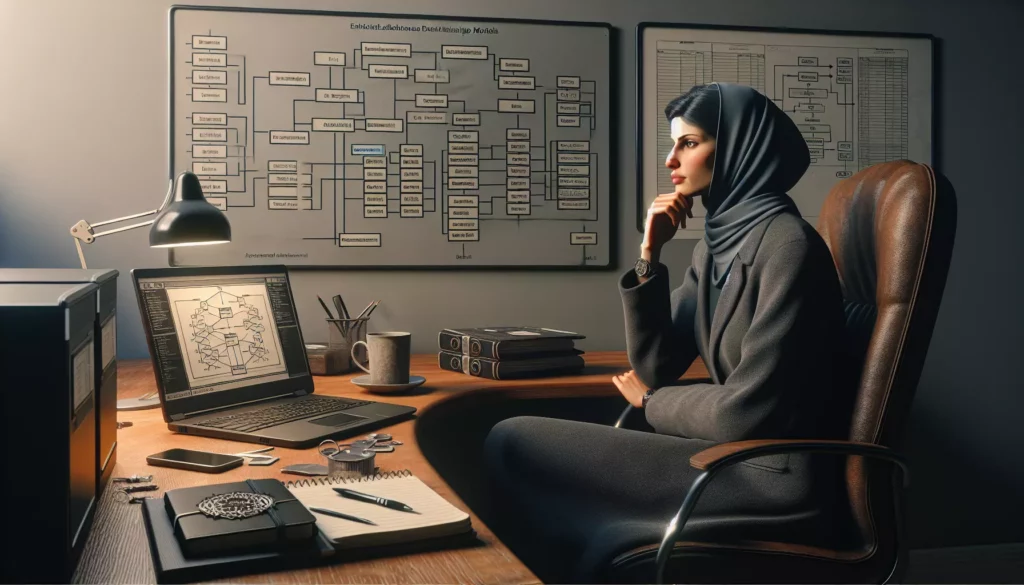
Database design interviews are a crucial part of the hiring process for database administrators, data engineers, and even some software engineering roles. These interviews assess your ability to design efficient, scalable, and robust database systems. If you’re preparing for a database design interview, especially at major tech companies like FAANG (Facebook, Amazon, Apple, Netflix, Google), it’s essential to know what to expect and how to prepare. In this comprehensive guide, we’ll explore the key aspects of database design interviews, common questions, and strategies to help you succeed.
1. Understanding the Basics of Database Design
Before diving into the specifics of what to expect in a database design interview, it’s crucial to have a solid foundation in the fundamentals of database design. This includes:
- Relational database concepts
- Entity-Relationship (ER) modeling
- Normalization and denormalization
- SQL (Structured Query Language)
- ACID properties (Atomicity, Consistency, Isolation, Durability)
- Indexing and query optimization
- Database transactions and concurrency control
Interviewers will expect you to have a strong grasp of these concepts, as they form the building blocks of effective database design.
2. Types of Questions You May Encounter
Database design interviews typically include a mix of theoretical questions and practical design problems. Here are some common types of questions you might face:
2.1. Conceptual Questions
These questions test your understanding of database design principles and concepts. Examples include:
- What is normalization, and why is it important?
- Explain the difference between OLTP and OLAP systems.
- What are the ACID properties, and why are they crucial for database transactions?
- Describe the differences between clustered and non-clustered indexes.
2.2. SQL Queries
You may be asked to write SQL queries to demonstrate your proficiency in working with relational databases. These questions can range from simple SELECT statements to complex joins and subqueries. For example:
-- Write a SQL query to find the top 5 customers by total purchase amount
SELECT customer_id, SUM(purchase_amount) AS total_amount
FROM purchases
GROUP BY customer_id
ORDER BY total_amount DESC
LIMIT 5;
2.3. Database Schema Design
One of the most common types of questions in database design interviews involves creating a database schema for a given scenario. You might be asked to design a schema for systems like:
- An e-commerce platform
- A social media application
- A library management system
- A hotel reservation system
For these questions, you’ll need to identify entities, relationships, and attributes, and create an appropriate schema that efficiently represents the data and supports the required operations.
2.4. System Design and Scalability
Especially for more senior roles or positions at large tech companies, you may be asked about designing database systems that can handle large-scale data and high traffic. These questions might cover topics such as:
- Sharding and partitioning strategies
- Replication and data consistency
- Caching mechanisms
- Handling eventual consistency in distributed systems
2.5. Performance Optimization
Interviewers may present you with scenarios where a database is experiencing performance issues and ask you to identify potential problems and suggest optimizations. This could involve:
- Analyzing and optimizing slow queries
- Designing appropriate indexes
- Identifying and resolving bottlenecks in database operations
3. Preparing for the Interview
To excel in a database design interview, consider the following preparation strategies:
3.1. Review Fundamental Concepts
Ensure you have a solid understanding of database design principles, SQL, and related concepts. Review textbooks, online resources, and documentation for popular database management systems like MySQL, PostgreSQL, or Oracle.
3.2. Practice SQL Queries
Regularly practice writing SQL queries, focusing on both simple and complex scenarios. Use online platforms like LeetCode, HackerRank, or SQL Zoo to sharpen your skills.
3.3. Study Real-World Database Designs
Analyze and understand the database designs of popular applications or systems. This will give you insights into how large-scale databases are structured and optimized.
3.4. Work on Sample Design Problems
Practice designing database schemas for various scenarios. Start with simple systems and gradually move to more complex ones. Consider factors like scalability, performance, and data integrity in your designs.
3.5. Stay Updated on Industry Trends
Keep abreast of the latest developments in database technologies, including NoSQL databases, distributed systems, and cloud-based database solutions.
4. Common Interview Scenarios and How to Approach Them
Let’s explore some common interview scenarios and how to tackle them effectively:
4.1. Designing an E-commerce Database
Scenario: Design a database schema for an e-commerce platform that supports product listings, user accounts, orders, and reviews.
Approach:
- Identify the main entities: Users, Products, Orders, Reviews
- Define relationships: Users can place Orders, Orders contain Products, Users can write Reviews for Products
- Create tables with appropriate columns and data types
- Establish primary and foreign key relationships
- Consider additional features like inventory management, categories, and payment processing
Example schema (simplified):
CREATE TABLE Users (
user_id INT PRIMARY KEY,
username VARCHAR(50) UNIQUE,
email VARCHAR(100) UNIQUE,
password_hash VARCHAR(255),
created_at TIMESTAMP
);
CREATE TABLE Products (
product_id INT PRIMARY KEY,
name VARCHAR(100),
description TEXT,
price DECIMAL(10, 2),
stock_quantity INT,
category_id INT
);
CREATE TABLE Orders (
order_id INT PRIMARY KEY,
user_id INT,
order_date TIMESTAMP,
total_amount DECIMAL(10, 2),
status VARCHAR(20),
FOREIGN KEY (user_id) REFERENCES Users(user_id)
);
CREATE TABLE OrderItems (
order_item_id INT PRIMARY KEY,
order_id INT,
product_id INT,
quantity INT,
price DECIMAL(10, 2),
FOREIGN KEY (order_id) REFERENCES Orders(order_id),
FOREIGN KEY (product_id) REFERENCES Products(product_id)
);
CREATE TABLE Reviews (
review_id INT PRIMARY KEY,
user_id INT,
product_id INT,
rating INT,
comment TEXT,
created_at TIMESTAMP,
FOREIGN KEY (user_id) REFERENCES Users(user_id),
FOREIGN KEY (product_id) REFERENCES Products(product_id)
);
4.2. Optimizing a Slow Query
Scenario: You’re given a slow-performing query and asked to optimize it.
Approach:
- Analyze the query execution plan
- Identify potential bottlenecks (e.g., full table scans, inefficient joins)
- Consider adding appropriate indexes
- Rewrite the query if necessary (e.g., using subqueries or CTEs)
- Evaluate the impact of your changes on query performance
Example:
-- Original slow query
SELECT o.order_id, o.order_date, u.username, SUM(oi.quantity * oi.price) AS total_amount
FROM Orders o
JOIN Users u ON o.user_id = u.user_id
JOIN OrderItems oi ON o.order_id = oi.order_id
WHERE o.order_date > '2023-01-01'
GROUP BY o.order_id, o.order_date, u.username
ORDER BY total_amount DESC;
-- Optimized query (assuming appropriate indexes are in place)
SELECT o.order_id, o.order_date, u.username, o.total_amount
FROM Orders o
JOIN Users u ON o.user_id = u.user_id
WHERE o.order_date > '2023-01-01'
ORDER BY o.total_amount DESC;
In this example, we’ve moved the calculation of total_amount to the Orders table (assuming it’s pre-calculated and stored) and eliminated the need for the JOIN with OrderItems, potentially improving query performance significantly.
4.3. Designing a Scalable Social Media Database
Scenario: Design a database schema for a social media platform that can handle millions of users, posts, and interactions.
Approach:
- Identify core entities: Users, Posts, Comments, Likes, Friendships
- Consider sharding strategies for large tables (e.g., Users, Posts)
- Implement denormalization where appropriate to improve read performance
- Design for eventual consistency in distributed scenarios
- Plan for efficient data retrieval patterns (e.g., user timelines, trending posts)
Example schema and considerations:
-- Users table (sharded by user_id)
CREATE TABLE Users (
user_id BIGINT PRIMARY KEY,
username VARCHAR(50) UNIQUE,
email VARCHAR(100) UNIQUE,
password_hash VARCHAR(255),
created_at TIMESTAMP
);
-- Posts table (sharded by post_id)
CREATE TABLE Posts (
post_id BIGINT PRIMARY KEY,
user_id BIGINT,
content TEXT,
created_at TIMESTAMP,
likes_count INT,
comments_count INT,
FOREIGN KEY (user_id) REFERENCES Users(user_id)
);
-- Comments table (sharded by post_id)
CREATE TABLE Comments (
comment_id BIGINT PRIMARY KEY,
post_id BIGINT,
user_id BIGINT,
content TEXT,
created_at TIMESTAMP,
FOREIGN KEY (post_id) REFERENCES Posts(post_id),
FOREIGN KEY (user_id) REFERENCES Users(user_id)
);
-- Likes table (sharded by post_id)
CREATE TABLE Likes (
like_id BIGINT PRIMARY KEY,
post_id BIGINT,
user_id BIGINT,
created_at TIMESTAMP,
FOREIGN KEY (post_id) REFERENCES Posts(post_id),
FOREIGN KEY (user_id) REFERENCES Users(user_id)
);
-- Friendships table (sharded by user_id)
CREATE TABLE Friendships (
friendship_id BIGINT PRIMARY KEY,
user_id1 BIGINT,
user_id2 BIGINT,
status VARCHAR(20),
created_at TIMESTAMP,
FOREIGN KEY (user_id1) REFERENCES Users(user_id),
FOREIGN KEY (user_id2) REFERENCES Users(user_id)
);
Additional considerations:
- Implement a caching layer (e.g., Redis) for frequently accessed data like user profiles and post metadata
- Use a message queue (e.g., Kafka) for processing likes, comments, and other high-volume interactions
- Consider using a graph database for managing complex relationships and friend recommendations
- Implement a search index (e.g., Elasticsearch) for efficient content discovery
5. Tips for Success in Database Design Interviews
To increase your chances of success in a database design interview, keep these tips in mind:
5.1. Communicate Clearly
Explain your thought process as you work through problems. This gives the interviewer insight into your problem-solving approach and allows them to provide guidance if needed.
5.2. Ask Clarifying Questions
Don’t hesitate to ask for more information or clarification about the problem statement. This shows that you’re thorough and helps ensure you’re solving the right problem.
5.3. Consider Trade-offs
In database design, there are often multiple valid approaches. Be prepared to discuss the pros and cons of different design decisions and explain why you chose a particular approach.
5.4. Think About Scalability
Even for seemingly simple problems, consider how your solution would scale to handle large amounts of data or high traffic. This is particularly important for interviews at large tech companies.
5.5. Be Familiar with Different Database Types
While relational databases are common in interviews, be prepared to discuss other types of databases (e.g., NoSQL, NewSQL) and when they might be appropriate.
5.6. Practice Good Database Design Principles
Demonstrate your knowledge of best practices such as normalization, proper indexing, and efficient query design throughout the interview.
5.7. Stay Calm and Manage Your Time
Database design problems can be complex. Stay calm, break the problem down into manageable parts, and keep an eye on the time to ensure you cover all aspects of the question.
6. Conclusion
Database design interviews can be challenging, but with proper preparation and practice, you can approach them with confidence. Focus on building a strong foundation in database concepts, practice designing schemas for various scenarios, and stay updated on current trends in database technologies.
Remember that interviewers are not just looking for technically correct answers, but also for your ability to think critically, communicate effectively, and approach problems systematically. By demonstrating these skills along with your database design expertise, you’ll be well-positioned to succeed in your interview and land that dream job at a top tech company.
As you prepare, take advantage of resources like AlgoCademy, which offers interactive coding tutorials and AI-powered assistance to help you develop your skills in algorithmic thinking and problem-solving. These skills are invaluable not just for database design interviews, but for your overall growth as a technology professional.
Good luck with your database design interview preparation!