Why Your Debugging Process is Actually a Form of Meditation
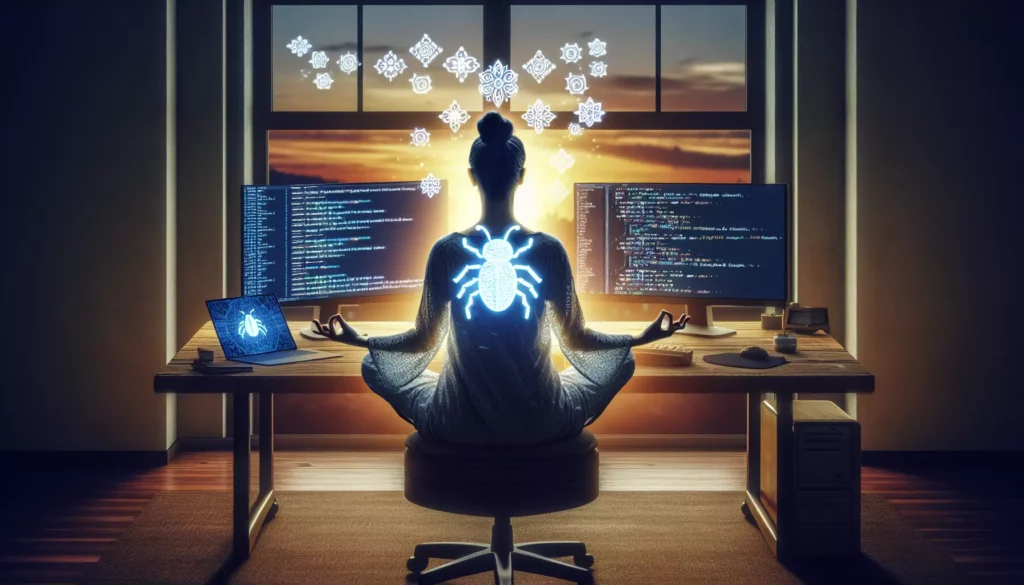
As software developers, we often find ourselves lost in the intricate maze of code, hunting down elusive bugs that seem to defy logic. This process, known as debugging, is an essential part of our daily routine. But have you ever stopped to consider that your debugging process might actually be a form of meditation? In this article, we’ll explore the surprising parallels between debugging and mindfulness practices, and how embracing this perspective can not only improve your coding skills but also contribute to your overall well-being.
The Zen of Debugging
At first glance, debugging might seem like the antithesis of meditation. It’s often associated with frustration, long hours, and mental strain. However, when we look closer, we can see that the core elements of effective debugging align remarkably well with meditative practices:
- Focused attention
- Present-moment awareness
- Non-judgmental observation
- Patience and persistence
- Letting go of attachments
Let’s dive deeper into each of these aspects and see how they manifest in both debugging and meditation.
Focused Attention: The Debugger’s Superpower
In meditation, practitioners are often instructed to focus their attention on a single point of reference, such as their breath or a mantra. This singular focus helps to quiet the mind and bring about a state of heightened awareness. Similarly, when debugging, we must narrow our focus to a specific part of the code or a particular behavior of the program.
Consider this scenario: You’re working on a complex web application, and suddenly, a user reports that the login functionality is broken. As you begin your debugging process, you start by focusing your attention on the authentication flow. This targeted approach is not unlike a meditator zeroing in on their breath, letting all other distractions fall away.
function authenticateUser(username, password) {
// Your attention is fully focused here
if (username === '' || password === '') {
return false;
}
// More authentication logic...
}
By maintaining this laser-like focus, you’re more likely to spot subtle issues that might otherwise go unnoticed. This level of concentration can lead to a state of flow, where time seems to slip away, and you become fully immersed in the task at hand – a state often described in meditative practices.
Present-Moment Awareness: Debugging in the Now
One of the core tenets of mindfulness meditation is staying present in the current moment. This means not getting caught up in thoughts about the past or worries about the future. In debugging, this translates to focusing on the current state of your code and its behavior, rather than getting bogged down by past mistakes or future implications.
When you’re knee-deep in a debugging session, you’re essentially practicing present-moment awareness. You’re observing the code as it is right now, watching its execution step by step, and analyzing its current output. This intense focus on the present can be incredibly grounding and can help you maintain clarity in the face of complex problems.
console.log('Current value of x:', x);
// Output: Current value of x: undefined
// You're fully present, observing the actual state
// rather than assuming what it should be
By staying rooted in the present moment of your code’s execution, you’re more likely to catch subtle bugs and unexpected behaviors that might elude a less focused developer.
Non-Judgmental Observation: The Key to Unbiased Debugging
In meditation, practitioners are encouraged to observe their thoughts and feelings without judgment. This non-judgmental stance allows for a clearer perception of reality. In debugging, adopting a similar approach can be incredibly beneficial.
When you encounter a bug, it’s easy to fall into patterns of self-criticism or frustration. However, by observing the code and its behavior without emotional attachment, you can maintain a clearer perspective and make more rational decisions about how to proceed.
// Instead of thinking: "This code is terrible! Who wrote this mess?"
// Observe without judgment: "This function is returning unexpected results.
// Let's examine why."
function calculateTotal(items) {
let total = 0;
for (let i = 0; i < items.length; i++) {
total += items[i].price;
}
return total;
}
By approaching debugging with a non-judgmental mindset, you’re more likely to see the code for what it is, rather than what you think it should be. This can lead to more accurate diagnoses of problems and more effective solutions.
Patience and Persistence: The Long Game of Debugging and Meditation
Both debugging and meditation require a great deal of patience and persistence. In meditation, you might sit for long periods, gently bringing your attention back to your breath each time your mind wanders. Similarly, in debugging, you often need to patiently work through various possibilities, methodically testing and eliminating hypotheses until you find the root cause of the issue.
This process can be time-consuming and sometimes frustrating, but it’s essential for both practices. In debugging, as in meditation, quick fixes are rare. The real breakthroughs often come after sustained effort and careful observation.
// A patient, persistent approach to debugging
function debugLoginIssue() {
console.log('Step 1: Checking user input validation');
// Test and observe...
console.log('Step 2: Verifying database connection');
// Test and observe...
console.log('Step 3: Examining authentication logic');
// Test and observe...
// Continue until the issue is resolved...
}
By cultivating patience and persistence in your debugging process, you’re not only more likely to solve complex problems but also developing valuable skills that translate to other areas of your life and work.
Letting Go of Attachments: The Art of Debugging Detachment
In meditation, practitioners learn to let go of attachments to thoughts, emotions, and outcomes. This principle is equally valuable in debugging. Often, we become attached to our initial hypotheses about what’s causing a bug, or we cling to certain parts of our code that we’re particularly proud of. However, effective debugging requires us to let go of these attachments and remain open to all possibilities.
Consider a scenario where you’ve written a beautiful, elegant function that you’re certain is working correctly. However, the bug persists, and all signs point to this function as the culprit. The ability to let go of your attachment to your code and objectively consider that it might be the source of the problem is crucial for effective debugging.
// Your beautifully crafted function
function calculateCompoundInterest(principal, rate, time, n) {
return principal * Math.pow((1 + rate / n), (n * time));
}
// Letting go of attachment and critically examining it
function debugCalculateCompoundInterest() {
console.log('Testing with known values...');
const result = calculateCompoundInterest(1000, 0.05, 5, 12);
console.log('Expected: 1283.36, Actual:', result.toFixed(2));
// If the result is unexpected, be willing to revise your function
}
By practicing this form of detachment in your debugging process, you’ll become more adaptable and open-minded, leading to quicker resolution of issues and more robust code overall.
The Mindful Debugger: Cultivating Awareness in Code
As we’ve seen, the parallels between debugging and meditation are numerous and significant. By approaching your debugging process with a mindful attitude, you can not only improve your coding skills but also cultivate valuable mental habits that extend beyond your work as a developer.
Here are some practical ways to incorporate mindfulness into your debugging routine:
- Start with a breath: Before diving into a debugging session, take a few deep breaths to center yourself and set your intention for the task ahead.
- Practice single-tasking: Resist the urge to multitask while debugging. Give your full attention to the problem at hand, just as you would in a meditation session.
- Use mindful logging: Instead of haphazardly sprinkling console.log statements throughout your code, approach logging with intention and awareness. Each log should serve a specific purpose in your debugging process.
- Take regular breaks: Just as in meditation, it’s important to give your mind regular rest periods during intense debugging sessions. Use these breaks to reset your focus and approach the problem with fresh eyes.
- Reflect on your process: After resolving a bug, take a moment to reflect on your debugging journey. What worked well? What could you improve? This reflective practice can enhance both your coding skills and your self-awareness.
// Example of mindful logging
function mindfulDebugging() {
console.log('=== Beginning mindful debugging session ===');
console.log('Observing initial state:', getCurrentState());
console.log('Executing function with awareness:');
const result = executeFunction();
console.log('Mindfully examining result:', result);
console.log('Reflecting on unexpected outcomes:');
if (result !== expectedResult) {
console.log('Unexpected result. Investigating further...');
// Further debugging steps...
}
console.log('=== Concluding mindful debugging session ===');
}
The Broader Impact: Debugging as a Life Skill
The mindful approach to debugging doesn’t just make you a better coder; it can have far-reaching effects on your overall problem-solving abilities and mental well-being. The skills you develop through mindful debugging – focused attention, present-moment awareness, non-judgmental observation, patience, persistence, and the ability to let go of attachments – are valuable in many aspects of life.
Consider how these skills might apply to:
- Troubleshooting issues in your personal relationships
- Approaching complex problems in other areas of your work or studies
- Managing stress and anxiety in your daily life
- Improving your decision-making processes
- Enhancing your overall emotional intelligence
By viewing your debugging process as a form of meditation, you’re not just honing your coding skills; you’re cultivating a mindset that can lead to greater clarity, resilience, and effectiveness in all areas of your life.
Conclusion: Embracing the Meditative Nature of Debugging
As we’ve explored throughout this article, the process of debugging shares many similarities with meditation practices. By recognizing and embracing these parallels, we can transform what is often seen as a frustrating and stressful task into an opportunity for growth, both as developers and as individuals.
The next time you find yourself deep in a debugging session, try to approach it with the mindset of a meditator. Focus your attention, stay present in the moment, observe without judgment, cultivate patience and persistence, and practice letting go of attachments. You may find that not only do you become a more effective debugger, but you also experience a sense of calm and clarity that extends well beyond your coding work.
Remember, every bug you encounter is not just a problem to be solved, but an opportunity to practice mindfulness and deepen your understanding of both your code and yourself. Happy debugging, and may your code be ever mindful!
Further Reading and Resources
If you’re interested in exploring the intersection of mindfulness and coding further, here are some resources you might find helpful:
- “The Zen Programmer” by Christian Grobmeier
- “Mindful Tech: How to Bring Balance to Our Digital Lives” by David M. Levy
- “The Pragmatic Programmer: Your Journey to Mastery” by Andrew Hunt and David Thomas (which touches on the importance of mindfulness in programming)
- Headspace or Calm apps for guided meditations that you can incorporate into your coding routine
- Online courses on mindfulness for programmers, available on platforms like Udemy or Coursera
By integrating mindfulness practices into your debugging process and overall approach to coding, you’ll not only become a more effective developer but also cultivate valuable skills that will serve you well in all aspects of your life. So the next time you’re faced with a particularly challenging bug, take a deep breath, center yourself, and remember: your debugging process is actually a form of meditation. Embrace it, and watch both your code and your mind transform.