The Art of Using LeetCode Problems to Predict Election Outcomes
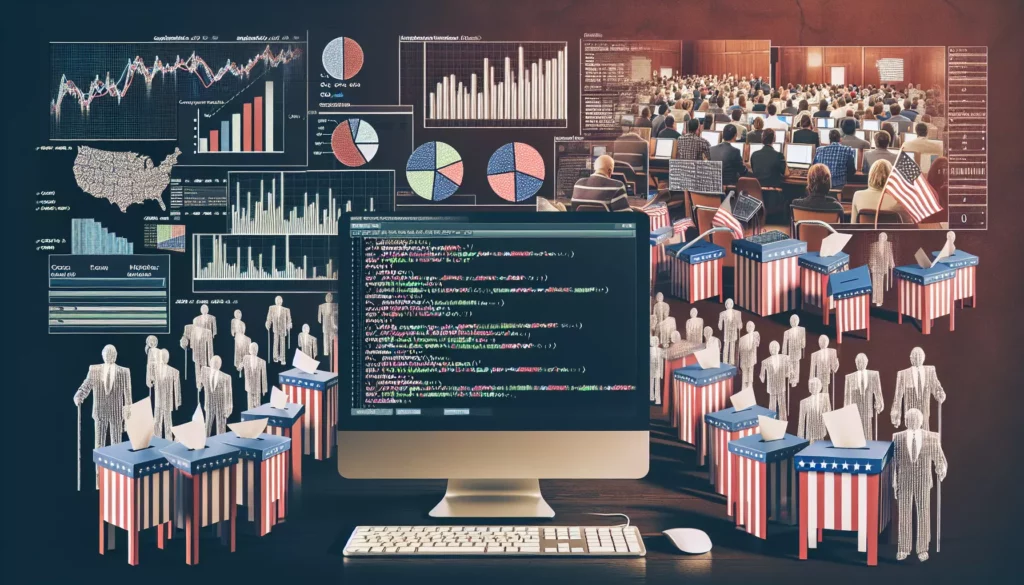
In the ever-evolving landscape of technology and politics, an unexpected convergence has emerged: the potential use of LeetCode problems to predict election outcomes. While this may sound like an outlandish concept at first, the underlying principles of algorithmic thinking and data analysis that are honed through solving LeetCode problems can indeed be applied to the complex world of electoral predictions. In this extensive exploration, we’ll delve into how the skills developed through coding practice on platforms like AlgoCademy can be leveraged to analyze and forecast political trends.
The Intersection of Coding and Politics
At first glance, coding challenges and political forecasting might seem worlds apart. However, both domains rely heavily on pattern recognition, data analysis, and problem-solving skills. LeetCode, a popular platform for coding practice, offers a wide array of algorithmic problems that inadvertently train developers in skills applicable to electoral analysis:
- Data structures for organizing voter information
- Algorithms for processing large datasets
- Pattern recognition in voting behaviors
- Optimization techniques for resource allocation in campaigns
These skills, when applied to the political sphere, can offer unique insights into voter trends and potential election outcomes.
Leveraging Data Structures for Voter Analysis
One of the fundamental aspects of both LeetCode problems and election prediction is the efficient organization and manipulation of data. Let’s explore how common data structures used in coding challenges can be applied to electoral analysis:
Arrays and Voter Demographics
Arrays, the most basic data structure, can be used to store and analyze voter demographics. For instance, a simple array could represent different age groups of voters:
const voterAgeGroups = [
18-24,
25-34,
35-44,
45-54,
55-64,
65+
];
By storing voter counts in such arrays, we can quickly access and manipulate demographic data, which is crucial for understanding the composition of the electorate.
Hash Tables for Rapid Lookup
Hash tables, often featured in LeetCode problems for their O(1) lookup time, can be invaluable in election prediction. They can be used to store and retrieve voter information quickly:
const voterInfo = {
"John Doe": {
age: 35,
party: "Independent",
votingHistory: [2016, 2018, 2020]
},
"Jane Smith": {
age: 42,
party: "Democrat",
votingHistory: [2018, 2020]
}
// ... more voters
};
This structure allows for instant access to individual voter profiles, which can be crucial when analyzing voting patterns or predicting turnout.
Graphs for Relationship Mapping
Graph problems are common on LeetCode, and their applications in electoral analysis are profound. Graphs can represent relationships between voters, influencers, and political entities:
class Voter {
constructor(name) {
this.name = name;
this.connections = [];
}
addConnection(voter) {
this.connections.push(voter);
}
}
const alice = new Voter("Alice");
const bob = new Voter("Bob");
const charlie = new Voter("Charlie");
alice.addConnection(bob);
bob.addConnection(charlie);
By mapping these relationships, we can analyze how information and influence spread through voter networks, potentially affecting election outcomes.
Algorithmic Approaches to Electoral Prediction
The algorithms practiced in LeetCode challenges can be adapted to solve complex problems in election prediction. Let’s examine how some common algorithmic paradigms can be applied:
Dynamic Programming for Trend Analysis
Dynamic programming, often used to solve optimization problems on LeetCode, can be applied to analyze voting trends over time. For example, we could use it to predict future voter turnout based on historical data:
function predictTurnout(pastTurnouts, factors) {
const n = pastTurnouts.length;
const dp = new Array(n).fill(0);
dp[0] = pastTurnouts[0];
for (let i = 1; i < n; i++) {
dp[i] = dp[i-1] * factors[i];
}
return dp[n-1];
}
const pastTurnouts = [0.6, 0.58, 0.62, 0.57];
const factors = [1, 1.02, 0.98, 1.05];
const predictedTurnout = predictTurnout(pastTurnouts, factors);
console.log(`Predicted turnout: ${predictedTurnout}`);
This simplified example demonstrates how dynamic programming can be used to make predictions based on historical data and influencing factors.
Greedy Algorithms for Resource Allocation
Greedy algorithms, which make locally optimal choices at each step, can be applied to optimize campaign resource allocation. For instance, deciding which districts to focus on for maximum impact:
function optimizeCampaignAllocation(districts, budget) {
districts.sort((a, b) => b.impactPerDollar - a.impactPerDollar);
let remainingBudget = budget;
const allocation = [];
for (const district of districts) {
if (remainingBudget >= district.cost) {
allocation.push(district);
remainingBudget -= district.cost;
}
}
return allocation;
}
const districts = [
{ name: "District A", cost: 1000, impactPerDollar: 5 },
{ name: "District B", cost: 1500, impactPerDollar: 4 },
{ name: "District C", cost: 800, impactPerDollar: 6 }
];
const budget = 2000;
const optimalAllocation = optimizeCampaignAllocation(districts, budget);
console.log("Optimal allocation:", optimalAllocation);
This greedy approach ensures that resources are allocated to districts with the highest impact per dollar spent, maximizing the campaign’s effectiveness.
Machine Learning Algorithms for Voter Behavior Prediction
While not typically covered in LeetCode problems, machine learning algorithms are an extension of the algorithmic thinking developed through coding practice. These can be particularly powerful in predicting voter behavior:
// Pseudo-code for a simple logistic regression model
class VoterPredictionModel {
constructor() {
this.weights = [0, 0, 0]; // age, income, education
this.bias = 0;
}
train(voterData, outcomes) {
// Training logic here
}
predict(voter) {
const z = this.weights[0] * voter.age +
this.weights[1] * voter.income +
this.weights[2] * voter.education +
this.bias;
return 1 / (1 + Math.exp(-z)); // Sigmoid function
}
}
const model = new VoterPredictionModel();
model.train(trainingData, trainingOutcomes);
const newVoter = { age: 35, income: 50000, education: 16 };
const likelihoodToVote = model.predict(newVoter);
console.log(`Likelihood to vote: ${likelihoodToVote}`);
This simplified model demonstrates how machine learning concepts can be applied to predict individual voting behavior based on demographic factors.
Pattern Recognition in Voting Behaviors
Pattern recognition is a crucial skill developed through solving LeetCode problems, and it’s equally important in electoral analysis. Let’s explore how this skill can be applied to identify voting patterns:
Time Series Analysis
Analyzing voting patterns over time can reveal trends and cycles in electoral behavior. This is similar to problems involving sequence analysis in coding challenges:
function analyzeVotingTrends(votingHistory) {
const trends = {};
for (let i = 1; i < votingHistory.length; i++) {
const change = votingHistory[i] - votingHistory[i-1];
if (trends[change]) {
trends[change]++;
} else {
trends[change] = 1;
}
}
return trends;
}
const partyAVotes = [45, 48, 47, 52, 50, 53];
const trends = analyzeVotingTrends(partyAVotes);
console.log("Voting trends:", trends);
This function identifies common changes in voting percentages, which could indicate recurring patterns in voter behavior.
Clustering Voters
Clustering algorithms, often used in data analysis, can be applied to group voters with similar characteristics or behaviors:
function kMeansClustering(voters, k, maxIterations = 100) {
// Initialize k random centroids
let centroids = initializeCentroids(voters, k);
for (let i = 0; i < maxIterations; i++) {
// Assign each voter to the nearest centroid
const clusters = assignToClusters(voters, centroids);
// Recalculate centroids
const newCentroids = recalculateCentroids(clusters);
// Check for convergence
if (centroidsEqual(centroids, newCentroids)) {
break;
}
centroids = newCentroids;
}
return centroids;
}
// Helper functions would be implemented here
const voters = [
{ age: 25, income: 30000 },
{ age: 35, income: 50000 },
{ age: 45, income: 70000 },
// ... more voters
];
const k = 3; // Number of clusters
const voterSegments = kMeansClustering(voters, k);
console.log("Voter segments:", voterSegments);
This clustering approach can help identify distinct voter segments, allowing for more targeted campaign strategies.
Optimization Techniques for Campaign Strategies
Optimization problems are a staple of LeetCode, and these skills directly translate to optimizing campaign strategies. Let’s look at some applications:
Knapsack Problem for Budget Allocation
The classic knapsack problem can be adapted to optimize budget allocation across different campaign activities:
function optimizeCampaignBudget(activities, totalBudget) {
const n = activities.length;
const dp = Array(n + 1).fill().map(() => Array(totalBudget + 1).fill(0));
for (let i = 1; i <= n; i++) {
for (let w = 1; w <= totalBudget; w++) {
if (activities[i-1].cost <= w) {
dp[i][w] = Math.max(
activities[i-1].impact + dp[i-1][w - activities[i-1].cost],
dp[i-1][w]
);
} else {
dp[i][w] = dp[i-1][w];
}
}
}
return dp[n][totalBudget];
}
const campaignActivities = [
{ name: "TV Ads", cost: 1000, impact: 500 },
{ name: "Social Media", cost: 500, impact: 300 },
{ name: "Door-to-door", cost: 300, impact: 200 },
{ name: "Phone Banking", cost: 200, impact: 150 }
];
const campaignBudget = 1500;
const maxImpact = optimizeCampaignBudget(campaignActivities, campaignBudget);
console.log(`Maximum campaign impact: ${maxImpact}`);
This approach ensures that the campaign budget is allocated to maximize overall impact.
Graph Algorithms for Influence Mapping
Graph algorithms can be used to map and analyze influence networks within the electorate:
class Voter {
constructor(id) {
this.id = id;
this.influencedBy = new Set();
}
addInfluencer(voter) {
this.influencedBy.add(voter);
}
}
function findKeyInfluencers(voters) {
const influenceCount = new Map();
for (const voter of voters) {
for (const influencer of voter.influencedBy) {
influenceCount.set(influencer, (influenceCount.get(influencer) || 0) + 1);
}
}
return Array.from(influenceCount.entries())
.sort((a, b) => b[1] - a[1])
.slice(0, 5); // Top 5 influencers
}
const voters = [
new Voter(1),
new Voter(2),
new Voter(3),
new Voter(4),
new Voter(5)
];
voters[1].addInfluencer(voters[0]);
voters[2].addInfluencer(voters[0]);
voters[3].addInfluencer(voters[1]);
voters[4].addInfluencer(voters[0]);
const keyInfluencers = findKeyInfluencers(voters);
console.log("Key influencers:", keyInfluencers);
Identifying key influencers can help campaigns focus their efforts on individuals who have the most significant impact on others’ voting decisions.
Challenges and Ethical Considerations
While the application of coding skills to election prediction offers exciting possibilities, it’s crucial to address the challenges and ethical considerations that come with this approach:
Data Privacy and Security
Handling voter data requires strict adherence to privacy laws and ethical guidelines. Developers must implement robust security measures to protect sensitive information:
class VoterDatabase {
constructor() {
this.voters = new Map();
}
addVoter(id, data) {
// Encrypt sensitive data before storing
const encryptedData = this.encryptData(data);
this.voters.set(id, encryptedData);
}
getVoter(id) {
const encryptedData = this.voters.get(id);
if (encryptedData) {
// Decrypt data before returning
return this.decryptData(encryptedData);
}
return null;
}
encryptData(data) {
// Implementation of encryption algorithm
}
decryptData(encryptedData) {
// Implementation of decryption algorithm
}
}
const voterDB = new VoterDatabase();
voterDB.addVoter("12345", { name: "John Doe", age: 35 });
const voter = voterDB.getVoter("12345");
console.log(voter);
This example demonstrates a basic approach to securing voter data through encryption.
Bias in Algorithms
Algorithms can inadvertently perpetuate or amplify biases present in the data. It’s crucial to implement checks and balances to identify and mitigate these biases:
function checkForBias(predictions, demographics) {
const biasByGroup = new Map();
for (let i = 0; i < predictions.length; i++) {
const group = demographics[i];
if (!biasByGroup.has(group)) {
biasByGroup.set(group, { count: 0, sum: 0 });
}
const groupStats = biasByGroup.get(group);
groupStats.count++;
groupStats.sum += predictions[i];
}
for (const [group, stats] of biasByGroup) {
const avgPrediction = stats.sum / stats.count;
console.log(`Average prediction for ${group}: ${avgPrediction}`);
}
}
const predictions = [0.7, 0.6, 0.8, 0.5, 0.9];
const demographics = ["A", "B", "A", "B", "C"];
checkForBias(predictions, demographics);
This function helps identify potential biases in predictions across different demographic groups.
Transparency and Explainability
As algorithms become more complex, ensuring transparency and explainability in election predictions becomes crucial. Developers should strive to create models that can be understood and audited by non-technical stakeholders:
class ExplainableVoterModel {
constructor() {
this.features = ["age", "income", "education"];
this.weights = [0.3, 0.4, 0.3]; // Simplified weights
}
predict(voter) {
let score = 0;
for (let i = 0; i < this.features.length; i++) {
score += voter[this.features[i]] * this.weights[i];
}
return score;
}
explainPrediction(voter) {
const prediction = this.predict(voter);
console.log(`Overall prediction score: ${prediction}`);
console.log("Feature contributions:");
for (let i = 0; i < this.features.length; i++) {
const contribution = voter[this.features[i]] * this.weights[i];
console.log(`${this.features[i]}: ${contribution}`);
}
}
}
const model = new ExplainableVoterModel();
const voter = { age: 35, income: 50000, education: 16 };
model.explainPrediction(voter);
This model provides a breakdown of how each feature contributes to the final prediction, enhancing transparency.
Conclusion
The intersection of coding skills honed through platforms like AlgoCademy and LeetCode with the complex world of election prediction opens up fascinating possibilities. By applying data structures, algorithms, and problem-solving techniques learned through coding challenges, we can develop innovative approaches to understanding and forecasting electoral outcomes.
However, as we’ve explored, this powerful combination comes with significant responsibilities. Developers venturing into this field must be acutely aware of the ethical implications of their work, prioritizing data privacy, algorithmic fairness, and transparency in their models.
As technology continues to evolve, the synergy between coding education and political analysis will likely grow stronger. Platforms like AlgoCademy play a crucial role in equipping the next generation of developers with the skills needed to tackle these complex, real-world challenges. By fostering a deep understanding of algorithms and data structures, coupled with an awareness of their broader implications, we can harness the power of code to enhance our democratic processes while maintaining the integrity and fairness of our electoral systems.
The art of using LeetCode problems to predict election outcomes is not just about technical prowess; it’s about responsible innovation that respects the fundamental principles of democracy. As we continue to explore this exciting frontier, let us do so with a commitment to ethical practices, transparency, and the betterment of our political processes.