How to Prepare for a DevOps Interview: A Comprehensive Guide
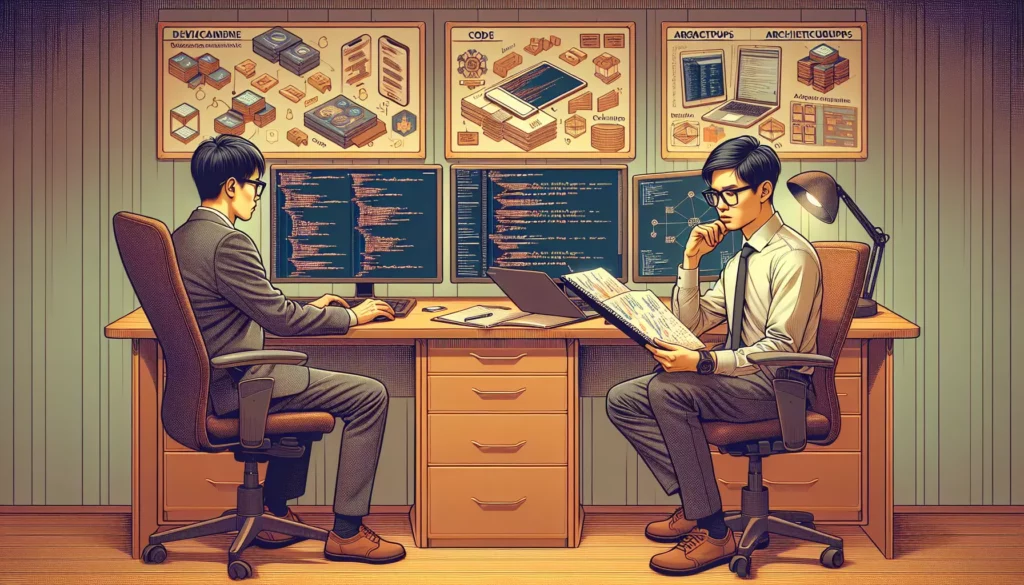
As the technology landscape continues to evolve, DevOps has become an increasingly crucial role in many organizations. If you’re gearing up for a DevOps interview, you’re in the right place. This comprehensive guide will walk you through the essential steps to prepare for your DevOps interview, ensuring you’re ready to showcase your skills and land that dream job.
Understanding DevOps: The Foundation of Your Preparation
Before diving into specific interview preparation strategies, it’s crucial to have a solid understanding of what DevOps is and why it’s important. DevOps is a set of practices that combines software development (Dev) and IT operations (Ops) to shorten the systems development life cycle and provide continuous delivery with high software quality.
Key aspects of DevOps include:
- Continuous Integration and Continuous Delivery (CI/CD)
- Infrastructure as Code (IaC)
- Configuration Management
- Containerization and Orchestration
- Monitoring and Logging
- Collaboration and Communication
Understanding these core concepts will form the foundation of your DevOps knowledge and help you navigate the interview process more effectively.
Essential Skills for DevOps Professionals
To excel in a DevOps role and ace your interview, you should be proficient in the following areas:
1. Programming and Scripting
While you don’t need to be an expert programmer, having a good grasp of scripting languages is essential. Focus on:
- Python
- Bash scripting
- PowerShell (for Windows environments)
Be prepared to write simple scripts or explain code snippets during your interview. For example, you might be asked to write a bash script to automate a repetitive task:
#!/bin/bash
# Script to backup files older than 30 days
find /path/to/files -type f -mtime +30 -exec cp {} /path/to/backup \;
echo "Backup completed successfully!"
2. Version Control Systems
Proficiency in Git is a must-have skill for any DevOps professional. Be familiar with:
- Basic Git commands
- Branching strategies
- Pull requests and code reviews
- Git workflows (e.g., Gitflow, GitHub Flow)
3. Continuous Integration and Continuous Delivery (CI/CD)
Understanding CI/CD pipelines is crucial. Be prepared to discuss:
- Popular CI/CD tools (Jenkins, GitLab CI, CircleCI)
- Pipeline design and implementation
- Automated testing strategies
- Deployment strategies (Blue-Green, Canary, Rolling updates)
4. Configuration Management and Infrastructure as Code (IaC)
Familiarize yourself with tools like:
- Ansible
- Puppet
- Chef
- Terraform
Be ready to explain how these tools work and provide examples of how you’ve used them in previous projects.
5. Containerization and Orchestration
Docker and Kubernetes are essential technologies in the DevOps world. Make sure you understand:
- Docker basics (images, containers, Dockerfiles)
- Container orchestration with Kubernetes
- Kubernetes concepts (pods, services, deployments)
6. Cloud Platforms
Familiarity with at least one major cloud platform is often required. Focus on:
- Amazon Web Services (AWS)
- Microsoft Azure
- Google Cloud Platform (GCP)
Understand core services, networking, and security concepts for your chosen platform.
7. Monitoring and Logging
Be prepared to discuss:
- Monitoring tools (Prometheus, Grafana, Nagios)
- Log management (ELK stack, Splunk)
- Alerting and incident response
Preparing for Common DevOps Interview Questions
While every interview is unique, there are some common questions you’re likely to encounter. Here are some examples and tips on how to approach them:
1. “Explain the concept of DevOps and its benefits.”
This is your chance to demonstrate your understanding of DevOps philosophy. Highlight the following points:
- Improved collaboration between development and operations teams
- Faster time-to-market for new features
- Increased reliability and stability of systems
- Better resource utilization
- Continuous improvement through feedback loops
2. “Describe a CI/CD pipeline you’ve implemented.”
When answering this question, structure your response as follows:
- Briefly describe the project and its requirements
- Explain the tools and technologies you used
- Walk through the stages of your pipeline (e.g., build, test, deploy)
- Highlight any challenges you faced and how you overcame them
- Discuss the outcomes and benefits of the implemented pipeline
3. “How would you handle a situation where a critical bug is discovered in production?”
This question assesses your problem-solving skills and your approach to incident management. Consider the following steps in your response:
- Assess the severity and impact of the bug
- Communicate with relevant stakeholders
- Implement a temporary fix or rollback if necessary
- Investigate the root cause
- Develop and test a permanent solution
- Deploy the fix through the proper CI/CD pipeline
- Conduct a post-mortem analysis to prevent similar issues in the future
4. “Explain the concept of Infrastructure as Code and its benefits.”
When answering this question, focus on:
- Definition of IaC (managing and provisioning infrastructure through code rather than manual processes)
- Benefits: consistency, version control, automation, scalability
- Popular tools: Terraform, CloudFormation, Ansible
- Real-world examples of how IaC improves DevOps workflows
5. “How do you ensure security in a DevOps environment?”
This question allows you to demonstrate your understanding of DevSecOps principles. Consider discussing:
- Integrating security checks into the CI/CD pipeline
- Implementing infrastructure and application scanning tools
- Managing secrets and credentials securely
- Applying the principle of least privilege
- Regular security audits and penetration testing
- Fostering a security-aware culture within the team
Technical Challenges and Coding Exercises
Many DevOps interviews include technical challenges or coding exercises. Here are some types of challenges you might encounter and how to prepare for them:
1. Scripting Exercises
You may be asked to write a script to solve a specific problem. For example:
“Write a Python script that monitors a log file for errors and sends an alert if a certain threshold is exceeded.”
Here’s a sample solution:
import time
import smtplib
from email.mime.text import MIMEText
def monitor_log(log_file, error_threshold):
error_count = 0
with open(log_file, 'r') as f:
f.seek(0, 2) # Go to the end of the file
while True:
line = f.readline()
if not line:
time.sleep(0.1) # Sleep briefly
continue
if "ERROR" in line:
error_count += 1
if error_count >= error_threshold:
send_alert(f"Error threshold exceeded: {error_count} errors found")
error_count = 0 # Reset the count
def send_alert(message):
sender = "your_email@example.com"
recipient = "admin@example.com"
msg = MIMEText(message)
msg['Subject'] = "Log Error Alert"
msg['From'] = sender
msg['To'] = recipient
with smtplib.SMTP('smtp.gmail.com', 587) as server:
server.starttls()
server.login(sender, "your_password")
server.send_message(msg)
if __name__ == "__main__":
monitor_log("/path/to/logfile.log", 5)
2. System Design Questions
You might be asked to design a scalable and resilient system. For example:
“Design a highly available web application that can handle millions of users.”
When approaching this type of question:
- Clarify requirements and constraints
- Outline the high-level architecture
- Discuss specific components (load balancers, caching, databases)
- Address scalability, reliability, and security concerns
- Explain monitoring and maintenance strategies
3. Troubleshooting Scenarios
You may be presented with a problematic scenario and asked how you would troubleshoot it. For example:
“A web application is experiencing intermittent slowdowns. How would you investigate and resolve the issue?”
Approach this type of question methodically:
- Gather information (logs, metrics, user reports)
- Check for recent changes or deployments
- Investigate potential bottlenecks (database, network, application code)
- Use monitoring tools to identify patterns
- Propose and test potential solutions
- Implement a fix and monitor for improvement
- Document the issue and solution for future reference
Soft Skills and Cultural Fit
While technical skills are crucial, don’t underestimate the importance of soft skills in a DevOps role. Be prepared to discuss:
- Collaboration and communication skills
- Problem-solving approach
- Ability to work in a fast-paced environment
- Continuous learning and adaptability
- Experience with Agile methodologies
Many interviewers will ask behavioral questions to assess these skills. Use the STAR method (Situation, Task, Action, Result) to structure your responses and provide concrete examples from your past experiences.
Researching the Company and Role
Before your interview, thoroughly research the company and the specific role you’re applying for. This will help you tailor your responses and ask informed questions. Consider:
- The company’s tech stack and DevOps practices
- Recent projects or initiatives related to DevOps
- The company’s values and culture
- Industry trends and challenges relevant to the organization
Practical Tips for Interview Day
- Test your technology: Ensure your internet connection, camera, and microphone are working properly for virtual interviews.
- Prepare your environment: Choose a quiet, well-lit space for the interview.
- Have relevant materials ready: Keep your resume, portfolio, and any notes nearby.
- Be punctual: Arrive early or log in to the virtual meeting room ahead of time.
- Stay calm and composed: Take deep breaths and pause before answering questions if needed.
- Ask thoughtful questions: Prepare questions about the role, team, and company to show your genuine interest.
- Follow up: Send a thank-you email after the interview, reiterating your interest in the position.
Conclusion
Preparing for a DevOps interview requires a combination of technical knowledge, practical experience, and soft skills. By focusing on the key areas outlined in this guide and practicing your responses to common questions, you’ll be well-equipped to showcase your abilities and land your dream DevOps role.
Remember, the field of DevOps is constantly evolving, so demonstrate your passion for continuous learning and improvement throughout the interview process. Good luck with your preparation, and may your next DevOps interview be a resounding success!