Why Your Code Efficiency is Inversely Proportional to Your Social Life
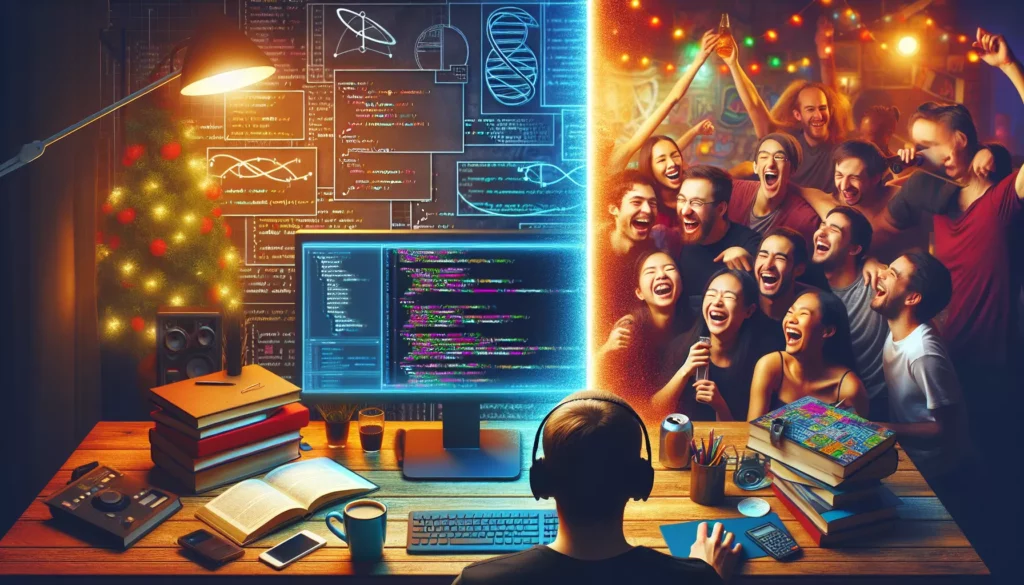
In the world of programming, there’s an unspoken rule that often rings true: the more efficient your code becomes, the less efficient your social life tends to be. As developers, we often find ourselves caught in a paradox where our pursuit of optimized algorithms and cleaner code inadvertently leads to a decline in our social interactions. This phenomenon, while not universal, is common enough to warrant exploration. In this comprehensive guide, we’ll delve into the reasons behind this inverse relationship, its implications, and how to strike a balance between coding prowess and social well-being.
The Coder’s Dilemma: Efficiency vs. Social Life
Let’s start by acknowledging the elephant in the room: coding is time-consuming. Whether you’re a beginner tackling your first “Hello, World!” program or a seasoned developer optimizing complex algorithms, the process of writing, testing, and refining code can eat up hours, days, or even weeks of your time. This time investment is often necessary to achieve the level of efficiency and elegance that we, as programmers, strive for in our work.
However, this dedication to our craft can come at a cost. The more time we spend hunched over our keyboards, the less time we have for social interactions, hobbies, and other activities that contribute to a well-rounded life. It’s not uncommon for developers to find themselves canceling plans with friends or missing out on social events because they’re “in the zone” or working on a particularly challenging problem.
The Pursuit of Code Efficiency
To understand why code efficiency can be so all-consuming, let’s break down what it entails:
- Algorithmic Optimization: Finding the most efficient way to solve a problem often requires exploring multiple approaches and analyzing their time and space complexities.
- Code Refactoring: Improving the structure and readability of existing code without changing its functionality.
- Performance Tuning: Identifying and eliminating bottlenecks to enhance the speed and responsiveness of applications.
- Memory Management: Ensuring efficient use of system resources, particularly in languages without automatic garbage collection.
- Continuous Learning: Staying up-to-date with new programming languages, frameworks, and best practices.
Each of these aspects demands significant time and mental energy. For example, consider the process of optimizing a sorting algorithm. A naive implementation of bubble sort might look like this:
def bubble_sort(arr):
n = len(arr)
for i in range(n):
for j in range(0, n - i - 1):
if arr[j] > arr[j + 1]:
arr[j], arr[j + 1] = arr[j + 1], arr[j]
return arr
While this works, it’s not the most efficient solution. A more optimized version might include early termination:
def optimized_bubble_sort(arr):
n = len(arr)
for i in range(n):
swapped = False
for j in range(0, n - i - 1):
if arr[j] > arr[j + 1]:
arr[j], arr[j + 1] = arr[j + 1], arr[j]
swapped = True
if not swapped:
break
return arr
This optimization can significantly reduce the number of comparisons for nearly sorted arrays. However, achieving this level of efficiency often requires hours of thought, experimentation, and testing – time that could otherwise be spent socializing.
The Social Cost of Coding Excellence
As we dive deeper into the world of efficient coding, several factors contribute to the deterioration of our social lives:
1. Time Consumption
The most obvious factor is time. Writing efficient code often requires extended periods of uninterrupted focus. This “deep work” state, while productive, can lead to neglecting social commitments and relationships.
2. Mental Fatigue
Coding, especially when dealing with complex algorithms or system architectures, can be mentally exhausting. After a long day of problem-solving, many developers find themselves too drained to engage in social activities.
3. Irregular Work Hours
The nature of coding often leads to irregular work hours. Debugging sessions can stretch late into the night, and inspiration for solving a problem might strike at odd hours. This unpredictability can make it challenging to maintain a consistent social schedule.
4. The “Just One More Bug” Syndrome
It’s common for programmers to fall into the trap of wanting to fix “just one more bug” or implement “one more feature” before calling it a day. This tendency can lead to missed social opportunities and strained relationships.
5. The Solitary Nature of Coding
While pair programming and collaborative coding exist, much of a developer’s work is solitary. This isolation can become habitual, making it harder to transition into social situations.
The Paradox of Productivity
Interestingly, the inverse relationship between code efficiency and social life creates a paradox of productivity. On one hand, dedicating more time to coding can lead to more efficient algorithms and cleaner code. On the other hand, a lack of social interaction and work-life balance can negatively impact overall productivity and creativity.
Research has shown that social interactions and diverse experiences can enhance problem-solving skills and spark creative thinking. By isolating ourselves in pursuit of code efficiency, we might actually be hindering our ability to come up with innovative solutions.
The Impact on Career Growth
While technical skills are crucial in the world of software development, soft skills and networking also play a significant role in career advancement. The ability to communicate effectively, work in teams, and build professional relationships can be just as important as writing efficient code.
Consider the following scenario: You’ve spent months perfecting an algorithm that reduces the time complexity of a critical operation from O(n^2) to O(n log n). It’s a significant achievement, but if you can’t effectively communicate the value of this optimization to non-technical stakeholders or collaborate with your team to integrate it into the larger system, its impact may be limited.
Striking a Balance: Code Efficiency and Social Well-being
So, how can we maintain our pursuit of code efficiency without completely sacrificing our social lives? Here are some strategies to help strike a balance:
1. Set Boundaries
Establish clear work hours and stick to them. When it’s time to socialize or relax, resist the urge to check your code or tinker with that nagging bug.
2. Practice Time Management
Use techniques like the Pomodoro Technique to structure your work time and ensure you’re taking regular breaks. This can help prevent burnout and make time for social activities.
3. Join Coding Communities
Participate in local meetups, hackathons, or online forums. This allows you to socialize with like-minded individuals while still engaging with your passion for coding.
4. Embrace Pair Programming
Pair programming not only can lead to more efficient code but also provides a social element to your coding practice.
5. Prioritize Self-care
Regular exercise, proper nutrition, and adequate sleep are crucial for both your coding efficiency and your ability to engage socially.
6. Schedule Social Activities
Treat social engagements with the same level of commitment as you would a coding deadline. Put them in your calendar and honor these commitments.
7. Cultivate Non-Coding Hobbies
Having interests outside of coding can provide a much-needed mental break and opportunities for social interaction.
The Role of Coding Education Platforms
Platforms like AlgoCademy play a crucial role in helping developers balance their pursuit of coding excellence with maintaining a healthy social life. Here’s how:
1. Structured Learning Paths
By providing well-organized curricula and learning paths, these platforms help developers manage their time more effectively. Instead of spending countless hours searching for resources or getting lost in tangential topics, learners can focus on a clear progression of skills.
2. Interactive Tutorials
Interactive coding exercises and tutorials offer a more engaging learning experience. This can make the learning process more efficient, potentially reducing the overall time commitment required to master new concepts.
3. Community Features
Many coding education platforms include community forums or discussion boards. These features allow learners to interact with peers, ask questions, and share knowledge, adding a social element to the learning process.
4. Time-Boxed Challenges
Timed coding challenges and competitions can help developers practice working efficiently within set time limits. This skill can translate to better time management in their regular coding practices.
5. Real-World Project Simulations
By offering projects that simulate real-world scenarios, these platforms help developers practice not just coding, but also the soft skills required in professional settings. This can include teamwork, communication, and project management.
The Importance of Algorithmic Thinking
One of the key focuses of platforms like AlgoCademy is developing algorithmic thinking skills. This approach to problem-solving can actually help improve both code efficiency and time management. Here’s how:
1. Problem Decomposition
Algorithmic thinking teaches you to break down complex problems into smaller, manageable parts. This skill is invaluable not just in coding, but also in organizing your time and tasks.
2. Efficiency Mindset
By constantly considering the efficiency of your algorithms, you develop a mindset that seeks optimization in all areas of life, including how you manage your time and social commitments.
3. Pattern Recognition
Algorithmic thinking enhances your ability to recognize patterns. This can help you identify inefficiencies in your daily routines and social interactions, allowing you to optimize your life beyond just your code.
For example, consider the classic problem of finding the maximum subarray sum. A naive approach might look like this:
def max_subarray_sum_naive(arr):
n = len(arr)
max_sum = float('-inf')
for i in range(n):
for j in range(i, n):
current_sum = sum(arr[i:j+1])
max_sum = max(max_sum, current_sum)
return max_sum
This solution has a time complexity of O(n^3). However, applying algorithmic thinking and recognizing patterns can lead to a much more efficient solution using Kadane’s algorithm:
def max_subarray_sum_kadane(arr):
max_sum = current_sum = arr[0]
for num in arr[1:]:
current_sum = max(num, current_sum + num)
max_sum = max(max_sum, current_sum)
return max_sum
This optimized solution has a time complexity of O(n), a significant improvement. The same principle of recognizing patterns and seeking optimizations can be applied to how we manage our time and social interactions.
The Future of Coding and Social Balance
As the field of software development continues to evolve, so too does our understanding of the importance of work-life balance. Future trends that may help address the inverse relationship between code efficiency and social life include:
1. AI-Assisted Coding
Advancements in AI and machine learning are leading to more sophisticated code completion and generation tools. These could potentially reduce the time required for routine coding tasks, freeing up more time for social activities.
2. Remote Work and Flexible Schedules
The increasing acceptance of remote work and flexible schedules in the tech industry may allow developers more freedom to balance their coding pursuits with social engagements.
3. Emphasis on Soft Skills
As the importance of soft skills in tech careers becomes more recognized, we may see a shift in education and workplace cultures to encourage a more balanced approach to skill development.
4. Wellness Initiatives in Tech Companies
More tech companies are implementing wellness programs and encouraging work-life balance, recognizing the long-term benefits for both employees and the company.
Conclusion
The inverse relationship between code efficiency and social life is a real challenge faced by many developers. However, it’s not an insurmountable one. By recognizing the importance of balance, utilizing resources like coding education platforms effectively, and applying the problem-solving skills we use in coding to our personal lives, we can strive for both coding excellence and a fulfilling social life.
Remember, the most efficient code is not always the one that runs the fastest, but the one that solves the problem effectively while allowing its creator to maintain a healthy, balanced life. As we continue to push the boundaries of what’s possible in software development, let’s not forget to also develop our abilities to connect, communicate, and engage with the world beyond our screens.
In the end, the true measure of a great developer isn’t just the efficiency of their code, but their ability to contribute positively to their team, their community, and their own well-being. So the next time you’re tempted to skip a social event to optimize that algorithm, remember: sometimes the most efficient solution is to step away from the keyboard and engage with the world around you. Your code, and your life, will be better for it.