How to Use Sorting Algorithms to Organize Your Life
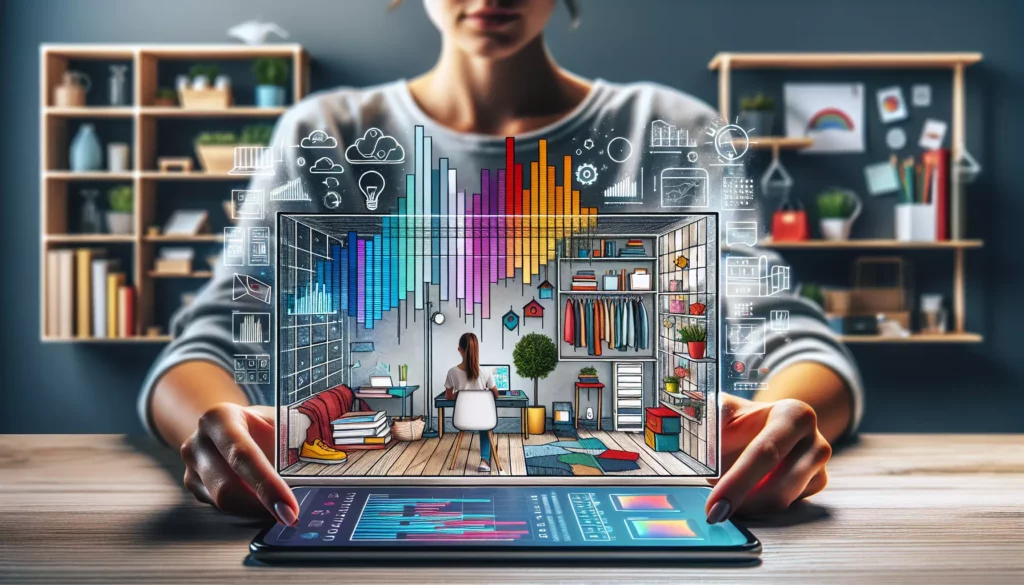
In the world of computer science, sorting algorithms are fundamental tools used to arrange data in a specific order. But did you know that these same principles can be applied to organize various aspects of your life? In this comprehensive guide, we’ll explore how sorting algorithms can be leveraged to bring order to your daily routines, boost productivity, and streamline decision-making processes. Let’s dive into the world of algorithmic thinking and discover how it can transform your personal and professional life.
Understanding Sorting Algorithms
Before we delve into practical applications, let’s briefly review some common sorting algorithms and their characteristics:
1. Bubble Sort
Bubble Sort is a simple sorting algorithm that repeatedly steps through the list, compares adjacent elements, and swaps them if they’re in the wrong order. While not the most efficient for large datasets, it’s easy to understand and implement.
def bubble_sort(arr):
n = len(arr)
for i in range(n):
for j in range(0, n - i - 1):
if arr[j] > arr[j + 1]:
arr[j], arr[j + 1] = arr[j + 1], arr[j]
return arr
2. Insertion Sort
Insertion Sort builds the final sorted array one item at a time. It’s efficient for small datasets and is often used when the input array is nearly sorted.
def insertion_sort(arr):
for i in range(1, len(arr)):
key = arr[i]
j = i - 1
while j >= 0 and key < arr[j]:
arr[j + 1] = arr[j]
j -= 1
arr[j + 1] = key
return arr
3. Quick Sort
Quick Sort is an efficient, divide-and-conquer algorithm that works by selecting a ‘pivot’ element and partitioning the array around it. It’s widely used due to its average-case time complexity of O(n log n).
def quick_sort(arr):
if len(arr) <= 1:
return arr
pivot = arr[len(arr) // 2]
left = [x for x in arr if x < pivot]
middle = [x for x in arr if x == pivot]
right = [x for x in arr if x > pivot]
return quick_sort(left) + middle + quick_sort(right)
4. Merge Sort
Merge Sort is another divide-and-conquer algorithm that divides the input array into two halves, recursively sorts them, and then merges the two sorted halves. It’s known for its stable sorting and predictable performance.
def merge_sort(arr):
if len(arr) <= 1:
return arr
mid = len(arr) // 2
left = merge_sort(arr[:mid])
right = merge_sort(arr[mid:])
return merge(left, right)
def merge(left, right):
result = []
i, j = 0, 0
while i < len(left) and j < len(right):
if left[i] <= right[j]:
result.append(left[i])
i += 1
else:
result.append(right[j])
j += 1
result.extend(left[i:])
result.extend(right[j:])
return result
Applying Sorting Algorithms to Daily Life
Now that we’ve refreshed our understanding of sorting algorithms, let’s explore how we can apply these concepts to various aspects of our lives:
1. Prioritizing Tasks with Insertion Sort
Insertion Sort can be an excellent model for prioritizing your daily tasks. Here’s how you can apply it:
- Start with your to-do list as an unsorted array.
- Take each task and “insert” it into its proper place based on priority.
- As you go through your day, new tasks can be efficiently inserted into the correct position.
This method works well because it allows for easy updates as new tasks come in, much like how Insertion Sort efficiently handles new elements in a nearly sorted list.
Example Implementation:
class Task:
def __init__(self, description, priority):
self.description = description
self.priority = priority
def task_insertion_sort(tasks):
for i in range(1, len(tasks)):
key = tasks[i]
j = i - 1
while j >= 0 and key.priority < tasks[j].priority:
tasks[j + 1] = tasks[j]
j -= 1
tasks[j + 1] = key
return tasks
# Usage
tasks = [
Task("Answer emails", 3),
Task("Prepare presentation", 1),
Task("Buy groceries", 4),
Task("Schedule meeting", 2)
]
sorted_tasks = task_insertion_sort(tasks)
for task in sorted_tasks:
print(f"{task.priority}: {task.description}")
2. Organizing Your Wardrobe with Bubble Sort
Bubble Sort’s simplicity makes it a great analogy for organizing your wardrobe:
- Start at one end of your closet.
- Compare adjacent items, swapping them if they’re out of order (e.g., by color, season, or formality).
- Repeat this process until you’ve gone through the entire closet without making any swaps.
This method is particularly useful for maintaining order in a small wardrobe or when you only need to make minor adjustments to your current organization.
Example Implementation:
def color_value(color):
color_order = ['red', 'orange', 'yellow', 'green', 'blue', 'indigo', 'violet']
return color_order.index(color.lower()) if color.lower() in color_order else len(color_order)
def wardrobe_bubble_sort(clothes):
n = len(clothes)
for i in range(n):
for j in range(0, n - i - 1):
if color_value(clothes[j]) > color_value(clothes[j + 1]):
clothes[j], clothes[j + 1] = clothes[j + 1], clothes[j]
return clothes
# Usage
wardrobe = ['Blue shirt', 'Red dress', 'Green pants', 'Yellow sweater', 'Indigo jacket']
sorted_wardrobe = wardrobe_bubble_sort(wardrobe)
print("Organized wardrobe:", sorted_wardrobe)
3. Decision Making with Quick Sort
Quick Sort’s divide-and-conquer approach can be adapted for complex decision-making processes:
- Choose a “pivot” criterion for your decision (e.g., cost, time, or impact).
- Divide your options into three categories: better than the pivot, equal to the pivot, and worse than the pivot.
- Recursively apply this process to the “better” and “worse” categories until you’ve narrowed down your options.
This method is particularly useful when faced with many options and multiple criteria to consider.
Example Implementation:
class Option:
def __init__(self, name, value):
self.name = name
self.value = value
def decision_quick_sort(options):
if len(options) <= 1:
return options
pivot = options[len(options) // 2].value
left = [x for x in options if x.value < pivot]
middle = [x for x in options if x.value == pivot]
right = [x for x in options if x.value > pivot]
return decision_quick_sort(left) + middle + decision_quick_sort(right)
# Usage
options = [
Option("Option A", 7),
Option("Option B", 3),
Option("Option C", 9),
Option("Option D", 5),
Option("Option E", 1)
]
sorted_options = decision_quick_sort(options)
print("Ranked options:")
for option in sorted_options:
print(f"{option.name}: {option.value}")
4. Long-term Planning with Merge Sort
Merge Sort’s methodical approach can be applied to long-term planning and goal setting:
- Break down your long-term goals into smaller, manageable sub-goals.
- Organize and prioritize these sub-goals independently.
- Merge these organized sub-goals back into a cohesive, prioritized plan.
This method is excellent for complex, multi-faceted projects or life plans that require careful organization and prioritization.
Example Implementation:
class Goal:
def __init__(self, description, priority):
self.description = description
self.priority = priority
def merge_goals(left, right):
result = []
i, j = 0, 0
while i < len(left) and j < len(right):
if left[i].priority <= right[j].priority:
result.append(left[i])
i += 1
else:
result.append(right[j])
j += 1
result.extend(left[i:])
result.extend(right[j:])
return result
def goal_merge_sort(goals):
if len(goals) <= 1:
return goals
mid = len(goals) // 2
left = goal_merge_sort(goals[:mid])
right = goal_merge_sort(goals[mid:])
return merge_goals(left, right)
# Usage
goals = [
Goal("Learn a new language", 3),
Goal("Start a business", 1),
Goal("Run a marathon", 4),
Goal("Write a book", 2),
Goal("Travel to 10 countries", 5)
]
sorted_goals = goal_merge_sort(goals)
print("Prioritized long-term goals:")
for goal in sorted_goals:
print(f"{goal.priority}: {goal.description}")
Benefits of Applying Sorting Algorithms to Life Organization
Incorporating sorting algorithms into your life organization strategies offers several advantages:
- Improved Efficiency: By systematically organizing tasks, belongings, and goals, you can reduce time spent on decision-making and searching for items.
- Better Priority Management: Sorting algorithms help you clearly identify and focus on high-priority items, ensuring that important tasks or goals don’t get overlooked.
- Scalability: These methods can be applied to both small, daily tasks and large, long-term projects, making them versatile tools for life organization.
- Logical Approach to Chaos: When faced with overwhelming options or tasks, sorting algorithms provide a structured approach to breaking down and organizing the chaos.
- Continuous Improvement: Like optimizing code, you can refine your organizational systems over time, leading to increasingly efficient processes.
Challenges and Considerations
While applying sorting algorithms to life organization can be beneficial, it’s important to consider some potential challenges:
- Oversimplification: Life is often more complex than a simple array of elements. Some situations may require more nuanced approaches.
- Time Investment: Initially, implementing these systems may require significant time and effort. However, the long-term benefits often outweigh the initial investment.
- Flexibility: Be prepared to adapt your chosen method as circumstances change. What works well in one situation may not be ideal in another.
- Balancing Structure and Spontaneity: While organization is beneficial, it’s important to leave room for flexibility and spontaneity in your life.
Advanced Applications: Combining Algorithms
As you become more comfortable with applying sorting algorithms to your life, you can start combining different approaches for more complex organizational challenges. Here are a few examples:
1. Hybrid Task Management System
Combine Insertion Sort for daily task management with Merge Sort for weekly or monthly planning:
- Use Insertion Sort to maintain a prioritized daily to-do list, easily adding new tasks as they arise.
- At the end of each week, use Merge Sort to consolidate and prioritize tasks for the upcoming week, merging completed tasks, ongoing projects, and new initiatives.
Example Implementation:
class Task:
def __init__(self, description, priority, deadline):
self.description = description
self.priority = priority
self.deadline = deadline
def daily_insertion_sort(tasks):
# Implementation as before
def weekly_merge_sort(tasks):
# Implementation as before
# Usage
daily_tasks = [Task("Reply to emails", 2, "today"), Task("Client meeting", 1, "today")]
weekly_tasks = [Task("Project proposal", 3, "Friday"), Task("Team review", 2, "Thursday")]
sorted_daily = daily_insertion_sort(daily_tasks)
sorted_weekly = weekly_merge_sort(weekly_tasks)
all_tasks = sorted_daily + sorted_weekly
final_sorted = weekly_merge_sort(all_tasks)
for task in final_sorted:
print(f"{task.priority}: {task.description} (Due: {task.deadline})")
2. Multi-criteria Decision Making
Use Quick Sort for initial filtering, followed by a weighted scoring system for final decisions:
- Apply Quick Sort to quickly eliminate options that don’t meet certain criteria.
- For the remaining options, use a more detailed scoring system that considers multiple factors.
Example Implementation:
class Option:
def __init__(self, name, cost, quality, convenience):
self.name = name
self.cost = cost
self.quality = quality
self.convenience = convenience
def quick_sort_by_cost(options):
# Implementation of Quick Sort based on cost
def weighted_score(option, weights):
return (option.cost * weights['cost'] +
option.quality * weights['quality'] +
option.convenience * weights['convenience'])
# Usage
options = [
Option("A", cost=100, quality=8, convenience=7),
Option("B", cost=80, quality=7, convenience=9),
Option("C", cost=120, quality=9, convenience=6),
Option("D", cost=90, quality=6, convenience=8)
]
# First, quick sort by cost
cost_sorted = quick_sort_by_cost(options)
# Then, apply weighted scoring to top 3 options
weights = {'cost': 0.5, 'quality': 0.3, 'convenience': 0.2}
top_options = cost_sorted[:3]
final_scores = [(option, weighted_score(option, weights)) for option in top_options]
final_scores.sort(key=lambda x: x[1], reverse=True)
print("Best option:", final_scores[0][0].name)
Integrating Algorithmic Thinking into Daily Life
Beyond specific applications of sorting algorithms, the broader concept of algorithmic thinking can be immensely beneficial in various aspects of life:
1. Problem Decomposition
Just as complex algorithms break problems into smaller, manageable parts, apply this approach to tackle large life challenges:
- Break down big goals into smaller, actionable steps.
- Identify the core components of a problem before attempting to solve it.
2. Pattern Recognition
Algorithms often rely on recognizing and exploiting patterns. In daily life, this translates to:
- Identifying recurring issues or bottlenecks in your routines.
- Recognizing successful strategies and replicating them in different contexts.
3. Efficiency Optimization
Just as we optimize algorithms for better performance, continuously look for ways to optimize your daily processes:
- Regularly review and refine your organizational systems.
- Automate repetitive tasks where possible.
- Eliminate unnecessary steps in your routines.
4. Data-Driven Decision Making
Algorithms work with data, and so should your life decisions:
- Collect and analyze data on your habits, productivity, and goals.
- Use this data to make informed decisions and adjustments.
Conclusion: The Algorithm of Life
Applying sorting algorithms and algorithmic thinking to life organization is more than just a novel approach to productivity—it’s a way of developing a systematic, efficient, and adaptable approach to navigating the complexities of modern life. By breaking down overwhelming tasks, prioritizing effectively, and continuously optimizing our processes, we can create a more organized, productive, and fulfilling life.
Remember, the goal isn’t to turn your life into a rigid, programmed routine, but rather to use these tools as a framework for making better decisions, managing time more effectively, and achieving your goals more efficiently. Like any good algorithm, your life organization system should be flexible, allowing for updates and optimizations as you grow and your circumstances change.
As you implement these strategies, you’ll likely find that the skills you develop—logical thinking, problem decomposition, and systematic approach to challenges—will benefit you not just in personal organization, but in many other areas of life and work. The beauty of algorithmic thinking is its universality; once mastered, it becomes a powerful tool for tackling a wide array of life’s challenges.
So, why not start today? Choose one area of your life that could benefit from better organization, select an appropriate “algorithm,” and begin the process of transformation. You might be surprised at how a little computational thinking can lead to significant improvements in your daily life. After all, if algorithms can sort vast amounts of data in milliseconds, imagine what they can do for your to-do list, career goals, or life ambitions.
Remember, the most efficient algorithm for organizing your life is the one that works best for you. Don’t be afraid to experiment, iterate, and optimize your approach over time. Happy sorting!