How to Effectively Communicate Your Thought Process During Interviews
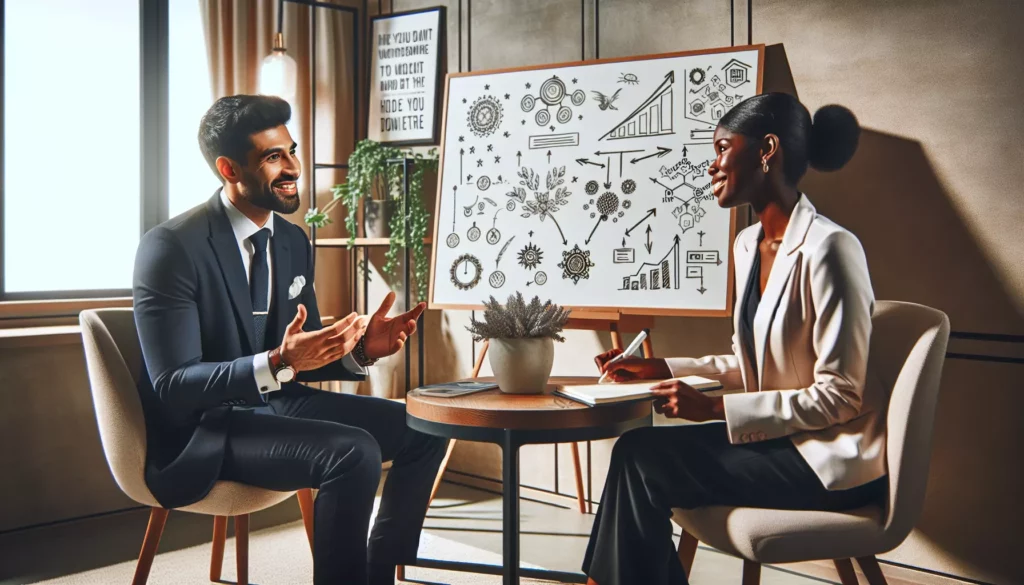
In the competitive world of tech interviews, particularly for coveted positions at FAANG (Facebook, Amazon, Apple, Netflix, Google) companies, your coding skills are just part of the equation. Equally important is your ability to effectively communicate your thought process as you tackle complex problems. This skill can make the difference between landing your dream job and falling short. In this comprehensive guide, we’ll explore strategies to articulate your thinking clearly and confidently during technical interviews, helping you showcase not just what you know, but how you approach problem-solving.
Why Communication Matters in Technical Interviews
Before diving into the specifics of how to communicate effectively, it’s crucial to understand why this skill is so valued by interviewers. Here are some key reasons:
- Insight into your problem-solving approach: Interviewers want to see how you think, not just the final solution you arrive at.
- Collaboration skills: Clear communication is essential for working in teams, a critical aspect of most tech roles.
- Ability to explain complex concepts: This skill is valuable when working with non-technical stakeholders or explaining your work to others.
- Adaptability: How you respond to hints or feedback during the interview process can indicate how well you’ll handle direction on the job.
Preparing for Effective Communication
Effective communication during an interview doesn’t happen by chance. It requires preparation and practice. Here are some steps you can take to prepare:
1. Practice Thinking Aloud
Get comfortable verbalizing your thoughts as you code. This can feel unnatural at first, but it’s a skill that improves with practice. Try these exercises:
- Solve coding problems on platforms like AlgoCademy while explaining your thought process out loud.
- Record yourself solving problems and review the recordings to identify areas for improvement.
- Participate in mock interviews where you can practice communicating under pressure.
2. Develop a Problem-Solving Framework
Having a structured approach to problem-solving can help you organize your thoughts and communicate more clearly. A common framework includes:
- Understand the problem
- Identify the inputs and outputs
- Consider edge cases
- Brainstorm potential approaches
- Choose an approach and explain why
- Implement the solution
- Test and refine
3. Build Your Technical Vocabulary
Familiarize yourself with technical terms relevant to the position you’re interviewing for. This will help you articulate your thoughts more precisely. Resources like AlgoCademy can help you learn and practice using the correct terminology in context.
4. Study Common Algorithms and Data Structures
Having a solid grasp of fundamental algorithms and data structures will make it easier to explain your approach to problems. Make sure you can not only implement these concepts but also explain their time and space complexity.
Strategies for Effective Communication During the Interview
Now that you’ve prepared, let’s look at specific strategies you can employ during the interview to communicate your thought process effectively:
1. Start with a High-Level Overview
Before diving into the details, give a brief overview of your approach. This helps the interviewer understand your overall strategy and shows that you can think at different levels of abstraction. For example:
“To solve this problem, I’m thinking of using a hash map to store the frequency of each element, and then iterating through the map to find the element with the highest frequency. This approach should give us a time complexity of O(n) and a space complexity of O(n) in the worst case.”
2. Think Out Loud
As you work through the problem, verbalize your thoughts. This includes:
- Explaining why you’re choosing a particular approach
- Discussing trade-offs between different solutions
- Mentioning any assumptions you’re making
- Pointing out potential edge cases or limitations of your approach
For example:
“I’m choosing to use a binary search here because we’re dealing with a sorted array, and binary search will give us a more efficient O(log n) time complexity compared to a linear search.”
3. Use Clear and Concise Language
While it’s important to be thorough, avoid rambling or using overly complex language. Aim for clarity and precision in your explanations. If you’re unsure about something, it’s better to admit it than to try to bluff your way through.
4. Visualize Your Thinking
Use diagrams or pseudocode to illustrate your ideas. This can be particularly helpful when explaining complex algorithms or data structures. For example, when explaining a tree traversal algorithm, you might draw the tree structure and walk through the traversal step-by-step.
5. Engage with the Interviewer
Remember that the interview is a two-way conversation. Engage with the interviewer by:
- Asking clarifying questions about the problem
- Checking if they want you to elaborate on any part of your explanation
- Responding thoughtfully to their hints or feedback
6. Explain Your Code as You Write It
As you implement your solution, explain what each part of your code does. This helps the interviewer follow your logic and shows that you can write clean, understandable code. For example:
// We'll use a hash map to store the frequency of each element
Map<Integer, Integer> frequencyMap = new HashMap<>();
// Iterate through the array to populate the frequency map
for (int num : nums) {
frequencyMap.put(num, frequencyMap.getOrDefault(num, 0) + 1);
}
// Now we'll find the element with the highest frequency
int maxFreq = 0;
int result = 0;
for (Map.Entry<Integer, Integer> entry : frequencyMap.entrySet()) {
if (entry.getValue() > maxFreq) {
maxFreq = entry.getValue();
result = entry.getKey();
}
}
// Return the element with the highest frequency
return result;
As you write this code, you might explain:
“I’m using a HashMap to store the frequency of each element. The key will be the element itself, and the value will be its frequency. We’ll iterate through the array once to populate this map. Then, we’ll iterate through the map to find the element with the highest frequency. This gives us a time complexity of O(n) for both operations, where n is the number of elements in the array.”
7. Discuss Time and Space Complexity
After implementing your solution, discuss its time and space complexity. This shows that you’re thinking about the efficiency of your code, not just its correctness. Be prepared to explain how you arrived at your complexity analysis.
8. Reflect on Your Solution
Once you’ve completed the problem, take a moment to reflect on your solution. Discuss:
- Potential optimizations or alternative approaches
- How the solution might scale with larger inputs
- Any trade-offs made in your implementation
This demonstrates your ability to critically evaluate your own work and consider different perspectives.
Handling Challenges During the Interview
Even with thorough preparation, you may encounter challenges during the interview. Here’s how to handle common situations:
1. When You’re Stuck
If you find yourself stuck on a problem, don’t panic. Instead:
- Communicate that you’re thinking through the problem
- Explain what you’ve considered so far and why those approaches might not work
- Ask for a hint if you’re truly stuck
For example:
“I’m considering a few approaches here. A brute force solution would be to check every pair of numbers, but that would give us O(n^2) time complexity, which isn’t ideal. I’m trying to think of a way to optimize this. Could you give me a hint about what data structure might be useful here?”
2. When You Make a Mistake
If you realize you’ve made a mistake, address it directly:
- Acknowledge the error
- Explain why it’s a mistake
- Propose a correction
This shows that you can recognize and learn from your mistakes, an important quality in any developer.
3. When You Need to Change Your Approach
If you realize midway that your initial approach isn’t optimal:
- Explain why you’re changing course
- Describe the new approach and its advantages
- Implement the new solution
This demonstrates flexibility and the ability to pivot when necessary.
Practice Makes Perfect
Effectively communicating your thought process is a skill that improves with practice. Here are some ways to hone this skill:
1. Use Coding Platforms
Platforms like AlgoCademy offer a wealth of coding problems and often provide explanations of optimal solutions. As you work through problems:
- Practice explaining your approach out loud before you start coding
- Compare your explanation to the provided solution explanations
- Pay attention to how the optimal solutions are described and try to incorporate similar language in your own explanations
2. Participate in Mock Interviews
Mock interviews provide a low-stakes environment to practice your communication skills. You can:
- Ask a friend or colleague to play the role of the interviewer
- Use online platforms that offer mock interview services
- Record your mock interviews and review them to identify areas for improvement
3. Join Coding Communities
Participating in coding communities can provide opportunities to explain your code to others and receive feedback. Consider:
- Joining online forums or discussion groups
- Contributing to open-source projects
- Attending local coding meetups or hackathons
4. Teach Others
Teaching is an excellent way to improve your ability to explain complex concepts. You could:
- Offer to tutor less experienced programmers
- Write blog posts explaining coding concepts
- Create video tutorials on coding topics
Conclusion
Effectively communicating your thought process during technical interviews is a crucial skill that can set you apart from other candidates. By preparing thoroughly, practicing regularly, and employing the strategies outlined in this guide, you can showcase not just your coding abilities, but also your problem-solving approach, your ability to articulate complex ideas, and your potential as a collaborative team member.
Remember, the goal is not just to solve the problem, but to demonstrate how you approach challenges, how you think through solutions, and how you can explain your reasoning clearly and concisely. With practice and persistence, you can master this skill and significantly improve your chances of success in technical interviews, particularly for highly competitive positions at top tech companies.
As you continue to develop your skills, platforms like AlgoCademy can provide valuable resources and practice opportunities. By combining these resources with consistent effort and a focus on clear communication, you’ll be well-prepared to tackle even the most challenging technical interviews with confidence.