How to Use Object-Oriented Programming to Understand Your Family Tree
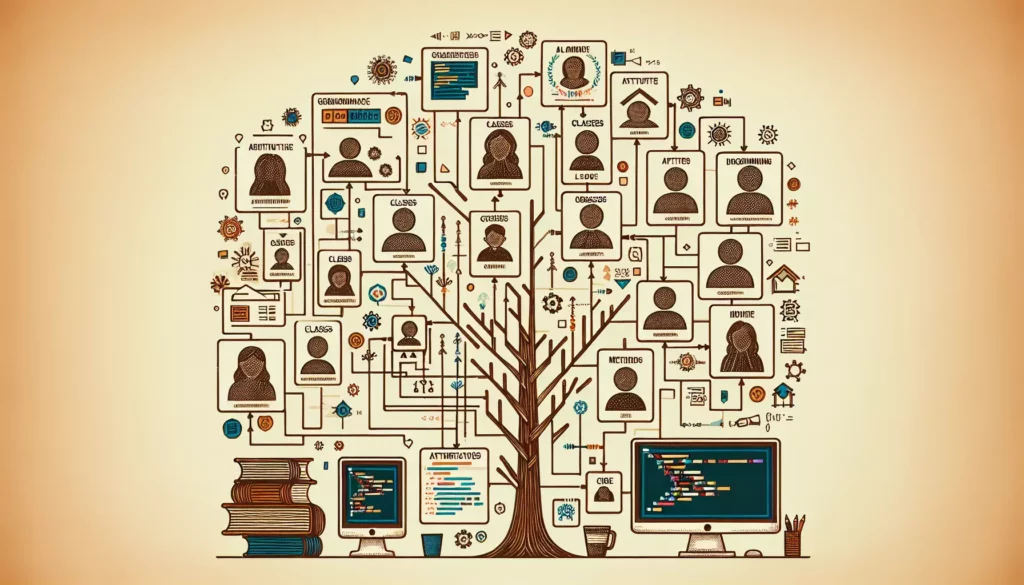
Have you ever wondered how to combine your passion for coding with your interest in genealogy? Look no further! In this comprehensive guide, we’ll explore how to use Object-Oriented Programming (OOP) principles to create a digital representation of your family tree. This approach not only helps you organize your family history but also provides an excellent opportunity to practice and understand key OOP concepts.
Table of Contents
- Introduction to OOP and Family Trees
- Defining Classes for Family Members
- Implementing Inheritance
- Encapsulation in Family Tree Management
- Polymorphism in Family Relationships
- Building the Tree Structure
- Implementing Methods for Tree Traversal and Analysis
- A Practical Example: Building Your Family Tree
- Advanced Features and Enhancements
- Conclusion
1. Introduction to OOP and Family Trees
Object-Oriented Programming is a programming paradigm that organizes code into objects, which are instances of classes. This approach is particularly well-suited for modeling real-world entities and their relationships, making it an ideal choice for representing a family tree.
In the context of a family tree, we can think of each family member as an object with properties (such as name, birth date, and gender) and methods (such as adding children or getting information about relatives). By using OOP, we can create a flexible and intuitive structure that mirrors the complexities of family relationships.
2. Defining Classes for Family Members
Let’s start by defining a basic class for a family member. This class will serve as the foundation for our family tree structure.
class FamilyMember:
def __init__(self, name, birth_date, gender):
self.name = name
self.birth_date = birth_date
self.gender = gender
self.parents = []
self.children = []
self.spouse = None
def add_child(self, child):
self.children.append(child)
child.parents.append(self)
def add_spouse(self, spouse):
self.spouse = spouse
spouse.spouse = self
def get_siblings(self):
siblings = []
for parent in self.parents:
for child in parent.children:
if child != self and child not in siblings:
siblings.append(child)
return siblings
This basic FamilyMember
class includes properties for name, birth date, gender, parents, children, and spouse. It also includes methods for adding children and spouses, as well as getting siblings.
3. Implementing Inheritance
Inheritance is a fundamental concept in OOP that allows us to create new classes based on existing ones. In the context of a family tree, we can use inheritance to represent different types of family members or to add specific functionality to certain branches of the family.
For example, let’s create a RoyalFamilyMember
class that inherits from FamilyMember
but includes additional properties and methods specific to royal lineage:
class RoyalFamilyMember(FamilyMember):
def __init__(self, name, birth_date, gender, title, reign_start=None):
super().__init__(name, birth_date, gender)
self.title = title
self.reign_start = reign_start
def get_full_title(self):
return f"{self.title} {self.name}"
def years_of_reign(self, current_year):
if self.reign_start:
return current_year - self.reign_start
return 0
This RoyalFamilyMember
class inherits all the properties and methods of FamilyMember
while adding new ones specific to royalty.
4. Encapsulation in Family Tree Management
Encapsulation is the bundling of data and the methods that operate on that data within a single unit or object. It’s a way of restricting direct access to some of an object’s components, which is a fundamental principle of data hiding in OOP.
Let’s modify our FamilyMember
class to include some private attributes and provide public methods to access and modify them:
class FamilyMember:
def __init__(self, name, birth_date, gender):
self._name = name
self._birth_date = birth_date
self._gender = gender
self._parents = []
self._children = []
self._spouse = None
def get_name(self):
return self._name
def set_name(self, name):
self._name = name
def get_birth_date(self):
return self._birth_date
def set_birth_date(self, birth_date):
self._birth_date = birth_date
def add_child(self, child):
self._children.append(child)
child._parents.append(self)
def get_children(self):
return self._children.copy() # Return a copy to prevent direct modification
# ... other methods ...
By using underscores to denote private attributes and providing public methods to access and modify them, we’ve implemented encapsulation. This allows us to control how the data is accessed and modified, potentially adding validation or additional logic in the future without changing the public interface of the class.
5. Polymorphism in Family Relationships
Polymorphism allows objects of different types to be treated as objects of a common base class. In our family tree context, we can use polymorphism to handle different types of family members uniformly.
Let’s create a method that demonstrates polymorphism:
def print_family_member_info(family_member):
print(f"Name: {family_member.get_name()}")
print(f"Birth Date: {family_member.get_birth_date()}")
if isinstance(family_member, RoyalFamilyMember):
print(f"Title: {family_member.get_full_title()}")
print(f"Years of Reign: {family_member.years_of_reign(2023)}")
# Usage:
regular_member = FamilyMember("John Doe", "1980-01-01", "Male")
royal_member = RoyalFamilyMember("Elizabeth", "1926-04-21", "Female", "Queen", 1952)
print_family_member_info(regular_member)
print_family_member_info(royal_member)
This print_family_member_info
function can handle both regular FamilyMember
objects and RoyalFamilyMember
objects, demonstrating polymorphism in action.
6. Building the Tree Structure
Now that we have our basic classes set up, let’s create a class to represent the entire family tree:
class FamilyTree:
def __init__(self, root):
self.root = root
def add_child(self, parent, child):
parent.add_child(child)
def add_spouse(self, person1, person2):
person1.add_spouse(person2)
def find_person(self, name):
return self._find_person_recursive(self.root, name)
def _find_person_recursive(self, current, name):
if current.get_name() == name:
return current
for child in current.get_children():
result = self._find_person_recursive(child, name)
if result:
return result
return None
def print_tree(self):
self._print_tree_recursive(self.root, 0)
def _print_tree_recursive(self, current, level):
print(" " * level + current.get_name())
for child in current.get_children():
self._print_tree_recursive(child, level + 1)
This FamilyTree
class provides methods for adding children and spouses, finding a person by name, and printing the entire tree structure.
7. Implementing Methods for Tree Traversal and Analysis
With our tree structure in place, we can now implement methods to traverse the tree and perform various analyses. Here are a few examples:
class FamilyTree:
# ... previous methods ...
def get_ancestors(self, person):
ancestors = []
self._get_ancestors_recursive(person, ancestors)
return ancestors
def _get_ancestors_recursive(self, person, ancestors):
for parent in person._parents:
ancestors.append(parent)
self._get_ancestors_recursive(parent, ancestors)
def get_descendants(self, person):
descendants = []
self._get_descendants_recursive(person, descendants)
return descendants
def _get_descendants_recursive(self, person, descendants):
for child in person.get_children():
descendants.append(child)
self._get_descendants_recursive(child, descendants)
def find_relationship(self, person1, person2):
if person1 == person2:
return "Same person"
if person2 in person1.get_children():
return "Parent-Child"
if person2 in person1._parents:
return "Child-Parent"
if person2 in person1.get_siblings():
return "Siblings"
if person2 == person1._spouse:
return "Spouses"
# More complex relationships can be determined by traversing the tree
return "Other/Unknown"
These methods allow us to find ancestors, descendants, and determine relationships between family members.
8. A Practical Example: Building Your Family Tree
Let’s put all of this together and create a simple family tree:
# Create family members
grandpa = FamilyMember("Grandpa", "1930-01-01", "Male")
grandma = FamilyMember("Grandma", "1935-01-01", "Female")
dad = FamilyMember("Dad", "1960-01-01", "Male")
mom = FamilyMember("Mom", "1962-01-01", "Female")
child1 = FamilyMember("Child1", "1990-01-01", "Female")
child2 = FamilyMember("Child2", "1992-01-01", "Male")
# Create the family tree
family_tree = FamilyTree(grandpa)
# Add relationships
family_tree.add_spouse(grandpa, grandma)
family_tree.add_child(grandpa, dad)
family_tree.add_spouse(dad, mom)
family_tree.add_child(dad, child1)
family_tree.add_child(dad, child2)
# Print the tree
print("Family Tree:")
family_tree.print_tree()
# Find relationships
print("\nRelationships:")
print(f"Relationship between {grandpa.get_name()} and {dad.get_name()}: {family_tree.find_relationship(grandpa, dad)}")
print(f"Relationship between {child1.get_name()} and {child2.get_name()}: {family_tree.find_relationship(child1, child2)}")
# Get ancestors and descendants
print(f"\nAncestors of {child1.get_name()}:")
for ancestor in family_tree.get_ancestors(child1):
print(ancestor.get_name())
print(f"\nDescendants of {grandpa.get_name()}:")
for descendant in family_tree.get_descendants(grandpa):
print(descendant.get_name())
This example demonstrates how to create a family tree, add relationships, and use various methods to analyze the family structure.
9. Advanced Features and Enhancements
Now that we have a basic family tree structure, we can consider adding more advanced features:
- Data Persistence: Implement methods to save and load family trees from files or databases.
- Visualization: Create a graphical representation of the family tree using a library like Graphviz.
- Date Handling: Improve date handling to calculate ages, generational gaps, etc.
- Event Tracking: Add the ability to track important life events for each family member.
- Family Name Analysis: Implement methods to analyze the prevalence and inheritance of family names.
Here’s an example of how we might implement data persistence using JSON:
import json
from datetime import datetime
class FamilyTree:
# ... previous methods ...
def save_to_file(self, filename):
data = self._serialize_tree(self.root)
with open(filename, 'w') as f:
json.dump(data, f, indent=2)
def _serialize_tree(self, node):
data = {
"name": node.get_name(),
"birth_date": node.get_birth_date(),
"gender": node._gender,
"children": [self._serialize_tree(child) for child in node.get_children()]
}
if node._spouse:
data["spouse"] = node._spouse.get_name()
return data
@classmethod
def load_from_file(cls, filename):
with open(filename, 'r') as f:
data = json.load(f)
root = cls._deserialize_tree(data)
return cls(root)
@classmethod
def _deserialize_tree(cls, data):
node = FamilyMember(data["name"], data["birth_date"], data["gender"])
for child_data in data["children"]:
child = cls._deserialize_tree(child_data)
node.add_child(child)
return node
# Usage:
family_tree.save_to_file("family_tree.json")
loaded_tree = FamilyTree.load_from_file("family_tree.json")
This implementation allows you to save your family tree to a JSON file and load it back, enabling data persistence across different sessions.
10. Conclusion
In this comprehensive guide, we’ve explored how to use Object-Oriented Programming principles to create a digital representation of a family tree. We’ve covered key OOP concepts such as classes, inheritance, encapsulation, and polymorphism, and applied them to the domain of genealogy.
By implementing a family tree using OOP, we’ve not only created a practical tool for organizing family history but also demonstrated how programming concepts can be applied to real-world scenarios. This approach provides a solid foundation for further enhancements and can be extended to more complex genealogical applications.
Remember, the power of OOP lies in its ability to model complex relationships and behaviors in an intuitive and extensible way. As you continue to develop your programming skills, look for opportunities to apply these principles to other domains and projects.
Happy coding, and may your family tree flourish in both the digital and real worlds!