How to Prepare for Multiple Rounds of Interviews: A Comprehensive Guide
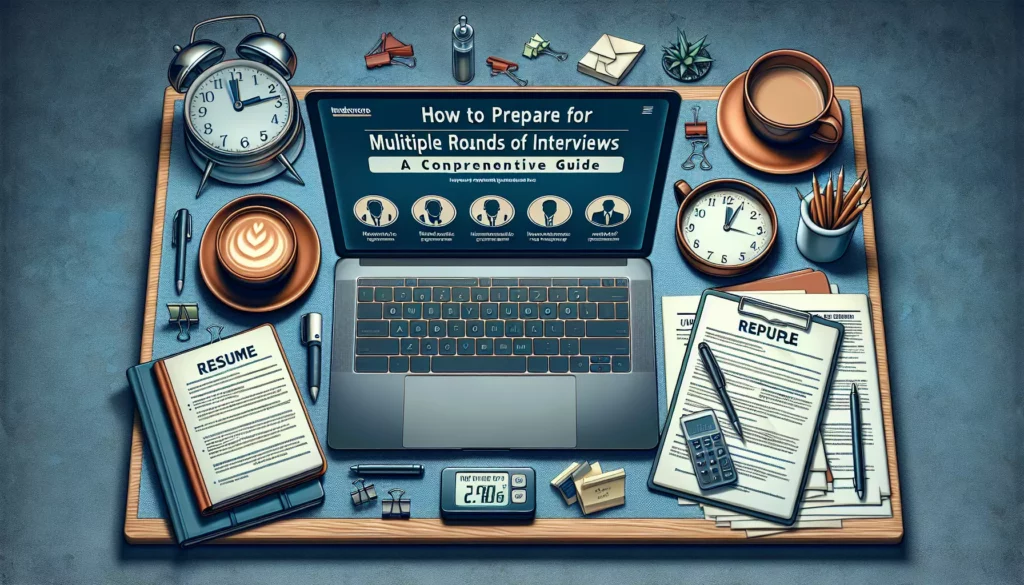
In today’s competitive job market, especially in the tech industry, it’s common for companies to conduct multiple rounds of interviews before making a final hiring decision. This process can be both exciting and daunting for job seekers. Whether you’re aiming for a position at a FAANG company (Facebook, Amazon, Apple, Netflix, Google) or any other tech giant, being well-prepared for each stage of the interview process is crucial. In this comprehensive guide, we’ll walk you through the steps to effectively prepare for multiple rounds of interviews, with a focus on technical roles in the software development field.
Understanding the Multi-Round Interview Process
Before diving into preparation strategies, it’s essential to understand why companies use multiple rounds of interviews and what each round typically entails.
Why Companies Use Multiple Interview Rounds
- To assess different aspects of a candidate’s skills and personality
- To involve various stakeholders in the hiring process
- To ensure a good fit for both the role and the company culture
- To thoroughly evaluate technical skills and problem-solving abilities
Common Interview Rounds in Tech Companies
- Initial Phone Screen or Online Assessment
- Technical Phone Interview
- On-Site Interviews (multiple rounds)
- Behavioral Interviews
- System Design Interview (for more senior roles)
- Final Interview with Leadership
Preparing for Each Round of Interviews
1. Initial Phone Screen or Online Assessment
The first round is often a brief phone call with a recruiter or an online coding assessment. Here’s how to prepare:
- Research the company thoroughly
- Review your resume and be prepared to discuss your experience
- Practice basic coding problems on platforms like AlgoCademy
- Familiarize yourself with online coding environments
For online assessments:
// Example of a typical online assessment problem
function findMissingNumber(nums) {
const n = nums.length + 1;
const expectedSum = (n * (n + 1)) / 2;
const actualSum = nums.reduce((sum, num) => sum + num, 0);
return expectedSum - actualSum;
}
// Test the function
console.log(findMissingNumber([3, 0, 1, 4, 6, 2])); // Output: 5
2. Technical Phone Interview
This round usually involves solving coding problems in real-time while explaining your thought process. To prepare:
- Practice coding problems daily
- Focus on data structures and algorithms
- Work on explaining your thought process clearly
- Set up a comfortable environment for the call
Example of a technical phone interview question:
// Implement a function to reverse a linked list
class ListNode {
constructor(val = 0, next = null) {
this.val = val;
this.next = next;
}
}
function reverseLinkedList(head) {
let prev = null;
let current = head;
while (current !== null) {
let nextTemp = current.next;
current.next = prev;
prev = current;
current = nextTemp;
}
return prev;
}
// Test the function
let head = new ListNode(1);
head.next = new ListNode(2);
head.next.next = new ListNode(3);
console.log(reverseLinkedList(head)); // Output: ListNode { val: 3, next: ListNode { val: 2, next: ListNode { val: 1, next: null } } }
3. On-Site Interviews
On-site interviews often consist of multiple rounds, each focusing on different aspects of your skills. Preparation tips include:
- Review and practice a wide range of algorithmic problems
- Be prepared to write code on a whiteboard or shared document
- Practice explaining your problem-solving approach
- Be ready to optimize your solutions for time and space complexity
Example of an on-site coding question:
// Implement a function to find the longest palindromic substring in a given string
function longestPalindromicSubstring(s) {
if (!s || s.length < 2) return s;
let start = 0, maxLength = 1;
function expandAroundCenter(left, right) {
while (left >= 0 && right < s.length && s[left] === s[right]) {
if (right - left + 1 > maxLength) {
start = left;
maxLength = right - left + 1;
}
left--;
right++;
}
}
for (let i = 0; i < s.length; i++) {
expandAroundCenter(i, i); // Odd length palindromes
expandAroundCenter(i, i + 1); // Even length palindromes
}
return s.substring(start, start + maxLength);
}
// Test the function
console.log(longestPalindromicSubstring("babad")); // Output: "bab" or "aba"
console.log(longestPalindromicSubstring("cbbd")); // Output: "bb"
4. Behavioral Interviews
Behavioral interviews assess your soft skills and how you handle various situations. To prepare:
- Use the STAR method (Situation, Task, Action, Result) to structure your answers
- Prepare examples of past experiences that demonstrate leadership, teamwork, and problem-solving
- Practice common behavioral questions
- Research the company’s values and culture
Example behavioral question:
“Tell me about a time when you had to work on a project with a difficult team member. How did you handle the situation?”
5. System Design Interview (for more senior roles)
System design interviews evaluate your ability to design large-scale systems. Preparation tips include:
- Study system design fundamentals (scalability, load balancing, caching, etc.)
- Practice designing common systems (e.g., URL shortener, social media feed)
- Understand trade-offs in system design decisions
- Be prepared to estimate system requirements and capacity
Example system design question:
“Design a distributed key-value store that can handle millions of concurrent users.”
6. Final Interview with Leadership
This round often focuses on your overall fit with the company. To prepare:
- Review the company’s mission, vision, and recent news
- Prepare thoughtful questions about the company’s future and your potential role
- Be ready to discuss your long-term career goals
- Practice articulating why you’re the best fit for the position
General Tips for Multiple Interview Rounds
1. Consistency is Key
Maintain consistency in your responses across all interview rounds. Interviewers often compare notes, so conflicting information can raise red flags.
2. Keep Your Energy Up
Multiple rounds of interviews can be exhausting. Make sure to:
- Get enough rest before each interview
- Stay hydrated and eat well
- Take short breaks between rounds if possible
- Maintain a positive attitude throughout the process
3. Continuous Learning
Use the time between interview rounds to:
- Review and improve on areas where you felt less confident
- Research any topics or technologies mentioned during previous rounds
- Practice more coding problems or system design questions
4. Seek Feedback
If possible, ask for feedback after each round. This can help you improve for subsequent interviews and show your eagerness to learn and grow.
5. Follow Up
Send a thank-you email after each interview round. This is an opportunity to:
- Express your appreciation for the interviewer’s time
- Reiterate your interest in the position
- Briefly address any points you feel you could have explained better during the interview
Leveraging AlgoCademy for Interview Preparation
AlgoCademy is an excellent resource for preparing for technical interviews, especially for coding and algorithmic problems. Here’s how you can make the most of it:
1. Practice Coding Problems
Use AlgoCademy’s extensive problem set to practice a wide range of coding challenges. Focus on:
- Array and string manipulation
- Linked lists and trees
- Graph algorithms
- Dynamic programming
- Sorting and searching algorithms
2. Utilize the AI-Powered Assistance
Take advantage of AlgoCademy’s AI-powered hints and explanations to understand problem-solving approaches better. This can help you develop a structured way of tackling new problems during interviews.
3. Time Your Problem-Solving
Practice solving problems within time constraints to simulate real interview conditions. Most technical interviews have a time limit, so getting comfortable with this pressure is crucial.
4. Review and Learn from Solutions
After solving a problem, review the provided solutions and explanations. Understanding multiple approaches to the same problem can greatly enhance your problem-solving skills.
5. Participate in Mock Interviews
If available, use AlgoCademy’s mock interview feature to simulate real interview scenarios. This can help you get comfortable with explaining your thought process while coding.
Conclusion
Preparing for multiple rounds of interviews, especially for technical roles at top tech companies, requires dedication, practice, and a structured approach. By understanding the purpose of each interview round and thoroughly preparing for them, you can significantly increase your chances of success.
Remember that the interview process is not just about showcasing your technical skills, but also about demonstrating your problem-solving abilities, communication skills, and cultural fit. Stay confident, be yourself, and view each round as an opportunity to learn and improve.
Leverage resources like AlgoCademy to hone your coding skills, and don’t forget to balance technical preparation with soft skills development. With thorough preparation and the right mindset, you’ll be well-equipped to tackle multiple rounds of interviews and land your dream job in the tech industry.
Good luck with your interviews!