How to Turn Your Code Duplication into a Cloning Experiment
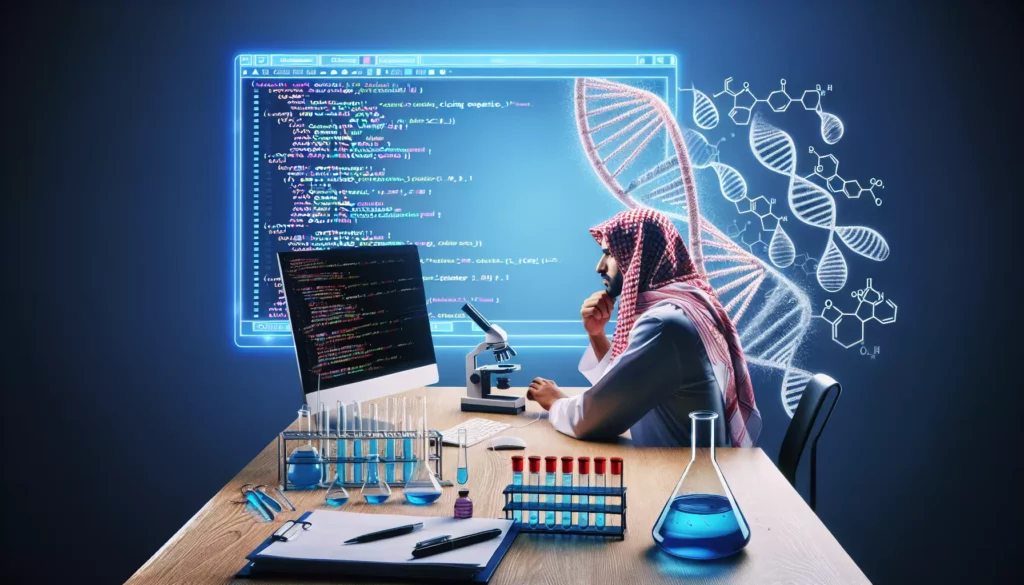
In the world of programming, code duplication is often seen as a cardinal sin. It’s the bane of maintainability, the nemesis of readability, and the arch-enemy of efficiency. But what if we told you that your code duplication could be the key to unlocking a fascinating journey into the realm of software cloning? Welcome to AlgoCademy’s guide on how to transform your redundant code into a thrilling cloning experiment!
Understanding Code Duplication
Before we dive into the exciting world of code cloning, let’s take a moment to understand what code duplication is and why it’s generally frowned upon in the programming community.
Code duplication occurs when identical or very similar code snippets appear in multiple places within a codebase. This can happen for various reasons:
- Copy-pasting code for quick solutions
- Lack of abstraction or modularization
- Time constraints leading to shortcuts
- Insufficient knowledge of existing codebase
While duplication might seem harmless at first, it can lead to several issues:
- Increased maintenance burden
- Higher risk of bugs (fix in one place, forget in another)
- Reduced code readability
- Bloated codebase size
However, today we’re going to look at code duplication from a different angle. Instead of immediately refactoring it away, we’re going to use it as a springboard for learning about code cloning techniques.
The Cloning Experiment: From Duplication to Replication
Now that we understand the nature of code duplication, let’s embark on our cloning experiment. We’ll take a step-by-step approach to transform our duplicated code into a sophisticated cloning mechanism.
Step 1: Identify the Duplicated Code
The first step in our experiment is to identify the duplicated code. Let’s say we have a simple function that calculates the area of a rectangle, and we’ve inadvertently duplicated it across multiple files:
// File: geometry_utils.js
function calculateRectangleArea(width, height) {
return width * height;
}
// File: shape_calculator.js
function calculateRectangleArea(width, height) {
return width * height;
}
// File: area_computer.js
function calculateRectangleArea(width, height) {
return width * height;
}
Step 2: Analyze the Duplication
Before we start cloning, let’s analyze our duplication. We can see that:
- The function name is identical across all instances
- The parameters are the same
- The implementation is identical
This is a perfect candidate for our cloning experiment!
Step 3: Create a Cloneable Template
Now, let’s create a template that we can use for cloning. We’ll use a higher-order function to generate our cloneable function:
function createAreaCalculator(shape) {
return function(dimension1, dimension2) {
if (shape === 'rectangle') {
return dimension1 * dimension2;
} else if (shape === 'triangle') {
return 0.5 * dimension1 * dimension2;
}
// Add more shapes as needed
}
}
Step 4: Implement the Cloning Mechanism
With our template in place, we can now implement a cloning mechanism. We’ll use JavaScript’s ability to create functions dynamically:
function cloneAreaCalculator(shape) {
const calculator = createAreaCalculator(shape);
const clonedCalculator = function(...args) {
console.log(`Calculating area for ${shape}`);
return calculator(...args);
}
return clonedCalculator;
}
Step 5: Use the Cloning Mechanism
Now we can use our cloning mechanism to create specific area calculators:
const calculateRectangleArea = cloneAreaCalculator('rectangle');
const calculateTriangleArea = cloneAreaCalculator('triangle');
console.log(calculateRectangleArea(5, 10)); // Output: Calculating area for rectangle \n 50
console.log(calculateTriangleArea(5, 10)); // Output: Calculating area for triangle \n 25
Benefits of Our Cloning Experiment
By transforming our code duplication into a cloning experiment, we’ve gained several benefits:
- Reduced Duplication: We’ve eliminated the need for multiple copies of similar functions.
- Increased Flexibility: We can easily add new shapes without duplicating code.
- Improved Maintainability: Changes to the core calculation logic only need to be made in one place.
- Enhanced Logging: We’ve added logging capabilities without cluttering the main calculation logic.
- Learning Opportunity: We’ve explored concepts like higher-order functions and closures.
Taking the Experiment Further
Our cloning experiment doesn’t have to stop here. We can take it further by exploring more advanced concepts:
Prototype-based Cloning
We can use JavaScript’s prototype-based inheritance to create more sophisticated clones:
function AreaCalculator(shape) {
this.shape = shape;
}
AreaCalculator.prototype.calculate = function(dimension1, dimension2) {
if (this.shape === 'rectangle') {
return dimension1 * dimension2;
} else if (this.shape === 'triangle') {
return 0.5 * dimension1 * dimension2;
}
}
function cloneAreaCalculator(shape) {
return new AreaCalculator(shape);
}
const rectangleCalculator = cloneAreaCalculator('rectangle');
console.log(rectangleCalculator.calculate(5, 10)); // Output: 50
Object.create() for Cloning
We can use Object.create()
to create clones with shared prototypes:
const areaCalculatorProto = {
calculate: function(dimension1, dimension2) {
if (this.shape === 'rectangle') {
return dimension1 * dimension2;
} else if (this.shape === 'triangle') {
return 0.5 * dimension1 * dimension2;
}
}
};
function cloneAreaCalculator(shape) {
const calculator = Object.create(areaCalculatorProto);
calculator.shape = shape;
return calculator;
}
const triangleCalculator = cloneAreaCalculator('triangle');
console.log(triangleCalculator.calculate(5, 10)); // Output: 25
ES6 Class-based Cloning
We can use ES6 classes to create a more structured cloning system:
class AreaCalculator {
constructor(shape) {
this.shape = shape;
}
calculate(dimension1, dimension2) {
if (this.shape === 'rectangle') {
return dimension1 * dimension2;
} else if (this.shape === 'triangle') {
return 0.5 * dimension1 * dimension2;
}
}
}
function cloneAreaCalculator(shape) {
return new AreaCalculator(shape);
}
const circleCalculator = cloneAreaCalculator('circle');
AreaCalculator.prototype.calculate = function(radius) {
if (this.shape === 'circle') {
return Math.PI * radius * radius;
}
// Fallback to original implementation
return this.calculate.apply(this, arguments);
}
console.log(circleCalculator.calculate(5)); // Output: 78.53981633974483
Real-world Applications of Code Cloning
While our experiment has been fun and educational, you might be wondering about the real-world applications of code cloning. Here are some scenarios where code cloning techniques can be valuable:
1. Plugin Systems
Many software applications use plugin systems to extend functionality. Code cloning techniques can be used to create a base plugin class that new plugins can clone and customize.
class Plugin {
constructor(name) {
this.name = name;
}
initialize() {
console.log(`Initializing ${this.name} plugin`);
}
execute() {
console.log(`Executing ${this.name} plugin`);
}
}
function clonePlugin(name) {
return new Plugin(name);
}
const loggingPlugin = clonePlugin('Logging');
loggingPlugin.execute = function() {
console.log(`Custom execution for ${this.name} plugin`);
}
loggingPlugin.initialize(); // Output: Initializing Logging plugin
loggingPlugin.execute(); // Output: Custom execution for Logging plugin
2. Factory Pattern Implementation
The Factory pattern is a creational design pattern that uses factory methods to deal with the problem of creating objects without having to specify the exact class of the object that will be created. Code cloning can be used to implement this pattern:
class Shape {
constructor(type) {
this.type = type;
}
draw() {
console.log(`Drawing a ${this.type}`);
}
}
class ShapeFactory {
static createShape(type) {
return new Shape(type);
}
}
const circle = ShapeFactory.createShape('circle');
circle.draw(); // Output: Drawing a circle
const square = ShapeFactory.createShape('square');
square.draw(); // Output: Drawing a square
3. Prototype Pattern
The Prototype pattern is used when the type of objects to create is determined by a prototypical instance, which is cloned to produce new objects. This is particularly useful when the cost of creating a new object is more expensive than copying an existing one:
const carPrototype = {
start: function() {
console.log('Starting the car...');
},
stop: function() {
console.log('Stopping the car...');
}
};
function createCar(model) {
const car = Object.create(carPrototype);
car.model = model;
return car;
}
const tesla = createCar('Tesla Model 3');
tesla.start(); // Output: Starting the car...
console.log(tesla.model); // Output: Tesla Model 3
const ford = createCar('Ford Mustang');
ford.stop(); // Output: Stopping the car...
console.log(ford.model); // Output: Ford Mustang
4. Mocking in Unit Tests
Code cloning techniques can be incredibly useful in creating mocks for unit testing. By cloning a base object or function, we can create mocks that behave similarly to the original but with controlled outputs:
class DatabaseConnection {
query(sql) {
// Actual database query logic
}
}
function createMockDatabase() {
const mockDb = Object.create(DatabaseConnection.prototype);
mockDb.query = function(sql) {
console.log(`Mock query executed: ${sql}`);
return [{ id: 1, name: 'Test User' }];
}
return mockDb;
}
// In a test file
const mockDb = createMockDatabase();
const result = mockDb.query('SELECT * FROM users');
console.log(result); // Output: [{ id: 1, name: 'Test User' }]
Ethical Considerations in Code Cloning
As we explore the world of code cloning, it’s important to consider the ethical implications of these techniques. While cloning can be a powerful tool for learning and solving certain problems, it’s crucial to use it responsibly:
1. Intellectual Property Rights
When cloning code, always ensure that you have the right to use and modify the original code. If you’re working with open-source software, make sure you comply with the license terms.
2. Attribution
If you’re cloning code from an external source, it’s good practice to provide attribution to the original author or source.
3. Code Quality
Cloning should not be used as a shortcut to avoid writing quality code. Always strive to understand the code you’re cloning and ensure it meets your project’s quality standards.
4. Security Implications
Be cautious when cloning code, especially from untrusted sources. Cloned code could potentially contain security vulnerabilities or malicious elements.
Conclusion: From Duplication to Innovation
We’ve come a long way from our initial code duplication. By approaching duplication as an opportunity for experimentation, we’ve explored various cloning techniques and their real-world applications. We’ve seen how cloning can be used to create flexible, maintainable code structures that can adapt to changing requirements.
Remember, the goal of this experiment wasn’t to encourage code duplication, but to show how we can learn from it and transform it into something more powerful and flexible. In your journey as a programmer, always be on the lookout for opportunities to turn challenges into learning experiences.
As you continue your coding education with AlgoCademy, keep these cloning techniques in your toolbox. They can be particularly useful when you’re tackling complex algorithmic problems or designing systems that require flexibility and extensibility.
Happy coding, and may your cloning experiments lead to exciting discoveries!